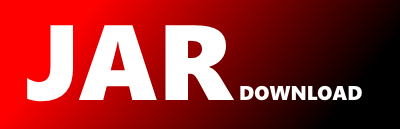
signrpc.Signer.scala Maven / Gradle / Ivy
The newest version!
// Generated by Pekko gRPC. DO NOT EDIT.
package signrpc
import org.apache.pekko
import pekko.annotation.ApiMayChange
import pekko.grpc.PekkoGrpcGenerated
/**
* Signer is a service that gives access to the signing functionality of the
* daemon's wallet.
*/
@PekkoGrpcGenerated
trait Signer {
/**
* SignOutputRaw is a method that can be used to generated a signature for a
* set of inputs/outputs to a transaction. Each request specifies details
* concerning how the outputs should be signed, which keys they should be
* signed with, and also any optional tweaks. The return value is a fixed
* 64-byte signature (the same format as we use on the wire in Lightning).
* If we are unable to sign using the specified keys, then an error will be
* returned.
*/
def signOutputRaw(in: signrpc.SignReq): scala.concurrent.Future[signrpc.SignResp]
/**
* ComputeInputScript generates a complete InputIndex for the passed
* transaction with the signature as defined within the passed SignDescriptor.
* This method should be capable of generating the proper input script for both
* regular p2wkh/p2tr outputs and p2wkh outputs nested within a regular p2sh
* output.
* Note that when using this method to sign inputs belonging to the wallet,
* the only items of the SignDescriptor that need to be populated are pkScript
* in the TxOut field, the value in that same field, and finally the input
* index.
*/
def computeInputScript(in: signrpc.SignReq): scala.concurrent.Future[signrpc.InputScriptResp]
/**
* SignMessage signs a message with the key specified in the key locator. The
* returned signature is fixed-size LN wire format encoded.
* The main difference to SignMessage in the main RPC is that a specific key is
* used to sign the message instead of the node identity private key.
*/
def signMessage(in: signrpc.SignMessageReq): scala.concurrent.Future[signrpc.SignMessageResp]
/**
* VerifyMessage verifies a signature over a message using the public key
* provided. The signature must be fixed-size LN wire format encoded.
* The main difference to VerifyMessage in the main RPC is that the public key
* used to sign the message does not have to be a node known to the network.
*/
def verifyMessage(in: signrpc.VerifyMessageReq): scala.concurrent.Future[signrpc.VerifyMessageResp]
/**
* DeriveSharedKey returns a shared secret key by performing Diffie-Hellman key
* derivation between the ephemeral public key in the request and the node's
* key specified in the key_desc parameter. Either a key locator or a raw
* public key is expected in the key_desc, if neither is supplied, defaults to
* the node's identity private key:
* P_shared = privKeyNode * ephemeralPubkey
* The resulting shared public key is serialized in the compressed format and
* hashed with sha256, resulting in the final key length of 256bit.
*/
def deriveSharedKey(in: signrpc.SharedKeyRequest): scala.concurrent.Future[signrpc.SharedKeyResponse]
/**
* MuSig2CombineKeys (experimental!) is a stateless helper RPC that can be used
* to calculate the combined MuSig2 public key from a list of all participating
* signers' public keys. This RPC is completely stateless and deterministic and
* does not create any signing session. It can be used to determine the Taproot
* public key that should be put in an on-chain output once all public keys are
* known. A signing session is only needed later when that output should be
* _spent_ again.
* NOTE: The MuSig2 BIP is not final yet and therefore this API must be
* considered to be HIGHLY EXPERIMENTAL and subject to change in upcoming
* releases. Backward compatibility is not guaranteed!
*/
def muSig2CombineKeys(in: signrpc.MuSig2CombineKeysRequest): scala.concurrent.Future[signrpc.MuSig2CombineKeysResponse]
/**
* MuSig2CreateSession (experimental!) creates a new MuSig2 signing session
* using the local key identified by the key locator. The complete list of all
* public keys of all signing parties must be provided, including the public
* key of the local signing key. If nonces of other parties are already known,
* they can be submitted as well to reduce the number of RPC calls necessary
* later on.
* NOTE: The MuSig2 BIP is not final yet and therefore this API must be
* considered to be HIGHLY EXPERIMENTAL and subject to change in upcoming
* releases. Backward compatibility is not guaranteed!
*/
def muSig2CreateSession(in: signrpc.MuSig2SessionRequest): scala.concurrent.Future[signrpc.MuSig2SessionResponse]
/**
* MuSig2RegisterNonces (experimental!) registers one or more public nonces of
* other signing participants for a session identified by its ID. This RPC can
* be called multiple times until all nonces are registered.
* NOTE: The MuSig2 BIP is not final yet and therefore this API must be
* considered to be HIGHLY EXPERIMENTAL and subject to change in upcoming
* releases. Backward compatibility is not guaranteed!
*/
def muSig2RegisterNonces(in: signrpc.MuSig2RegisterNoncesRequest): scala.concurrent.Future[signrpc.MuSig2RegisterNoncesResponse]
/**
* MuSig2Sign (experimental!) creates a partial signature using the local
* signing key that was specified when the session was created. This can only
* be called when all public nonces of all participants are known and have been
* registered with the session. If this node isn't responsible for combining
* all the partial signatures, then the cleanup flag should be set, indicating
* that the session can be removed from memory once the signature was produced.
* NOTE: The MuSig2 BIP is not final yet and therefore this API must be
* considered to be HIGHLY EXPERIMENTAL and subject to change in upcoming
* releases. Backward compatibility is not guaranteed!
*/
def muSig2Sign(in: signrpc.MuSig2SignRequest): scala.concurrent.Future[signrpc.MuSig2SignResponse]
/**
* MuSig2CombineSig (experimental!) combines the given partial signature(s)
* with the local one, if it already exists. Once a partial signature of all
* participants is registered, the final signature will be combined and
* returned.
* NOTE: The MuSig2 BIP is not final yet and therefore this API must be
* considered to be HIGHLY EXPERIMENTAL and subject to change in upcoming
* releases. Backward compatibility is not guaranteed!
*/
def muSig2CombineSig(in: signrpc.MuSig2CombineSigRequest): scala.concurrent.Future[signrpc.MuSig2CombineSigResponse]
/**
* MuSig2Cleanup (experimental!) allows a caller to clean up a session early in
* cases where it's obvious that the signing session won't succeed and the
* resources can be released.
* NOTE: The MuSig2 BIP is not final yet and therefore this API must be
* considered to be HIGHLY EXPERIMENTAL and subject to change in upcoming
* releases. Backward compatibility is not guaranteed!
*/
def muSig2Cleanup(in: signrpc.MuSig2CleanupRequest): scala.concurrent.Future[signrpc.MuSig2CleanupResponse]
}
@PekkoGrpcGenerated
object Signer extends pekko.grpc.ServiceDescription {
val name = "signrpc.Signer"
val descriptor: com.google.protobuf.Descriptors.FileDescriptor =
signrpc.SignerProto.javaDescriptor;
object Serializers {
import pekko.grpc.scaladsl.ScalapbProtobufSerializer
val SignReqSerializer = new ScalapbProtobufSerializer(signrpc.SignReq.messageCompanion)
val SignMessageReqSerializer = new ScalapbProtobufSerializer(signrpc.SignMessageReq.messageCompanion)
val VerifyMessageReqSerializer = new ScalapbProtobufSerializer(signrpc.VerifyMessageReq.messageCompanion)
val SharedKeyRequestSerializer = new ScalapbProtobufSerializer(signrpc.SharedKeyRequest.messageCompanion)
val MuSig2CombineKeysRequestSerializer = new ScalapbProtobufSerializer(signrpc.MuSig2CombineKeysRequest.messageCompanion)
val MuSig2SessionRequestSerializer = new ScalapbProtobufSerializer(signrpc.MuSig2SessionRequest.messageCompanion)
val MuSig2RegisterNoncesRequestSerializer = new ScalapbProtobufSerializer(signrpc.MuSig2RegisterNoncesRequest.messageCompanion)
val MuSig2SignRequestSerializer = new ScalapbProtobufSerializer(signrpc.MuSig2SignRequest.messageCompanion)
val MuSig2CombineSigRequestSerializer = new ScalapbProtobufSerializer(signrpc.MuSig2CombineSigRequest.messageCompanion)
val MuSig2CleanupRequestSerializer = new ScalapbProtobufSerializer(signrpc.MuSig2CleanupRequest.messageCompanion)
val SignRespSerializer = new ScalapbProtobufSerializer(signrpc.SignResp.messageCompanion)
val InputScriptRespSerializer = new ScalapbProtobufSerializer(signrpc.InputScriptResp.messageCompanion)
val SignMessageRespSerializer = new ScalapbProtobufSerializer(signrpc.SignMessageResp.messageCompanion)
val VerifyMessageRespSerializer = new ScalapbProtobufSerializer(signrpc.VerifyMessageResp.messageCompanion)
val SharedKeyResponseSerializer = new ScalapbProtobufSerializer(signrpc.SharedKeyResponse.messageCompanion)
val MuSig2CombineKeysResponseSerializer = new ScalapbProtobufSerializer(signrpc.MuSig2CombineKeysResponse.messageCompanion)
val MuSig2SessionResponseSerializer = new ScalapbProtobufSerializer(signrpc.MuSig2SessionResponse.messageCompanion)
val MuSig2RegisterNoncesResponseSerializer = new ScalapbProtobufSerializer(signrpc.MuSig2RegisterNoncesResponse.messageCompanion)
val MuSig2SignResponseSerializer = new ScalapbProtobufSerializer(signrpc.MuSig2SignResponse.messageCompanion)
val MuSig2CombineSigResponseSerializer = new ScalapbProtobufSerializer(signrpc.MuSig2CombineSigResponse.messageCompanion)
val MuSig2CleanupResponseSerializer = new ScalapbProtobufSerializer(signrpc.MuSig2CleanupResponse.messageCompanion)
}
@ApiMayChange
@PekkoGrpcGenerated
object MethodDescriptors {
import pekko.grpc.internal.Marshaller
import io.grpc.MethodDescriptor
import Serializers._
val signOutputRawDescriptor: MethodDescriptor[signrpc.SignReq, signrpc.SignResp] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("signrpc.Signer", "SignOutputRaw"))
.setRequestMarshaller(new Marshaller(SignReqSerializer))
.setResponseMarshaller(new Marshaller(SignRespSerializer))
.setSampledToLocalTracing(true)
.build()
val computeInputScriptDescriptor: MethodDescriptor[signrpc.SignReq, signrpc.InputScriptResp] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("signrpc.Signer", "ComputeInputScript"))
.setRequestMarshaller(new Marshaller(SignReqSerializer))
.setResponseMarshaller(new Marshaller(InputScriptRespSerializer))
.setSampledToLocalTracing(true)
.build()
val signMessageDescriptor: MethodDescriptor[signrpc.SignMessageReq, signrpc.SignMessageResp] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("signrpc.Signer", "SignMessage"))
.setRequestMarshaller(new Marshaller(SignMessageReqSerializer))
.setResponseMarshaller(new Marshaller(SignMessageRespSerializer))
.setSampledToLocalTracing(true)
.build()
val verifyMessageDescriptor: MethodDescriptor[signrpc.VerifyMessageReq, signrpc.VerifyMessageResp] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("signrpc.Signer", "VerifyMessage"))
.setRequestMarshaller(new Marshaller(VerifyMessageReqSerializer))
.setResponseMarshaller(new Marshaller(VerifyMessageRespSerializer))
.setSampledToLocalTracing(true)
.build()
val deriveSharedKeyDescriptor: MethodDescriptor[signrpc.SharedKeyRequest, signrpc.SharedKeyResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("signrpc.Signer", "DeriveSharedKey"))
.setRequestMarshaller(new Marshaller(SharedKeyRequestSerializer))
.setResponseMarshaller(new Marshaller(SharedKeyResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val muSig2CombineKeysDescriptor: MethodDescriptor[signrpc.MuSig2CombineKeysRequest, signrpc.MuSig2CombineKeysResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("signrpc.Signer", "MuSig2CombineKeys"))
.setRequestMarshaller(new Marshaller(MuSig2CombineKeysRequestSerializer))
.setResponseMarshaller(new Marshaller(MuSig2CombineKeysResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val muSig2CreateSessionDescriptor: MethodDescriptor[signrpc.MuSig2SessionRequest, signrpc.MuSig2SessionResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("signrpc.Signer", "MuSig2CreateSession"))
.setRequestMarshaller(new Marshaller(MuSig2SessionRequestSerializer))
.setResponseMarshaller(new Marshaller(MuSig2SessionResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val muSig2RegisterNoncesDescriptor: MethodDescriptor[signrpc.MuSig2RegisterNoncesRequest, signrpc.MuSig2RegisterNoncesResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("signrpc.Signer", "MuSig2RegisterNonces"))
.setRequestMarshaller(new Marshaller(MuSig2RegisterNoncesRequestSerializer))
.setResponseMarshaller(new Marshaller(MuSig2RegisterNoncesResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val muSig2SignDescriptor: MethodDescriptor[signrpc.MuSig2SignRequest, signrpc.MuSig2SignResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("signrpc.Signer", "MuSig2Sign"))
.setRequestMarshaller(new Marshaller(MuSig2SignRequestSerializer))
.setResponseMarshaller(new Marshaller(MuSig2SignResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val muSig2CombineSigDescriptor: MethodDescriptor[signrpc.MuSig2CombineSigRequest, signrpc.MuSig2CombineSigResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("signrpc.Signer", "MuSig2CombineSig"))
.setRequestMarshaller(new Marshaller(MuSig2CombineSigRequestSerializer))
.setResponseMarshaller(new Marshaller(MuSig2CombineSigResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val muSig2CleanupDescriptor: MethodDescriptor[signrpc.MuSig2CleanupRequest, signrpc.MuSig2CleanupResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("signrpc.Signer", "MuSig2Cleanup"))
.setRequestMarshaller(new Marshaller(MuSig2CleanupRequestSerializer))
.setResponseMarshaller(new Marshaller(MuSig2CleanupResponseSerializer))
.setSampledToLocalTracing(true)
.build()
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy