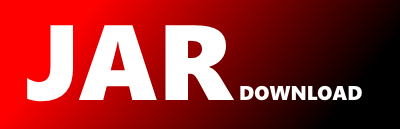
lnrpc.SendRequest.scala Maven / Gradle / Ivy
The newest version!
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package lnrpc
import org.bitcoins.lnd.rpc.LndUtils._
/** @param dest
*
* The identity pubkey of the payment recipient. When using REST, this field
* must be encoded as base64.
* @param destString
*
* The hex-encoded identity pubkey of the payment recipient. Deprecated now
* that the REST gateway supports base64 encoding of bytes fields.
* @param amt
*
* The amount to send expressed in satoshis.
*
* The fields amt and amt_msat are mutually exclusive.
* @param amtMsat
*
* The amount to send expressed in millisatoshis.
*
* The fields amt and amt_msat are mutually exclusive.
* @param paymentHash
*
* The hash to use within the payment's HTLC. When using REST, this field
* must be encoded as base64.
* @param paymentHashString
*
* The hex-encoded hash to use within the payment's HTLC. Deprecated now
* that the REST gateway supports base64 encoding of bytes fields.
* @param paymentRequest
*
* A bare-bones invoice for a payment within the Lightning Network. With the
* details of the invoice, the sender has all the data necessary to send a
* payment to the recipient.
* @param finalCltvDelta
*
* The CLTV delta from the current height that should be used to set the
* timelock for the final hop.
* @param feeLimit
*
* The maximum number of satoshis that will be paid as a fee of the payment.
* This value can be represented either as a percentage of the amount being
* sent, or as a fixed amount of the maximum fee the user is willing the pay to
* send the payment. If not specified, lnd will use a default value of 100%
* fees for small amounts (<=1k sat) or 5% fees for larger amounts.
* @param outgoingChanId
*
* The channel id of the channel that must be taken to the first hop. If zero,
* any channel may be used.
* @param lastHopPubkey
*
* The pubkey of the last hop of the route. If empty, any hop may be used.
* @param cltvLimit
*
* An optional maximum total time lock for the route. This should not exceed
* lnd's `--max-cltv-expiry` setting. If zero, then the value of
* `--max-cltv-expiry` is enforced.
* @param destCustomRecords
*
* An optional field that can be used to pass an arbitrary set of TLV records
* to a peer which understands the new records. This can be used to pass
* application specific data during the payment attempt. Record types are
* required to be in the custom range >= 65536. When using REST, the values
* must be encoded as base64.
* @param allowSelfPayment
* If set, circular payments to self are permitted.
* @param destFeatures
*
* Features assumed to be supported by the final node. All transitive feature
* dependencies must also be set properly. For a given feature bit pair, either
* optional or remote may be set, but not both. If this field is nil or empty,
* the router will try to load destination features from the graph as a
* fallback.
* @param paymentAddr
*
* The payment address of the generated invoice.
*/
@SerialVersionUID(0L)
final case class SendRequest(
dest: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
@scala.deprecated(message="Marked as deprecated in proto file", "") destString: _root_.scala.Predef.String = "",
amt: _root_.scala.Long = 0L,
amtMsat: _root_.scala.Long = 0L,
paymentHash: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
@scala.deprecated(message="Marked as deprecated in proto file", "") paymentHashString: _root_.scala.Predef.String = "",
paymentRequest: _root_.scala.Predef.String = "",
finalCltvDelta: _root_.scala.Int = 0,
feeLimit: _root_.scala.Option[lnrpc.FeeLimit] = _root_.scala.None,
outgoingChanId: org.bitcoins.core.number.UInt64 = lnrpc.SendRequest._typemapper_outgoingChanId.toCustom(0L),
lastHopPubkey: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
cltvLimit: org.bitcoins.core.number.UInt32 = lnrpc.SendRequest._typemapper_cltvLimit.toCustom(0),
destCustomRecords: _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString] = _root_.scala.collection.immutable.Map.empty,
allowSelfPayment: _root_.scala.Boolean = false,
destFeatures: _root_.scala.Seq[lnrpc.FeatureBit] = _root_.scala.Seq.empty,
paymentAddr: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[SendRequest] {
private[this] def destFeaturesSerializedSize = {
if (__destFeaturesSerializedSizeField == 0) __destFeaturesSerializedSizeField = {
var __s: _root_.scala.Int = 0
destFeatures.foreach(__i => __s += _root_.com.google.protobuf.CodedOutputStream.computeEnumSizeNoTag(__i.value))
__s
}
__destFeaturesSerializedSizeField
}
@transient private[this] var __destFeaturesSerializedSizeField: _root_.scala.Int = 0
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = dest
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = destString
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(2, __value)
}
};
{
val __value = amt
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(3, __value)
}
};
{
val __value = amtMsat
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(12, __value)
}
};
{
val __value = paymentHash
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(4, __value)
}
};
{
val __value = paymentHashString
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(5, __value)
}
};
{
val __value = paymentRequest
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(6, __value)
}
};
{
val __value = finalCltvDelta
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt32Size(7, __value)
}
};
if (feeLimit.isDefined) {
val __value = feeLimit.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
{
val __value = lnrpc.SendRequest._typemapper_outgoingChanId.toBase(outgoingChanId)
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt64Size(9, __value)
}
};
{
val __value = lastHopPubkey
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(13, __value)
}
};
{
val __value = lnrpc.SendRequest._typemapper_cltvLimit.toBase(cltvLimit)
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt32Size(10, __value)
}
};
destCustomRecords.foreach { __item =>
val __value = lnrpc.SendRequest._typemapper_destCustomRecords.toBase(__item)
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = allowSelfPayment
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(14, __value)
}
};
if (destFeatures.nonEmpty) {
val __localsize = destFeaturesSerializedSize
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__localsize) + __localsize
}
{
val __value = paymentAddr
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(16, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = dest
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = destString
if (!__v.isEmpty) {
_output__.writeString(2, __v)
}
};
{
val __v = amt
if (__v != 0L) {
_output__.writeInt64(3, __v)
}
};
{
val __v = paymentHash
if (!__v.isEmpty) {
_output__.writeBytes(4, __v)
}
};
{
val __v = paymentHashString
if (!__v.isEmpty) {
_output__.writeString(5, __v)
}
};
{
val __v = paymentRequest
if (!__v.isEmpty) {
_output__.writeString(6, __v)
}
};
{
val __v = finalCltvDelta
if (__v != 0) {
_output__.writeInt32(7, __v)
}
};
feeLimit.foreach { __v =>
val __m = __v
_output__.writeTag(8, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = lnrpc.SendRequest._typemapper_outgoingChanId.toBase(outgoingChanId)
if (__v != 0L) {
_output__.writeUInt64(9, __v)
}
};
{
val __v = lnrpc.SendRequest._typemapper_cltvLimit.toBase(cltvLimit)
if (__v != 0) {
_output__.writeUInt32(10, __v)
}
};
destCustomRecords.foreach { __v =>
val __m = lnrpc.SendRequest._typemapper_destCustomRecords.toBase(__v)
_output__.writeTag(11, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = amtMsat
if (__v != 0L) {
_output__.writeInt64(12, __v)
}
};
{
val __v = lastHopPubkey
if (!__v.isEmpty) {
_output__.writeBytes(13, __v)
}
};
{
val __v = allowSelfPayment
if (__v != false) {
_output__.writeBool(14, __v)
}
};
if (destFeatures.nonEmpty) {
_output__.writeTag(15, 2)
_output__.writeUInt32NoTag(destFeaturesSerializedSize)
destFeatures.foreach((_output__.writeEnumNoTag _).compose((_: lnrpc.FeatureBit).value))
};
{
val __v = paymentAddr
if (!__v.isEmpty) {
_output__.writeBytes(16, __v)
}
};
unknownFields.writeTo(_output__)
}
def withDest(__v: _root_.com.google.protobuf.ByteString): SendRequest = copy(dest = __v)
def withDestString(__v: _root_.scala.Predef.String): SendRequest = copy(destString = __v)
def withAmt(__v: _root_.scala.Long): SendRequest = copy(amt = __v)
def withAmtMsat(__v: _root_.scala.Long): SendRequest = copy(amtMsat = __v)
def withPaymentHash(__v: _root_.com.google.protobuf.ByteString): SendRequest = copy(paymentHash = __v)
def withPaymentHashString(__v: _root_.scala.Predef.String): SendRequest = copy(paymentHashString = __v)
def withPaymentRequest(__v: _root_.scala.Predef.String): SendRequest = copy(paymentRequest = __v)
def withFinalCltvDelta(__v: _root_.scala.Int): SendRequest = copy(finalCltvDelta = __v)
def getFeeLimit: lnrpc.FeeLimit = feeLimit.getOrElse(lnrpc.FeeLimit.defaultInstance)
def clearFeeLimit: SendRequest = copy(feeLimit = _root_.scala.None)
def withFeeLimit(__v: lnrpc.FeeLimit): SendRequest = copy(feeLimit = Option(__v))
def withOutgoingChanId(__v: org.bitcoins.core.number.UInt64): SendRequest = copy(outgoingChanId = __v)
def withLastHopPubkey(__v: _root_.com.google.protobuf.ByteString): SendRequest = copy(lastHopPubkey = __v)
def withCltvLimit(__v: org.bitcoins.core.number.UInt32): SendRequest = copy(cltvLimit = __v)
def clearDestCustomRecords = copy(destCustomRecords = _root_.scala.collection.immutable.Map.empty)
def addDestCustomRecords(__vs: (org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString) *): SendRequest = addAllDestCustomRecords(__vs)
def addAllDestCustomRecords(__vs: Iterable[(org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString)]): SendRequest = copy(destCustomRecords = destCustomRecords ++ __vs)
def withDestCustomRecords(__v: _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString]): SendRequest = copy(destCustomRecords = __v)
def withAllowSelfPayment(__v: _root_.scala.Boolean): SendRequest = copy(allowSelfPayment = __v)
def clearDestFeatures = copy(destFeatures = _root_.scala.Seq.empty)
def addDestFeatures(__vs: lnrpc.FeatureBit *): SendRequest = addAllDestFeatures(__vs)
def addAllDestFeatures(__vs: Iterable[lnrpc.FeatureBit]): SendRequest = copy(destFeatures = destFeatures ++ __vs)
def withDestFeatures(__v: _root_.scala.Seq[lnrpc.FeatureBit]): SendRequest = copy(destFeatures = __v)
def withPaymentAddr(__v: _root_.com.google.protobuf.ByteString): SendRequest = copy(paymentAddr = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = dest
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = destString
if (__t != "") __t else null
}
case 3 => {
val __t = amt
if (__t != 0L) __t else null
}
case 12 => {
val __t = amtMsat
if (__t != 0L) __t else null
}
case 4 => {
val __t = paymentHash
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 5 => {
val __t = paymentHashString
if (__t != "") __t else null
}
case 6 => {
val __t = paymentRequest
if (__t != "") __t else null
}
case 7 => {
val __t = finalCltvDelta
if (__t != 0) __t else null
}
case 8 => feeLimit.orNull
case 9 => {
val __t = lnrpc.SendRequest._typemapper_outgoingChanId.toBase(outgoingChanId)
if (__t != 0L) __t else null
}
case 13 => {
val __t = lastHopPubkey
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 10 => {
val __t = lnrpc.SendRequest._typemapper_cltvLimit.toBase(cltvLimit)
if (__t != 0) __t else null
}
case 11 => destCustomRecords.iterator.map(lnrpc.SendRequest._typemapper_destCustomRecords.toBase(_)).toSeq
case 14 => {
val __t = allowSelfPayment
if (__t != false) __t else null
}
case 15 => destFeatures.iterator.map(_.javaValueDescriptor).toSeq
case 16 => {
val __t = paymentAddr
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(dest)
case 2 => _root_.scalapb.descriptors.PString(destString)
case 3 => _root_.scalapb.descriptors.PLong(amt)
case 12 => _root_.scalapb.descriptors.PLong(amtMsat)
case 4 => _root_.scalapb.descriptors.PByteString(paymentHash)
case 5 => _root_.scalapb.descriptors.PString(paymentHashString)
case 6 => _root_.scalapb.descriptors.PString(paymentRequest)
case 7 => _root_.scalapb.descriptors.PInt(finalCltvDelta)
case 8 => feeLimit.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 9 => _root_.scalapb.descriptors.PLong(lnrpc.SendRequest._typemapper_outgoingChanId.toBase(outgoingChanId))
case 13 => _root_.scalapb.descriptors.PByteString(lastHopPubkey)
case 10 => _root_.scalapb.descriptors.PInt(lnrpc.SendRequest._typemapper_cltvLimit.toBase(cltvLimit))
case 11 => _root_.scalapb.descriptors.PRepeated(destCustomRecords.iterator.map(lnrpc.SendRequest._typemapper_destCustomRecords.toBase(_).toPMessage).toVector)
case 14 => _root_.scalapb.descriptors.PBoolean(allowSelfPayment)
case 15 => _root_.scalapb.descriptors.PRepeated(destFeatures.iterator.map(__e => _root_.scalapb.descriptors.PEnum(__e.scalaValueDescriptor)).toVector)
case 16 => _root_.scalapb.descriptors.PByteString(paymentAddr)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: lnrpc.SendRequest.type = lnrpc.SendRequest
// @@protoc_insertion_point(GeneratedMessage[lnrpc.SendRequest])
}
object SendRequest extends scalapb.GeneratedMessageCompanion[lnrpc.SendRequest] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[lnrpc.SendRequest] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): lnrpc.SendRequest = {
var __dest: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __destString: _root_.scala.Predef.String = ""
var __amt: _root_.scala.Long = 0L
var __amtMsat: _root_.scala.Long = 0L
var __paymentHash: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __paymentHashString: _root_.scala.Predef.String = ""
var __paymentRequest: _root_.scala.Predef.String = ""
var __finalCltvDelta: _root_.scala.Int = 0
var __feeLimit: _root_.scala.Option[lnrpc.FeeLimit] = _root_.scala.None
var __outgoingChanId: _root_.scala.Long = 0L
var __lastHopPubkey: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __cltvLimit: _root_.scala.Int = 0
val __destCustomRecords: _root_.scala.collection.mutable.Builder[(org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString), _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString]] = _root_.scala.collection.immutable.Map.newBuilder[org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString]
var __allowSelfPayment: _root_.scala.Boolean = false
val __destFeatures: _root_.scala.collection.immutable.VectorBuilder[lnrpc.FeatureBit] = new _root_.scala.collection.immutable.VectorBuilder[lnrpc.FeatureBit]
var __paymentAddr: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__dest = _input__.readBytes()
case 18 =>
__destString = _input__.readStringRequireUtf8()
case 24 =>
__amt = _input__.readInt64()
case 96 =>
__amtMsat = _input__.readInt64()
case 34 =>
__paymentHash = _input__.readBytes()
case 42 =>
__paymentHashString = _input__.readStringRequireUtf8()
case 50 =>
__paymentRequest = _input__.readStringRequireUtf8()
case 56 =>
__finalCltvDelta = _input__.readInt32()
case 66 =>
__feeLimit = Option(__feeLimit.fold(_root_.scalapb.LiteParser.readMessage[lnrpc.FeeLimit](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 72 =>
__outgoingChanId = _input__.readUInt64()
case 106 =>
__lastHopPubkey = _input__.readBytes()
case 80 =>
__cltvLimit = _input__.readUInt32()
case 90 =>
__destCustomRecords += lnrpc.SendRequest._typemapper_destCustomRecords.toCustom(_root_.scalapb.LiteParser.readMessage[lnrpc.SendRequest.DestCustomRecordsEntry](_input__))
case 112 =>
__allowSelfPayment = _input__.readBool()
case 120 =>
__destFeatures += lnrpc.FeatureBit.fromValue(_input__.readEnum())
case 122 => {
val length = _input__.readRawVarint32()
val oldLimit = _input__.pushLimit(length)
while (_input__.getBytesUntilLimit > 0) {
__destFeatures += lnrpc.FeatureBit.fromValue(_input__.readEnum())
}
_input__.popLimit(oldLimit)
}
case 130 =>
__paymentAddr = _input__.readBytes()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
lnrpc.SendRequest(
dest = __dest,
destString = __destString,
amt = __amt,
amtMsat = __amtMsat,
paymentHash = __paymentHash,
paymentHashString = __paymentHashString,
paymentRequest = __paymentRequest,
finalCltvDelta = __finalCltvDelta,
feeLimit = __feeLimit,
outgoingChanId = lnrpc.SendRequest._typemapper_outgoingChanId.toCustom(__outgoingChanId),
lastHopPubkey = __lastHopPubkey,
cltvLimit = lnrpc.SendRequest._typemapper_cltvLimit.toCustom(__cltvLimit),
destCustomRecords = __destCustomRecords.result(),
allowSelfPayment = __allowSelfPayment,
destFeatures = __destFeatures.result(),
paymentAddr = __paymentAddr,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[lnrpc.SendRequest] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
lnrpc.SendRequest(
dest = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
destString = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
amt = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
amtMsat = __fieldsMap.get(scalaDescriptor.findFieldByNumber(12).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
paymentHash = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
paymentHashString = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
paymentRequest = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
finalCltvDelta = __fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).map(_.as[_root_.scala.Int]).getOrElse(0),
feeLimit = __fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).flatMap(_.as[_root_.scala.Option[lnrpc.FeeLimit]]),
outgoingChanId = lnrpc.SendRequest._typemapper_outgoingChanId.toCustom(__fieldsMap.get(scalaDescriptor.findFieldByNumber(9).get).map(_.as[_root_.scala.Long]).getOrElse(0L)),
lastHopPubkey = __fieldsMap.get(scalaDescriptor.findFieldByNumber(13).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
cltvLimit = lnrpc.SendRequest._typemapper_cltvLimit.toCustom(__fieldsMap.get(scalaDescriptor.findFieldByNumber(10).get).map(_.as[_root_.scala.Int]).getOrElse(0)),
destCustomRecords = __fieldsMap.get(scalaDescriptor.findFieldByNumber(11).get).map(_.as[_root_.scala.Seq[lnrpc.SendRequest.DestCustomRecordsEntry]]).getOrElse(_root_.scala.Seq.empty).iterator.map(lnrpc.SendRequest._typemapper_destCustomRecords.toCustom(_)).toMap,
allowSelfPayment = __fieldsMap.get(scalaDescriptor.findFieldByNumber(14).get).map(_.as[_root_.scala.Boolean]).getOrElse(false),
destFeatures = __fieldsMap.get(scalaDescriptor.findFieldByNumber(15).get).map(_.as[_root_.scala.Seq[_root_.scalapb.descriptors.EnumValueDescriptor]]).getOrElse(_root_.scala.Seq.empty).iterator.map(__e => lnrpc.FeatureBit.fromValue(__e.number)).toSeq,
paymentAddr = __fieldsMap.get(scalaDescriptor.findFieldByNumber(16).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = LightningProto.javaDescriptor.getMessageTypes().get(12)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = LightningProto.scalaDescriptor.messages(12)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 8 => __out = lnrpc.FeeLimit
case 11 => __out = lnrpc.SendRequest.DestCustomRecordsEntry
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.lnrpc.SendRequest.DestCustomRecordsEntry
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 15 => lnrpc.FeatureBit
}
}
lazy val defaultInstance = lnrpc.SendRequest(
dest = _root_.com.google.protobuf.ByteString.EMPTY,
destString = "",
amt = 0L,
amtMsat = 0L,
paymentHash = _root_.com.google.protobuf.ByteString.EMPTY,
paymentHashString = "",
paymentRequest = "",
finalCltvDelta = 0,
feeLimit = _root_.scala.None,
outgoingChanId = lnrpc.SendRequest._typemapper_outgoingChanId.toCustom(0L),
lastHopPubkey = _root_.com.google.protobuf.ByteString.EMPTY,
cltvLimit = lnrpc.SendRequest._typemapper_cltvLimit.toCustom(0),
destCustomRecords = _root_.scala.collection.immutable.Map.empty,
allowSelfPayment = false,
destFeatures = _root_.scala.Seq.empty,
paymentAddr = _root_.com.google.protobuf.ByteString.EMPTY
)
@SerialVersionUID(0L)
final case class DestCustomRecordsEntry(
key: org.bitcoins.core.number.UInt64 = lnrpc.SendRequest.DestCustomRecordsEntry._typemapper_key.toCustom(0L),
value: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[DestCustomRecordsEntry] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = lnrpc.SendRequest.DestCustomRecordsEntry._typemapper_key.toBase(key)
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt64Size(1, __value)
}
};
{
val __value = value
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(2, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = lnrpc.SendRequest.DestCustomRecordsEntry._typemapper_key.toBase(key)
if (__v != 0L) {
_output__.writeUInt64(1, __v)
}
};
{
val __v = value
if (!__v.isEmpty) {
_output__.writeBytes(2, __v)
}
};
unknownFields.writeTo(_output__)
}
def withKey(__v: org.bitcoins.core.number.UInt64): DestCustomRecordsEntry = copy(key = __v)
def withValue(__v: _root_.com.google.protobuf.ByteString): DestCustomRecordsEntry = copy(value = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = lnrpc.SendRequest.DestCustomRecordsEntry._typemapper_key.toBase(key)
if (__t != 0L) __t else null
}
case 2 => {
val __t = value
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PLong(lnrpc.SendRequest.DestCustomRecordsEntry._typemapper_key.toBase(key))
case 2 => _root_.scalapb.descriptors.PByteString(value)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: lnrpc.SendRequest.DestCustomRecordsEntry.type = lnrpc.SendRequest.DestCustomRecordsEntry
// @@protoc_insertion_point(GeneratedMessage[lnrpc.SendRequest.DestCustomRecordsEntry])
}
object DestCustomRecordsEntry extends scalapb.GeneratedMessageCompanion[lnrpc.SendRequest.DestCustomRecordsEntry] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[lnrpc.SendRequest.DestCustomRecordsEntry] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): lnrpc.SendRequest.DestCustomRecordsEntry = {
var __key: _root_.scala.Long = 0L
var __value: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 8 =>
__key = _input__.readUInt64()
case 18 =>
__value = _input__.readBytes()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
lnrpc.SendRequest.DestCustomRecordsEntry(
key = lnrpc.SendRequest.DestCustomRecordsEntry._typemapper_key.toCustom(__key),
value = __value,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[lnrpc.SendRequest.DestCustomRecordsEntry] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
lnrpc.SendRequest.DestCustomRecordsEntry(
key = lnrpc.SendRequest.DestCustomRecordsEntry._typemapper_key.toCustom(__fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Long]).getOrElse(0L)),
value = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = lnrpc.SendRequest.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = lnrpc.SendRequest.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = lnrpc.SendRequest.DestCustomRecordsEntry(
key = lnrpc.SendRequest.DestCustomRecordsEntry._typemapper_key.toCustom(0L),
value = _root_.com.google.protobuf.ByteString.EMPTY
)
implicit class DestCustomRecordsEntryLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.SendRequest.DestCustomRecordsEntry]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, lnrpc.SendRequest.DestCustomRecordsEntry](_l) {
def key: _root_.scalapb.lenses.Lens[UpperPB, org.bitcoins.core.number.UInt64] = field(_.key)((c_, f_) => c_.copy(key = f_))
def value: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.value)((c_, f_) => c_.copy(value = f_))
}
final val KEY_FIELD_NUMBER = 1
final val VALUE_FIELD_NUMBER = 2
@transient
private[lnrpc] val _typemapper_key: _root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64] = implicitly[_root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64]]
@transient
implicit val keyValueMapper: _root_.scalapb.TypeMapper[lnrpc.SendRequest.DestCustomRecordsEntry, (org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString)] =
_root_.scalapb.TypeMapper[lnrpc.SendRequest.DestCustomRecordsEntry, (org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString)](__m => (__m.key, __m.value))(__p => lnrpc.SendRequest.DestCustomRecordsEntry(__p._1, __p._2))
def of(
key: org.bitcoins.core.number.UInt64,
value: _root_.com.google.protobuf.ByteString
): _root_.lnrpc.SendRequest.DestCustomRecordsEntry = _root_.lnrpc.SendRequest.DestCustomRecordsEntry(
key,
value
)
// @@protoc_insertion_point(GeneratedMessageCompanion[lnrpc.SendRequest.DestCustomRecordsEntry])
}
implicit class SendRequestLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.SendRequest]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, lnrpc.SendRequest](_l) {
def dest: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.dest)((c_, f_) => c_.copy(dest = f_))
def destString: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.destString)((c_, f_) => c_.copy(destString = f_))
def amt: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.amt)((c_, f_) => c_.copy(amt = f_))
def amtMsat: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.amtMsat)((c_, f_) => c_.copy(amtMsat = f_))
def paymentHash: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.paymentHash)((c_, f_) => c_.copy(paymentHash = f_))
def paymentHashString: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.paymentHashString)((c_, f_) => c_.copy(paymentHashString = f_))
def paymentRequest: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.paymentRequest)((c_, f_) => c_.copy(paymentRequest = f_))
def finalCltvDelta: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.finalCltvDelta)((c_, f_) => c_.copy(finalCltvDelta = f_))
def feeLimit: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.FeeLimit] = field(_.getFeeLimit)((c_, f_) => c_.copy(feeLimit = Option(f_)))
def optionalFeeLimit: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[lnrpc.FeeLimit]] = field(_.feeLimit)((c_, f_) => c_.copy(feeLimit = f_))
def outgoingChanId: _root_.scalapb.lenses.Lens[UpperPB, org.bitcoins.core.number.UInt64] = field(_.outgoingChanId)((c_, f_) => c_.copy(outgoingChanId = f_))
def lastHopPubkey: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.lastHopPubkey)((c_, f_) => c_.copy(lastHopPubkey = f_))
def cltvLimit: _root_.scalapb.lenses.Lens[UpperPB, org.bitcoins.core.number.UInt32] = field(_.cltvLimit)((c_, f_) => c_.copy(cltvLimit = f_))
def destCustomRecords: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString]] = field(_.destCustomRecords)((c_, f_) => c_.copy(destCustomRecords = f_))
def allowSelfPayment: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.allowSelfPayment)((c_, f_) => c_.copy(allowSelfPayment = f_))
def destFeatures: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[lnrpc.FeatureBit]] = field(_.destFeatures)((c_, f_) => c_.copy(destFeatures = f_))
def paymentAddr: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.paymentAddr)((c_, f_) => c_.copy(paymentAddr = f_))
}
final val DEST_FIELD_NUMBER = 1
final val DEST_STRING_FIELD_NUMBER = 2
final val AMT_FIELD_NUMBER = 3
final val AMT_MSAT_FIELD_NUMBER = 12
final val PAYMENT_HASH_FIELD_NUMBER = 4
final val PAYMENT_HASH_STRING_FIELD_NUMBER = 5
final val PAYMENT_REQUEST_FIELD_NUMBER = 6
final val FINAL_CLTV_DELTA_FIELD_NUMBER = 7
final val FEE_LIMIT_FIELD_NUMBER = 8
final val OUTGOING_CHAN_ID_FIELD_NUMBER = 9
final val LAST_HOP_PUBKEY_FIELD_NUMBER = 13
final val CLTV_LIMIT_FIELD_NUMBER = 10
final val DEST_CUSTOM_RECORDS_FIELD_NUMBER = 11
final val ALLOW_SELF_PAYMENT_FIELD_NUMBER = 14
final val DEST_FEATURES_FIELD_NUMBER = 15
final val PAYMENT_ADDR_FIELD_NUMBER = 16
@transient
private[lnrpc] val _typemapper_outgoingChanId: _root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64] = implicitly[_root_.scalapb.TypeMapper[_root_.scala.Long, org.bitcoins.core.number.UInt64]]
@transient
private[lnrpc] val _typemapper_cltvLimit: _root_.scalapb.TypeMapper[_root_.scala.Int, org.bitcoins.core.number.UInt32] = implicitly[_root_.scalapb.TypeMapper[_root_.scala.Int, org.bitcoins.core.number.UInt32]]
@transient
private[lnrpc] val _typemapper_destCustomRecords: _root_.scalapb.TypeMapper[lnrpc.SendRequest.DestCustomRecordsEntry, (org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString)] = implicitly[_root_.scalapb.TypeMapper[lnrpc.SendRequest.DestCustomRecordsEntry, (org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString)]]
def of(
dest: _root_.com.google.protobuf.ByteString,
destString: _root_.scala.Predef.String,
amt: _root_.scala.Long,
amtMsat: _root_.scala.Long,
paymentHash: _root_.com.google.protobuf.ByteString,
paymentHashString: _root_.scala.Predef.String,
paymentRequest: _root_.scala.Predef.String,
finalCltvDelta: _root_.scala.Int,
feeLimit: _root_.scala.Option[lnrpc.FeeLimit],
outgoingChanId: org.bitcoins.core.number.UInt64,
lastHopPubkey: _root_.com.google.protobuf.ByteString,
cltvLimit: org.bitcoins.core.number.UInt32,
destCustomRecords: _root_.scala.collection.immutable.Map[org.bitcoins.core.number.UInt64, _root_.com.google.protobuf.ByteString],
allowSelfPayment: _root_.scala.Boolean,
destFeatures: _root_.scala.Seq[lnrpc.FeatureBit],
paymentAddr: _root_.com.google.protobuf.ByteString
): _root_.lnrpc.SendRequest = _root_.lnrpc.SendRequest(
dest,
destString,
amt,
amtMsat,
paymentHash,
paymentHashString,
paymentRequest,
finalCltvDelta,
feeLimit,
outgoingChanId,
lastHopPubkey,
cltvLimit,
destCustomRecords,
allowSelfPayment,
destFeatures,
paymentAddr
)
// @@protoc_insertion_point(GeneratedMessageCompanion[lnrpc.SendRequest])
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy