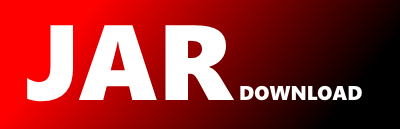
routerrpc.SendPaymentRequest.scala Maven / Gradle / Ivy
The newest version!
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package routerrpc
/** @param dest
* The identity pubkey of the payment recipient
* @param amt
*
* Number of satoshis to send.
*
* The fields amt and amt_msat are mutually exclusive.
* @param amtMsat
*
* Number of millisatoshis to send.
*
* The fields amt and amt_msat are mutually exclusive.
* @param paymentHash
* The hash to use within the payment's HTLC
* @param finalCltvDelta
*
* The CLTV delta from the current height that should be used to set the
* timelock for the final hop.
* @param paymentAddr
* An optional payment addr to be included within the last hop of the route.
* @param paymentRequest
*
* A bare-bones invoice for a payment within the Lightning Network. With the
* details of the invoice, the sender has all the data necessary to send a
* payment to the recipient. The amount in the payment request may be zero. In
* that case it is required to set the amt field as well. If no payment request
* is specified, the following fields are required: dest, amt and payment_hash.
* @param timeoutSeconds
*
* An upper limit on the amount of time we should spend when attempting to
* fulfill the payment. This is expressed in seconds. If we cannot make a
* successful payment within this time frame, an error will be returned.
* This field must be non-zero.
* @param feeLimitSat
*
* The maximum number of satoshis that will be paid as a fee of the payment.
* If this field is left to the default value of 0, only zero-fee routes will
* be considered. This usually means single hop routes connecting directly to
* the destination. To send the payment without a fee limit, use max int here.
*
* The fields fee_limit_sat and fee_limit_msat are mutually exclusive.
* @param feeLimitMsat
*
* The maximum number of millisatoshis that will be paid as a fee of the
* payment. If this field is left to the default value of 0, only zero-fee
* routes will be considered. This usually means single hop routes connecting
* directly to the destination. To send the payment without a fee limit, use
* max int here.
*
* The fields fee_limit_sat and fee_limit_msat are mutually exclusive.
* @param outgoingChanId
*
* Deprecated, use outgoing_chan_ids. The channel id of the channel that must
* be taken to the first hop. If zero, any channel may be used (unless
* outgoing_chan_ids are set).
* @param outgoingChanIds
*
* The channel ids of the channels are allowed for the first hop. If empty,
* any channel may be used.
* @param lastHopPubkey
*
* The pubkey of the last hop of the route. If empty, any hop may be used.
* @param cltvLimit
*
* An optional maximum total time lock for the route. This should not exceed
* lnd's `--max-cltv-expiry` setting. If zero, then the value of
* `--max-cltv-expiry` is enforced.
* @param routeHints
*
* Optional route hints to reach the destination through private channels.
* @param destCustomRecords
*
* An optional field that can be used to pass an arbitrary set of TLV records
* to a peer which understands the new records. This can be used to pass
* application specific data during the payment attempt. Record types are
* required to be in the custom range >= 65536. When using REST, the values
* must be encoded as base64.
* @param allowSelfPayment
* If set, circular payments to self are permitted.
* @param destFeatures
*
* Features assumed to be supported by the final node. All transitive feature
* dependencies must also be set properly. For a given feature bit pair, either
* optional or remote may be set, but not both. If this field is nil or empty,
* the router will try to load destination features from the graph as a
* fallback.
* @param maxParts
*
* The maximum number of partial payments that may be use to complete the full
* amount.
* @param noInflightUpdates
*
* If set, only the final payment update is streamed back. Intermediate updates
* that show which htlcs are still in flight are suppressed.
* @param maxShardSizeMsat
*
* The largest payment split that should be attempted when making a payment if
* splitting is necessary. Setting this value will effectively cause lnd to
* split more aggressively, vs only when it thinks it needs to. Note that this
* value is in milli-satoshis.
* @param amp
*
* If set, an AMP-payment will be attempted.
* @param timePref
*
* The time preference for this payment. Set to -1 to optimize for fees
* only, to 1 to optimize for reliability only or a value inbetween for a mix.
*/
@SerialVersionUID(0L)
final case class SendPaymentRequest(
dest: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
amt: _root_.scala.Long = 0L,
amtMsat: _root_.scala.Long = 0L,
paymentHash: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
finalCltvDelta: _root_.scala.Int = 0,
paymentAddr: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
paymentRequest: _root_.scala.Predef.String = "",
timeoutSeconds: _root_.scala.Int = 0,
feeLimitSat: _root_.scala.Long = 0L,
feeLimitMsat: _root_.scala.Long = 0L,
@scala.deprecated(message="Marked as deprecated in proto file", "") outgoingChanId: _root_.scala.Long = 0L,
outgoingChanIds: _root_.scala.Seq[_root_.scala.Long] = _root_.scala.Seq.empty,
lastHopPubkey: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
cltvLimit: _root_.scala.Int = 0,
routeHints: _root_.scala.Seq[lnrpc.RouteHint] = _root_.scala.Seq.empty,
destCustomRecords: _root_.scala.collection.immutable.Map[_root_.scala.Long, _root_.com.google.protobuf.ByteString] = _root_.scala.collection.immutable.Map.empty,
allowSelfPayment: _root_.scala.Boolean = false,
destFeatures: _root_.scala.Seq[lnrpc.FeatureBit] = _root_.scala.Seq.empty,
maxParts: _root_.scala.Int = 0,
noInflightUpdates: _root_.scala.Boolean = false,
maxShardSizeMsat: _root_.scala.Long = 0L,
amp: _root_.scala.Boolean = false,
timePref: _root_.scala.Double = 0.0,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[SendPaymentRequest] {
private[this] def outgoingChanIdsSerializedSize = {
if (__outgoingChanIdsSerializedSizeField == 0) __outgoingChanIdsSerializedSizeField = {
var __s: _root_.scala.Int = 0
outgoingChanIds.foreach(__i => __s += _root_.com.google.protobuf.CodedOutputStream.computeUInt64SizeNoTag(__i))
__s
}
__outgoingChanIdsSerializedSizeField
}
@transient private[this] var __outgoingChanIdsSerializedSizeField: _root_.scala.Int = 0
private[this] def destFeaturesSerializedSize = {
if (__destFeaturesSerializedSizeField == 0) __destFeaturesSerializedSizeField = {
var __s: _root_.scala.Int = 0
destFeatures.foreach(__i => __s += _root_.com.google.protobuf.CodedOutputStream.computeEnumSizeNoTag(__i.value))
__s
}
__destFeaturesSerializedSizeField
}
@transient private[this] var __destFeaturesSerializedSizeField: _root_.scala.Int = 0
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = dest
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = amt
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(2, __value)
}
};
{
val __value = amtMsat
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(12, __value)
}
};
{
val __value = paymentHash
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(3, __value)
}
};
{
val __value = finalCltvDelta
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt32Size(4, __value)
}
};
{
val __value = paymentAddr
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(20, __value)
}
};
{
val __value = paymentRequest
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeStringSize(5, __value)
}
};
{
val __value = timeoutSeconds
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt32Size(6, __value)
}
};
{
val __value = feeLimitSat
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(7, __value)
}
};
{
val __value = feeLimitMsat
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt64Size(13, __value)
}
};
{
val __value = outgoingChanId
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt64Size(8, __value)
}
};
if (outgoingChanIds.nonEmpty) {
val __localsize = outgoingChanIdsSerializedSize
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__localsize) + __localsize
}
{
val __value = lastHopPubkey
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(14, __value)
}
};
{
val __value = cltvLimit
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt32Size(9, __value)
}
};
routeHints.foreach { __item =>
val __value = __item
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
destCustomRecords.foreach { __item =>
val __value = routerrpc.SendPaymentRequest._typemapper_destCustomRecords.toBase(__item)
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
}
{
val __value = allowSelfPayment
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(15, __value)
}
};
if (destFeatures.nonEmpty) {
val __localsize = destFeaturesSerializedSize
__size += 2 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__localsize) + __localsize
}
{
val __value = maxParts
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt32Size(17, __value)
}
};
{
val __value = noInflightUpdates
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(18, __value)
}
};
{
val __value = maxShardSizeMsat
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt64Size(21, __value)
}
};
{
val __value = amp
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(22, __value)
}
};
{
val __value = timePref
if (__value != 0.0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeDoubleSize(23, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = dest
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = amt
if (__v != 0L) {
_output__.writeInt64(2, __v)
}
};
{
val __v = paymentHash
if (!__v.isEmpty) {
_output__.writeBytes(3, __v)
}
};
{
val __v = finalCltvDelta
if (__v != 0) {
_output__.writeInt32(4, __v)
}
};
{
val __v = paymentRequest
if (!__v.isEmpty) {
_output__.writeString(5, __v)
}
};
{
val __v = timeoutSeconds
if (__v != 0) {
_output__.writeInt32(6, __v)
}
};
{
val __v = feeLimitSat
if (__v != 0L) {
_output__.writeInt64(7, __v)
}
};
{
val __v = outgoingChanId
if (__v != 0L) {
_output__.writeUInt64(8, __v)
}
};
{
val __v = cltvLimit
if (__v != 0) {
_output__.writeInt32(9, __v)
}
};
routeHints.foreach { __v =>
val __m = __v
_output__.writeTag(10, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
destCustomRecords.foreach { __v =>
val __m = routerrpc.SendPaymentRequest._typemapper_destCustomRecords.toBase(__v)
_output__.writeTag(11, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = amtMsat
if (__v != 0L) {
_output__.writeInt64(12, __v)
}
};
{
val __v = feeLimitMsat
if (__v != 0L) {
_output__.writeInt64(13, __v)
}
};
{
val __v = lastHopPubkey
if (!__v.isEmpty) {
_output__.writeBytes(14, __v)
}
};
{
val __v = allowSelfPayment
if (__v != false) {
_output__.writeBool(15, __v)
}
};
if (destFeatures.nonEmpty) {
_output__.writeTag(16, 2)
_output__.writeUInt32NoTag(destFeaturesSerializedSize)
destFeatures.foreach((_output__.writeEnumNoTag _).compose((_: lnrpc.FeatureBit).value))
};
{
val __v = maxParts
if (__v != 0) {
_output__.writeUInt32(17, __v)
}
};
{
val __v = noInflightUpdates
if (__v != false) {
_output__.writeBool(18, __v)
}
};
if (outgoingChanIds.nonEmpty) {
_output__.writeTag(19, 2)
_output__.writeUInt32NoTag(outgoingChanIdsSerializedSize)
outgoingChanIds.foreach(_output__.writeUInt64NoTag)
};
{
val __v = paymentAddr
if (!__v.isEmpty) {
_output__.writeBytes(20, __v)
}
};
{
val __v = maxShardSizeMsat
if (__v != 0L) {
_output__.writeUInt64(21, __v)
}
};
{
val __v = amp
if (__v != false) {
_output__.writeBool(22, __v)
}
};
{
val __v = timePref
if (__v != 0.0) {
_output__.writeDouble(23, __v)
}
};
unknownFields.writeTo(_output__)
}
def withDest(__v: _root_.com.google.protobuf.ByteString): SendPaymentRequest = copy(dest = __v)
def withAmt(__v: _root_.scala.Long): SendPaymentRequest = copy(amt = __v)
def withAmtMsat(__v: _root_.scala.Long): SendPaymentRequest = copy(amtMsat = __v)
def withPaymentHash(__v: _root_.com.google.protobuf.ByteString): SendPaymentRequest = copy(paymentHash = __v)
def withFinalCltvDelta(__v: _root_.scala.Int): SendPaymentRequest = copy(finalCltvDelta = __v)
def withPaymentAddr(__v: _root_.com.google.protobuf.ByteString): SendPaymentRequest = copy(paymentAddr = __v)
def withPaymentRequest(__v: _root_.scala.Predef.String): SendPaymentRequest = copy(paymentRequest = __v)
def withTimeoutSeconds(__v: _root_.scala.Int): SendPaymentRequest = copy(timeoutSeconds = __v)
def withFeeLimitSat(__v: _root_.scala.Long): SendPaymentRequest = copy(feeLimitSat = __v)
def withFeeLimitMsat(__v: _root_.scala.Long): SendPaymentRequest = copy(feeLimitMsat = __v)
def withOutgoingChanId(__v: _root_.scala.Long): SendPaymentRequest = copy(outgoingChanId = __v)
def clearOutgoingChanIds = copy(outgoingChanIds = _root_.scala.Seq.empty)
def addOutgoingChanIds(__vs: _root_.scala.Long *): SendPaymentRequest = addAllOutgoingChanIds(__vs)
def addAllOutgoingChanIds(__vs: Iterable[_root_.scala.Long]): SendPaymentRequest = copy(outgoingChanIds = outgoingChanIds ++ __vs)
def withOutgoingChanIds(__v: _root_.scala.Seq[_root_.scala.Long]): SendPaymentRequest = copy(outgoingChanIds = __v)
def withLastHopPubkey(__v: _root_.com.google.protobuf.ByteString): SendPaymentRequest = copy(lastHopPubkey = __v)
def withCltvLimit(__v: _root_.scala.Int): SendPaymentRequest = copy(cltvLimit = __v)
def clearRouteHints = copy(routeHints = _root_.scala.Seq.empty)
def addRouteHints(__vs: lnrpc.RouteHint *): SendPaymentRequest = addAllRouteHints(__vs)
def addAllRouteHints(__vs: Iterable[lnrpc.RouteHint]): SendPaymentRequest = copy(routeHints = routeHints ++ __vs)
def withRouteHints(__v: _root_.scala.Seq[lnrpc.RouteHint]): SendPaymentRequest = copy(routeHints = __v)
def clearDestCustomRecords = copy(destCustomRecords = _root_.scala.collection.immutable.Map.empty)
def addDestCustomRecords(__vs: (_root_.scala.Long, _root_.com.google.protobuf.ByteString) *): SendPaymentRequest = addAllDestCustomRecords(__vs)
def addAllDestCustomRecords(__vs: Iterable[(_root_.scala.Long, _root_.com.google.protobuf.ByteString)]): SendPaymentRequest = copy(destCustomRecords = destCustomRecords ++ __vs)
def withDestCustomRecords(__v: _root_.scala.collection.immutable.Map[_root_.scala.Long, _root_.com.google.protobuf.ByteString]): SendPaymentRequest = copy(destCustomRecords = __v)
def withAllowSelfPayment(__v: _root_.scala.Boolean): SendPaymentRequest = copy(allowSelfPayment = __v)
def clearDestFeatures = copy(destFeatures = _root_.scala.Seq.empty)
def addDestFeatures(__vs: lnrpc.FeatureBit *): SendPaymentRequest = addAllDestFeatures(__vs)
def addAllDestFeatures(__vs: Iterable[lnrpc.FeatureBit]): SendPaymentRequest = copy(destFeatures = destFeatures ++ __vs)
def withDestFeatures(__v: _root_.scala.Seq[lnrpc.FeatureBit]): SendPaymentRequest = copy(destFeatures = __v)
def withMaxParts(__v: _root_.scala.Int): SendPaymentRequest = copy(maxParts = __v)
def withNoInflightUpdates(__v: _root_.scala.Boolean): SendPaymentRequest = copy(noInflightUpdates = __v)
def withMaxShardSizeMsat(__v: _root_.scala.Long): SendPaymentRequest = copy(maxShardSizeMsat = __v)
def withAmp(__v: _root_.scala.Boolean): SendPaymentRequest = copy(amp = __v)
def withTimePref(__v: _root_.scala.Double): SendPaymentRequest = copy(timePref = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = dest
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = amt
if (__t != 0L) __t else null
}
case 12 => {
val __t = amtMsat
if (__t != 0L) __t else null
}
case 3 => {
val __t = paymentHash
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 4 => {
val __t = finalCltvDelta
if (__t != 0) __t else null
}
case 20 => {
val __t = paymentAddr
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 5 => {
val __t = paymentRequest
if (__t != "") __t else null
}
case 6 => {
val __t = timeoutSeconds
if (__t != 0) __t else null
}
case 7 => {
val __t = feeLimitSat
if (__t != 0L) __t else null
}
case 13 => {
val __t = feeLimitMsat
if (__t != 0L) __t else null
}
case 8 => {
val __t = outgoingChanId
if (__t != 0L) __t else null
}
case 19 => outgoingChanIds
case 14 => {
val __t = lastHopPubkey
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 9 => {
val __t = cltvLimit
if (__t != 0) __t else null
}
case 10 => routeHints
case 11 => destCustomRecords.iterator.map(routerrpc.SendPaymentRequest._typemapper_destCustomRecords.toBase(_)).toSeq
case 15 => {
val __t = allowSelfPayment
if (__t != false) __t else null
}
case 16 => destFeatures.iterator.map(_.javaValueDescriptor).toSeq
case 17 => {
val __t = maxParts
if (__t != 0) __t else null
}
case 18 => {
val __t = noInflightUpdates
if (__t != false) __t else null
}
case 21 => {
val __t = maxShardSizeMsat
if (__t != 0L) __t else null
}
case 22 => {
val __t = amp
if (__t != false) __t else null
}
case 23 => {
val __t = timePref
if (__t != 0.0) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(dest)
case 2 => _root_.scalapb.descriptors.PLong(amt)
case 12 => _root_.scalapb.descriptors.PLong(amtMsat)
case 3 => _root_.scalapb.descriptors.PByteString(paymentHash)
case 4 => _root_.scalapb.descriptors.PInt(finalCltvDelta)
case 20 => _root_.scalapb.descriptors.PByteString(paymentAddr)
case 5 => _root_.scalapb.descriptors.PString(paymentRequest)
case 6 => _root_.scalapb.descriptors.PInt(timeoutSeconds)
case 7 => _root_.scalapb.descriptors.PLong(feeLimitSat)
case 13 => _root_.scalapb.descriptors.PLong(feeLimitMsat)
case 8 => _root_.scalapb.descriptors.PLong(outgoingChanId)
case 19 => _root_.scalapb.descriptors.PRepeated(outgoingChanIds.iterator.map(_root_.scalapb.descriptors.PLong(_)).toVector)
case 14 => _root_.scalapb.descriptors.PByteString(lastHopPubkey)
case 9 => _root_.scalapb.descriptors.PInt(cltvLimit)
case 10 => _root_.scalapb.descriptors.PRepeated(routeHints.iterator.map(_.toPMessage).toVector)
case 11 => _root_.scalapb.descriptors.PRepeated(destCustomRecords.iterator.map(routerrpc.SendPaymentRequest._typemapper_destCustomRecords.toBase(_).toPMessage).toVector)
case 15 => _root_.scalapb.descriptors.PBoolean(allowSelfPayment)
case 16 => _root_.scalapb.descriptors.PRepeated(destFeatures.iterator.map(__e => _root_.scalapb.descriptors.PEnum(__e.scalaValueDescriptor)).toVector)
case 17 => _root_.scalapb.descriptors.PInt(maxParts)
case 18 => _root_.scalapb.descriptors.PBoolean(noInflightUpdates)
case 21 => _root_.scalapb.descriptors.PLong(maxShardSizeMsat)
case 22 => _root_.scalapb.descriptors.PBoolean(amp)
case 23 => _root_.scalapb.descriptors.PDouble(timePref)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: routerrpc.SendPaymentRequest.type = routerrpc.SendPaymentRequest
// @@protoc_insertion_point(GeneratedMessage[routerrpc.SendPaymentRequest])
}
object SendPaymentRequest extends scalapb.GeneratedMessageCompanion[routerrpc.SendPaymentRequest] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[routerrpc.SendPaymentRequest] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): routerrpc.SendPaymentRequest = {
var __dest: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __amt: _root_.scala.Long = 0L
var __amtMsat: _root_.scala.Long = 0L
var __paymentHash: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __finalCltvDelta: _root_.scala.Int = 0
var __paymentAddr: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __paymentRequest: _root_.scala.Predef.String = ""
var __timeoutSeconds: _root_.scala.Int = 0
var __feeLimitSat: _root_.scala.Long = 0L
var __feeLimitMsat: _root_.scala.Long = 0L
var __outgoingChanId: _root_.scala.Long = 0L
val __outgoingChanIds: _root_.scala.collection.immutable.VectorBuilder[_root_.scala.Long] = new _root_.scala.collection.immutable.VectorBuilder[_root_.scala.Long]
var __lastHopPubkey: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __cltvLimit: _root_.scala.Int = 0
val __routeHints: _root_.scala.collection.immutable.VectorBuilder[lnrpc.RouteHint] = new _root_.scala.collection.immutable.VectorBuilder[lnrpc.RouteHint]
val __destCustomRecords: _root_.scala.collection.mutable.Builder[(_root_.scala.Long, _root_.com.google.protobuf.ByteString), _root_.scala.collection.immutable.Map[_root_.scala.Long, _root_.com.google.protobuf.ByteString]] = _root_.scala.collection.immutable.Map.newBuilder[_root_.scala.Long, _root_.com.google.protobuf.ByteString]
var __allowSelfPayment: _root_.scala.Boolean = false
val __destFeatures: _root_.scala.collection.immutable.VectorBuilder[lnrpc.FeatureBit] = new _root_.scala.collection.immutable.VectorBuilder[lnrpc.FeatureBit]
var __maxParts: _root_.scala.Int = 0
var __noInflightUpdates: _root_.scala.Boolean = false
var __maxShardSizeMsat: _root_.scala.Long = 0L
var __amp: _root_.scala.Boolean = false
var __timePref: _root_.scala.Double = 0.0
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__dest = _input__.readBytes()
case 16 =>
__amt = _input__.readInt64()
case 96 =>
__amtMsat = _input__.readInt64()
case 26 =>
__paymentHash = _input__.readBytes()
case 32 =>
__finalCltvDelta = _input__.readInt32()
case 162 =>
__paymentAddr = _input__.readBytes()
case 42 =>
__paymentRequest = _input__.readStringRequireUtf8()
case 48 =>
__timeoutSeconds = _input__.readInt32()
case 56 =>
__feeLimitSat = _input__.readInt64()
case 104 =>
__feeLimitMsat = _input__.readInt64()
case 64 =>
__outgoingChanId = _input__.readUInt64()
case 152 =>
__outgoingChanIds += _input__.readUInt64()
case 154 => {
val length = _input__.readRawVarint32()
val oldLimit = _input__.pushLimit(length)
while (_input__.getBytesUntilLimit > 0) {
__outgoingChanIds += _input__.readUInt64()
}
_input__.popLimit(oldLimit)
}
case 114 =>
__lastHopPubkey = _input__.readBytes()
case 72 =>
__cltvLimit = _input__.readInt32()
case 82 =>
__routeHints += _root_.scalapb.LiteParser.readMessage[lnrpc.RouteHint](_input__)
case 90 =>
__destCustomRecords += routerrpc.SendPaymentRequest._typemapper_destCustomRecords.toCustom(_root_.scalapb.LiteParser.readMessage[routerrpc.SendPaymentRequest.DestCustomRecordsEntry](_input__))
case 120 =>
__allowSelfPayment = _input__.readBool()
case 128 =>
__destFeatures += lnrpc.FeatureBit.fromValue(_input__.readEnum())
case 130 => {
val length = _input__.readRawVarint32()
val oldLimit = _input__.pushLimit(length)
while (_input__.getBytesUntilLimit > 0) {
__destFeatures += lnrpc.FeatureBit.fromValue(_input__.readEnum())
}
_input__.popLimit(oldLimit)
}
case 136 =>
__maxParts = _input__.readUInt32()
case 144 =>
__noInflightUpdates = _input__.readBool()
case 168 =>
__maxShardSizeMsat = _input__.readUInt64()
case 176 =>
__amp = _input__.readBool()
case 185 =>
__timePref = _input__.readDouble()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
routerrpc.SendPaymentRequest(
dest = __dest,
amt = __amt,
amtMsat = __amtMsat,
paymentHash = __paymentHash,
finalCltvDelta = __finalCltvDelta,
paymentAddr = __paymentAddr,
paymentRequest = __paymentRequest,
timeoutSeconds = __timeoutSeconds,
feeLimitSat = __feeLimitSat,
feeLimitMsat = __feeLimitMsat,
outgoingChanId = __outgoingChanId,
outgoingChanIds = __outgoingChanIds.result(),
lastHopPubkey = __lastHopPubkey,
cltvLimit = __cltvLimit,
routeHints = __routeHints.result(),
destCustomRecords = __destCustomRecords.result(),
allowSelfPayment = __allowSelfPayment,
destFeatures = __destFeatures.result(),
maxParts = __maxParts,
noInflightUpdates = __noInflightUpdates,
maxShardSizeMsat = __maxShardSizeMsat,
amp = __amp,
timePref = __timePref,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[routerrpc.SendPaymentRequest] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
routerrpc.SendPaymentRequest(
dest = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
amt = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
amtMsat = __fieldsMap.get(scalaDescriptor.findFieldByNumber(12).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
paymentHash = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
finalCltvDelta = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.scala.Int]).getOrElse(0),
paymentAddr = __fieldsMap.get(scalaDescriptor.findFieldByNumber(20).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
paymentRequest = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Predef.String]).getOrElse(""),
timeoutSeconds = __fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scala.Int]).getOrElse(0),
feeLimitSat = __fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
feeLimitMsat = __fieldsMap.get(scalaDescriptor.findFieldByNumber(13).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
outgoingChanId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
outgoingChanIds = __fieldsMap.get(scalaDescriptor.findFieldByNumber(19).get).map(_.as[_root_.scala.Seq[_root_.scala.Long]]).getOrElse(_root_.scala.Seq.empty),
lastHopPubkey = __fieldsMap.get(scalaDescriptor.findFieldByNumber(14).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
cltvLimit = __fieldsMap.get(scalaDescriptor.findFieldByNumber(9).get).map(_.as[_root_.scala.Int]).getOrElse(0),
routeHints = __fieldsMap.get(scalaDescriptor.findFieldByNumber(10).get).map(_.as[_root_.scala.Seq[lnrpc.RouteHint]]).getOrElse(_root_.scala.Seq.empty),
destCustomRecords = __fieldsMap.get(scalaDescriptor.findFieldByNumber(11).get).map(_.as[_root_.scala.Seq[routerrpc.SendPaymentRequest.DestCustomRecordsEntry]]).getOrElse(_root_.scala.Seq.empty).iterator.map(routerrpc.SendPaymentRequest._typemapper_destCustomRecords.toCustom(_)).toMap,
allowSelfPayment = __fieldsMap.get(scalaDescriptor.findFieldByNumber(15).get).map(_.as[_root_.scala.Boolean]).getOrElse(false),
destFeatures = __fieldsMap.get(scalaDescriptor.findFieldByNumber(16).get).map(_.as[_root_.scala.Seq[_root_.scalapb.descriptors.EnumValueDescriptor]]).getOrElse(_root_.scala.Seq.empty).iterator.map(__e => lnrpc.FeatureBit.fromValue(__e.number)).toSeq,
maxParts = __fieldsMap.get(scalaDescriptor.findFieldByNumber(17).get).map(_.as[_root_.scala.Int]).getOrElse(0),
noInflightUpdates = __fieldsMap.get(scalaDescriptor.findFieldByNumber(18).get).map(_.as[_root_.scala.Boolean]).getOrElse(false),
maxShardSizeMsat = __fieldsMap.get(scalaDescriptor.findFieldByNumber(21).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
amp = __fieldsMap.get(scalaDescriptor.findFieldByNumber(22).get).map(_.as[_root_.scala.Boolean]).getOrElse(false),
timePref = __fieldsMap.get(scalaDescriptor.findFieldByNumber(23).get).map(_.as[_root_.scala.Double]).getOrElse(0.0)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = RouterProto.javaDescriptor.getMessageTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = RouterProto.scalaDescriptor.messages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 10 => __out = lnrpc.RouteHint
case 11 => __out = routerrpc.SendPaymentRequest.DestCustomRecordsEntry
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] =
Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]](
_root_.routerrpc.SendPaymentRequest.DestCustomRecordsEntry
)
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 16 => lnrpc.FeatureBit
}
}
lazy val defaultInstance = routerrpc.SendPaymentRequest(
dest = _root_.com.google.protobuf.ByteString.EMPTY,
amt = 0L,
amtMsat = 0L,
paymentHash = _root_.com.google.protobuf.ByteString.EMPTY,
finalCltvDelta = 0,
paymentAddr = _root_.com.google.protobuf.ByteString.EMPTY,
paymentRequest = "",
timeoutSeconds = 0,
feeLimitSat = 0L,
feeLimitMsat = 0L,
outgoingChanId = 0L,
outgoingChanIds = _root_.scala.Seq.empty,
lastHopPubkey = _root_.com.google.protobuf.ByteString.EMPTY,
cltvLimit = 0,
routeHints = _root_.scala.Seq.empty,
destCustomRecords = _root_.scala.collection.immutable.Map.empty,
allowSelfPayment = false,
destFeatures = _root_.scala.Seq.empty,
maxParts = 0,
noInflightUpdates = false,
maxShardSizeMsat = 0L,
amp = false,
timePref = 0.0
)
@SerialVersionUID(0L)
final case class DestCustomRecordsEntry(
key: _root_.scala.Long = 0L,
value: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[DestCustomRecordsEntry] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = key
if (__value != 0L) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt64Size(1, __value)
}
};
{
val __value = value
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(2, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = key
if (__v != 0L) {
_output__.writeUInt64(1, __v)
}
};
{
val __v = value
if (!__v.isEmpty) {
_output__.writeBytes(2, __v)
}
};
unknownFields.writeTo(_output__)
}
def withKey(__v: _root_.scala.Long): DestCustomRecordsEntry = copy(key = __v)
def withValue(__v: _root_.com.google.protobuf.ByteString): DestCustomRecordsEntry = copy(value = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = key
if (__t != 0L) __t else null
}
case 2 => {
val __t = value
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PLong(key)
case 2 => _root_.scalapb.descriptors.PByteString(value)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: routerrpc.SendPaymentRequest.DestCustomRecordsEntry.type = routerrpc.SendPaymentRequest.DestCustomRecordsEntry
// @@protoc_insertion_point(GeneratedMessage[routerrpc.SendPaymentRequest.DestCustomRecordsEntry])
}
object DestCustomRecordsEntry extends scalapb.GeneratedMessageCompanion[routerrpc.SendPaymentRequest.DestCustomRecordsEntry] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[routerrpc.SendPaymentRequest.DestCustomRecordsEntry] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): routerrpc.SendPaymentRequest.DestCustomRecordsEntry = {
var __key: _root_.scala.Long = 0L
var __value: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 8 =>
__key = _input__.readUInt64()
case 18 =>
__value = _input__.readBytes()
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
routerrpc.SendPaymentRequest.DestCustomRecordsEntry(
key = __key,
value = __value,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[routerrpc.SendPaymentRequest.DestCustomRecordsEntry] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
routerrpc.SendPaymentRequest.DestCustomRecordsEntry(
key = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.scala.Long]).getOrElse(0L),
value = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = routerrpc.SendPaymentRequest.javaDescriptor.getNestedTypes().get(0)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = routerrpc.SendPaymentRequest.scalaDescriptor.nestedMessages(0)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = throw new MatchError(__fieldNumber)
lazy val defaultInstance = routerrpc.SendPaymentRequest.DestCustomRecordsEntry(
key = 0L,
value = _root_.com.google.protobuf.ByteString.EMPTY
)
implicit class DestCustomRecordsEntryLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, routerrpc.SendPaymentRequest.DestCustomRecordsEntry]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, routerrpc.SendPaymentRequest.DestCustomRecordsEntry](_l) {
def key: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.key)((c_, f_) => c_.copy(key = f_))
def value: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.value)((c_, f_) => c_.copy(value = f_))
}
final val KEY_FIELD_NUMBER = 1
final val VALUE_FIELD_NUMBER = 2
@transient
implicit val keyValueMapper: _root_.scalapb.TypeMapper[routerrpc.SendPaymentRequest.DestCustomRecordsEntry, (_root_.scala.Long, _root_.com.google.protobuf.ByteString)] =
_root_.scalapb.TypeMapper[routerrpc.SendPaymentRequest.DestCustomRecordsEntry, (_root_.scala.Long, _root_.com.google.protobuf.ByteString)](__m => (__m.key, __m.value))(__p => routerrpc.SendPaymentRequest.DestCustomRecordsEntry(__p._1, __p._2))
def of(
key: _root_.scala.Long,
value: _root_.com.google.protobuf.ByteString
): _root_.routerrpc.SendPaymentRequest.DestCustomRecordsEntry = _root_.routerrpc.SendPaymentRequest.DestCustomRecordsEntry(
key,
value
)
// @@protoc_insertion_point(GeneratedMessageCompanion[routerrpc.SendPaymentRequest.DestCustomRecordsEntry])
}
implicit class SendPaymentRequestLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, routerrpc.SendPaymentRequest]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, routerrpc.SendPaymentRequest](_l) {
def dest: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.dest)((c_, f_) => c_.copy(dest = f_))
def amt: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.amt)((c_, f_) => c_.copy(amt = f_))
def amtMsat: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.amtMsat)((c_, f_) => c_.copy(amtMsat = f_))
def paymentHash: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.paymentHash)((c_, f_) => c_.copy(paymentHash = f_))
def finalCltvDelta: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.finalCltvDelta)((c_, f_) => c_.copy(finalCltvDelta = f_))
def paymentAddr: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.paymentAddr)((c_, f_) => c_.copy(paymentAddr = f_))
def paymentRequest: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Predef.String] = field(_.paymentRequest)((c_, f_) => c_.copy(paymentRequest = f_))
def timeoutSeconds: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.timeoutSeconds)((c_, f_) => c_.copy(timeoutSeconds = f_))
def feeLimitSat: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.feeLimitSat)((c_, f_) => c_.copy(feeLimitSat = f_))
def feeLimitMsat: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.feeLimitMsat)((c_, f_) => c_.copy(feeLimitMsat = f_))
def outgoingChanId: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.outgoingChanId)((c_, f_) => c_.copy(outgoingChanId = f_))
def outgoingChanIds: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[_root_.scala.Long]] = field(_.outgoingChanIds)((c_, f_) => c_.copy(outgoingChanIds = f_))
def lastHopPubkey: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.lastHopPubkey)((c_, f_) => c_.copy(lastHopPubkey = f_))
def cltvLimit: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.cltvLimit)((c_, f_) => c_.copy(cltvLimit = f_))
def routeHints: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[lnrpc.RouteHint]] = field(_.routeHints)((c_, f_) => c_.copy(routeHints = f_))
def destCustomRecords: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.collection.immutable.Map[_root_.scala.Long, _root_.com.google.protobuf.ByteString]] = field(_.destCustomRecords)((c_, f_) => c_.copy(destCustomRecords = f_))
def allowSelfPayment: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.allowSelfPayment)((c_, f_) => c_.copy(allowSelfPayment = f_))
def destFeatures: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Seq[lnrpc.FeatureBit]] = field(_.destFeatures)((c_, f_) => c_.copy(destFeatures = f_))
def maxParts: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.maxParts)((c_, f_) => c_.copy(maxParts = f_))
def noInflightUpdates: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.noInflightUpdates)((c_, f_) => c_.copy(noInflightUpdates = f_))
def maxShardSizeMsat: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Long] = field(_.maxShardSizeMsat)((c_, f_) => c_.copy(maxShardSizeMsat = f_))
def amp: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.amp)((c_, f_) => c_.copy(amp = f_))
def timePref: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Double] = field(_.timePref)((c_, f_) => c_.copy(timePref = f_))
}
final val DEST_FIELD_NUMBER = 1
final val AMT_FIELD_NUMBER = 2
final val AMT_MSAT_FIELD_NUMBER = 12
final val PAYMENT_HASH_FIELD_NUMBER = 3
final val FINAL_CLTV_DELTA_FIELD_NUMBER = 4
final val PAYMENT_ADDR_FIELD_NUMBER = 20
final val PAYMENT_REQUEST_FIELD_NUMBER = 5
final val TIMEOUT_SECONDS_FIELD_NUMBER = 6
final val FEE_LIMIT_SAT_FIELD_NUMBER = 7
final val FEE_LIMIT_MSAT_FIELD_NUMBER = 13
final val OUTGOING_CHAN_ID_FIELD_NUMBER = 8
final val OUTGOING_CHAN_IDS_FIELD_NUMBER = 19
final val LAST_HOP_PUBKEY_FIELD_NUMBER = 14
final val CLTV_LIMIT_FIELD_NUMBER = 9
final val ROUTE_HINTS_FIELD_NUMBER = 10
final val DEST_CUSTOM_RECORDS_FIELD_NUMBER = 11
final val ALLOW_SELF_PAYMENT_FIELD_NUMBER = 15
final val DEST_FEATURES_FIELD_NUMBER = 16
final val MAX_PARTS_FIELD_NUMBER = 17
final val NO_INFLIGHT_UPDATES_FIELD_NUMBER = 18
final val MAX_SHARD_SIZE_MSAT_FIELD_NUMBER = 21
final val AMP_FIELD_NUMBER = 22
final val TIME_PREF_FIELD_NUMBER = 23
@transient
private[routerrpc] val _typemapper_destCustomRecords: _root_.scalapb.TypeMapper[routerrpc.SendPaymentRequest.DestCustomRecordsEntry, (_root_.scala.Long, _root_.com.google.protobuf.ByteString)] = implicitly[_root_.scalapb.TypeMapper[routerrpc.SendPaymentRequest.DestCustomRecordsEntry, (_root_.scala.Long, _root_.com.google.protobuf.ByteString)]]
def of(
dest: _root_.com.google.protobuf.ByteString,
amt: _root_.scala.Long,
amtMsat: _root_.scala.Long,
paymentHash: _root_.com.google.protobuf.ByteString,
finalCltvDelta: _root_.scala.Int,
paymentAddr: _root_.com.google.protobuf.ByteString,
paymentRequest: _root_.scala.Predef.String,
timeoutSeconds: _root_.scala.Int,
feeLimitSat: _root_.scala.Long,
feeLimitMsat: _root_.scala.Long,
outgoingChanId: _root_.scala.Long,
outgoingChanIds: _root_.scala.Seq[_root_.scala.Long],
lastHopPubkey: _root_.com.google.protobuf.ByteString,
cltvLimit: _root_.scala.Int,
routeHints: _root_.scala.Seq[lnrpc.RouteHint],
destCustomRecords: _root_.scala.collection.immutable.Map[_root_.scala.Long, _root_.com.google.protobuf.ByteString],
allowSelfPayment: _root_.scala.Boolean,
destFeatures: _root_.scala.Seq[lnrpc.FeatureBit],
maxParts: _root_.scala.Int,
noInflightUpdates: _root_.scala.Boolean,
maxShardSizeMsat: _root_.scala.Long,
amp: _root_.scala.Boolean,
timePref: _root_.scala.Double
): _root_.routerrpc.SendPaymentRequest = _root_.routerrpc.SendPaymentRequest(
dest,
amt,
amtMsat,
paymentHash,
finalCltvDelta,
paymentAddr,
paymentRequest,
timeoutSeconds,
feeLimitSat,
feeLimitMsat,
outgoingChanId,
outgoingChanIds,
lastHopPubkey,
cltvLimit,
routeHints,
destCustomRecords,
allowSelfPayment,
destFeatures,
maxParts,
noInflightUpdates,
maxShardSizeMsat,
amp,
timePref
)
// @@protoc_insertion_point(GeneratedMessageCompanion[routerrpc.SendPaymentRequest])
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy