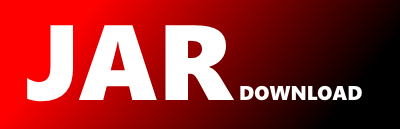
routerrpc.RouterHandler.scala Maven / Gradle / Ivy
The newest version!
// Generated by Pekko gRPC. DO NOT EDIT.
package routerrpc
import scala.concurrent.ExecutionContext
import org.apache.pekko
import pekko.grpc.scaladsl.{ GrpcExceptionHandler, GrpcMarshalling }
import pekko.grpc.Trailers
import pekko.actor.ActorSystem
import pekko.actor.ClassicActorSystemProvider
import pekko.annotation.ApiMayChange
import pekko.http.scaladsl.model
import pekko.stream.{Materializer, SystemMaterializer}
import pekko.grpc.internal.TelemetryExtension
import pekko.grpc.PekkoGrpcGenerated
/*
* Generated by Pekko gRPC. DO NOT EDIT.
*
* The API of this class may still change in future Pekko gRPC versions, see for instance
* https://github.com/akka/akka-grpc/issues/994
*/
@ApiMayChange
@PekkoGrpcGenerated
object RouterHandler {
private val notFound = scala.concurrent.Future.successful(model.HttpResponse(model.StatusCodes.NotFound))
private val unsupportedMediaType = scala.concurrent.Future.successful(model.HttpResponse(model.StatusCodes.UnsupportedMediaType))
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` with `RouterHandler.partial` when combining
* several services.
*/
def apply(implementation: Router)(implicit system: ClassicActorSystemProvider): model.HttpRequest => scala.concurrent.Future[model.HttpResponse] =
partial(implementation).orElse { case _ => notFound }
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` with `RouterHandler.partial` when combining
* several services.
*/
def apply(implementation: Router, eHandler: ActorSystem => PartialFunction[Throwable, Trailers])(implicit system: ClassicActorSystemProvider): model.HttpRequest => scala.concurrent.Future[model.HttpResponse] =
partial(implementation, Router.name, eHandler).orElse { case _ => notFound }
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` with `RouterHandler.partial` when combining
* several services.
*
* Registering a gRPC service under a custom prefix is not widely supported and strongly discouraged by the specification.
*/
def apply(implementation: Router, prefix: String)(implicit system: ClassicActorSystemProvider): model.HttpRequest => scala.concurrent.Future[model.HttpResponse] =
partial(implementation, prefix).orElse { case _ => notFound }
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` with `RouterHandler.partial` when combining
* several services.
*
* Registering a gRPC service under a custom prefix is not widely supported and strongly discouraged by the specification.
*/
def apply(implementation: Router, prefix: String, eHandler: ActorSystem => PartialFunction[Throwable, Trailers])(implicit system: ClassicActorSystemProvider): model.HttpRequest => scala.concurrent.Future[model.HttpResponse] =
partial(implementation, prefix, eHandler).orElse { case _ => notFound }
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler. The generated handler falls back to a reflection handler for
* `Router` and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` with `RouterHandler.partial` when combining
* several services.
*/
def withServerReflection(implementation: Router)(implicit system: ClassicActorSystemProvider): model.HttpRequest => scala.concurrent.Future[model.HttpResponse] =
pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound(
RouterHandler.partial(implementation),
pekko.grpc.scaladsl.ServerReflection.partial(List(Router)))
/**
* Creates a partial `HttpRequest` to `HttpResponse` handler that can be combined with handlers of other
* services with `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` and then used in for example
* `Http().bindAndHandleAsync`.
*
* Use `RouterHandler.apply` if the server is only handling one service.
*
* Registering a gRPC service under a custom prefix is not widely supported and strongly discouraged by the specification.
*/
def partial(implementation: Router, prefix: String = Router.name, eHandler: ActorSystem => PartialFunction[Throwable, Trailers] = GrpcExceptionHandler.defaultMapper)(implicit system: ClassicActorSystemProvider): PartialFunction[model.HttpRequest, scala.concurrent.Future[model.HttpResponse]] = {
implicit val mat: Materializer = SystemMaterializer(system).materializer
implicit val ec: ExecutionContext = mat.executionContext
val spi = TelemetryExtension(system).spi
import Router.Serializers._
def handle(request: model.HttpRequest, method: String): scala.concurrent.Future[model.HttpResponse] =
GrpcMarshalling.negotiated(request, (reader, writer) =>
(method match {
case "SendPaymentV2" =>
GrpcMarshalling.unmarshal(request.entity)(SendPaymentRequestSerializer, mat, reader)
.map(implementation.sendPaymentV2(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(lnrpc_PaymentSerializer, writer, system))
case "TrackPaymentV2" =>
GrpcMarshalling.unmarshal(request.entity)(TrackPaymentRequestSerializer, mat, reader)
.map(implementation.trackPaymentV2(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(lnrpc_PaymentSerializer, writer, system))
case "TrackPayments" =>
GrpcMarshalling.unmarshal(request.entity)(TrackPaymentsRequestSerializer, mat, reader)
.map(implementation.trackPayments(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(lnrpc_PaymentSerializer, writer, system))
case "EstimateRouteFee" =>
GrpcMarshalling.unmarshal(request.entity)(RouteFeeRequestSerializer, mat, reader)
.flatMap(implementation.estimateRouteFee(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(RouteFeeResponseSerializer, writer, system))
case "SendToRoute" =>
GrpcMarshalling.unmarshal(request.entity)(SendToRouteRequestSerializer, mat, reader)
.flatMap(implementation.sendToRoute(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(SendToRouteResponseSerializer, writer, system))
case "SendToRouteV2" =>
GrpcMarshalling.unmarshal(request.entity)(SendToRouteRequestSerializer, mat, reader)
.flatMap(implementation.sendToRouteV2(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(lnrpc_HTLCAttemptSerializer, writer, system))
case "ResetMissionControl" =>
GrpcMarshalling.unmarshal(request.entity)(ResetMissionControlRequestSerializer, mat, reader)
.flatMap(implementation.resetMissionControl(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ResetMissionControlResponseSerializer, writer, system))
case "QueryMissionControl" =>
GrpcMarshalling.unmarshal(request.entity)(QueryMissionControlRequestSerializer, mat, reader)
.flatMap(implementation.queryMissionControl(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(QueryMissionControlResponseSerializer, writer, system))
case "XImportMissionControl" =>
GrpcMarshalling.unmarshal(request.entity)(XImportMissionControlRequestSerializer, mat, reader)
.flatMap(implementation.xImportMissionControl(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(XImportMissionControlResponseSerializer, writer, system))
case "GetMissionControlConfig" =>
GrpcMarshalling.unmarshal(request.entity)(GetMissionControlConfigRequestSerializer, mat, reader)
.flatMap(implementation.getMissionControlConfig(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(GetMissionControlConfigResponseSerializer, writer, system))
case "SetMissionControlConfig" =>
GrpcMarshalling.unmarshal(request.entity)(SetMissionControlConfigRequestSerializer, mat, reader)
.flatMap(implementation.setMissionControlConfig(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(SetMissionControlConfigResponseSerializer, writer, system))
case "QueryProbability" =>
GrpcMarshalling.unmarshal(request.entity)(QueryProbabilityRequestSerializer, mat, reader)
.flatMap(implementation.queryProbability(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(QueryProbabilityResponseSerializer, writer, system))
case "BuildRoute" =>
GrpcMarshalling.unmarshal(request.entity)(BuildRouteRequestSerializer, mat, reader)
.flatMap(implementation.buildRoute(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(BuildRouteResponseSerializer, writer, system))
case "SubscribeHtlcEvents" =>
GrpcMarshalling.unmarshal(request.entity)(SubscribeHtlcEventsRequestSerializer, mat, reader)
.map(implementation.subscribeHtlcEvents(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(HtlcEventSerializer, writer, system))
case "SendPayment" =>
GrpcMarshalling.unmarshal(request.entity)(SendPaymentRequestSerializer, mat, reader)
.map(implementation.sendPayment(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(PaymentStatusSerializer, writer, system))
case "TrackPayment" =>
GrpcMarshalling.unmarshal(request.entity)(TrackPaymentRequestSerializer, mat, reader)
.map(implementation.trackPayment(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(PaymentStatusSerializer, writer, system))
case "HtlcInterceptor" =>
GrpcMarshalling.unmarshalStream(request.entity)(ForwardHtlcInterceptResponseSerializer, mat, reader)
.map(implementation.htlcInterceptor(_))
.map(e => GrpcMarshalling.marshalStream(e, eHandler)(ForwardHtlcInterceptRequestSerializer, writer, system))
case "UpdateChanStatus" =>
GrpcMarshalling.unmarshal(request.entity)(UpdateChanStatusRequestSerializer, mat, reader)
.flatMap(implementation.updateChanStatus(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(UpdateChanStatusResponseSerializer, writer, system))
case m => scala.concurrent.Future.failed(new NotImplementedError(s"Not implemented: $m"))
})
.recoverWith(GrpcExceptionHandler.from(eHandler(system.classicSystem))(system, writer))
).getOrElse(unsupportedMediaType)
Function.unlift((req: model.HttpRequest) => req.uri.path match {
case model.Uri.Path.Slash(model.Uri.Path.Segment(`prefix`, model.Uri.Path.Slash(model.Uri.Path.Segment(method, model.Uri.Path.Empty)))) =>
Some(handle(spi.onRequest(prefix, method, req), method))
case _ =>
None
})
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy