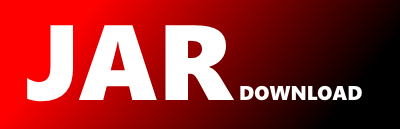
neutrinorpc.GetBlockResponse.scala Maven / Gradle / Ivy
The newest version!
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package neutrinorpc
/** @param hash
* The block hash (same as provided).
* @param confirmations
* The number of confirmations.
* @param strippedSize
* The block size excluding witness data.
* @param size
* The block size (bytes).
* @param weight
* The block weight as defined in BIP 141.
* @param height
* The block height or index.
* @param version
* The block version.
* @param versionHex
* The block version.
* @param merkleroot
* The merkle root.
* @param tx
* List of transaction ids.
* @param time
* The block time in seconds since epoch (Jan 1 1970 GMT).
* @param nonce
* The nonce.
* @param bits
* The bits in hex notation.
* @param ntx
* The number of transactions in the block.
* @param previousBlockHash
* The hash of the previous block.
* @param rawHex
* The raw hex of the block.
*/
@SerialVersionUID(0L)
final case class GetBlockResponse(
hash: _root_.scala.Predef.String = "",
confirmations: _root_.scala.Long = 0L,
strippedSize: _root_.scala.Long = 0L,
size: _root_.scala.Long = 0L,
weight: _root_.scala.Long = 0L,
height: _root_.scala.Int = 0,
version: _root_.scala.Int = 0,
versionHex: _root_.scala.Predef.String = "",
merkleroot: _root_.scala.Predef.String = "",
tx: _root_.scala.Seq[_root_.scala.Predef.String] = _root_.scala.Seq.empty,
time: _root_.scala.Long = 0L,
nonce: _root_.scala.Int = 0,
bits: _root_.scala.Predef.String = "",
ntx: _root_.scala.Int = 0,
previousBlockHash: _root_.scala.Predef.String = "",
rawHex: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[GetBlockResponse] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = hash
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(1, __value)
}
};
{
val __value = confirmations
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(2, __value)
}
};
{
val __value = strippedSize
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(3, __value)
}
};
{
val __value = size
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(4, __value)
}
};
{
val __value = weight
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(5, __value)
}
};
{
val __value = height
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt32Size(6, __value)
}
};
{
val __value = version
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt32Size(7, __value)
}
};
{
val __value = versionHex
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(8, __value)
}
};
{
val __value = merkleroot
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(9, __value)
}
};
tx.foreach { __item =>
val __value = __item
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(10, __value)
}
{
val __value = time
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(11, __value)
}
};
{
val __value = nonce
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt32Size(12, __value)
}
};
{
val __value = bits
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(13, __value)
}
};
{
val __value = ntx
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt32Size(14, __value)
}
};
{
val __value = previousBlockHash
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(15, __value)
}
};
{
val __value = rawHex
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(16, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = hash
if (!__v.isEmpty) {
_output__.writeString(1, __v)
}
};
{
val __v = confirmations
if (__v != 0L) {
_output__.writeInt64(2, __v)
}
};
{
val __v = strippedSize
if (__v != 0L) {
_output__.writeInt64(3, __v)
}
};
{
val __v = size
if (__v != 0L) {
_output__.writeInt64(4, __v)
}
};
{
val __v = weight
if (__v != 0L) {
_output__.writeInt64(5, __v)
}
};
{
val __v = height
if (__v != 0) {
_output__.writeInt32(6, __v)
}
};
{
val __v = version
if (__v != 0) {
_output__.writeInt32(7, __v)
}
};
{
val __v = versionHex
if (!__v.isEmpty) {
_output__.writeString(8, __v)
}
};
{
val __v = merkleroot
if (!__v.isEmpty) {
_output__.writeString(9, __v)
}
};
tx.foreach { __v =>
val __m = __v
_output__.writeString(10, __m)
};
{
val __v = time
if (__v != 0L) {
_output__.writeInt64(11, __v)
}
};
{
val __v = nonce
if (__v != 0) {
_output__.writeUInt32(12, __v)
}
};
{
val __v = bits
if (!__v.isEmpty) {
_output__.writeString(13, __v)
}
};
{
val __v = ntx
if (__v != 0) {
_output__.writeInt32(14, __v)
}
};
{
val __v = previousBlockHash
if (!__v.isEmpty) {
_output__.writeString(15, __v)
}
};
{
val __v = rawHex
if (!__v.isEmpty) {
_output__.writeBytes(16, __v)
}
};
unknownFields.writeTo(_output__)
}
def withHash(__v: _root_.scala.Predef.String): GetBlockResponse = copy(hash = __v)
def withConfirmations(__v: _root_.scala.Long): GetBlockResponse = copy(confirmations = __v)
def withStrippedSize(__v: _root_.scala.Long): GetBlockResponse = copy(strippedSize = __v)
def withSize(__v: _root_.scala.Long): GetBlockResponse = copy(size = __v)
def withWeight(__v: _root_.scala.Long): GetBlockResponse = copy(weight = __v)
def withHeight(__v: _root_.scala.Int): GetBlockResponse = copy(height = __v)
def withVersion(__v: _root_.scala.Int): GetBlockResponse = copy(version = __v)
def withVersionHex(__v: _root_.scala.Predef.String): GetBlockResponse = copy(versionHex = __v)
def withMerkleroot(__v: _root_.scala.Predef.String): GetBlockResponse = copy(merkleroot = __v)
def clearTx = copy(tx = _root_.scala.Seq.empty)
def addTx(__vs: _root_.scala.Predef.String *): GetBlockResponse = addAllTx(__vs)
def addAllTx(__vs: Iterable[_root_.scala.Predef.String]): GetBlockResponse = copy(tx = tx ++ __vs)
def withTx(__v: _root_.scala.Seq[_root_.scala.Predef.String]): GetBlockResponse = copy(tx = __v)
def withTime(__v: _root_.scala.Long): GetBlockResponse = copy(time = __v)
def withNonce(__v: _root_.scala.Int): GetBlockResponse = copy(nonce = __v)
def withBits(__v: _root_.scala.Predef.String): GetBlockResponse = copy(bits = __v)
def withNtx(__v: _root_.scala.Int): GetBlockResponse = copy(ntx = __v)
def withPreviousBlockHash(__v: _root_.scala.Predef.String): GetBlockResponse = copy(previousBlockHash = __v)
def withRawHex(__v: _root_.com.google.protobuf.ByteString): GetBlockResponse = copy(rawHex = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = hash
if (__t != "") __t else null
}
case 2 => {
val __t = confirmations
if (__t != 0L) __t else null
}
case 3 => {
val __t = strippedSize
if (__t != 0L) __t else null
}
case 4 => {
val __t = size
if (__t != 0L) __t else null
}
case 5 => {
val __t = weight
if (__t != 0L) __t else null
}
case 6 => {
val __t = height
if (__t != 0) __t else null
}
case 7 => {
val __t = version
if (__t != 0) __t else null
}
case 8 => {
val __t = versionHex
if (__t != "") __t else null
}
case 9 => {
val __t = merkleroot
if (__t != "") __t else null
}
case 10 => tx
case 11 => {
val __t = time
if (__t != 0L) __t else null
}
case 12 => {
val __t = nonce
if (__t != 0) __t else null
}
case 13 => {
val __t = bits
if (__t != "") __t else null
}
case 14 => {
val __t = ntx
if (__t != 0) __t else null
}
case 15 => {
val __t = previousBlockHash
if (__t != "") __t else null
}
case 16 => {
val __t = rawHex
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PString(hash)
case 2 => _root_.scalapb.descriptors.PLong(confirmations)
case 3 => _root_.scalapb.descriptors.PLong(strippedSize)
case 4 => _root_.scalapb.descriptors.PLong(size)
case 5 => _root_.scalapb.descriptors.PLong(weight)
case 6 => _root_.scalapb.descriptors.PInt(height)
case 7 => _root_.scalapb.descriptors.PInt(version)
case 8 => _root_.scalapb.descriptors.PString(versionHex)
case 9 => _root_.scalapb.descriptors.PString(merkleroot)
case 10 => _root_.scalapb.descriptors.PRepeated(tx.iterator.map(_root_.scalapb.descriptors.PString(_)).toVector)
case 11 => _root_.scalapb.descriptors.PLong(time)
case 12 => _root_.scalapb.descriptors.PInt(nonce)
case 13 => _root_.scalapb.descriptors.PString(bits)
case 14 => _root_.scalapb.descriptors.PInt(ntx)
case 15 => _root_.scalapb.descriptors.PString(previousBlockHash)
case 16 => _root_.scalapb.descriptors.PByteString(rawHex)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: neutrinorpc.GetBlockResponse.type = neutrinorpc.GetBlockResponse
// @@protoc_insertion_point(GeneratedMessage[neutrinorpc.GetBlockResponse])
}
object GetBlockResponse extends scalapb.GeneratedMessageCompanion[neutrinorpc.GetBlockResponse] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[neutrinorpc.GetBlockResponse] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): neutrinorpc.GetBlockResponse = {
var __hash: _root_.scala.Predef.String = ""
var __confirmations: _root_.scala.Long = 0L
var __strippedSize: _root_.scala.Long = 0L
var __size: _root_.scala.Long = 0L
var __weight: _root_.scala.Long = 0L
var __height: _root_.scala.Int = 0
var __version: _root_.scala.Int = 0
var __versionHex: _root_.scala.Predef.String = ""
var __merkleroot: _root_.scala.Predef.String = ""
val __tx: _root_.scala.collection.immutable.VectorBuilder[_root_.scala.Predef.String] = new _root_.scala.collection.immutable.VectorBuilder[_root_.scala.Predef.String]
var __time: _root_.scala.Long = 0L
var __nonce: _root_.scala.Int = 0
var __bits: _root_.scala.Predef.String = ""
var __ntx: _root_.scala.Int = 0
var __previousBlockHash: _root_.scala.Predef.String = ""
var __rawHex: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__hash = _input__.readStringRequireUtf8()
case 16 =>
__confirmations = _input__.readInt64()
case 24 =>
__strippedSize = _input__.readInt64()
case 32 =>
__size = _input__.readInt64()
case 40 =>
__weight = _input__.readInt64()
case 48 =>
__height = _input__.readInt32()
case 56 =>
__version = _input__.readInt32()
case 66 =>
__versionHex = _input__.readStringRequireUtf8()
case 74 =>
__merkleroot = _input__.readStringRequireUtf8()
case 82 =>
__tx += _input__.readStringRequireUtf8()
case 88 =>
__time = _input__.readInt64()
case 96 =>
__nonce = _input__.readUInt32()
case 106 =>
__bits = _input__.readStringRequireUtf8()
case 112 =>
__ntx = _input__.readInt32()
case 122 =>
__previousBlockHash = _input__.readStringRequireUtf8()
case 130 =>
__rawHex = _input__.readBytes()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
neutrinorpc.GetBlockResponse(
hash = __hash,
confirmations = __confirmations,
strippedSize = __strippedSize,
size = __size,
weight = __weight,
height = __height,
version = __version,
versionHex = __versionHex,
merkleroot = __merkleroot,
tx = __tx.result(),
time = __time,
nonce = __nonce,
bits = __bits,
ntx = __ntx,
previousBlockHash = __previousBlockHash,
rawHex = __rawHex,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[neutrinorpc.GetBlockResponse] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
neutrinorpc.GetBlockResponse(
hash = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
confirmations = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
strippedSize = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
size = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
weight = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
height = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scala.Int]).getOrElse(0),
version = __fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).map(_.as[_root_.scala.Int]).getOrElse(0),
versionHex = __fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
merkleroot = __fieldsMap.get(scalaDescriptor.findFieldByNumber(9).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
tx = __fieldsMap.get(scalaDescriptor.findFieldByNumber(10).get).map(_.as[_root_.scala.Seq[_root_.scala.Predef.String]]).getOrElse(_root_.scala.Seq.empty),
time = __fieldsMap.get(scalaDescriptor.findFieldByNumber(11).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
nonce = __fieldsMap.get(scalaDescriptor.findFieldByNumber(12).get).map(_.as[_root_.scala.Int]).getOrElse(0),
bits = __fieldsMap.get(scalaDescriptor.findFieldByNumber(13).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
ntx = __fieldsMap.get(scalaDescriptor.findFieldByNumber(14).get).map(_.as[_root_.scala.Int]).getOrElse(0),
previousBlockHash = __fieldsMap.get(scalaDescriptor.findFieldByNumber(15).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
rawHex = __fieldsMap.get(scalaDescriptor.findFieldByNumber(16).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = NeutrinoProto.javaDescriptor.getMessageTypes().get(11)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = NeutrinoProto.scalaDescriptor.messages(11)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = neutrinorpc.GetBlockResponse(
hash = "",
confirmations = 0L,
strippedSize = 0L,
size = 0L,
weight = 0L,
height = 0,
version = 0,
versionHex = "",
merkleroot = "",
tx = _root_.scala.Seq.empty,
time = 0L,
nonce = 0,
bits = "",
ntx = 0,
previousBlockHash = "",
rawHex = _root_.com.google.protobuf.ByteString.EMPTY
)
implicit class GetBlockResponseLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, neutrinorpc.GetBlockResponse]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, neutrinorpc.GetBlockResponse](_l) {
def hash: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.hash)((c_, f_) => c_.copy(hash = f_))
def confirmations: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.confirmations)((c_, f_) => c_.copy(confirmations = f_))
def strippedSize: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.strippedSize)((c_, f_) => c_.copy(strippedSize = f_))
def size: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.size)((c_, f_) => c_.copy(size = f_))
def weight: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.weight)((c_, f_) => c_.copy(weight = f_))
def height: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.height)((c_, f_) => c_.copy(height = f_))
def version: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.version)((c_, f_) => c_.copy(version = f_))
def versionHex: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.versionHex)((c_, f_) => c_.copy(versionHex = f_))
def merkleroot: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.merkleroot)((c_, f_) => c_.copy(merkleroot = f_))
def tx: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[_root_.scala.Predef.String]] = field(_.tx)((c_, f_) => c_.copy(tx = f_))
def time: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.time)((c_, f_) => c_.copy(time = f_))
def nonce: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.nonce)((c_, f_) => c_.copy(nonce = f_))
def bits: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.bits)((c_, f_) => c_.copy(bits = f_))
def ntx: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.ntx)((c_, f_) => c_.copy(ntx = f_))
def previousBlockHash: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.previousBlockHash)((c_, f_) => c_.copy(previousBlockHash = f_))
def rawHex: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.rawHex)((c_, f_) => c_.copy(rawHex = f_))
}
final val HASH_FIELD_NUMBER = 1
final val CONFIRMATIONS_FIELD_NUMBER = 2
final val STRIPPED_SIZE_FIELD_NUMBER = 3
final val SIZE_FIELD_NUMBER = 4
final val WEIGHT_FIELD_NUMBER = 5
final val HEIGHT_FIELD_NUMBER = 6
final val VERSION_FIELD_NUMBER = 7
final val VERSION_HEX_FIELD_NUMBER = 8
final val MERKLEROOT_FIELD_NUMBER = 9
final val TX_FIELD_NUMBER = 10
final val TIME_FIELD_NUMBER = 11
final val NONCE_FIELD_NUMBER = 12
final val BITS_FIELD_NUMBER = 13
final val NTX_FIELD_NUMBER = 14
final val PREVIOUS_BLOCK_HASH_FIELD_NUMBER = 15
final val RAW_HEX_FIELD_NUMBER = 16
def of(
hash: _root_.scala.Predef.String,
confirmations: _root_.scala.Long,
strippedSize: _root_.scala.Long,
size: _root_.scala.Long,
weight: _root_.scala.Long,
height: _root_.scala.Int,
version: _root_.scala.Int,
versionHex: _root_.scala.Predef.String,
merkleroot: _root_.scala.Predef.String,
tx: _root_.scala.Seq[_root_.scala.Predef.String],
time: _root_.scala.Long,
nonce: _root_.scala.Int,
bits: _root_.scala.Predef.String,
ntx: _root_.scala.Int,
previousBlockHash: _root_.scala.Predef.String,
rawHex: _root_.com.google.protobuf.ByteString
): _root_.neutrinorpc.GetBlockResponse = _root_.neutrinorpc.GetBlockResponse(
hash,
confirmations,
strippedSize,
size,
weight,
height,
version,
versionHex,
merkleroot,
tx,
time,
nonce,
bits,
ntx,
previousBlockHash,
rawHex
)
// @@protoc_insertion_point(GeneratedMessageCompanion[neutrinorpc.GetBlockResponse])
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy