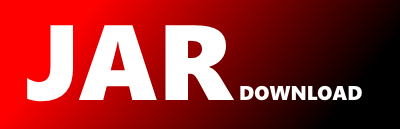
walletrpc.WalletKit.scala Maven / Gradle / Ivy
The newest version!
// Generated by Pekko gRPC. DO NOT EDIT.
package walletrpc
import org.apache.pekko
import pekko.annotation.ApiMayChange
import pekko.grpc.PekkoGrpcGenerated
/**
* WalletKit is a service that gives access to the core functionalities of the
* daemon's wallet.
*/
@PekkoGrpcGenerated
trait WalletKit {
/**
* ListUnspent returns a list of all utxos spendable by the wallet with a
* number of confirmations between the specified minimum and maximum. By
* default, all utxos are listed. To list only the unconfirmed utxos, set
* the unconfirmed_only to true.
*/
def listUnspent(in: walletrpc.ListUnspentRequest): scala.concurrent.Future[walletrpc.ListUnspentResponse]
/**
* LeaseOutput locks an output to the given ID, preventing it from being
* available for any future coin selection attempts. The absolute time of the
* lock's expiration is returned. The expiration of the lock can be extended by
* successive invocations of this RPC. Outputs can be unlocked before their
* expiration through `ReleaseOutput`.
*/
def leaseOutput(in: walletrpc.LeaseOutputRequest): scala.concurrent.Future[walletrpc.LeaseOutputResponse]
/**
* ReleaseOutput unlocks an output, allowing it to be available for coin
* selection if it remains unspent. The ID should match the one used to
* originally lock the output.
*/
def releaseOutput(in: walletrpc.ReleaseOutputRequest): scala.concurrent.Future[walletrpc.ReleaseOutputResponse]
/**
* ListLeases lists all currently locked utxos.
*/
def listLeases(in: walletrpc.ListLeasesRequest): scala.concurrent.Future[walletrpc.ListLeasesResponse]
/**
* DeriveNextKey attempts to derive the *next* key within the key family
* (account in BIP43) specified. This method should return the next external
* child within this branch.
*/
def deriveNextKey(in: walletrpc.KeyReq): scala.concurrent.Future[signrpc.KeyDescriptor]
/**
* DeriveKey attempts to derive an arbitrary key specified by the passed
* KeyLocator.
*/
def deriveKey(in: signrpc.KeyLocator): scala.concurrent.Future[signrpc.KeyDescriptor]
/**
* NextAddr returns the next unused address within the wallet.
*/
def nextAddr(in: walletrpc.AddrRequest): scala.concurrent.Future[walletrpc.AddrResponse]
/**
* ListAccounts retrieves all accounts belonging to the wallet by default. A
* name and key scope filter can be provided to filter through all of the
* wallet accounts and return only those matching.
*/
def listAccounts(in: walletrpc.ListAccountsRequest): scala.concurrent.Future[walletrpc.ListAccountsResponse]
/**
* RequiredReserve returns the minimum amount of satoshis that should be kept
* in the wallet in order to fee bump anchor channels if necessary. The value
* scales with the number of public anchor channels but is capped at a maximum.
*/
def requiredReserve(in: walletrpc.RequiredReserveRequest): scala.concurrent.Future[walletrpc.RequiredReserveResponse]
/**
* ListAddresses retrieves all the addresses along with their balance. An
* account name filter can be provided to filter through all of the
* wallet accounts and return the addresses of only those matching.
*/
def listAddresses(in: walletrpc.ListAddressesRequest): scala.concurrent.Future[walletrpc.ListAddressesResponse]
/**
* SignMessageWithAddr returns the compact signature (base64 encoded) created
* with the private key of the provided address. This requires the address
* to be solely based on a public key lock (no scripts). Obviously the internal
* lnd wallet has to possess the private key of the address otherwise
* an error is returned.
* This method aims to provide full compatibility with the bitcoin-core and
* btcd implementation. Bitcoin-core's algorithm is not specified in a
* BIP and only applicable for legacy addresses. This method enhances the
* signing for additional address types: P2WKH, NP2WKH, P2TR.
* For P2TR addresses this represents a special case. ECDSA is used to create
* a compact signature which makes the public key of the signature recoverable.
*/
def signMessageWithAddr(in: walletrpc.SignMessageWithAddrRequest): scala.concurrent.Future[walletrpc.SignMessageWithAddrResponse]
/**
* VerifyMessageWithAddr returns the validity and the recovered public key of
* the provided compact signature (base64 encoded). The verification is
* twofold. First the validity of the signature itself is checked and then
* it is verified that the recovered public key of the signature equals
* the public key of the provided address. There is no dependence on the
* private key of the address therefore also external addresses are allowed
* to verify signatures.
* Supported address types are P2PKH, P2WKH, NP2WKH, P2TR.
* This method is the counterpart of the related signing method
* (SignMessageWithAddr) and aims to provide full compatibility to
* bitcoin-core's implementation. Although bitcoin-core/btcd only provide
* this functionality for legacy addresses this function enhances it to
* the address types: P2PKH, P2WKH, NP2WKH, P2TR.
* The verification for P2TR addresses is a special case and requires the
* ECDSA compact signature to compare the reovered public key to the internal
* taproot key. The compact ECDSA signature format was used because there
* are still no known compact signature schemes for schnorr signatures.
*/
def verifyMessageWithAddr(in: walletrpc.VerifyMessageWithAddrRequest): scala.concurrent.Future[walletrpc.VerifyMessageWithAddrResponse]
/**
* ImportAccount imports an account backed by an account extended public key.
* The master key fingerprint denotes the fingerprint of the root key
* corresponding to the account public key (also known as the key with
* derivation path m/). This may be required by some hardware wallets for
* proper identification and signing.
* The address type can usually be inferred from the key's version, but may be
* required for certain keys to map them into the proper scope.
* For BIP-0044 keys, an address type must be specified as we intend to not
* support importing BIP-0044 keys into the wallet using the legacy
* pay-to-pubkey-hash (P2PKH) scheme. A nested witness address type will force
* the standard BIP-0049 derivation scheme, while a witness address type will
* force the standard BIP-0084 derivation scheme.
* For BIP-0049 keys, an address type must also be specified to make a
* distinction between the standard BIP-0049 address schema (nested witness
* pubkeys everywhere) and our own BIP-0049Plus address schema (nested pubkeys
* externally, witness pubkeys internally).
* NOTE: Events (deposits/spends) for keys derived from an account will only be
* detected by lnd if they happen after the import. Rescans to detect past
* events will be supported later on.
*/
def importAccount(in: walletrpc.ImportAccountRequest): scala.concurrent.Future[walletrpc.ImportAccountResponse]
/**
* ImportPublicKey imports a public key as watch-only into the wallet. The
* public key is converted into a simple address of the given type and that
* address script is watched on chain. For Taproot keys, this will only watch
* the BIP-0086 style output script. Use ImportTapscript for more advanced key
* spend or script spend outputs.
* NOTE: Events (deposits/spends) for a key will only be detected by lnd if
* they happen after the import. Rescans to detect past events will be
* supported later on.
*/
def importPublicKey(in: walletrpc.ImportPublicKeyRequest): scala.concurrent.Future[walletrpc.ImportPublicKeyResponse]
/**
* ImportTapscript imports a Taproot script and internal key and adds the
* resulting Taproot output key as a watch-only output script into the wallet.
* For BIP-0086 style Taproot keys (no root hash commitment and no script spend
* path) use ImportPublicKey.
* NOTE: Events (deposits/spends) for a key will only be detected by lnd if
* they happen after the import. Rescans to detect past events will be
* supported later on.
* NOTE: Taproot keys imported through this RPC currently _cannot_ be used for
* funding PSBTs. Only tracking the balance and UTXOs is currently supported.
*/
def importTapscript(in: walletrpc.ImportTapscriptRequest): scala.concurrent.Future[walletrpc.ImportTapscriptResponse]
/**
* PublishTransaction attempts to publish the passed transaction to the
* network. Once this returns without an error, the wallet will continually
* attempt to re-broadcast the transaction on start up, until it enters the
* chain.
*/
def publishTransaction(in: walletrpc.Transaction): scala.concurrent.Future[walletrpc.PublishResponse]
/**
* SendOutputs is similar to the existing sendmany call in Bitcoind, and
* allows the caller to create a transaction that sends to several outputs at
* once. This is ideal when wanting to batch create a set of transactions.
*/
def sendOutputs(in: walletrpc.SendOutputsRequest): scala.concurrent.Future[walletrpc.SendOutputsResponse]
/**
* EstimateFee attempts to query the internal fee estimator of the wallet to
* determine the fee (in sat/kw) to attach to a transaction in order to
* achieve the confirmation target.
*/
def estimateFee(in: walletrpc.EstimateFeeRequest): scala.concurrent.Future[walletrpc.EstimateFeeResponse]
/**
* PendingSweeps returns lists of on-chain outputs that lnd is currently
* attempting to sweep within its central batching engine. Outputs with similar
* fee rates are batched together in order to sweep them within a single
* transaction.
* NOTE: Some of the fields within PendingSweepsRequest are not guaranteed to
* remain supported. This is an advanced API that depends on the internals of
* the UtxoSweeper, so things may change.
*/
def pendingSweeps(in: walletrpc.PendingSweepsRequest): scala.concurrent.Future[walletrpc.PendingSweepsResponse]
/**
* BumpFee bumps the fee of an arbitrary input within a transaction. This RPC
* takes a different approach than bitcoind's bumpfee command. lnd has a
* central batching engine in which inputs with similar fee rates are batched
* together to save on transaction fees. Due to this, we cannot rely on
* bumping the fee on a specific transaction, since transactions can change at
* any point with the addition of new inputs. The list of inputs that
* currently exist within lnd's central batching engine can be retrieved
* through the PendingSweeps RPC.
* When bumping the fee of an input that currently exists within lnd's central
* batching engine, a higher fee transaction will be created that replaces the
* lower fee transaction through the Replace-By-Fee (RBF) policy. If it
* This RPC also serves useful when wanting to perform a Child-Pays-For-Parent
* (CPFP), where the child transaction pays for its parent's fee. This can be
* done by specifying an outpoint within the low fee transaction that is under
* the control of the wallet.
* The fee preference can be expressed either as a specific fee rate or a delta
* of blocks in which the output should be swept on-chain within. If a fee
* preference is not explicitly specified, then an error is returned.
* Note that this RPC currently doesn't perform any validation checks on the
* fee preference being provided. For now, the responsibility of ensuring that
* the new fee preference is sufficient is delegated to the user.
*/
def bumpFee(in: walletrpc.BumpFeeRequest): scala.concurrent.Future[walletrpc.BumpFeeResponse]
/**
* ListSweeps returns a list of the sweep transactions our node has produced.
* Note that these sweeps may not be confirmed yet, as we record sweeps on
* broadcast, not confirmation.
*/
def listSweeps(in: walletrpc.ListSweepsRequest): scala.concurrent.Future[walletrpc.ListSweepsResponse]
/**
* LabelTransaction adds a label to a transaction. If the transaction already
* has a label the call will fail unless the overwrite bool is set. This will
* overwrite the exiting transaction label. Labels must not be empty, and
* cannot exceed 500 characters.
*/
def labelTransaction(in: walletrpc.LabelTransactionRequest): scala.concurrent.Future[walletrpc.LabelTransactionResponse]
/**
* FundPsbt creates a fully populated PSBT that contains enough inputs to fund
* the outputs specified in the template. There are two ways of specifying a
* template: Either by passing in a PSBT with at least one output declared or
* by passing in a raw TxTemplate message.
* If there are no inputs specified in the template, coin selection is
* performed automatically. If the template does contain any inputs, it is
* assumed that full coin selection happened externally and no additional
* inputs are added. If the specified inputs aren't enough to fund the outputs
* with the given fee rate, an error is returned.
* After either selecting or verifying the inputs, all input UTXOs are locked
* with an internal app ID.
* NOTE: If this method returns without an error, it is the caller's
* responsibility to either spend the locked UTXOs (by finalizing and then
* publishing the transaction) or to unlock/release the locked UTXOs in case of
* an error on the caller's side.
*/
def fundPsbt(in: walletrpc.FundPsbtRequest): scala.concurrent.Future[walletrpc.FundPsbtResponse]
/**
* SignPsbt expects a partial transaction with all inputs and outputs fully
* declared and tries to sign all unsigned inputs that have all required fields
* (UTXO information, BIP32 derivation information, witness or sig scripts)
* set.
* If no error is returned, the PSBT is ready to be given to the next signer or
* to be finalized if lnd was the last signer.
* NOTE: This RPC only signs inputs (and only those it can sign), it does not
* perform any other tasks (such as coin selection, UTXO locking or
* input/output/fee value validation, PSBT finalization). Any input that is
* incomplete will be skipped.
*/
def signPsbt(in: walletrpc.SignPsbtRequest): scala.concurrent.Future[walletrpc.SignPsbtResponse]
/**
* FinalizePsbt expects a partial transaction with all inputs and outputs fully
* declared and tries to sign all inputs that belong to the wallet. Lnd must be
* the last signer of the transaction. That means, if there are any unsigned
* non-witness inputs or inputs without UTXO information attached or inputs
* without witness data that do not belong to lnd's wallet, this method will
* fail. If no error is returned, the PSBT is ready to be extracted and the
* final TX within to be broadcast.
* NOTE: This method does NOT publish the transaction once finalized. It is the
* caller's responsibility to either publish the transaction on success or
* unlock/release any locked UTXOs in case of an error in this method.
*/
def finalizePsbt(in: walletrpc.FinalizePsbtRequest): scala.concurrent.Future[walletrpc.FinalizePsbtResponse]
}
@PekkoGrpcGenerated
object WalletKit extends pekko.grpc.ServiceDescription {
val name = "walletrpc.WalletKit"
val descriptor: com.google.protobuf.Descriptors.FileDescriptor =
walletrpc.WalletkitProto.javaDescriptor;
object Serializers {
import pekko.grpc.scaladsl.ScalapbProtobufSerializer
val ListUnspentRequestSerializer = new ScalapbProtobufSerializer(walletrpc.ListUnspentRequest.messageCompanion)
val LeaseOutputRequestSerializer = new ScalapbProtobufSerializer(walletrpc.LeaseOutputRequest.messageCompanion)
val ReleaseOutputRequestSerializer = new ScalapbProtobufSerializer(walletrpc.ReleaseOutputRequest.messageCompanion)
val ListLeasesRequestSerializer = new ScalapbProtobufSerializer(walletrpc.ListLeasesRequest.messageCompanion)
val KeyReqSerializer = new ScalapbProtobufSerializer(walletrpc.KeyReq.messageCompanion)
val signrpc_KeyLocatorSerializer = new ScalapbProtobufSerializer(signrpc.KeyLocator.messageCompanion)
val AddrRequestSerializer = new ScalapbProtobufSerializer(walletrpc.AddrRequest.messageCompanion)
val ListAccountsRequestSerializer = new ScalapbProtobufSerializer(walletrpc.ListAccountsRequest.messageCompanion)
val RequiredReserveRequestSerializer = new ScalapbProtobufSerializer(walletrpc.RequiredReserveRequest.messageCompanion)
val ListAddressesRequestSerializer = new ScalapbProtobufSerializer(walletrpc.ListAddressesRequest.messageCompanion)
val SignMessageWithAddrRequestSerializer = new ScalapbProtobufSerializer(walletrpc.SignMessageWithAddrRequest.messageCompanion)
val VerifyMessageWithAddrRequestSerializer = new ScalapbProtobufSerializer(walletrpc.VerifyMessageWithAddrRequest.messageCompanion)
val ImportAccountRequestSerializer = new ScalapbProtobufSerializer(walletrpc.ImportAccountRequest.messageCompanion)
val ImportPublicKeyRequestSerializer = new ScalapbProtobufSerializer(walletrpc.ImportPublicKeyRequest.messageCompanion)
val ImportTapscriptRequestSerializer = new ScalapbProtobufSerializer(walletrpc.ImportTapscriptRequest.messageCompanion)
val TransactionSerializer = new ScalapbProtobufSerializer(walletrpc.Transaction.messageCompanion)
val SendOutputsRequestSerializer = new ScalapbProtobufSerializer(walletrpc.SendOutputsRequest.messageCompanion)
val EstimateFeeRequestSerializer = new ScalapbProtobufSerializer(walletrpc.EstimateFeeRequest.messageCompanion)
val PendingSweepsRequestSerializer = new ScalapbProtobufSerializer(walletrpc.PendingSweepsRequest.messageCompanion)
val BumpFeeRequestSerializer = new ScalapbProtobufSerializer(walletrpc.BumpFeeRequest.messageCompanion)
val ListSweepsRequestSerializer = new ScalapbProtobufSerializer(walletrpc.ListSweepsRequest.messageCompanion)
val LabelTransactionRequestSerializer = new ScalapbProtobufSerializer(walletrpc.LabelTransactionRequest.messageCompanion)
val FundPsbtRequestSerializer = new ScalapbProtobufSerializer(walletrpc.FundPsbtRequest.messageCompanion)
val SignPsbtRequestSerializer = new ScalapbProtobufSerializer(walletrpc.SignPsbtRequest.messageCompanion)
val FinalizePsbtRequestSerializer = new ScalapbProtobufSerializer(walletrpc.FinalizePsbtRequest.messageCompanion)
val ListUnspentResponseSerializer = new ScalapbProtobufSerializer(walletrpc.ListUnspentResponse.messageCompanion)
val LeaseOutputResponseSerializer = new ScalapbProtobufSerializer(walletrpc.LeaseOutputResponse.messageCompanion)
val ReleaseOutputResponseSerializer = new ScalapbProtobufSerializer(walletrpc.ReleaseOutputResponse.messageCompanion)
val ListLeasesResponseSerializer = new ScalapbProtobufSerializer(walletrpc.ListLeasesResponse.messageCompanion)
val signrpc_KeyDescriptorSerializer = new ScalapbProtobufSerializer(signrpc.KeyDescriptor.messageCompanion)
val AddrResponseSerializer = new ScalapbProtobufSerializer(walletrpc.AddrResponse.messageCompanion)
val ListAccountsResponseSerializer = new ScalapbProtobufSerializer(walletrpc.ListAccountsResponse.messageCompanion)
val RequiredReserveResponseSerializer = new ScalapbProtobufSerializer(walletrpc.RequiredReserveResponse.messageCompanion)
val ListAddressesResponseSerializer = new ScalapbProtobufSerializer(walletrpc.ListAddressesResponse.messageCompanion)
val SignMessageWithAddrResponseSerializer = new ScalapbProtobufSerializer(walletrpc.SignMessageWithAddrResponse.messageCompanion)
val VerifyMessageWithAddrResponseSerializer = new ScalapbProtobufSerializer(walletrpc.VerifyMessageWithAddrResponse.messageCompanion)
val ImportAccountResponseSerializer = new ScalapbProtobufSerializer(walletrpc.ImportAccountResponse.messageCompanion)
val ImportPublicKeyResponseSerializer = new ScalapbProtobufSerializer(walletrpc.ImportPublicKeyResponse.messageCompanion)
val ImportTapscriptResponseSerializer = new ScalapbProtobufSerializer(walletrpc.ImportTapscriptResponse.messageCompanion)
val PublishResponseSerializer = new ScalapbProtobufSerializer(walletrpc.PublishResponse.messageCompanion)
val SendOutputsResponseSerializer = new ScalapbProtobufSerializer(walletrpc.SendOutputsResponse.messageCompanion)
val EstimateFeeResponseSerializer = new ScalapbProtobufSerializer(walletrpc.EstimateFeeResponse.messageCompanion)
val PendingSweepsResponseSerializer = new ScalapbProtobufSerializer(walletrpc.PendingSweepsResponse.messageCompanion)
val BumpFeeResponseSerializer = new ScalapbProtobufSerializer(walletrpc.BumpFeeResponse.messageCompanion)
val ListSweepsResponseSerializer = new ScalapbProtobufSerializer(walletrpc.ListSweepsResponse.messageCompanion)
val LabelTransactionResponseSerializer = new ScalapbProtobufSerializer(walletrpc.LabelTransactionResponse.messageCompanion)
val FundPsbtResponseSerializer = new ScalapbProtobufSerializer(walletrpc.FundPsbtResponse.messageCompanion)
val SignPsbtResponseSerializer = new ScalapbProtobufSerializer(walletrpc.SignPsbtResponse.messageCompanion)
val FinalizePsbtResponseSerializer = new ScalapbProtobufSerializer(walletrpc.FinalizePsbtResponse.messageCompanion)
}
@ApiMayChange
@PekkoGrpcGenerated
object MethodDescriptors {
import pekko.grpc.internal.Marshaller
import io.grpc.MethodDescriptor
import Serializers._
val listUnspentDescriptor: MethodDescriptor[walletrpc.ListUnspentRequest, walletrpc.ListUnspentResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "ListUnspent"))
.setRequestMarshaller(new Marshaller(ListUnspentRequestSerializer))
.setResponseMarshaller(new Marshaller(ListUnspentResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val leaseOutputDescriptor: MethodDescriptor[walletrpc.LeaseOutputRequest, walletrpc.LeaseOutputResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "LeaseOutput"))
.setRequestMarshaller(new Marshaller(LeaseOutputRequestSerializer))
.setResponseMarshaller(new Marshaller(LeaseOutputResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val releaseOutputDescriptor: MethodDescriptor[walletrpc.ReleaseOutputRequest, walletrpc.ReleaseOutputResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "ReleaseOutput"))
.setRequestMarshaller(new Marshaller(ReleaseOutputRequestSerializer))
.setResponseMarshaller(new Marshaller(ReleaseOutputResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val listLeasesDescriptor: MethodDescriptor[walletrpc.ListLeasesRequest, walletrpc.ListLeasesResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "ListLeases"))
.setRequestMarshaller(new Marshaller(ListLeasesRequestSerializer))
.setResponseMarshaller(new Marshaller(ListLeasesResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val deriveNextKeyDescriptor: MethodDescriptor[walletrpc.KeyReq, signrpc.KeyDescriptor] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "DeriveNextKey"))
.setRequestMarshaller(new Marshaller(KeyReqSerializer))
.setResponseMarshaller(new Marshaller(signrpc_KeyDescriptorSerializer))
.setSampledToLocalTracing(true)
.build()
val deriveKeyDescriptor: MethodDescriptor[signrpc.KeyLocator, signrpc.KeyDescriptor] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "DeriveKey"))
.setRequestMarshaller(new Marshaller(signrpc_KeyLocatorSerializer))
.setResponseMarshaller(new Marshaller(signrpc_KeyDescriptorSerializer))
.setSampledToLocalTracing(true)
.build()
val nextAddrDescriptor: MethodDescriptor[walletrpc.AddrRequest, walletrpc.AddrResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "NextAddr"))
.setRequestMarshaller(new Marshaller(AddrRequestSerializer))
.setResponseMarshaller(new Marshaller(AddrResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val listAccountsDescriptor: MethodDescriptor[walletrpc.ListAccountsRequest, walletrpc.ListAccountsResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "ListAccounts"))
.setRequestMarshaller(new Marshaller(ListAccountsRequestSerializer))
.setResponseMarshaller(new Marshaller(ListAccountsResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val requiredReserveDescriptor: MethodDescriptor[walletrpc.RequiredReserveRequest, walletrpc.RequiredReserveResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "RequiredReserve"))
.setRequestMarshaller(new Marshaller(RequiredReserveRequestSerializer))
.setResponseMarshaller(new Marshaller(RequiredReserveResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val listAddressesDescriptor: MethodDescriptor[walletrpc.ListAddressesRequest, walletrpc.ListAddressesResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "ListAddresses"))
.setRequestMarshaller(new Marshaller(ListAddressesRequestSerializer))
.setResponseMarshaller(new Marshaller(ListAddressesResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val signMessageWithAddrDescriptor: MethodDescriptor[walletrpc.SignMessageWithAddrRequest, walletrpc.SignMessageWithAddrResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "SignMessageWithAddr"))
.setRequestMarshaller(new Marshaller(SignMessageWithAddrRequestSerializer))
.setResponseMarshaller(new Marshaller(SignMessageWithAddrResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val verifyMessageWithAddrDescriptor: MethodDescriptor[walletrpc.VerifyMessageWithAddrRequest, walletrpc.VerifyMessageWithAddrResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "VerifyMessageWithAddr"))
.setRequestMarshaller(new Marshaller(VerifyMessageWithAddrRequestSerializer))
.setResponseMarshaller(new Marshaller(VerifyMessageWithAddrResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val importAccountDescriptor: MethodDescriptor[walletrpc.ImportAccountRequest, walletrpc.ImportAccountResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "ImportAccount"))
.setRequestMarshaller(new Marshaller(ImportAccountRequestSerializer))
.setResponseMarshaller(new Marshaller(ImportAccountResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val importPublicKeyDescriptor: MethodDescriptor[walletrpc.ImportPublicKeyRequest, walletrpc.ImportPublicKeyResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "ImportPublicKey"))
.setRequestMarshaller(new Marshaller(ImportPublicKeyRequestSerializer))
.setResponseMarshaller(new Marshaller(ImportPublicKeyResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val importTapscriptDescriptor: MethodDescriptor[walletrpc.ImportTapscriptRequest, walletrpc.ImportTapscriptResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "ImportTapscript"))
.setRequestMarshaller(new Marshaller(ImportTapscriptRequestSerializer))
.setResponseMarshaller(new Marshaller(ImportTapscriptResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val publishTransactionDescriptor: MethodDescriptor[walletrpc.Transaction, walletrpc.PublishResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "PublishTransaction"))
.setRequestMarshaller(new Marshaller(TransactionSerializer))
.setResponseMarshaller(new Marshaller(PublishResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val sendOutputsDescriptor: MethodDescriptor[walletrpc.SendOutputsRequest, walletrpc.SendOutputsResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "SendOutputs"))
.setRequestMarshaller(new Marshaller(SendOutputsRequestSerializer))
.setResponseMarshaller(new Marshaller(SendOutputsResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val estimateFeeDescriptor: MethodDescriptor[walletrpc.EstimateFeeRequest, walletrpc.EstimateFeeResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "EstimateFee"))
.setRequestMarshaller(new Marshaller(EstimateFeeRequestSerializer))
.setResponseMarshaller(new Marshaller(EstimateFeeResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val pendingSweepsDescriptor: MethodDescriptor[walletrpc.PendingSweepsRequest, walletrpc.PendingSweepsResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "PendingSweeps"))
.setRequestMarshaller(new Marshaller(PendingSweepsRequestSerializer))
.setResponseMarshaller(new Marshaller(PendingSweepsResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val bumpFeeDescriptor: MethodDescriptor[walletrpc.BumpFeeRequest, walletrpc.BumpFeeResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "BumpFee"))
.setRequestMarshaller(new Marshaller(BumpFeeRequestSerializer))
.setResponseMarshaller(new Marshaller(BumpFeeResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val listSweepsDescriptor: MethodDescriptor[walletrpc.ListSweepsRequest, walletrpc.ListSweepsResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "ListSweeps"))
.setRequestMarshaller(new Marshaller(ListSweepsRequestSerializer))
.setResponseMarshaller(new Marshaller(ListSweepsResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val labelTransactionDescriptor: MethodDescriptor[walletrpc.LabelTransactionRequest, walletrpc.LabelTransactionResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "LabelTransaction"))
.setRequestMarshaller(new Marshaller(LabelTransactionRequestSerializer))
.setResponseMarshaller(new Marshaller(LabelTransactionResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val fundPsbtDescriptor: MethodDescriptor[walletrpc.FundPsbtRequest, walletrpc.FundPsbtResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "FundPsbt"))
.setRequestMarshaller(new Marshaller(FundPsbtRequestSerializer))
.setResponseMarshaller(new Marshaller(FundPsbtResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val signPsbtDescriptor: MethodDescriptor[walletrpc.SignPsbtRequest, walletrpc.SignPsbtResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "SignPsbt"))
.setRequestMarshaller(new Marshaller(SignPsbtRequestSerializer))
.setResponseMarshaller(new Marshaller(SignPsbtResponseSerializer))
.setSampledToLocalTracing(true)
.build()
val finalizePsbtDescriptor: MethodDescriptor[walletrpc.FinalizePsbtRequest, walletrpc.FinalizePsbtResponse] =
MethodDescriptor.newBuilder()
.setType(
MethodDescriptor.MethodType.UNARY
)
.setFullMethodName(MethodDescriptor.generateFullMethodName("walletrpc.WalletKit", "FinalizePsbt"))
.setRequestMarshaller(new Marshaller(FinalizePsbtRequestSerializer))
.setResponseMarshaller(new Marshaller(FinalizePsbtResponseSerializer))
.setSampledToLocalTracing(true)
.build()
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy