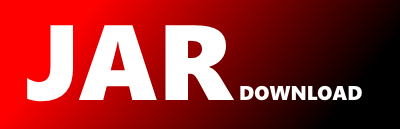
signrpc.SignDescriptor.scala Maven / Gradle / Ivy
The newest version!
// Generated by the Scala Plugin for the Protocol Buffer Compiler.
// Do not edit!
//
// Protofile syntax: PROTO3
package signrpc
/** @param keyDesc
*
* A descriptor that precisely describes *which* key to use for signing. This
* may provide the raw public key directly, or require the Signer to re-derive
* the key according to the populated derivation path.
*
* Note that if the key descriptor was obtained through walletrpc.DeriveKey,
* then the key locator MUST always be provided, since the derived keys are not
* persisted unlike with DeriveNextKey.
* @param singleTweak
*
* A scalar value that will be added to the private key corresponding to the
* above public key to obtain the private key to be used to sign this input.
* This value is typically derived via the following computation:
*
* derivedKey = privkey + sha256(perCommitmentPoint || pubKey) mod N
* @param doubleTweak
*
* A private key that will be used in combination with its corresponding
* private key to derive the private key that is to be used to sign the target
* input. Within the Lightning protocol, this value is typically the
* commitment secret from a previously revoked commitment transaction. This
* value is in combination with two hash values, and the original private key
* to derive the private key to be used when signing.
*
* k = (privKey*sha256(pubKey || tweakPub) +
* tweakPriv*sha256(tweakPub || pubKey)) mod N
* @param tapTweak
*
* The 32 byte input to the taproot tweak derivation that is used to derive
* the output key from an internal key: outputKey = internalKey +
* tagged_hash("tapTweak", internalKey || tapTweak).
*
* When doing a BIP 86 spend, this field can be an empty byte slice.
*
* When doing a normal key path spend, with the output key committing to an
* actual script root, then this field should be: the tapscript root hash.
* @param witnessScript
*
* The full script required to properly redeem the output. This field will
* only be populated if a p2tr, p2wsh or a p2sh output is being signed. If a
* taproot script path spend is being attempted, then this should be the raw
* leaf script.
* @param output
*
* A description of the output being spent. The value and script MUST be
* provided.
* @param sighash
*
* The target sighash type that should be used when generating the final
* sighash, and signature.
* @param inputIndex
*
* The target input within the transaction that should be signed.
* @param signMethod
*
* The sign method specifies how the input should be signed. Depending on the
* method, either the tap_tweak, witness_script or both need to be specified.
* Defaults to SegWit v0 signing to be backward compatible with older RPC
* clients.
*/
@SerialVersionUID(0L)
final case class SignDescriptor(
keyDesc: _root_.scala.Option[signrpc.KeyDescriptor] = _root_.scala.None,
singleTweak: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
doubleTweak: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
tapTweak: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
witnessScript: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY,
output: _root_.scala.Option[signrpc.TxOut] = _root_.scala.None,
sighash: _root_.scala.Int = 0,
inputIndex: _root_.scala.Int = 0,
signMethod: signrpc.SignMethod = signrpc.SignMethod.SIGN_METHOD_WITNESS_V0,
unknownFields: _root_.scalapb.UnknownFieldSet = _root_.scalapb.UnknownFieldSet.empty
) extends scalapb.GeneratedMessage with scalapb.lenses.Updatable[SignDescriptor] {
@transient
private[this] var __serializedSizeMemoized: _root_.scala.Int = 0
private[this] def __computeSerializedSize(): _root_.scala.Int = {
var __size = 0
if (keyDesc.isDefined) {
val __value = keyDesc.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
{
val __value = singleTweak
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(2, __value)
}
};
{
val __value = doubleTweak
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(3, __value)
}
};
{
val __value = tapTweak
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(10, __value)
}
};
{
val __value = witnessScript
if (!__value.isEmpty) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeBytesSize(4, __value)
}
};
if (output.isDefined) {
val __value = output.get
__size += 1 + _root_.com.google.protobuf.CodedOutputStream.computeUInt32SizeNoTag(__value.serializedSize) + __value.serializedSize
};
{
val __value = sighash
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeUInt32Size(7, __value)
}
};
{
val __value = inputIndex
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeInt32Size(8, __value)
}
};
{
val __value = signMethod.value
if (__value != 0) {
__size += _root_.com.google.protobuf.CodedOutputStream.computeEnumSize(9, __value)
}
};
__size += unknownFields.serializedSize
__size
}
override def serializedSize: _root_.scala.Int = {
var __size = __serializedSizeMemoized
if (__size == 0) {
__size = __computeSerializedSize() + 1
__serializedSizeMemoized = __size
}
__size - 1
}
def writeTo(`_output__`: _root_.com.google.protobuf.CodedOutputStream): _root_.scala.Unit = {
keyDesc.foreach { __v =>
val __m = __v
_output__.writeTag(1, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = singleTweak
if (!__v.isEmpty) {
_output__.writeBytes(2, __v)
}
};
{
val __v = doubleTweak
if (!__v.isEmpty) {
_output__.writeBytes(3, __v)
}
};
{
val __v = witnessScript
if (!__v.isEmpty) {
_output__.writeBytes(4, __v)
}
};
output.foreach { __v =>
val __m = __v
_output__.writeTag(5, 2)
_output__.writeUInt32NoTag(__m.serializedSize)
__m.writeTo(_output__)
};
{
val __v = sighash
if (__v != 0) {
_output__.writeUInt32(7, __v)
}
};
{
val __v = inputIndex
if (__v != 0) {
_output__.writeInt32(8, __v)
}
};
{
val __v = signMethod.value
if (__v != 0) {
_output__.writeEnum(9, __v)
}
};
{
val __v = tapTweak
if (!__v.isEmpty) {
_output__.writeBytes(10, __v)
}
};
unknownFields.writeTo(_output__)
}
def getKeyDesc: signrpc.KeyDescriptor = keyDesc.getOrElse(signrpc.KeyDescriptor.defaultInstance)
def clearKeyDesc: SignDescriptor = copy(keyDesc = _root_.scala.None)
def withKeyDesc(__v: signrpc.KeyDescriptor): SignDescriptor = copy(keyDesc = Option(__v))
def withSingleTweak(__v: _root_.com.google.protobuf.ByteString): SignDescriptor = copy(singleTweak = __v)
def withDoubleTweak(__v: _root_.com.google.protobuf.ByteString): SignDescriptor = copy(doubleTweak = __v)
def withTapTweak(__v: _root_.com.google.protobuf.ByteString): SignDescriptor = copy(tapTweak = __v)
def withWitnessScript(__v: _root_.com.google.protobuf.ByteString): SignDescriptor = copy(witnessScript = __v)
def getOutput: signrpc.TxOut = output.getOrElse(signrpc.TxOut.defaultInstance)
def clearOutput: SignDescriptor = copy(output = _root_.scala.None)
def withOutput(__v: signrpc.TxOut): SignDescriptor = copy(output = Option(__v))
def withSighash(__v: _root_.scala.Int): SignDescriptor = copy(sighash = __v)
def withInputIndex(__v: _root_.scala.Int): SignDescriptor = copy(inputIndex = __v)
def withSignMethod(__v: signrpc.SignMethod): SignDescriptor = copy(signMethod = __v)
def withUnknownFields(__v: _root_.scalapb.UnknownFieldSet) = copy(unknownFields = __v)
def discardUnknownFields = copy(unknownFields = _root_.scalapb.UnknownFieldSet.empty)
def getFieldByNumber(__fieldNumber: _root_.scala.Int): _root_.scala.Any = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 1 => keyDesc.orNull
case 2 => {
val __t = singleTweak
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 3 => {
val __t = doubleTweak
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 10 => {
val __t = tapTweak
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 4 => {
val __t = witnessScript
if (__t != _root_.com.google.protobuf.ByteString.EMPTY) __t else null
}
case 5 => output.orNull
case 7 => {
val __t = sighash
if (__t != 0) __t else null
}
case 8 => {
val __t = inputIndex
if (__t != 0) __t else null
}
case 9 => {
val __t = signMethod.javaValueDescriptor
if (__t.getNumber() != 0) __t else null
}
}
}
def getField(__field: _root_.scalapb.descriptors.FieldDescriptor): _root_.scalapb.descriptors.PValue = {
_root_.scala.Predef.require(__field.containingMessage eq companion.scalaDescriptor)
(__field.number: @_root_.scala.unchecked) match {
case 1 => keyDesc.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 2 => _root_.scalapb.descriptors.PByteString(singleTweak)
case 3 => _root_.scalapb.descriptors.PByteString(doubleTweak)
case 10 => _root_.scalapb.descriptors.PByteString(tapTweak)
case 4 => _root_.scalapb.descriptors.PByteString(witnessScript)
case 5 => output.map(_.toPMessage).getOrElse(_root_.scalapb.descriptors.PEmpty)
case 7 => _root_.scalapb.descriptors.PInt(sighash)
case 8 => _root_.scalapb.descriptors.PInt(inputIndex)
case 9 => _root_.scalapb.descriptors.PEnum(signMethod.scalaValueDescriptor)
}
}
def toProtoString: _root_.scala.Predef.String = _root_.scalapb.TextFormat.printToUnicodeString(this)
def companion: signrpc.SignDescriptor.type = signrpc.SignDescriptor
// @@protoc_insertion_point(GeneratedMessage[signrpc.SignDescriptor])
}
object SignDescriptor extends scalapb.GeneratedMessageCompanion[signrpc.SignDescriptor] {
implicit def messageCompanion: scalapb.GeneratedMessageCompanion[signrpc.SignDescriptor] = this
def parseFrom(`_input__`: _root_.com.google.protobuf.CodedInputStream): signrpc.SignDescriptor = {
var __keyDesc: _root_.scala.Option[signrpc.KeyDescriptor] = _root_.scala.None
var __singleTweak: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __doubleTweak: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __tapTweak: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __witnessScript: _root_.com.google.protobuf.ByteString = _root_.com.google.protobuf.ByteString.EMPTY
var __output: _root_.scala.Option[signrpc.TxOut] = _root_.scala.None
var __sighash: _root_.scala.Int = 0
var __inputIndex: _root_.scala.Int = 0
var __signMethod: signrpc.SignMethod = signrpc.SignMethod.SIGN_METHOD_WITNESS_V0
var `_unknownFields__`: _root_.scalapb.UnknownFieldSet.Builder = null
var _done__ = false
while (!_done__) {
val _tag__ = _input__.readTag()
_tag__ match {
case 0 => _done__ = true
case 10 =>
__keyDesc = Option(__keyDesc.fold(_root_.scalapb.LiteParser.readMessage[signrpc.KeyDescriptor](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 18 =>
__singleTweak = _input__.readBytes()
case 26 =>
__doubleTweak = _input__.readBytes()
case 82 =>
__tapTweak = _input__.readBytes()
case 34 =>
__witnessScript = _input__.readBytes()
case 42 =>
__output = Option(__output.fold(_root_.scalapb.LiteParser.readMessage[signrpc.TxOut](_input__))(_root_.scalapb.LiteParser.readMessage(_input__, _)))
case 56 =>
__sighash = _input__.readUInt32()
case 64 =>
__inputIndex = _input__.readInt32()
case 72 =>
__signMethod = signrpc.SignMethod.fromValue(_input__.readEnum())
case tag =>
if (_unknownFields__ == null) {
_unknownFields__ = new _root_.scalapb.UnknownFieldSet.Builder()
}
_unknownFields__.parseField(tag, _input__)
}
}
signrpc.SignDescriptor(
keyDesc = __keyDesc,
singleTweak = __singleTweak,
doubleTweak = __doubleTweak,
tapTweak = __tapTweak,
witnessScript = __witnessScript,
output = __output,
sighash = __sighash,
inputIndex = __inputIndex,
signMethod = __signMethod,
unknownFields = if (_unknownFields__ == null) _root_.scalapb.UnknownFieldSet.empty else _unknownFields__.result()
)
}
implicit def messageReads: _root_.scalapb.descriptors.Reads[signrpc.SignDescriptor] = _root_.scalapb.descriptors.Reads{
case _root_.scalapb.descriptors.PMessage(__fieldsMap) =>
_root_.scala.Predef.require(__fieldsMap.keys.forall(_.containingMessage eq scalaDescriptor), "FieldDescriptor does not match message type.")
signrpc.SignDescriptor(
keyDesc = __fieldsMap.get(scalaDescriptor.findFieldByNumber(1).get).flatMap(_.as[_root_.scala.Option[signrpc.KeyDescriptor]]),
singleTweak = __fieldsMap.get(scalaDescriptor.findFieldByNumber(2).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
doubleTweak = __fieldsMap.get(scalaDescriptor.findFieldByNumber(3).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
tapTweak = __fieldsMap.get(scalaDescriptor.findFieldByNumber(10).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
witnessScript = __fieldsMap.get(scalaDescriptor.findFieldByNumber(4).get).map(_.as[_root_.com.google.protobuf.ByteString]).getOrElse(_root_.com.google.protobuf.ByteString.EMPTY),
output = __fieldsMap.get(scalaDescriptor.findFieldByNumber(5).get).flatMap(_.as[_root_.scala.Option[signrpc.TxOut]]),
sighash = __fieldsMap.get(scalaDescriptor.findFieldByNumber(7).get).map(_.as[_root_.scala.Int]).getOrElse(0),
inputIndex = __fieldsMap.get(scalaDescriptor.findFieldByNumber(8).get).map(_.as[_root_.scala.Int]).getOrElse(0),
signMethod = signrpc.SignMethod.fromValue(__fieldsMap.get(scalaDescriptor.findFieldByNumber(9).get).map(_.as[_root_.scalapb.descriptors.EnumValueDescriptor]).getOrElse(signrpc.SignMethod.SIGN_METHOD_WITNESS_V0.scalaValueDescriptor).number)
)
case _ => throw new RuntimeException("Expected PMessage")
}
def javaDescriptor: _root_.com.google.protobuf.Descriptors.Descriptor = SignerProto.javaDescriptor.getMessageTypes().get(3)
def scalaDescriptor: _root_.scalapb.descriptors.Descriptor = SignerProto.scalaDescriptor.messages(3)
def messageCompanionForFieldNumber(__number: _root_.scala.Int): _root_.scalapb.GeneratedMessageCompanion[_] = {
var __out: _root_.scalapb.GeneratedMessageCompanion[_] = null
(__number: @_root_.scala.unchecked) match {
case 1 => __out = signrpc.KeyDescriptor
case 5 => __out = signrpc.TxOut
}
__out
}
lazy val nestedMessagesCompanions: Seq[_root_.scalapb.GeneratedMessageCompanion[_ <: _root_.scalapb.GeneratedMessage]] = Seq.empty
def enumCompanionForFieldNumber(__fieldNumber: _root_.scala.Int): _root_.scalapb.GeneratedEnumCompanion[_] = {
(__fieldNumber: @_root_.scala.unchecked) match {
case 9 => signrpc.SignMethod
}
}
lazy val defaultInstance = signrpc.SignDescriptor(
keyDesc = _root_.scala.None,
singleTweak = _root_.com.google.protobuf.ByteString.EMPTY,
doubleTweak = _root_.com.google.protobuf.ByteString.EMPTY,
tapTweak = _root_.com.google.protobuf.ByteString.EMPTY,
witnessScript = _root_.com.google.protobuf.ByteString.EMPTY,
output = _root_.scala.None,
sighash = 0,
inputIndex = 0,
signMethod = signrpc.SignMethod.SIGN_METHOD_WITNESS_V0
)
implicit class SignDescriptorLens[UpperPB](_l: _root_.scalapb.lenses.Lens[UpperPB, signrpc.SignDescriptor]) extends _root_.scalapb.lenses.ObjectLens[UpperPB, signrpc.SignDescriptor](_l) {
def keyDesc: _root_.scalapb.lenses.Lens[UpperPB, signrpc.KeyDescriptor] = field(_.getKeyDesc)((c_, f_) => c_.copy(keyDesc = Option(f_)))
def optionalKeyDesc: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[signrpc.KeyDescriptor]] = field(_.keyDesc)((c_, f_) => c_.copy(keyDesc = f_))
def singleTweak: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.singleTweak)((c_, f_) => c_.copy(singleTweak = f_))
def doubleTweak: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.doubleTweak)((c_, f_) => c_.copy(doubleTweak = f_))
def tapTweak: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.tapTweak)((c_, f_) => c_.copy(tapTweak = f_))
def witnessScript: _root_.scalapb.lenses.Lens[UpperPB, _root_.com.google.protobuf.ByteString] = field(_.witnessScript)((c_, f_) => c_.copy(witnessScript = f_))
def output: _root_.scalapb.lenses.Lens[UpperPB, signrpc.TxOut] = field(_.getOutput)((c_, f_) => c_.copy(output = Option(f_)))
def optionalOutput: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Option[signrpc.TxOut]] = field(_.output)((c_, f_) => c_.copy(output = f_))
def sighash: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.sighash)((c_, f_) => c_.copy(sighash = f_))
def inputIndex: _root_.scalapb.lenses.Lens[UpperPB, _root_.scala.Int] = field(_.inputIndex)((c_, f_) => c_.copy(inputIndex = f_))
def signMethod: _root_.scalapb.lenses.Lens[UpperPB, signrpc.SignMethod] = field(_.signMethod)((c_, f_) => c_.copy(signMethod = f_))
}
final val KEY_DESC_FIELD_NUMBER = 1
final val SINGLE_TWEAK_FIELD_NUMBER = 2
final val DOUBLE_TWEAK_FIELD_NUMBER = 3
final val TAP_TWEAK_FIELD_NUMBER = 10
final val WITNESS_SCRIPT_FIELD_NUMBER = 4
final val OUTPUT_FIELD_NUMBER = 5
final val SIGHASH_FIELD_NUMBER = 7
final val INPUT_INDEX_FIELD_NUMBER = 8
final val SIGN_METHOD_FIELD_NUMBER = 9
def of(
keyDesc: _root_.scala.Option[signrpc.KeyDescriptor],
singleTweak: _root_.com.google.protobuf.ByteString,
doubleTweak: _root_.com.google.protobuf.ByteString,
tapTweak: _root_.com.google.protobuf.ByteString,
witnessScript: _root_.com.google.protobuf.ByteString,
output: _root_.scala.Option[signrpc.TxOut],
sighash: _root_.scala.Int,
inputIndex: _root_.scala.Int,
signMethod: signrpc.SignMethod
): _root_.signrpc.SignDescriptor = _root_.signrpc.SignDescriptor(
keyDesc,
singleTweak,
doubleTweak,
tapTweak,
witnessScript,
output,
sighash,
inputIndex,
signMethod
)
// @@protoc_insertion_point(GeneratedMessageCompanion[signrpc.SignDescriptor])
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy