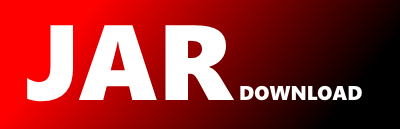
walletrpc.WalletKitClient.scala Maven / Gradle / Ivy
The newest version!
// Generated by Pekko gRPC. DO NOT EDIT.
package walletrpc
import scala.concurrent.ExecutionContext
import org.apache.pekko
import pekko.actor.ClassicActorSystemProvider
import pekko.grpc.GrpcChannel
import pekko.grpc.GrpcClientCloseException
import pekko.grpc.GrpcClientSettings
import pekko.grpc.scaladsl.PekkoGrpcClient
import pekko.grpc.internal.NettyClientUtils
import pekko.grpc.PekkoGrpcGenerated
import pekko.grpc.scaladsl.SingleResponseRequestBuilder
import pekko.grpc.internal.ScalaUnaryRequestBuilder
// Not sealed so users can extend to write their stubs
@PekkoGrpcGenerated
trait WalletKitClient extends WalletKit with WalletKitClientPowerApi with PekkoGrpcClient
@PekkoGrpcGenerated
object WalletKitClient {
def apply(settings: GrpcClientSettings)(implicit sys: ClassicActorSystemProvider): WalletKitClient =
new DefaultWalletKitClient(GrpcChannel(settings), isChannelOwned = true)
def apply(channel: GrpcChannel)(implicit sys: ClassicActorSystemProvider): WalletKitClient =
new DefaultWalletKitClient(channel, isChannelOwned = false)
private class DefaultWalletKitClient(channel: GrpcChannel, isChannelOwned: Boolean)(implicit sys: ClassicActorSystemProvider) extends WalletKitClient {
import WalletKit.MethodDescriptors._
private implicit val ex: ExecutionContext = sys.classicSystem.dispatcher
private val settings = channel.settings
private val options = NettyClientUtils.callOptions(settings)
private def listUnspentRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(listUnspentDescriptor, channel, options, settings)
private def leaseOutputRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(leaseOutputDescriptor, channel, options, settings)
private def releaseOutputRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(releaseOutputDescriptor, channel, options, settings)
private def listLeasesRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(listLeasesDescriptor, channel, options, settings)
private def deriveNextKeyRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(deriveNextKeyDescriptor, channel, options, settings)
private def deriveKeyRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(deriveKeyDescriptor, channel, options, settings)
private def nextAddrRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(nextAddrDescriptor, channel, options, settings)
private def listAccountsRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(listAccountsDescriptor, channel, options, settings)
private def requiredReserveRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(requiredReserveDescriptor, channel, options, settings)
private def listAddressesRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(listAddressesDescriptor, channel, options, settings)
private def signMessageWithAddrRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(signMessageWithAddrDescriptor, channel, options, settings)
private def verifyMessageWithAddrRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(verifyMessageWithAddrDescriptor, channel, options, settings)
private def importAccountRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(importAccountDescriptor, channel, options, settings)
private def importPublicKeyRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(importPublicKeyDescriptor, channel, options, settings)
private def importTapscriptRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(importTapscriptDescriptor, channel, options, settings)
private def publishTransactionRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(publishTransactionDescriptor, channel, options, settings)
private def sendOutputsRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(sendOutputsDescriptor, channel, options, settings)
private def estimateFeeRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(estimateFeeDescriptor, channel, options, settings)
private def pendingSweepsRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(pendingSweepsDescriptor, channel, options, settings)
private def bumpFeeRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(bumpFeeDescriptor, channel, options, settings)
private def listSweepsRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(listSweepsDescriptor, channel, options, settings)
private def labelTransactionRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(labelTransactionDescriptor, channel, options, settings)
private def fundPsbtRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(fundPsbtDescriptor, channel, options, settings)
private def signPsbtRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(signPsbtDescriptor, channel, options, settings)
private def finalizePsbtRequestBuilder(channel: pekko.grpc.internal.InternalChannel) =
new ScalaUnaryRequestBuilder(finalizePsbtDescriptor, channel, options, settings)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer listUnspent(walletrpc.ListUnspentRequest) if possible.
*/
override def listUnspent(): SingleResponseRequestBuilder[walletrpc.ListUnspentRequest, walletrpc.ListUnspentResponse] =
listUnspentRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of listUnspent
*/
def listUnspent(in: walletrpc.ListUnspentRequest): scala.concurrent.Future[walletrpc.ListUnspentResponse] =
listUnspent().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer leaseOutput(walletrpc.LeaseOutputRequest) if possible.
*/
override def leaseOutput(): SingleResponseRequestBuilder[walletrpc.LeaseOutputRequest, walletrpc.LeaseOutputResponse] =
leaseOutputRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of leaseOutput
*/
def leaseOutput(in: walletrpc.LeaseOutputRequest): scala.concurrent.Future[walletrpc.LeaseOutputResponse] =
leaseOutput().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer releaseOutput(walletrpc.ReleaseOutputRequest) if possible.
*/
override def releaseOutput(): SingleResponseRequestBuilder[walletrpc.ReleaseOutputRequest, walletrpc.ReleaseOutputResponse] =
releaseOutputRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of releaseOutput
*/
def releaseOutput(in: walletrpc.ReleaseOutputRequest): scala.concurrent.Future[walletrpc.ReleaseOutputResponse] =
releaseOutput().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer listLeases(walletrpc.ListLeasesRequest) if possible.
*/
override def listLeases(): SingleResponseRequestBuilder[walletrpc.ListLeasesRequest, walletrpc.ListLeasesResponse] =
listLeasesRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of listLeases
*/
def listLeases(in: walletrpc.ListLeasesRequest): scala.concurrent.Future[walletrpc.ListLeasesResponse] =
listLeases().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer deriveNextKey(walletrpc.KeyReq) if possible.
*/
override def deriveNextKey(): SingleResponseRequestBuilder[walletrpc.KeyReq, signrpc.KeyDescriptor] =
deriveNextKeyRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of deriveNextKey
*/
def deriveNextKey(in: walletrpc.KeyReq): scala.concurrent.Future[signrpc.KeyDescriptor] =
deriveNextKey().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer deriveKey(signrpc.KeyLocator) if possible.
*/
override def deriveKey(): SingleResponseRequestBuilder[signrpc.KeyLocator, signrpc.KeyDescriptor] =
deriveKeyRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of deriveKey
*/
def deriveKey(in: signrpc.KeyLocator): scala.concurrent.Future[signrpc.KeyDescriptor] =
deriveKey().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer nextAddr(walletrpc.AddrRequest) if possible.
*/
override def nextAddr(): SingleResponseRequestBuilder[walletrpc.AddrRequest, walletrpc.AddrResponse] =
nextAddrRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of nextAddr
*/
def nextAddr(in: walletrpc.AddrRequest): scala.concurrent.Future[walletrpc.AddrResponse] =
nextAddr().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer listAccounts(walletrpc.ListAccountsRequest) if possible.
*/
override def listAccounts(): SingleResponseRequestBuilder[walletrpc.ListAccountsRequest, walletrpc.ListAccountsResponse] =
listAccountsRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of listAccounts
*/
def listAccounts(in: walletrpc.ListAccountsRequest): scala.concurrent.Future[walletrpc.ListAccountsResponse] =
listAccounts().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer requiredReserve(walletrpc.RequiredReserveRequest) if possible.
*/
override def requiredReserve(): SingleResponseRequestBuilder[walletrpc.RequiredReserveRequest, walletrpc.RequiredReserveResponse] =
requiredReserveRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of requiredReserve
*/
def requiredReserve(in: walletrpc.RequiredReserveRequest): scala.concurrent.Future[walletrpc.RequiredReserveResponse] =
requiredReserve().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer listAddresses(walletrpc.ListAddressesRequest) if possible.
*/
override def listAddresses(): SingleResponseRequestBuilder[walletrpc.ListAddressesRequest, walletrpc.ListAddressesResponse] =
listAddressesRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of listAddresses
*/
def listAddresses(in: walletrpc.ListAddressesRequest): scala.concurrent.Future[walletrpc.ListAddressesResponse] =
listAddresses().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer signMessageWithAddr(walletrpc.SignMessageWithAddrRequest) if possible.
*/
override def signMessageWithAddr(): SingleResponseRequestBuilder[walletrpc.SignMessageWithAddrRequest, walletrpc.SignMessageWithAddrResponse] =
signMessageWithAddrRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of signMessageWithAddr
*/
def signMessageWithAddr(in: walletrpc.SignMessageWithAddrRequest): scala.concurrent.Future[walletrpc.SignMessageWithAddrResponse] =
signMessageWithAddr().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer verifyMessageWithAddr(walletrpc.VerifyMessageWithAddrRequest) if possible.
*/
override def verifyMessageWithAddr(): SingleResponseRequestBuilder[walletrpc.VerifyMessageWithAddrRequest, walletrpc.VerifyMessageWithAddrResponse] =
verifyMessageWithAddrRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of verifyMessageWithAddr
*/
def verifyMessageWithAddr(in: walletrpc.VerifyMessageWithAddrRequest): scala.concurrent.Future[walletrpc.VerifyMessageWithAddrResponse] =
verifyMessageWithAddr().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer importAccount(walletrpc.ImportAccountRequest) if possible.
*/
override def importAccount(): SingleResponseRequestBuilder[walletrpc.ImportAccountRequest, walletrpc.ImportAccountResponse] =
importAccountRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of importAccount
*/
def importAccount(in: walletrpc.ImportAccountRequest): scala.concurrent.Future[walletrpc.ImportAccountResponse] =
importAccount().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer importPublicKey(walletrpc.ImportPublicKeyRequest) if possible.
*/
override def importPublicKey(): SingleResponseRequestBuilder[walletrpc.ImportPublicKeyRequest, walletrpc.ImportPublicKeyResponse] =
importPublicKeyRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of importPublicKey
*/
def importPublicKey(in: walletrpc.ImportPublicKeyRequest): scala.concurrent.Future[walletrpc.ImportPublicKeyResponse] =
importPublicKey().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer importTapscript(walletrpc.ImportTapscriptRequest) if possible.
*/
override def importTapscript(): SingleResponseRequestBuilder[walletrpc.ImportTapscriptRequest, walletrpc.ImportTapscriptResponse] =
importTapscriptRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of importTapscript
*/
def importTapscript(in: walletrpc.ImportTapscriptRequest): scala.concurrent.Future[walletrpc.ImportTapscriptResponse] =
importTapscript().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer publishTransaction(walletrpc.Transaction) if possible.
*/
override def publishTransaction(): SingleResponseRequestBuilder[walletrpc.Transaction, walletrpc.PublishResponse] =
publishTransactionRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of publishTransaction
*/
def publishTransaction(in: walletrpc.Transaction): scala.concurrent.Future[walletrpc.PublishResponse] =
publishTransaction().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer sendOutputs(walletrpc.SendOutputsRequest) if possible.
*/
override def sendOutputs(): SingleResponseRequestBuilder[walletrpc.SendOutputsRequest, walletrpc.SendOutputsResponse] =
sendOutputsRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of sendOutputs
*/
def sendOutputs(in: walletrpc.SendOutputsRequest): scala.concurrent.Future[walletrpc.SendOutputsResponse] =
sendOutputs().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer estimateFee(walletrpc.EstimateFeeRequest) if possible.
*/
override def estimateFee(): SingleResponseRequestBuilder[walletrpc.EstimateFeeRequest, walletrpc.EstimateFeeResponse] =
estimateFeeRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of estimateFee
*/
def estimateFee(in: walletrpc.EstimateFeeRequest): scala.concurrent.Future[walletrpc.EstimateFeeResponse] =
estimateFee().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer pendingSweeps(walletrpc.PendingSweepsRequest) if possible.
*/
override def pendingSweeps(): SingleResponseRequestBuilder[walletrpc.PendingSweepsRequest, walletrpc.PendingSweepsResponse] =
pendingSweepsRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of pendingSweeps
*/
def pendingSweeps(in: walletrpc.PendingSweepsRequest): scala.concurrent.Future[walletrpc.PendingSweepsResponse] =
pendingSweeps().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer bumpFee(walletrpc.BumpFeeRequest) if possible.
*/
override def bumpFee(): SingleResponseRequestBuilder[walletrpc.BumpFeeRequest, walletrpc.BumpFeeResponse] =
bumpFeeRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of bumpFee
*/
def bumpFee(in: walletrpc.BumpFeeRequest): scala.concurrent.Future[walletrpc.BumpFeeResponse] =
bumpFee().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer listSweeps(walletrpc.ListSweepsRequest) if possible.
*/
override def listSweeps(): SingleResponseRequestBuilder[walletrpc.ListSweepsRequest, walletrpc.ListSweepsResponse] =
listSweepsRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of listSweeps
*/
def listSweeps(in: walletrpc.ListSweepsRequest): scala.concurrent.Future[walletrpc.ListSweepsResponse] =
listSweeps().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer labelTransaction(walletrpc.LabelTransactionRequest) if possible.
*/
override def labelTransaction(): SingleResponseRequestBuilder[walletrpc.LabelTransactionRequest, walletrpc.LabelTransactionResponse] =
labelTransactionRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of labelTransaction
*/
def labelTransaction(in: walletrpc.LabelTransactionRequest): scala.concurrent.Future[walletrpc.LabelTransactionResponse] =
labelTransaction().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer fundPsbt(walletrpc.FundPsbtRequest) if possible.
*/
override def fundPsbt(): SingleResponseRequestBuilder[walletrpc.FundPsbtRequest, walletrpc.FundPsbtResponse] =
fundPsbtRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of fundPsbt
*/
def fundPsbt(in: walletrpc.FundPsbtRequest): scala.concurrent.Future[walletrpc.FundPsbtResponse] =
fundPsbt().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer signPsbt(walletrpc.SignPsbtRequest) if possible.
*/
override def signPsbt(): SingleResponseRequestBuilder[walletrpc.SignPsbtRequest, walletrpc.SignPsbtResponse] =
signPsbtRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of signPsbt
*/
def signPsbt(in: walletrpc.SignPsbtRequest): scala.concurrent.Future[walletrpc.SignPsbtResponse] =
signPsbt().invoke(in)
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer finalizePsbt(walletrpc.FinalizePsbtRequest) if possible.
*/
override def finalizePsbt(): SingleResponseRequestBuilder[walletrpc.FinalizePsbtRequest, walletrpc.FinalizePsbtResponse] =
finalizePsbtRequestBuilder(channel.internalChannel)
/**
* For access to method metadata use the parameterless version of finalizePsbt
*/
def finalizePsbt(in: walletrpc.FinalizePsbtRequest): scala.concurrent.Future[walletrpc.FinalizePsbtResponse] =
finalizePsbt().invoke(in)
override def close(): scala.concurrent.Future[pekko.Done] =
if (isChannelOwned) channel.close()
else throw new GrpcClientCloseException()
override def closed: scala.concurrent.Future[pekko.Done] = channel.closed()
}
}
@PekkoGrpcGenerated
trait WalletKitClientPowerApi {
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer listUnspent(walletrpc.ListUnspentRequest) if possible.
*/
def listUnspent(): SingleResponseRequestBuilder[walletrpc.ListUnspentRequest, walletrpc.ListUnspentResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer leaseOutput(walletrpc.LeaseOutputRequest) if possible.
*/
def leaseOutput(): SingleResponseRequestBuilder[walletrpc.LeaseOutputRequest, walletrpc.LeaseOutputResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer releaseOutput(walletrpc.ReleaseOutputRequest) if possible.
*/
def releaseOutput(): SingleResponseRequestBuilder[walletrpc.ReleaseOutputRequest, walletrpc.ReleaseOutputResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer listLeases(walletrpc.ListLeasesRequest) if possible.
*/
def listLeases(): SingleResponseRequestBuilder[walletrpc.ListLeasesRequest, walletrpc.ListLeasesResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer deriveNextKey(walletrpc.KeyReq) if possible.
*/
def deriveNextKey(): SingleResponseRequestBuilder[walletrpc.KeyReq, signrpc.KeyDescriptor] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer deriveKey(signrpc.KeyLocator) if possible.
*/
def deriveKey(): SingleResponseRequestBuilder[signrpc.KeyLocator, signrpc.KeyDescriptor] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer nextAddr(walletrpc.AddrRequest) if possible.
*/
def nextAddr(): SingleResponseRequestBuilder[walletrpc.AddrRequest, walletrpc.AddrResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer listAccounts(walletrpc.ListAccountsRequest) if possible.
*/
def listAccounts(): SingleResponseRequestBuilder[walletrpc.ListAccountsRequest, walletrpc.ListAccountsResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer requiredReserve(walletrpc.RequiredReserveRequest) if possible.
*/
def requiredReserve(): SingleResponseRequestBuilder[walletrpc.RequiredReserveRequest, walletrpc.RequiredReserveResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer listAddresses(walletrpc.ListAddressesRequest) if possible.
*/
def listAddresses(): SingleResponseRequestBuilder[walletrpc.ListAddressesRequest, walletrpc.ListAddressesResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer signMessageWithAddr(walletrpc.SignMessageWithAddrRequest) if possible.
*/
def signMessageWithAddr(): SingleResponseRequestBuilder[walletrpc.SignMessageWithAddrRequest, walletrpc.SignMessageWithAddrResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer verifyMessageWithAddr(walletrpc.VerifyMessageWithAddrRequest) if possible.
*/
def verifyMessageWithAddr(): SingleResponseRequestBuilder[walletrpc.VerifyMessageWithAddrRequest, walletrpc.VerifyMessageWithAddrResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer importAccount(walletrpc.ImportAccountRequest) if possible.
*/
def importAccount(): SingleResponseRequestBuilder[walletrpc.ImportAccountRequest, walletrpc.ImportAccountResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer importPublicKey(walletrpc.ImportPublicKeyRequest) if possible.
*/
def importPublicKey(): SingleResponseRequestBuilder[walletrpc.ImportPublicKeyRequest, walletrpc.ImportPublicKeyResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer importTapscript(walletrpc.ImportTapscriptRequest) if possible.
*/
def importTapscript(): SingleResponseRequestBuilder[walletrpc.ImportTapscriptRequest, walletrpc.ImportTapscriptResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer publishTransaction(walletrpc.Transaction) if possible.
*/
def publishTransaction(): SingleResponseRequestBuilder[walletrpc.Transaction, walletrpc.PublishResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer sendOutputs(walletrpc.SendOutputsRequest) if possible.
*/
def sendOutputs(): SingleResponseRequestBuilder[walletrpc.SendOutputsRequest, walletrpc.SendOutputsResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer estimateFee(walletrpc.EstimateFeeRequest) if possible.
*/
def estimateFee(): SingleResponseRequestBuilder[walletrpc.EstimateFeeRequest, walletrpc.EstimateFeeResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer pendingSweeps(walletrpc.PendingSweepsRequest) if possible.
*/
def pendingSweeps(): SingleResponseRequestBuilder[walletrpc.PendingSweepsRequest, walletrpc.PendingSweepsResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer bumpFee(walletrpc.BumpFeeRequest) if possible.
*/
def bumpFee(): SingleResponseRequestBuilder[walletrpc.BumpFeeRequest, walletrpc.BumpFeeResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer listSweeps(walletrpc.ListSweepsRequest) if possible.
*/
def listSweeps(): SingleResponseRequestBuilder[walletrpc.ListSweepsRequest, walletrpc.ListSweepsResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer labelTransaction(walletrpc.LabelTransactionRequest) if possible.
*/
def labelTransaction(): SingleResponseRequestBuilder[walletrpc.LabelTransactionRequest, walletrpc.LabelTransactionResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer fundPsbt(walletrpc.FundPsbtRequest) if possible.
*/
def fundPsbt(): SingleResponseRequestBuilder[walletrpc.FundPsbtRequest, walletrpc.FundPsbtResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer signPsbt(walletrpc.SignPsbtRequest) if possible.
*/
def signPsbt(): SingleResponseRequestBuilder[walletrpc.SignPsbtRequest, walletrpc.SignPsbtResponse] = ???
/**
* Lower level "lifted" version of the method, giving access to request metadata etc.
* prefer finalizePsbt(walletrpc.FinalizePsbtRequest) if possible.
*/
def finalizePsbt(): SingleResponseRequestBuilder[walletrpc.FinalizePsbtRequest, walletrpc.FinalizePsbtResponse] = ???
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy