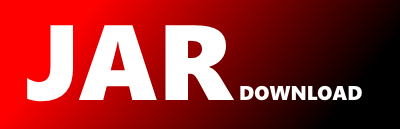
lnrpc.WalletUnlocker.scala Maven / Gradle / Ivy
The newest version!
// Generated by Pekko gRPC. DO NOT EDIT.
package lnrpc
import org.apache.pekko
import pekko.annotation.ApiMayChange
import pekko.grpc.PekkoGrpcGenerated
/**
* WalletUnlocker is a service that is used to set up a wallet password for
* lnd at first startup, and unlock a previously set up wallet.
*/
@PekkoGrpcGenerated
trait WalletUnlocker {
/**
* GenSeed is the first method that should be used to instantiate a new lnd
* instance. This method allows a caller to generate a new aezeed cipher seed
* given an optional passphrase. If provided, the passphrase will be necessary
* to decrypt the cipherseed to expose the internal wallet seed.
* Once the cipherseed is obtained and verified by the user, the InitWallet
* method should be used to commit the newly generated seed, and create the
* wallet.
*/
def genSeed(in: lnrpc.GenSeedRequest): scala.concurrent.Future[lnrpc.GenSeedResponse]
/**
* InitWallet is used when lnd is starting up for the first time to fully
* initialize the daemon and its internal wallet. At the very least a wallet
* password must be provided. This will be used to encrypt sensitive material
* on disk.
* In the case of a recovery scenario, the user can also specify their aezeed
* mnemonic and passphrase. If set, then the daemon will use this prior state
* to initialize its internal wallet.
* Alternatively, this can be used along with the GenSeed RPC to obtain a
* seed, then present it to the user. Once it has been verified by the user,
* the seed can be fed into this RPC in order to commit the new wallet.
*/
def initWallet(in: lnrpc.InitWalletRequest): scala.concurrent.Future[lnrpc.InitWalletResponse]
/**
* lncli: `unlock`
* UnlockWallet is used at startup of lnd to provide a password to unlock
* the wallet database.
*/
def unlockWallet(in: lnrpc.UnlockWalletRequest): scala.concurrent.Future[lnrpc.UnlockWalletResponse]
/**
* lncli: `changepassword`
* ChangePassword changes the password of the encrypted wallet. This will
* automatically unlock the wallet database if successful.
*/
def changePassword(in: lnrpc.ChangePasswordRequest): scala.concurrent.Future[lnrpc.ChangePasswordResponse]
}
@PekkoGrpcGenerated
object WalletUnlocker extends pekko.grpc.ServiceDescription {
val name = "lnrpc.WalletUnlocker"
val descriptor: com.google.protobuf.Descriptors.FileDescriptor =
lnrpc.WalletunlockerProto.javaDescriptor;
object Serializers {
import pekko.grpc.scaladsl.ScalapbProtobufSerializer
val GenSeedRequestSerializer = new ScalapbProtobufSerializer(lnrpc.GenSeedRequest.messageCompanion)
val InitWalletRequestSerializer = new ScalapbProtobufSerializer(lnrpc.InitWalletRequest.messageCompanion)
val UnlockWalletRequestSerializer = new ScalapbProtobufSerializer(lnrpc.UnlockWalletRequest.messageCompanion)
val ChangePasswordRequestSerializer = new ScalapbProtobufSerializer(lnrpc.ChangePasswordRequest.messageCompanion)
val GenSeedResponseSerializer = new ScalapbProtobufSerializer(lnrpc.GenSeedResponse.messageCompanion)
val InitWalletResponseSerializer = new ScalapbProtobufSerializer(lnrpc.InitWalletResponse.messageCompanion)
val UnlockWalletResponseSerializer = new ScalapbProtobufSerializer(lnrpc.UnlockWalletResponse.messageCompanion)
val ChangePasswordResponseSerializer = new ScalapbProtobufSerializer(lnrpc.ChangePasswordResponse.messageCompanion)
}
@ApiMayChange
@PekkoGrpcGenerated
object MethodDescriptors {
import pekko.grpc.internal.Marshaller
import io.grpc.MethodDescriptor
import Serializers._
val genSeedDescriptor: MethodDescriptor[lnrpc.GenSeedRequest, lnrpc.GenSeedResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.WalletUnlocker", "GenSeed"))
.setRequestMarshaller(new Marshaller(GenSeedRequestSerializer))
.setResponseMarshaller(new Marshaller(GenSeedResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val initWalletDescriptor: MethodDescriptor[lnrpc.InitWalletRequest, lnrpc.InitWalletResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.WalletUnlocker", "InitWallet"))
.setRequestMarshaller(new Marshaller(InitWalletRequestSerializer))
.setResponseMarshaller(new Marshaller(InitWalletResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val unlockWalletDescriptor: MethodDescriptor[lnrpc.UnlockWalletRequest, lnrpc.UnlockWalletResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.WalletUnlocker", "UnlockWallet"))
.setRequestMarshaller(new Marshaller(UnlockWalletRequestSerializer))
.setResponseMarshaller(new Marshaller(UnlockWalletResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val changePasswordDescriptor: MethodDescriptor[lnrpc.ChangePasswordRequest, lnrpc.ChangePasswordResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("lnrpc.WalletUnlocker", "ChangePassword"))
.setRequestMarshaller(new Marshaller(ChangePasswordRequestSerializer))
.setResponseMarshaller(new Marshaller(ChangePasswordResponseSerializer))
.setSampledToLocalTracing(true)
.build()
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy