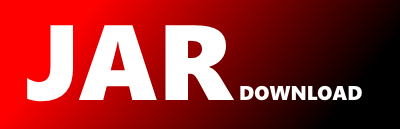
lnrpc.Transaction.scala Maven / Gradle / Ivy
The newest version!
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package lnrpc
import org.bitcoins.lnd.rpc.LndUtils._
/** @param txHash
* The transaction hash
* @param amount
* The transaction amount, denominated in satoshis
* @param numConfirmations
* The number of confirmations
* @param blockHash
* The hash of the block this transaction was included in
* @param blockHeight
* The height of the block this transaction was included in
* @param timeStamp
* Timestamp of this transaction
* @param totalFees
* Fees paid for this transaction
* @param destAddresses
* Addresses that received funds for this transaction. Deprecated as it is
* now incorporated in the output_details field.
* @param outputDetails
* Outputs that received funds for this transaction
* @param rawTxHex
* The raw transaction hex.
* @param label
* A label that was optionally set on transaction broadcast.
* @param previousOutpoints
* PreviousOutpoints/Inputs of this transaction.
*/
@SerialVersionUID(0L)
final case class Transaction(
txHash: _root_.scala.Predef.String = "",
amount: _root_.scala.Long = 0L,
numConfirmations: _root_.scala.Int = 0,
blockHash: _root_.scala.Predef.String = "",
blockHeight: _root_.scala.Int = 0,
timeStamp: _root_.scala.Long = 0L,
totalFees: _root_.scala.Long = 0L,
@scala.deprecated(message="Marked as deprecated in proto file", "") destAddresses: _root_.scala.Seq[_root_.scala.Predef.String] = _root_.scala.Seq.empty,
outputDetails: _root_.scala.Seq[lnrpc.OutputDetail] = _root_.scala.Seq.empty,
rawTxHex: _root_.scala.Predef.String = "",
label: _root_.scala.Predef.String = "",
previousOutpoints: _root_.scala.Seq[lnrpc.PreviousOutPoint] = _root_.scala.Seq.empty,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[Transaction] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = txHash
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(1, __value)
}
};
{
val __value = amount
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(2, __value)
}
};
{
val __value = numConfirmations
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt32Size(3, __value)
}
};
{
val __value = blockHash
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(4, __value)
}
};
{
val __value = blockHeight
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt32Size(5, __value)
}
};
{
val __value = timeStamp
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(6, __value)
}
};
{
val __value = totalFees
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(7, __value)
}
};
destAddresses.foreach { __item =>
val __value = __item
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(8, __value)
}
outputDetails.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = rawTxHex
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(9, __value)
}
};
{
val __value = label
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(10, __value)
}
};
previousOutpoints.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = txHash
if (!__v.isEmpty) {
_output__.writeString(1, __v)
}
};
{
val __v = amount
if (__v != 0L) {
_output__.writeInt64(2, __v)
}
};
{
val __v = numConfirmations
if (__v != 0) {
_output__.writeInt32(3, __v)
}
};
{
val __v = blockHash
if (!__v.isEmpty) {
_output__.writeString(4, __v)
}
};
{
val __v = blockHeight
if (__v != 0) {
_output__.writeInt32(5, __v)
}
};
{
val __v = timeStamp
if (__v != 0L) {
_output__.writeInt64(6, __v)
}
};
{
val __v = totalFees
if (__v != 0L) {
_output__.writeInt64(7, __v)
}
};
destAddresses.foreach { __v =>
val __m = __v
_output__.writeString(8, __m)
};
{
val __v = rawTxHex
if (!__v.isEmpty) {
_output__.writeString(9, __v)
}
};
{
val __v = label
if (!__v.isEmpty) {
_output__.writeString(10, __v)
}
};
outputDetails.foreach { __v =>
val __m = __v
_output__.writeTag(11, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
previousOutpoints.foreach { __v =>
val __m = __v
_output__.writeTag(12, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
unknownFields.writeTo(_output__)
}
def withTxHash(__v: _root_.scala.Predef.String): Transaction = copy(txHash = __v)
def withAmount(__v: _root_.scala.Long): Transaction = copy(amount = __v)
def withNumConfirmations(__v: _root_.scala.Int): Transaction = copy(numConfirmations = __v)
def withBlockHash(__v: _root_.scala.Predef.String): Transaction = copy(blockHash = __v)
def withBlockHeight(__v: _root_.scala.Int): Transaction = copy(blockHeight = __v)
def withTimeStamp(__v: _root_.scala.Long): Transaction = copy(timeStamp = __v)
def withTotalFees(__v: _root_.scala.Long): Transaction = copy(totalFees = __v)
def clearDestAddresses = copy(destAddresses = _root_.scala.Seq.empty)
def addDestAddresses(__vs: _root_.scala.Predef.String *): Transaction = addAllDestAddresses(__vs)
def addAllDestAddresses(__vs: Iterable[_root_.scala.Predef.String]): Transaction = copy(destAddresses = destAddresses ++ __vs)
def withDestAddresses(__v: _root_.scala.Seq[_root_.scala.Predef.String]): Transaction = copy(destAddresses = __v)
def clearOutputDetails = copy(outputDetails = _root_.scala.Seq.empty)
def addOutputDetails(__vs: lnrpc.OutputDetail *): Transaction = addAllOutputDetails(__vs)
def addAllOutputDetails(__vs: Iterable[lnrpc.OutputDetail]): Transaction = copy(outputDetails = outputDetails ++ __vs)
def withOutputDetails(__v: _root_.scala.Seq[lnrpc.OutputDetail]): Transaction = copy(outputDetails = __v)
def withRawTxHex(__v: _root_.scala.Predef.String): Transaction = copy(rawTxHex = __v)
def withLabel(__v: _root_.scala.Predef.String): Transaction = copy(label = __v)
def clearPreviousOutpoints = copy(previousOutpoints = _root_.scala.Seq.empty)
def addPreviousOutpoints(__vs: lnrpc.PreviousOutPoint *): Transaction = addAllPreviousOutpoints(__vs)
def addAllPreviousOutpoints(__vs: Iterable[lnrpc.PreviousOutPoint]): Transaction = copy(previousOutpoints = previousOutpoints ++ __vs)
def withPreviousOutpoints(__v: _root_.scala.Seq[lnrpc.PreviousOutPoint]): Transaction = copy(previousOutpoints = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = txHash
if (__t != "") __t else null
}
case 2 => {
val __t = amount
if (__t != 0L) __t else null
}
case 3 => {
val __t = numConfirmations
if (__t != 0) __t else null
}
case 4 => {
val __t = blockHash
if (__t != "") __t else null
}
case 5 => {
val __t = blockHeight
if (__t != 0) __t else null
}
case 6 => {
val __t = timeStamp
if (__t != 0L) __t else null
}
case 7 => {
val __t = totalFees
if (__t != 0L) __t else null
}
case 8 => destAddresses
case 11 => outputDetails
case 9 => {
val __t = rawTxHex
if (__t != "") __t else null
}
case 10 => {
val __t = label
if (__t != "") __t else null
}
case 12 => previousOutpoints
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PString(txHash)
case 2 => _root_.scalapb.descriptors.PLong(amount)
case 3 => _root_.scalapb.descriptors.PInt(numConfirmations)
case 4 => _root_.scalapb.descriptors.PString(blockHash)
case 5 => _root_.scalapb.descriptors.PInt(blockHeight)
case 6 => _root_.scalapb.descriptors.PLong(timeStamp)
case 7 => _root_.scalapb.descriptors.PLong(totalFees)
case 8 => _root_.scalapb.descriptors.PRepeated(destAddresses.iterator.map(_root_.scalapb.descriptors.PString(_)).toVector)
case 11 => _root_.scalapb.descriptors.PRepeated(outputDetails.iterator.map(_.toPMessage).toVector)
case 9 => _root_.scalapb.descriptors.PString(rawTxHex)
case 10 => _root_.scalapb.descriptors.PString(label)
case 12 => _root_.scalapb.descriptors.PRepeated(previousOutpoints.iterator.map(_.toPMessage).toVector)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: lnrpc.Transaction.type = lnrpc.Transaction
// @@protoc_insertion_point(GeneratedMessage[lnrpc.Transaction])
}
object Transaction extends scalapb.GeneratedMessageCompanion[lnrpc.Transaction] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[lnrpc.Transaction] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): lnrpc.Transaction = {
var __txHash: _root_.scala.Predef.String = ""
var __amount: _root_.scala.Long = 0L
var __numConfirmations: _root_.scala.Int = 0
var __blockHash: _root_.scala.Predef.String = ""
var __blockHeight: _root_.scala.Int = 0
var __timeStamp: _root_.scala.Long = 0L
var __totalFees: _root_.scala.Long = 0L
val __destAddresses: _root_.scala.collection.immutable.VectorBuilder[_root_.scala.Predef.String] = new _root_.scala.collection.immutable.VectorBuilder[_root_.scala.Predef.String]
val __outputDetails: _root_.scala.collection.immutable.VectorBuilder[lnrpc.OutputDetail] = new _root_.scala.collection.immutable.VectorBuilder[lnrpc.OutputDetail]
var __rawTxHex: _root_.scala.Predef.String = ""
var __label: _root_.scala.Predef.String = ""
val __previousOutpoints: _root_.scala.collection.immutable.VectorBuilder[lnrpc.PreviousOutPoint] = new _root_.scala.collection.immutable.VectorBuilder[lnrpc.PreviousOutPoint]
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__txHash = _input__.readStringRequireUtf8()
case 16 =>
__amount = _input__.readInt64()
case 24 =>
__numConfirmations = _input__.readInt32()
case 34 =>
__blockHash = _input__.readStringRequireUtf8()
case 40 =>
__blockHeight = _input__.readInt32()
case 48 =>
__timeStamp = _input__.readInt64()
case 56 =>
__totalFees = _input__.readInt64()
case 66 =>
__destAddresses += _input__.readStringRequireUtf8()
case 90 =>
__outputDetails += _root_.scalapb.LiteParser.readMessage[lnrpc.OutputDetail](_input__)
case 74 =>
__rawTxHex = _input__.readStringRequireUtf8()
case 82 =>
__label = _input__.readStringRequireUtf8()
case 98 =>
__previousOutpoints += _root_.scalapb.LiteParser.readMessage[lnrpc.PreviousOutPoint](_input__)
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
lnrpc.Transaction(
txHash = __txHash,
amount = __amount,
numConfirmations = __numConfirmations,
blockHash = __blockHash,
blockHeight = __blockHeight,
timeStamp = __timeStamp,
totalFees = __totalFees,
destAddresses = __destAddresses.result(),
outputDetails = __outputDetails.result(),
rawTxHex = __rawTxHex,
label = __label,
previousOutpoints = __previousOutpoints.result(),
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[lnrpc.Transaction] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
lnrpc.Transaction(
txHash = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
amount = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
numConfirmations = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Int]).getOrElse(0),
blockHash = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
blockHeight = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Int]).getOrElse(0),
timeStamp = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
totalFees = __fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
destAddresses = __fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).map(_.as[_root_.scala.Seq[_root_.scala.Predef.String]]).getOrElse(_root_.scala.Seq.empty),
outputDetails = __fieldsMap.get(scalaDescriptor.findFieldByNumber(11).get).map(_.as[_root_.scala.Seq[lnrpc.OutputDetail]]).getOrElse(_root_.scala.Seq.empty),
rawTxHex = __fieldsMap.get(scalaDescriptor.findFieldByNumber(9).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
label = __fieldsMap.get(scalaDescriptor.findFieldByNumber(10).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
previousOutpoints = __fieldsMap.get(scalaDescriptor.findFieldByNumber(12).get).map(_.as[_root_.scala.Seq[lnrpc.PreviousOutPoint]]).getOrElse(_root_.scala.Seq.empty)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = LightningProto.javaDescriptor.getMessageTypes().get(8)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = LightningProto.scalaDescriptor.messages(8)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 11 => __out = lnrpc.OutputDetail
case 12 => __out = lnrpc.PreviousOutPoint
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = lnrpc.Transaction(
txHash = "",
amount = 0L,
numConfirmations = 0,
blockHash = "",
blockHeight = 0,
timeStamp = 0L,
totalFees = 0L,
destAddresses = _root_.scala.Seq.empty,
outputDetails = _root_.scala.Seq.empty,
rawTxHex = "",
label = "",
previousOutpoints = _root_.scala.Seq.empty
)
implicit class TransactionLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, lnrpc.Transaction]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, lnrpc.Transaction](_l) {
def txHash: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.txHash)((c_, f_) => c_.copy(txHash = f_))
def amount: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.amount)((c_, f_) => c_.copy(amount = f_))
def numConfirmations: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.numConfirmations)((c_, f_) => c_.copy(numConfirmations = f_))
def blockHash: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.blockHash)((c_, f_) => c_.copy(blockHash = f_))
def blockHeight: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.blockHeight)((c_, f_) => c_.copy(blockHeight = f_))
def timeStamp: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.timeStamp)((c_, f_) => c_.copy(timeStamp = f_))
def totalFees: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.totalFees)((c_, f_) => c_.copy(totalFees = f_))
def destAddresses: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[_root_.scala.Predef.String]] = field(_.destAddresses)((c_, f_) => c_.copy(destAddresses = f_))
def outputDetails: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[lnrpc.OutputDetail]] = field(_.outputDetails)((c_, f_) => c_.copy(outputDetails = f_))
def rawTxHex: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.rawTxHex)((c_, f_) => c_.copy(rawTxHex = f_))
def label: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.label)((c_, f_) => c_.copy(label = f_))
def previousOutpoints: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[lnrpc.PreviousOutPoint]] = field(_.previousOutpoints)((c_, f_) => c_.copy(previousOutpoints = f_))
}
final val TX_HASH_FIELD_NUMBER = 1
final val AMOUNT_FIELD_NUMBER = 2
final val NUM_CONFIRMATIONS_FIELD_NUMBER = 3
final val BLOCK_HASH_FIELD_NUMBER = 4
final val BLOCK_HEIGHT_FIELD_NUMBER = 5
final val TIME_STAMP_FIELD_NUMBER = 6
final val TOTAL_FEES_FIELD_NUMBER = 7
final val DEST_ADDRESSES_FIELD_NUMBER = 8
final val OUTPUT_DETAILS_FIELD_NUMBER = 11
final val RAW_TX_HEX_FIELD_NUMBER = 9
final val LABEL_FIELD_NUMBER = 10
final val PREVIOUS_OUTPOINTS_FIELD_NUMBER = 12
def of(
txHash: _root_.scala.Predef.String,
amount: _root_.scala.Long,
numConfirmations: _root_.scala.Int,
blockHash: _root_.scala.Predef.String,
blockHeight: _root_.scala.Int,
timeStamp: _root_.scala.Long,
totalFees: _root_.scala.Long,
destAddresses: _root_.scala.Seq[_root_.scala.Predef.String],
outputDetails: _root_.scala.Seq[lnrpc.OutputDetail],
rawTxHex: _root_.scala.Predef.String,
label: _root_.scala.Predef.String,
previousOutpoints: _root_.scala.Seq[lnrpc.PreviousOutPoint]
): _root_.lnrpc.Transaction = _root_.lnrpc.Transaction(
txHash,
amount,
numConfirmations,
blockHash,
blockHeight,
timeStamp,
totalFees,
destAddresses,
outputDetails,
rawTxHex,
label,
previousOutpoints
)
// @@protoc_insertion_point(GeneratedMessageCompanion[lnrpc.Transaction])
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy