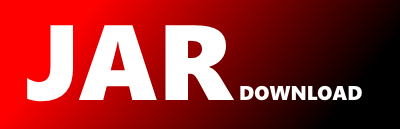
signrpc.MuSig2SessionResponse.scala Maven / Gradle / Ivy
The newest version!
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package signrpc
/** @param sessionId
*
* The unique ID that represents this signing session. A session can be used
* for producing a signature a single time. If the signing fails for any
* reason, a new session with the same participants needs to be created.
* @param combinedKey
*
* The combined public key (in the 32-byte x-only format) with all tweaks
* applied to it. If a taproot tweak is specified, this corresponds to the
* taproot key that can be put into the on-chain output.
* @param taprootInternalKey
*
* The raw combined public key (in the 32-byte x-only format) before any tweaks
* are applied to it. If a taproot tweak is specified, this corresponds to the
* internal key that needs to be put into the witness if the script spend path
* is used.
* @param localPublicNonces
*
* The two public nonces the local signer uses, combined into a single value
* of 66 bytes. Can be split into the two 33-byte points to get the individual
* nonces.
* @param haveAllNonces
*
* Indicates whether all nonces required to start the signing process are known
* now.
* @param version
*
* The version of the MuSig2 BIP that was used to create the session.
*/
@SerialVersionUID(0L)
final case class MuSig2SessionResponse(
sessionId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
combinedKey: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
taprootInternalKey: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
localPublicNonces: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
haveAllNonces: _root_.scala.Boolean = false,
version: signrpc.MuSig2Version = signrpc.MuSig2Version.MUSIG2_VERSION_UNDEFINED,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[MuSig2SessionResponse] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
{
val __value = sessionId
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(1, __value)
}
};
{
val __value = combinedKey
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(2, __value)
}
};
{
val __value = taprootInternalKey
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(3, __value)
}
};
{
val __value = localPublicNonces
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(4, __value)
}
};
{
val __value = haveAllNonces
if (__value != false) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBoolSize(5, __value)
}
};
{
val __value = version.value
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeEnumSize(6, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
{
val __v = sessionId
if (!__v.isEmpty) {
_output__.writeBytes(1, __v)
}
};
{
val __v = combinedKey
if (!__v.isEmpty) {
_output__.writeBytes(2, __v)
}
};
{
val __v = taprootInternalKey
if (!__v.isEmpty) {
_output__.writeBytes(3, __v)
}
};
{
val __v = localPublicNonces
if (!__v.isEmpty) {
_output__.writeBytes(4, __v)
}
};
{
val __v = haveAllNonces
if (__v != false) {
_output__.writeBool(5, __v)
}
};
{
val __v = version.value
if (__v != 0) {
_output__.writeEnum(6, __v)
}
};
unknownFields.writeTo(_output__)
}
def withSessionId(__v: _root_.com.google.protobuf.ByteString): MuSig2SessionResponse = copy(sessionId = __v)
def withCombinedKey(__v: _root_.com.google.protobuf.ByteString): MuSig2SessionResponse = copy(combinedKey = __v)
def withTaprootInternalKey(__v: _root_.com.google.protobuf.ByteString): MuSig2SessionResponse = copy(taprootInternalKey = __v)
def withLocalPublicNonces(__v: _root_.com.google.protobuf.ByteString): MuSig2SessionResponse = copy(localPublicNonces = __v)
def withHaveAllNonces(__v: _root_.scala.Boolean): MuSig2SessionResponse = copy(haveAllNonces = __v)
def withVersion(__v: signrpc.MuSig2Version): MuSig2SessionResponse = copy(version = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => {
val __t = sessionId
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 2 => {
val __t = combinedKey
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 3 => {
val __t = taprootInternalKey
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 4 => {
val __t = localPublicNonces
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 5 => {
val __t = haveAllNonces
if (__t != false) __t else null
}
case 6 => {
val __t = version.javaValueDescriptor
if (__t.getNumber() != 0) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => _root_.scalapb.descriptors.PByteString(sessionId)
case 2 => _root_.scalapb.descriptors.PByteString(combinedKey)
case 3 => _root_.scalapb.descriptors.PByteString(taprootInternalKey)
case 4 => _root_.scalapb.descriptors.PByteString(localPublicNonces)
case 5 => _root_.scalapb.descriptors.PBoolean(haveAllNonces)
case 6 => _root_.scalapb.descriptors.PEnum(version.scalaValueDescriptor)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: signrpc.MuSig2SessionResponse.type = signrpc.MuSig2SessionResponse
// @@protoc_insertion_point(GeneratedMessage[signrpc.MuSig2SessionResponse])
}
object MuSig2SessionResponse extends scalapb.GeneratedMessageCompanion[signrpc.MuSig2SessionResponse] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[signrpc.MuSig2SessionResponse] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): signrpc.MuSig2SessionResponse = {
var __sessionId: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __combinedKey: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __taprootInternalKey: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __localPublicNonces: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __haveAllNonces: _root_.scala.Boolean = false
var __version: signrpc.MuSig2Version = signrpc.MuSig2Version.MUSIG2_VERSION_UNDEFINED
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__sessionId = _input__.readBytes()
case 18 =>
__combinedKey = _input__.readBytes()
case 26 =>
__taprootInternalKey = _input__.readBytes()
case 34 =>
__localPublicNonces = _input__.readBytes()
case 40 =>
__haveAllNonces = _input__.readBool()
case 48 =>
__version = signrpc.MuSig2Version.fromValue(_input__.readEnum())
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
signrpc.MuSig2SessionResponse(
sessionId = __sessionId,
combinedKey = __combinedKey,
taprootInternalKey = __taprootInternalKey,
localPublicNonces = __localPublicNonces,
haveAllNonces = __haveAllNonces,
version = __version,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[signrpc.MuSig2SessionResponse] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
signrpc.MuSig2SessionResponse(
sessionId = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
combinedKey = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
taprootInternalKey = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
localPublicNonces = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
haveAllNonces = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).map(_.as[_root_.scala.Boolean]).getOrElse(false),
version = signrpc.MuSig2Version.fromValue(__fieldsMap.get(scalaDescriptor.findFieldByNumber(6).get).map(_.as[_root_.scalapb.descriptors.EnumValueDescriptor]).getOrElse(signrpc.MuSig2Version.MUSIG2_VERSION_UNDEFINED.scalaValueDescriptor).number)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = SignerProto.javaDescriptor.getMessageTypes().get(19)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = SignerProto.scalaDescriptor.messages(19)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = throw new MatchError(__number)
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 6 => signrpc.MuSig2Version
}
}
lazy val defaultInstance = signrpc.MuSig2SessionResponse(
sessionId = _root_.com.google.protobuf.ByteString.EMPTY,
combinedKey = _root_.com.google.protobuf.ByteString.EMPTY,
taprootInternalKey = _root_.com.google.protobuf.ByteString.EMPTY,
localPublicNonces = _root_.com.google.protobuf.ByteString.EMPTY,
haveAllNonces = false,
version = signrpc.MuSig2Version.MUSIG2_VERSION_UNDEFINED
)
implicit class MuSig2SessionResponseLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, signrpc.MuSig2SessionResponse]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, signrpc.MuSig2SessionResponse](_l) {
def sessionId: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.sessionId)((c_, f_) => c_.copy(sessionId = f_))
def combinedKey: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.combinedKey)((c_, f_) => c_.copy(combinedKey = f_))
def taprootInternalKey: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.taprootInternalKey)((c_, f_) => c_.copy(taprootInternalKey = f_))
def localPublicNonces: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.localPublicNonces)((c_, f_) => c_.copy(localPublicNonces = f_))
def haveAllNonces: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Boolean] = field(_.haveAllNonces)((c_, f_) => c_.copy(haveAllNonces = f_))
def version: _root_.scalapb.lenses.Lens[UpperPB, signrpc.MuSig2Version] = field(_.version)((c_, f_) => c_.copy(version = f_))
}
final val SESSION_ID_FIELD_NUMBER = 1
final val COMBINED_KEY_FIELD_NUMBER = 2
final val TAPROOT_INTERNAL_KEY_FIELD_NUMBER = 3
final val LOCAL_PUBLIC_NONCES_FIELD_NUMBER = 4
final val HAVE_ALL_NONCES_FIELD_NUMBER = 5
final val VERSION_FIELD_NUMBER = 6
def of(
sessionId: _root_.com.google.protobuf.ByteString,
combinedKey: _root_.com.google.protobuf.ByteString,
taprootInternalKey: _root_.com.google.protobuf.ByteString,
localPublicNonces: _root_.com.google.protobuf.ByteString,
haveAllNonces: _root_.scala.Boolean,
version: signrpc.MuSig2Version
): _root_.signrpc.MuSig2SessionResponse = _root_.signrpc.MuSig2SessionResponse(
sessionId,
combinedKey,
taprootInternalKey,
localPublicNonces,
haveAllNonces,
version
)
// @@protoc_insertion_point(GeneratedMessageCompanion[signrpc.MuSig2SessionResponse])
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy