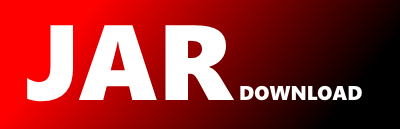
wtclientrpc.WatchtowerClientHandler.scala Maven / Gradle / Ivy
The newest version!
// Generated by Pekko gRPC. DO NOT EDIT.
package wtclientrpc
import scala.concurrent.ExecutionContext
import org.apache.pekko
import pekko.grpc.scaladsl.{ GrpcExceptionHandler, GrpcMarshalling }
import pekko.grpc.Trailers
import pekko.actor.ActorSystem
import pekko.actor.ClassicActorSystemProvider
import pekko.annotation.ApiMayChange
import pekko.http.scaladsl.model
import pekko.stream.{Materializer, SystemMaterializer}
import pekko.grpc.internal.TelemetryExtension
import pekko.grpc.PekkoGrpcGenerated
/*
* Generated by Pekko gRPC. DO NOT EDIT.
*
* The API of this class may still change in future Pekko gRPC versions, see for instance
* https://github.com/akka/akka-grpc/issues/994
*/
@ApiMayChange
@PekkoGrpcGenerated
object WatchtowerClientHandler {
private val notFound = scala.concurrent.Future.successful(model.HttpResponse(model.StatusCodes.NotFound))
private val unsupportedMediaType = scala.concurrent.Future.successful(model.HttpResponse(model.StatusCodes.UnsupportedMediaType))
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` with `WatchtowerClientHandler.partial` when combining
* several services.
*/
def apply(implementation: WatchtowerClient)(implicit system: ClassicActorSystemProvider): model.HttpRequest => scala.concurrent.Future[model.HttpResponse] =
partial(implementation).orElse { case _ => notFound }
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` with `WatchtowerClientHandler.partial` when combining
* several services.
*/
def apply(implementation: WatchtowerClient, eHandler: ActorSystem => PartialFunction[Throwable, Trailers])(implicit system: ClassicActorSystemProvider): model.HttpRequest => scala.concurrent.Future[model.HttpResponse] =
partial(implementation, WatchtowerClient.name, eHandler).orElse { case _ => notFound }
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` with `WatchtowerClientHandler.partial` when combining
* several services.
*
* Registering a gRPC service under a custom prefix is not widely supported and strongly discouraged by the specification.
*/
def apply(implementation: WatchtowerClient, prefix: String)(implicit system: ClassicActorSystemProvider): model.HttpRequest => scala.concurrent.Future[model.HttpResponse] =
partial(implementation, prefix).orElse { case _ => notFound }
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` with `WatchtowerClientHandler.partial` when combining
* several services.
*
* Registering a gRPC service under a custom prefix is not widely supported and strongly discouraged by the specification.
*/
def apply(implementation: WatchtowerClient, prefix: String, eHandler: ActorSystem => PartialFunction[Throwable, Trailers])(implicit system: ClassicActorSystemProvider): model.HttpRequest => scala.concurrent.Future[model.HttpResponse] =
partial(implementation, prefix, eHandler).orElse { case _ => notFound }
/**
* Creates a `HttpRequest` to `HttpResponse` handler that can be used in for example `Http().bindAndHandleAsync`
* for the generated partial function handler. The generated handler falls back to a reflection handler for
* `WatchtowerClient` and ends with `StatusCodes.NotFound` if the request is not matching.
*
* Use `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` with `WatchtowerClientHandler.partial` when combining
* several services.
*/
def withServerReflection(implementation: WatchtowerClient)(implicit system: ClassicActorSystemProvider): model.HttpRequest => scala.concurrent.Future[model.HttpResponse] =
pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound(
WatchtowerClientHandler.partial(implementation),
pekko.grpc.scaladsl.ServerReflection.partial(List(WatchtowerClient)))
/**
* Creates a partial `HttpRequest` to `HttpResponse` handler that can be combined with handlers of other
* services with `org.apache.pekko.grpc.scaladsl.ServiceHandler.concatOrNotFound` and then used in for example
* `Http().bindAndHandleAsync`.
*
* Use `WatchtowerClientHandler.apply` if the server is only handling one service.
*
* Registering a gRPC service under a custom prefix is not widely supported and strongly discouraged by the specification.
*/
def partial(implementation: WatchtowerClient, prefix: String = WatchtowerClient.name, eHandler: ActorSystem => PartialFunction[Throwable, Trailers] = GrpcExceptionHandler.defaultMapper)(implicit system: ClassicActorSystemProvider): PartialFunction[model.HttpRequest, scala.concurrent.Future[model.HttpResponse]] = {
implicit val mat: Materializer = SystemMaterializer(system).materializer
implicit val ec: ExecutionContext = mat.executionContext
val spi = TelemetryExtension(system).spi
import WatchtowerClient.Serializers._
def handle(request: model.HttpRequest, method: String): scala.concurrent.Future[model.HttpResponse] =
GrpcMarshalling.negotiated(request, (reader, writer) =>
(method match {
case "AddTower" =>
GrpcMarshalling.unmarshal(request.entity)(AddTowerRequestSerializer, mat, reader)
.flatMap(implementation.addTower(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(AddTowerResponseSerializer, writer, system))
case "RemoveTower" =>
GrpcMarshalling.unmarshal(request.entity)(RemoveTowerRequestSerializer, mat, reader)
.flatMap(implementation.removeTower(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(RemoveTowerResponseSerializer, writer, system))
case "ListTowers" =>
GrpcMarshalling.unmarshal(request.entity)(ListTowersRequestSerializer, mat, reader)
.flatMap(implementation.listTowers(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(ListTowersResponseSerializer, writer, system))
case "GetTowerInfo" =>
GrpcMarshalling.unmarshal(request.entity)(GetTowerInfoRequestSerializer, mat, reader)
.flatMap(implementation.getTowerInfo(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(TowerSerializer, writer, system))
case "Stats" =>
GrpcMarshalling.unmarshal(request.entity)(StatsRequestSerializer, mat, reader)
.flatMap(implementation.stats(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(StatsResponseSerializer, writer, system))
case "Policy" =>
GrpcMarshalling.unmarshal(request.entity)(PolicyRequestSerializer, mat, reader)
.flatMap(implementation.policy(_))
.map(e => GrpcMarshalling.marshal(e, eHandler)(PolicyResponseSerializer, writer, system))
case m => scala.concurrent.Future.failed(new NotImplementedError(s"Not implemented: $m"))
})
.recoverWith(GrpcExceptionHandler.from(eHandler(system.classicSystem))(system, writer))
).getOrElse(unsupportedMediaType)
Function.unlift((req: model.HttpRequest) => req.uri.path match {
case model.Uri.Path.Slash(model.Uri.Path.Segment(`prefix`, model.Uri.Path.Slash(model.Uri.Path.Segment(method, model.Uri.Path.Empty)))) =>
Some(handle(spi.onRequest(prefix, method, req), method))
case _ =>
None
})
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy