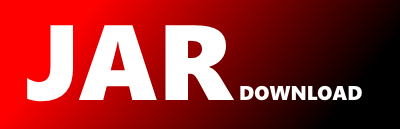
lnrpc.Peer.scala Maven / Gradle / Ivy
The newest version!
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package lnrpc
import org.bitcoins.lnd.rpc.LndUtils._
/** @param pubKey
* The identity pubkey of the peer
* @param address
* Network address of the peer; eg `127.0.0.1:10011`
* @param bytesSent
* Bytes of data transmitted to this peer
* @param bytesRecv
* Bytes of data transmitted from this peer
* @param satSent
* Satoshis sent to this peer
* @param satRecv
* Satoshis received from this peer
* @param inbound
* A channel is inbound if the counterparty initiated the channel
* @param pingTime
* Ping time to this peer
* @param syncType
* The type of sync we are currently performing with this peer.
* @param features
* Features advertised by the remote peer in their init message.
* @param errors
*
* The latest errors received from our peer with timestamps, limited to the 10
* most recent errors. These errors are tracked across peer connections, but
* are not persisted across lnd restarts. Note that these errors are only
* stored for peers that we have channels open with, to prevent peers from
* spamming us with errors at no cost.
* @param flapCount
*
* The number of times we have recorded this peer going offline or coming
* online, recorded across restarts. Note that this value is decreased over
* time if the peer has not recently flapped, so that we can forgive peers
* with historically high flap counts.
* @param lastFlapNs
*
* The timestamp of the last flap we observed for this peer. If this value is
* zero, we have not observed any flaps for this peer.
* @param lastPingPayload
*
* The last ping payload the peer has sent to us.
*/
@SerialVersionUID(0L)
final case class Peer(
pubKey: _root_.scala.Predef.String = "",
address: _root_.scala.Predef.String = "",
bytesSent: org.bitcoins.core.number.UInt64 = lnrpc.Peer._typemapper_bytesSent.toCustom(0L),
bytesRecv: org.bitcoins.core.number.UInt64 = lnrpc.Peer._typemapper_bytesRecv.toCustom(0L),
satSent: _root_.scala.Long = 0L,
satRecv: _root_.scala.Long = 0L,
inbound: _root_.scala.Boolean = false,
pingTime: _root_.scala.Long = 0L,
syncType: lnrpc.Peer.SyncType = lnrpc.Peer.SyncType.UNKNOWN_SYNC,
features: _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt32, lnrpc.Feature] = _root_.scala.collection.immutable.Map.empty,
errors: _root_.scala.Seq[lnrpc.TimestampedError] = _root_.scala.Seq.empty,
flapCount: _root_.scala.Int = 0,
lastFlapNs: _root_.scala.Long = 0L,
lastPingPayload: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Peer] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = pubKey
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(1, __value)
}
};
{
val __value = address
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(3, __value)
}
};
{
val __value = lnrpc.Peer._typemapper_bytesSent.toBase(bytesSent)
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt64Size(4, __value)
}
};
{
val __value = lnrpc.Peer._typemapper_bytesRecv.toBase(bytesRecv)
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt64Size(5, __value)
}
};
{
val __value = satSent
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(6, __value)
}
};
{
val __value = satRecv
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(7, __value)
}
};
{
val __value = inbound
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(8, __value)
}
};
{
val __value = pingTime
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(9, __value)
}
};
{
val __value = syncType.value
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeEnumSize(10, __value)
}
};
features.foreach { __item =>
val __value = lnrpc.Peer._typemapper_features.toBase(__item)
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
errors.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = flapCount
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt32Size(13, __value)
}
};
{
val __value = lastFlapNs
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(14, __value)
}
};
{
val __value = lastPingPayload
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(15, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = pubKey
if (!__v.isEmpty) {
_output__.writeString(1, __v)
}
};
{
val __v = address
if (!__v.isEmpty) {
_output__.writeString(3, __v)
}
};
{
val __v = lnrpc.Peer._typemapper_bytesSent.toBase(bytesSent)
if (__v != 0L) {
_output__.writeUInt64(4, __v)
}
};
{
val __v = lnrpc.Peer._typemapper_bytesRecv.toBase(bytesRecv)
if (__v != 0L) {
_output__.writeUInt64(5, __v)
}
};
{
val __v = satSent
if (__v != 0L) {
_output__.writeInt64(6, __v)
}
};
{
val __v = satRecv
if (__v != 0L) {
_output__.writeInt64(7, __v)
}
};
{
val __v = inbound
if (__v != false) {
_output__.writeBool(8, __v)
}
};
{
val __v = pingTime
if (__v != 0L) {
_output__.writeInt64(9, __v)
}
};
{
val __v = syncType.value
if (__v != 0) {
_output__.writeEnum(10, __v)
}
};
features.foreach { __v =>
val __m = lnrpc.Peer._typemapper_features.toBase(__v)
_output__.writeTag(11, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
errors.foreach { __v =>
val __m = __v
_output__.writeTag(12, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = flapCount
if (__v != 0) {
_output__.writeInt32(13, __v)
}
};
{
val __v = lastFlapNs
if (__v != 0L) {
_output__.writeInt64(14, __v)
}
};
{
val __v = lastPingPayload
if (!__v.isEmpty) {
_output__.writeBytes(15, __v)
}
};
unknownFields.writeTo(_output__)
}
def withPubKey(__v: _root_.scala.Predef.String): Peer = copy(pubKey = __v)
def withAddress(__v: _root_.scala.Predef.String): Peer = copy(address = __v)
def withBytesSent(__v: org.bitcoins.core.number.UInt64): Peer = copy(bytesSent = __v)
def withBytesRecv(__v: org.bitcoins.core.number.UInt64): Peer = copy(bytesRecv = __v)
def withSatSent(__v: _root_.scala.Long): Peer = copy(satSent = __v)
def withSatRecv(__v: _root_.scala.Long): Peer = copy(satRecv = __v)
def withInbound(__v: _root_.scala.Boolean): Peer = copy(inbound = __v)
def withPingTime(__v: _root_.scala.Long): Peer = copy(pingTime = __v)
def withSyncType(__v: lnrpc.Peer.SyncType): Peer = copy(syncType = __v)
def clearFeatures = copy(features = _root_.scala.collection.immutable.Map.empty)
def addFeatures(__vs: (org.bitcoins.core.number.UInt32, lnrpc.Feature) *): Peer = addAllFeatures(__vs)
def addAllFeatures(__vs: Iterable[(org.bitcoins.core.number.UInt32, lnrpc.Feature)]): Peer = copy(features = features ++ __vs)
def withFeatures(__v: _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt32, lnrpc.Feature]): Peer = copy(features = __v)
def clearErrors = copy(errors = _root_.scala.Seq.empty)
def addErrors(__vs: lnrpc.TimestampedError *): Peer = addAllErrors(__vs)
def addAllErrors(__vs: Iterable[lnrpc.TimestampedError]): Peer = copy(errors = errors ++ __vs)
def withErrors(__v: _root_.scala.Seq[lnrpc.TimestampedError]): Peer = copy(errors = __v)
def withFlapCount(__v: _root_.scala.Int): Peer = copy(flapCount = __v)
def withLastFlapNs(__v: _root_.scala.Long): Peer = copy(lastFlapNs = __v)
def withLastPingPayload(__v: _root_.com.google.protobuf.ByteString): Peer = copy(lastPingPayload = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = pubKey
if (__t != "") __t else null
}
case 3 => {
val __t = address
if (__t != "") __t else null
}
case 4 => {
val __t = lnrpc.Peer._typemapper_bytesSent.toBase(bytesSent)
if (__t != 0L) __t else null
}
case 5 => {
val __t = lnrpc.Peer._typemapper_bytesRecv.toBase(bytesRecv)
if (__t != 0L) __t else null
}
case 6 => {
val __t = satSent
if (__t != 0L) __t else null
}
case 7 => {
val __t = satRecv
if (__t != 0L) __t else null
}
case 8 => {
val __t = inbound
if (__t != false) __t else null
}
case 9 => {
val __t = pingTime
if (__t != 0L) __t else null
}
case 10 => {
val __t = syncType.javaValueDescriptor
if (__t.getNumber() != 0) __t else null
}
case 11 => features.iterator.map(lnrpc.Peer._typemapper_features.toBase(_)).toSeq
case 12 => errors
case 13 => {
val __t = flapCount
if (__t != 0) __t else null
}
case 14 => {
val __t = lastFlapNs
if (__t != 0L) __t else null
}
case 15 => {
val __t = lastPingPayload
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PString(pubKey)
case 3 => _root_.scalapb.descriptors.PString(address)
case 4 => _root_.scalapb.descriptors.PLong(lnrpc.Peer._typemapper_bytesSent.toBase(bytesSent))
case 5 => _root_.scalapb.descriptors.PLong(lnrpc.Peer._typemapper_bytesRecv.toBase(bytesRecv))
case 6 => _root_.scalapb.descriptors.PLong(satSent)
case 7 => _root_.scalapb.descriptors.PLong(satRecv)
case 8 => _root_.scalapb.descriptors.PBoolean(inbound)
case 9 => _root_.scalapb.descriptors.PLong(pingTime)
case 10 => _root_.scalapb.descriptors.PEnum(syncType.scalaValueDescriptor)
case 11 => _root_.scalapb.descriptors.PRepeated(features.iterator.map(lnrpc.Peer._typemapper_features.toBase(_).toPMessage).toVector)
case 12 => _root_.scalapb.descriptors.PRepeated(errors.iterator.map(_.toPMessage).toVector)
case 13 => _root_.scalapb.descriptors.PInt(flapCount)
case 14 => _root_.scalapb.descriptors.PLong(lastFlapNs)
case 15 => _root_.scalapb.descriptors.PByteString(lastPingPayload)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: lnrpc.Peer.type = lnrpc.Peer
// @@protoc_insertion_point(GeneratedMessage[lnrpc.Peer])
}
object Peer extends scalapb.GeneratedMessageCompanion[lnrpc.Peer] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[lnrpc.Peer] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): lnrpc.Peer = {
var __pubKey: _root_.scala.Predef.String = ""
var __address: _root_.scala.Predef.String = ""
var __bytesSent: _root_.scala.Long = 0L
var __bytesRecv: _root_.scala.Long = 0L
var __satSent: _root_.scala.Long = 0L
var __satRecv: _root_.scala.Long = 0L
var __inbound: _root_.scala.Boolean = false
var __pingTime: _root_.scala.Long = 0L
var __syncType: lnrpc.Peer.SyncType = lnrpc.Peer.SyncType.UNKNOWN_SYNC
val __features: _root_.scala.collection.mutable.Builder[(org.bitcoins.core.number.UInt32, lnrpc.Feature), _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt32, lnrpc.Feature]] = _root_.scala.collection.immutable.Map.newBuilder[org.bitcoins.core.number.UInt32, lnrpc.Feature]
val __errors: _root_.scala.collection.immutable.VectorBuilder[lnrpc.TimestampedError] = new _root_.scala.collection.immutable.VectorBuilder[lnrpc.TimestampedError]
var __flapCount: _root_.scala.Int = 0
var __lastFlapNs: _root_.scala.Long = 0L
var __lastPingPayload: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__pubKey = _input__.readStringRequireUtf8()
case 26 =>
__address = _input__.readStringRequireUtf8()
case 32 =>
__bytesSent = _input__.readUInt64()
case 40 =>
__bytesRecv = _input__.readUInt64()
case 48 =>
__satSent = _input__.readInt64()
case 56 =>
__satRecv = _input__.readInt64()
case 64 =>
__inbound = _input__.readBool()
case 72 =>
__pingTime = _input__.readInt64()
case 80 =>
__syncType = lnrpc.Peer.SyncType.fromValue(_input__.readEnum())
case 90 =>
__features += lnrpc.Peer._typemapper_features.toCustom(_root_.scalapb.LiteParser.readMessage[lnrpc.Peer.FeaturesEntry](_input__))
case 98 =>
__errors += _root_.scalapb.LiteParser.readMessage[lnrpc.TimestampedError](_input__)
case 104 =>
__flapCount = _input__.readInt32()
case 112 =>
__lastFlapNs = _input__.readInt64()
case 122 =>
__lastPingPayload = _input__.readBytes()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
lnrpc.Peer(
pubKey = __pubKey,
address = __address,
bytesSent = lnrpc.Peer._typemapper_bytesSent.toCustom(__bytesSent),
bytesRecv = lnrpc.Peer._typemapper_bytesRecv.toCustom(__bytesRecv),
satSent = __satSent,
satRecv = __satRecv,
inbound = __inbound,
pingTime = __pingTime,
syncType = __syncType,
features = __features.result(),
errors = __errors.result(),
flapCount = __flapCount,
lastFlapNs = __lastFlapNs,
lastPingPayload = __lastPingPayload,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[lnrpc.Peer] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
lnrpc.Peer(
pubKey = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
address = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
bytesSent = lnrpc.Peer._typemapper_bytesSent.toCustom(__fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Long]).getOrElse(0L)),
bytesRecv = lnrpc.Peer._typemapper_bytesRecv.toCustom(__fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Long]).getOrElse(0L)),
satSent = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
satRecv = __fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
inbound = __fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).map(_.as[_root_.scala.Boolean]).getOrElse(false),
pingTime = __fieldsMap.get(scalaDescriptor.findFieldByNumber(9).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
syncType = lnrpc.Peer.SyncType.fromValue(__fieldsMap.get(scalaDescriptor.findFieldByNumber(10).get).map(_.as[_root_.scalapb.descriptors.EnumValueDescriptor]).getOrElse(lnrpc.Peer.SyncType.UNKNOWN_SYNC.scalaValueDescriptor).number),
features = __fieldsMap.get(scalaDescriptor.findFieldByNumber(11).get).map(_.as[_root_.scala.Seq[lnrpc.Peer.FeaturesEntry]]).getOrElse(_root_.scala.Seq.empty).iterator.map(lnrpc.Peer._typemapper_features.toCustom(_)).toMap,
errors = __fieldsMap.get(scalaDescriptor.findFieldByNumber(12).get).map(_.as[_root_.scala.Seq[lnrpc.TimestampedError]]).getOrElse(_root_.scala.Seq.empty),
flapCount = __fieldsMap.get(scalaDescriptor.findFieldByNumber(13).get).map(_.as[_root_.scala.Int]).getOrElse(0),
lastFlapNs = __fieldsMap.get(scalaDescriptor.findFieldByNumber(14).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
lastPingPayload = __fieldsMap.get(scalaDescriptor.findFieldByNumber(15).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = LightningProto.javaDescriptor.getMessageTypes().get(51)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = LightningProto.scalaDescriptor.messages(51)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 11 => __out = lnrpc.Peer.FeaturesEntry
case 12 => __out = lnrpc.TimestampedError
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.lnrpc.Peer.FeaturesEntry
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 10 => lnrpc.Peer.SyncType
}
}
lazy val defaultInstance = lnrpc.Peer(
pubKey = "",
address = "",
bytesSent = lnrpc.Peer._typemapper_bytesSent.toCustom(0L),
bytesRecv = lnrpc.Peer._typemapper_bytesRecv.toCustom(0L),
satSent = 0L,
satRecv = 0L,
inbound = false,
pingTime = 0L,
syncType = lnrpc.Peer.SyncType.UNKNOWN_SYNC,
features = _root_.scala.collection.immutable.Map.empty,
errors = _root_.scala.Seq.empty,
flapCount = 0,
lastFlapNs = 0L,
lastPingPayload = _root_.com.google.protobuf.ByteString.EMPTY
)
sealed abstract class SyncType(val value: _root_.scala.Int) extends _root_.scalapb.GeneratedEnum {
type EnumType = SyncType
def isUnknownSync: _root_.scala.Boolean = false
def isActiveSync: _root_.scala.Boolean = false
def isPassiveSync: _root_.scala.Boolean = false
def isPinnedSync: _root_.scala.Boolean = false
def companion: _root_.scalapb.GeneratedEnumCompanion[SyncType] = lnrpc.Peer.SyncType
final def asRecognized: _root_.scala.Option[lnrpc.Peer.SyncType.Recognized] = if (isUnrecognized) _root_.scala.None else _root_.scala.Some(this.asInstanceOf[lnrpc.Peer.SyncType.Recognized])
}
object SyncType extends _root_.scalapb.GeneratedEnumCompanion[SyncType] {
sealed trait Recognized extends SyncType
implicit def enumCompanion: _root_.scalapb.GeneratedEnumCompanion[SyncType] = this
/**
* Denotes that we cannot determine the peer's current sync type.
*/
@SerialVersionUID(0L)
case object UNKNOWN_SYNC extends SyncType(0) with SyncType.Recognized {
val index = 0
val name = "UNKNOWN_SYNC"
override def isUnknownSync: _root_.scala.Boolean = true
}
/**
* Denotes that we are actively receiving new graph updates from the peer.
*/
@SerialVersionUID(0L)
case object ACTIVE_SYNC extends SyncType(1) with SyncType.Recognized {
val index = 1
val name = "ACTIVE_SYNC"
override def isActiveSync: _root_.scala.Boolean = true
}
/**
* Denotes that we are not receiving new graph updates from the peer.
*/
@SerialVersionUID(0L)
case object PASSIVE_SYNC extends SyncType(2) with SyncType.Recognized {
val index = 2
val name = "PASSIVE_SYNC"
override def isPassiveSync: _root_.scala.Boolean = true
}
/**
* Denotes that this peer is pinned into an active sync.
*/
@SerialVersionUID(0L)
case object PINNED_SYNC extends SyncType(3) with SyncType.Recognized {
val index = 3
val name = "PINNED_SYNC"
override def isPinnedSync: _root_.scala.Boolean = true
}
@SerialVersionUID(0L)
final case class Unrecognized(unrecognizedValue: _root_.scala.Int) extends SyncType(unrecognizedValue) with _root_.scalapb.UnrecognizedEnum
lazy val values = scala.collection.immutable.Seq(UNKNOWN_SYNC, ACTIVE_SYNC, PASSIVE_SYNC, PINNED_SYNC)
def fromValue(__value: _root_.scala.Int): SyncType = __value match {
case 0 => UNKNOWN_SYNC
case 1 => ACTIVE_SYNC
case 2 => PASSIVE_SYNC
case 3 => PINNED_SYNC
case __other => Unrecognized(__other)
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.EnumDescriptor = lnrpc.Peer.javaDescriptor.getEnumTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.EnumDescriptor = lnrpc.Peer.scalaDescriptor.enums(0)
}
@SerialVersionUID(0L)
final case class FeaturesEntry(
key: org.bitcoins.core.number.UInt32 = lnrpc.Peer.FeaturesEntry._typemapper_key.toCustom(0),
value: _root_.scala.Option[lnrpc.Feature] = _root_.scala.None,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[FeaturesEntry] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = lnrpc.Peer.FeaturesEntry._typemapper_key.toBase(key)
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt32Size(1, __value)
}
};
if (value.isDefined) {
val __value = value.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = lnrpc.Peer.FeaturesEntry._typemapper_key.toBase(key)
if (__v != 0) {
_output__.writeUInt32(1, __v)
}
};
value.foreach { __v =>
val __m = __v
_output__.writeTag(2, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withKey(__v: org.bitcoins.core.number.UInt32): FeaturesEntry = copy(key = __v)
def getValue: lnrpc.Feature = value.getOrElse(lnrpc.Feature.defaultInstance)
def clearValue: FeaturesEntry = copy(value = _root_.scala.None)
def withValue(__v: lnrpc.Feature): FeaturesEntry = copy(value = Option(__v))
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = lnrpc.Peer.FeaturesEntry._typemapper_key.toBase(key)
if (__t != 0) __t else null
}
case 2 => value.orNull
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PInt(lnrpc.Peer.FeaturesEntry._typemapper_key.toBase(key))
case 2 => value.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: lnrpc.Peer.FeaturesEntry.type = lnrpc.Peer.FeaturesEntry
// @@protoc_insertion_point(GeneratedMessage[lnrpc.Peer.FeaturesEntry])
}
object FeaturesEntry extends scalapb.GeneratedMessageCompanion[lnrpc.Peer.FeaturesEntry] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[lnrpc.Peer.FeaturesEntry] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): lnrpc.Peer.FeaturesEntry = {
var __key: _root_.scala.Int = 0
var __value: _root_.scala.Option[lnrpc.Feature] = _root_.scala.None
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 8 =>
__key = _input__.readUInt32()
case 18 =>
__value = Option(__value.fold(_root_.scalapb.LiteParser.readMessage[lnrpc.Feature](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
lnrpc.Peer.FeaturesEntry(
key = lnrpc.Peer.FeaturesEntry._typemapper_key.toCustom(__key),
value = __value,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[lnrpc.Peer.FeaturesEntry] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
lnrpc.Peer.FeaturesEntry(
key = lnrpc.Peer.FeaturesEntry._typemapper_key.toCustom(__fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Int]).getOrElse(0)),
value = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).flatMap(_.as[_root_.scala.Option[lnrpc.Feature]])
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = lnrpc.Peer.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = lnrpc.Peer.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 2 => __out = lnrpc.Feature
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = lnrpc.Peer.FeaturesEntry(
key = lnrpc.Peer.FeaturesEntry._typemapper_key.toCustom(0),
value = _root_.scala.None
)
implicit class FeaturesEntryLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.Peer.FeaturesEntry]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, lnrpc.Peer.FeaturesEntry](_l) {
def key: _root_.scalapb.lenses.Lens[UpperPB, org.bitcoins.core.number.UInt32] = field(_.key)((c_, f_) => c_.copy(key = f_))
def value: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.Feature] = field(_.getValue)((c_, f_) => c_.copy(value = Option(f_)))
def optionalValue: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[lnrpc.Feature]] = field(_.value)((c_, f_) => c_.copy(value = f_))
}
final val KEY_FIELD_NUMBER = 1
final val VALUE_FIELD_NUMBER = 2
@transient
private[lnrpc] val _typemapper_key: _root_.scalapb.TypeMapper[_root_.scala.Int, org.bitcoins.core.number.UInt32] = implicitly[_root_.scalapb.TypeMapper[_root_.scala.Int, org.bitcoins.core.number.UInt32]]
@transient
implicit val keyValueMapper: _root_.scalapb.TypeMapper[lnrpc.Peer.FeaturesEntry, (org.bitcoins.core.number.UInt32, lnrpc.Feature)] =
_root_.scalapb.TypeMapper[lnrpc.Peer.FeaturesEntry, (org.bitcoins.core.number.UInt32, lnrpc.Feature)](__m => (__m.key, __m.getValue))(__p => lnrpc.Peer.FeaturesEntry(__p._1, Some(__p._2)))
def of(
key: org.bitcoins.core.number.UInt32,
value: _root_.scala.Option[lnrpc.Feature]
): _root_.lnrpc.Peer.FeaturesEntry = _root_.lnrpc.Peer.FeaturesEntry(
key,
value
)
// @@protoc_insertion_point(GeneratedMessageCompanion[lnrpc.Peer.FeaturesEntry])
}
implicit class PeerLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.Peer]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, lnrpc.Peer](_l) {
def pubKey: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.pubKey)((c_, f_) => c_.copy(pubKey = f_))
def address: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.address)((c_, f_) => c_.copy(address = f_))
def bytesSent: _root_.scalapb.lenses.Lens[UpperPB, org.bitcoins.core.number.UInt64] = field(_.bytesSent)((c_, f_) => c_.copy(bytesSent = f_))
def bytesRecv: _root_.scalapb.lenses.Lens[UpperPB, org.bitcoins.core.number.UInt64] = field(_.bytesRecv)((c_, f_) => c_.copy(bytesRecv = f_))
def satSent: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.satSent)((c_, f_) => c_.copy(satSent = f_))
def satRecv: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.satRecv)((c_, f_) => c_.copy(satRecv = f_))
def inbound: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.inbound)((c_, f_) => c_.copy(inbound = f_))
def pingTime: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.pingTime)((c_, f_) => c_.copy(pingTime = f_))
def syncType: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.Peer.SyncType] = field(_.syncType)((c_, f_) => c_.copy(syncType = f_))
def features: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt32, lnrpc.Feature]] = field(_.features)((c_, f_) => c_.copy(features = f_))
def errors: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[lnrpc.TimestampedError]] = field(_.errors)((c_, f_) => c_.copy(errors = f_))
def flapCount: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.flapCount)((c_, f_) => c_.copy(flapCount = f_))
def lastFlapNs: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.lastFlapNs)((c_, f_) => c_.copy(lastFlapNs = f_))
def lastPingPayload: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.lastPingPayload)((c_, f_) => c_.copy(lastPingPayload = f_))
}
final val PUB_KEY_FIELD_NUMBER = 1
final val ADDRESS_FIELD_NUMBER = 3
final val BYTES_SENT_FIELD_NUMBER = 4
final val BYTES_RECV_FIELD_NUMBER = 5
final val SAT_SENT_FIELD_NUMBER = 6
final val SAT_RECV_FIELD_NUMBER = 7
final val INBOUND_FIELD_NUMBER = 8
final val PING_TIME_FIELD_NUMBER = 9
final val SYNC_TYPE_FIELD_NUMBER = 10
final val FEATURES_FIELD_NUMBER = 11
final val ERRORS_FIELD_NUMBER = 12
final val FLAP_COUNT_FIELD_NUMBER = 13
final val LAST_FLAP_NS_FIELD_NUMBER = 14
final val LAST_PING_PAYLOAD_FIELD_NUMBER = 15
@transient
private[lnrpc] val _typemapper_bytesSent: _root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64] = implicitly[_root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64]]
@transient
private[lnrpc] val _typemapper_bytesRecv: _root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64] = implicitly[_root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64]]
@transient
private[lnrpc] val _typemapper_features: _root_.scalapb.TypeMapper[lnrpc.Peer.FeaturesEntry, (org.bitcoins.core.number.UInt32, lnrpc.Feature)] = implicitly[_root_.scalapb.TypeMapper[lnrpc.Peer.FeaturesEntry, (org.bitcoins.core.number.UInt32, lnrpc.Feature)]]
def of(
pubKey: _root_.scala.Predef.String,
address: _root_.scala.Predef.String,
bytesSent: org.bitcoins.core.number.UInt64,
bytesRecv: org.bitcoins.core.number.UInt64,
satSent: _root_.scala.Long,
satRecv: _root_.scala.Long,
inbound: _root_.scala.Boolean,
pingTime: _root_.scala.Long,
syncType: lnrpc.Peer.SyncType,
features: _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt32, lnrpc.Feature],
errors: _root_.scala.Seq[lnrpc.TimestampedError],
flapCount: _root_.scala.Int,
lastFlapNs: _root_.scala.Long,
lastPingPayload: _root_.com.google.protobuf.ByteString
): _root_.lnrpc.Peer = _root_.lnrpc.Peer(
pubKey,
address,
bytesSent,
bytesRecv,
satSent,
satRecv,
inbound,
pingTime,
syncType,
features,
errors,
flapCount,
lastFlapNs,
lastPingPayload
)
// @@protoc_insertion_point(GeneratedMessageCompanion[lnrpc.Peer])
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy