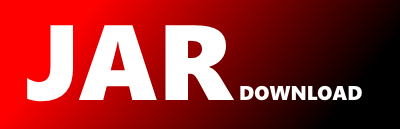
org.bitcoinj.wallet.Protos Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: wallet.proto
package org.bitcoinj.wallet;
public final class Protos {
private Protos() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface PeerAddressOrBuilder extends
// @@protoc_insertion_point(interface_extends:wallet.PeerAddress)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes ip_address = 1;
*/
boolean hasIpAddress();
/**
* required bytes ip_address = 1;
*/
com.google.protobuf.ByteString getIpAddress();
/**
* required uint32 port = 2;
*/
boolean hasPort();
/**
* required uint32 port = 2;
*/
int getPort();
/**
* required uint64 services = 3;
*/
boolean hasServices();
/**
* required uint64 services = 3;
*/
long getServices();
}
/**
* Protobuf type {@code wallet.PeerAddress}
*/
public static final class PeerAddress extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:wallet.PeerAddress)
PeerAddressOrBuilder {
private static final long serialVersionUID = 0L;
// Use PeerAddress.newBuilder() to construct.
private PeerAddress(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private PeerAddress() {
ipAddress_ = com.google.protobuf.ByteString.EMPTY;
port_ = 0;
services_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private PeerAddress(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
bitField0_ |= 0x00000001;
ipAddress_ = input.readBytes();
break;
}
case 16: {
bitField0_ |= 0x00000002;
port_ = input.readUInt32();
break;
}
case 24: {
bitField0_ |= 0x00000004;
services_ = input.readUInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_PeerAddress_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_PeerAddress_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoinj.wallet.Protos.PeerAddress.class, org.bitcoinj.wallet.Protos.PeerAddress.Builder.class);
}
private int bitField0_;
public static final int IP_ADDRESS_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString ipAddress_;
/**
* required bytes ip_address = 1;
*/
public boolean hasIpAddress() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes ip_address = 1;
*/
public com.google.protobuf.ByteString getIpAddress() {
return ipAddress_;
}
public static final int PORT_FIELD_NUMBER = 2;
private int port_;
/**
* required uint32 port = 2;
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required uint32 port = 2;
*/
public int getPort() {
return port_;
}
public static final int SERVICES_FIELD_NUMBER = 3;
private long services_;
/**
* required uint64 services = 3;
*/
public boolean hasServices() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required uint64 services = 3;
*/
public long getServices() {
return services_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasIpAddress()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasPort()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasServices()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, ipAddress_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt32(2, port_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeUInt64(3, services_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, ipAddress_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(2, port_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt64Size(3, services_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.bitcoinj.wallet.Protos.PeerAddress)) {
return super.equals(obj);
}
org.bitcoinj.wallet.Protos.PeerAddress other = (org.bitcoinj.wallet.Protos.PeerAddress) obj;
boolean result = true;
result = result && (hasIpAddress() == other.hasIpAddress());
if (hasIpAddress()) {
result = result && getIpAddress()
.equals(other.getIpAddress());
}
result = result && (hasPort() == other.hasPort());
if (hasPort()) {
result = result && (getPort()
== other.getPort());
}
result = result && (hasServices() == other.hasServices());
if (hasServices()) {
result = result && (getServices()
== other.getServices());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasIpAddress()) {
hash = (37 * hash) + IP_ADDRESS_FIELD_NUMBER;
hash = (53 * hash) + getIpAddress().hashCode();
}
if (hasPort()) {
hash = (37 * hash) + PORT_FIELD_NUMBER;
hash = (53 * hash) + getPort();
}
if (hasServices()) {
hash = (37 * hash) + SERVICES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getServices());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.bitcoinj.wallet.Protos.PeerAddress parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoinj.wallet.Protos.PeerAddress parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.PeerAddress parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoinj.wallet.Protos.PeerAddress parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.PeerAddress parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoinj.wallet.Protos.PeerAddress parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.PeerAddress parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.bitcoinj.wallet.Protos.PeerAddress parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.PeerAddress parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.bitcoinj.wallet.Protos.PeerAddress parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.PeerAddress parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.bitcoinj.wallet.Protos.PeerAddress parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.bitcoinj.wallet.Protos.PeerAddress prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wallet.PeerAddress}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:wallet.PeerAddress)
org.bitcoinj.wallet.Protos.PeerAddressOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_PeerAddress_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_PeerAddress_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoinj.wallet.Protos.PeerAddress.class, org.bitcoinj.wallet.Protos.PeerAddress.Builder.class);
}
// Construct using org.bitcoinj.wallet.Protos.PeerAddress.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
ipAddress_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
port_ = 0;
bitField0_ = (bitField0_ & ~0x00000002);
services_ = 0L;
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_PeerAddress_descriptor;
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.PeerAddress getDefaultInstanceForType() {
return org.bitcoinj.wallet.Protos.PeerAddress.getDefaultInstance();
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.PeerAddress build() {
org.bitcoinj.wallet.Protos.PeerAddress result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.PeerAddress buildPartial() {
org.bitcoinj.wallet.Protos.PeerAddress result = new org.bitcoinj.wallet.Protos.PeerAddress(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.ipAddress_ = ipAddress_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.port_ = port_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
result.services_ = services_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.bitcoinj.wallet.Protos.PeerAddress) {
return mergeFrom((org.bitcoinj.wallet.Protos.PeerAddress)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.bitcoinj.wallet.Protos.PeerAddress other) {
if (other == org.bitcoinj.wallet.Protos.PeerAddress.getDefaultInstance()) return this;
if (other.hasIpAddress()) {
setIpAddress(other.getIpAddress());
}
if (other.hasPort()) {
setPort(other.getPort());
}
if (other.hasServices()) {
setServices(other.getServices());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasIpAddress()) {
return false;
}
if (!hasPort()) {
return false;
}
if (!hasServices()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.bitcoinj.wallet.Protos.PeerAddress parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.bitcoinj.wallet.Protos.PeerAddress) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString ipAddress_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes ip_address = 1;
*/
public boolean hasIpAddress() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes ip_address = 1;
*/
public com.google.protobuf.ByteString getIpAddress() {
return ipAddress_;
}
/**
* required bytes ip_address = 1;
*/
public Builder setIpAddress(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
ipAddress_ = value;
onChanged();
return this;
}
/**
* required bytes ip_address = 1;
*/
public Builder clearIpAddress() {
bitField0_ = (bitField0_ & ~0x00000001);
ipAddress_ = getDefaultInstance().getIpAddress();
onChanged();
return this;
}
private int port_ ;
/**
* required uint32 port = 2;
*/
public boolean hasPort() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
* required uint32 port = 2;
*/
public int getPort() {
return port_;
}
/**
* required uint32 port = 2;
*/
public Builder setPort(int value) {
bitField0_ |= 0x00000002;
port_ = value;
onChanged();
return this;
}
/**
* required uint32 port = 2;
*/
public Builder clearPort() {
bitField0_ = (bitField0_ & ~0x00000002);
port_ = 0;
onChanged();
return this;
}
private long services_ ;
/**
* required uint64 services = 3;
*/
public boolean hasServices() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* required uint64 services = 3;
*/
public long getServices() {
return services_;
}
/**
* required uint64 services = 3;
*/
public Builder setServices(long value) {
bitField0_ |= 0x00000004;
services_ = value;
onChanged();
return this;
}
/**
* required uint64 services = 3;
*/
public Builder clearServices() {
bitField0_ = (bitField0_ & ~0x00000004);
services_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:wallet.PeerAddress)
}
// @@protoc_insertion_point(class_scope:wallet.PeerAddress)
private static final org.bitcoinj.wallet.Protos.PeerAddress DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.bitcoinj.wallet.Protos.PeerAddress();
}
public static org.bitcoinj.wallet.Protos.PeerAddress getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PeerAddress parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new PeerAddress(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.PeerAddress getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EncryptedDataOrBuilder extends
// @@protoc_insertion_point(interface_extends:wallet.EncryptedData)
com.google.protobuf.MessageOrBuilder {
/**
*
* The initialisation vector for the AES encryption (16 bytes)
*
*
* required bytes initialisation_vector = 1;
*/
boolean hasInitialisationVector();
/**
*
* The initialisation vector for the AES encryption (16 bytes)
*
*
* required bytes initialisation_vector = 1;
*/
com.google.protobuf.ByteString getInitialisationVector();
/**
*
* The encrypted private key
*
*
* required bytes encrypted_private_key = 2;
*/
boolean hasEncryptedPrivateKey();
/**
*
* The encrypted private key
*
*
* required bytes encrypted_private_key = 2;
*/
com.google.protobuf.ByteString getEncryptedPrivateKey();
}
/**
* Protobuf type {@code wallet.EncryptedData}
*/
public static final class EncryptedData extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:wallet.EncryptedData)
EncryptedDataOrBuilder {
private static final long serialVersionUID = 0L;
// Use EncryptedData.newBuilder() to construct.
private EncryptedData(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private EncryptedData() {
initialisationVector_ = com.google.protobuf.ByteString.EMPTY;
encryptedPrivateKey_ = com.google.protobuf.ByteString.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private EncryptedData(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
bitField0_ |= 0x00000001;
initialisationVector_ = input.readBytes();
break;
}
case 18: {
bitField0_ |= 0x00000002;
encryptedPrivateKey_ = input.readBytes();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_EncryptedData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_EncryptedData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoinj.wallet.Protos.EncryptedData.class, org.bitcoinj.wallet.Protos.EncryptedData.Builder.class);
}
private int bitField0_;
public static final int INITIALISATION_VECTOR_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString initialisationVector_;
/**
*
* The initialisation vector for the AES encryption (16 bytes)
*
*
* required bytes initialisation_vector = 1;
*/
public boolean hasInitialisationVector() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* The initialisation vector for the AES encryption (16 bytes)
*
*
* required bytes initialisation_vector = 1;
*/
public com.google.protobuf.ByteString getInitialisationVector() {
return initialisationVector_;
}
public static final int ENCRYPTED_PRIVATE_KEY_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString encryptedPrivateKey_;
/**
*
* The encrypted private key
*
*
* required bytes encrypted_private_key = 2;
*/
public boolean hasEncryptedPrivateKey() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* The encrypted private key
*
*
* required bytes encrypted_private_key = 2;
*/
public com.google.protobuf.ByteString getEncryptedPrivateKey() {
return encryptedPrivateKey_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasInitialisationVector()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasEncryptedPrivateKey()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, initialisationVector_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, encryptedPrivateKey_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, initialisationVector_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, encryptedPrivateKey_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.bitcoinj.wallet.Protos.EncryptedData)) {
return super.equals(obj);
}
org.bitcoinj.wallet.Protos.EncryptedData other = (org.bitcoinj.wallet.Protos.EncryptedData) obj;
boolean result = true;
result = result && (hasInitialisationVector() == other.hasInitialisationVector());
if (hasInitialisationVector()) {
result = result && getInitialisationVector()
.equals(other.getInitialisationVector());
}
result = result && (hasEncryptedPrivateKey() == other.hasEncryptedPrivateKey());
if (hasEncryptedPrivateKey()) {
result = result && getEncryptedPrivateKey()
.equals(other.getEncryptedPrivateKey());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasInitialisationVector()) {
hash = (37 * hash) + INITIALISATION_VECTOR_FIELD_NUMBER;
hash = (53 * hash) + getInitialisationVector().hashCode();
}
if (hasEncryptedPrivateKey()) {
hash = (37 * hash) + ENCRYPTED_PRIVATE_KEY_FIELD_NUMBER;
hash = (53 * hash) + getEncryptedPrivateKey().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.bitcoinj.wallet.Protos.EncryptedData parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoinj.wallet.Protos.EncryptedData parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.EncryptedData parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoinj.wallet.Protos.EncryptedData parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.EncryptedData parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoinj.wallet.Protos.EncryptedData parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.EncryptedData parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.bitcoinj.wallet.Protos.EncryptedData parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.EncryptedData parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.bitcoinj.wallet.Protos.EncryptedData parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.EncryptedData parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.bitcoinj.wallet.Protos.EncryptedData parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.bitcoinj.wallet.Protos.EncryptedData prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wallet.EncryptedData}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:wallet.EncryptedData)
org.bitcoinj.wallet.Protos.EncryptedDataOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_EncryptedData_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_EncryptedData_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoinj.wallet.Protos.EncryptedData.class, org.bitcoinj.wallet.Protos.EncryptedData.Builder.class);
}
// Construct using org.bitcoinj.wallet.Protos.EncryptedData.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
initialisationVector_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
encryptedPrivateKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_EncryptedData_descriptor;
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.EncryptedData getDefaultInstanceForType() {
return org.bitcoinj.wallet.Protos.EncryptedData.getDefaultInstance();
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.EncryptedData build() {
org.bitcoinj.wallet.Protos.EncryptedData result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.EncryptedData buildPartial() {
org.bitcoinj.wallet.Protos.EncryptedData result = new org.bitcoinj.wallet.Protos.EncryptedData(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.initialisationVector_ = initialisationVector_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.encryptedPrivateKey_ = encryptedPrivateKey_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.bitcoinj.wallet.Protos.EncryptedData) {
return mergeFrom((org.bitcoinj.wallet.Protos.EncryptedData)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.bitcoinj.wallet.Protos.EncryptedData other) {
if (other == org.bitcoinj.wallet.Protos.EncryptedData.getDefaultInstance()) return this;
if (other.hasInitialisationVector()) {
setInitialisationVector(other.getInitialisationVector());
}
if (other.hasEncryptedPrivateKey()) {
setEncryptedPrivateKey(other.getEncryptedPrivateKey());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasInitialisationVector()) {
return false;
}
if (!hasEncryptedPrivateKey()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.bitcoinj.wallet.Protos.EncryptedData parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.bitcoinj.wallet.Protos.EncryptedData) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString initialisationVector_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The initialisation vector for the AES encryption (16 bytes)
*
*
* required bytes initialisation_vector = 1;
*/
public boolean hasInitialisationVector() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* The initialisation vector for the AES encryption (16 bytes)
*
*
* required bytes initialisation_vector = 1;
*/
public com.google.protobuf.ByteString getInitialisationVector() {
return initialisationVector_;
}
/**
*
* The initialisation vector for the AES encryption (16 bytes)
*
*
* required bytes initialisation_vector = 1;
*/
public Builder setInitialisationVector(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
initialisationVector_ = value;
onChanged();
return this;
}
/**
*
* The initialisation vector for the AES encryption (16 bytes)
*
*
* required bytes initialisation_vector = 1;
*/
public Builder clearInitialisationVector() {
bitField0_ = (bitField0_ & ~0x00000001);
initialisationVector_ = getDefaultInstance().getInitialisationVector();
onChanged();
return this;
}
private com.google.protobuf.ByteString encryptedPrivateKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The encrypted private key
*
*
* required bytes encrypted_private_key = 2;
*/
public boolean hasEncryptedPrivateKey() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* The encrypted private key
*
*
* required bytes encrypted_private_key = 2;
*/
public com.google.protobuf.ByteString getEncryptedPrivateKey() {
return encryptedPrivateKey_;
}
/**
*
* The encrypted private key
*
*
* required bytes encrypted_private_key = 2;
*/
public Builder setEncryptedPrivateKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
encryptedPrivateKey_ = value;
onChanged();
return this;
}
/**
*
* The encrypted private key
*
*
* required bytes encrypted_private_key = 2;
*/
public Builder clearEncryptedPrivateKey() {
bitField0_ = (bitField0_ & ~0x00000002);
encryptedPrivateKey_ = getDefaultInstance().getEncryptedPrivateKey();
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:wallet.EncryptedData)
}
// @@protoc_insertion_point(class_scope:wallet.EncryptedData)
private static final org.bitcoinj.wallet.Protos.EncryptedData DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.bitcoinj.wallet.Protos.EncryptedData();
}
public static org.bitcoinj.wallet.Protos.EncryptedData getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public EncryptedData parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new EncryptedData(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.EncryptedData getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface DeterministicKeyOrBuilder extends
// @@protoc_insertion_point(interface_extends:wallet.DeterministicKey)
com.google.protobuf.MessageOrBuilder {
/**
*
* Random data that allows us to extend a key. Without this, we can't figure out the next key in the chain and
* should just treat it as a regular ORIGINAL type key.
*
*
* required bytes chain_code = 1;
*/
boolean hasChainCode();
/**
*
* Random data that allows us to extend a key. Without this, we can't figure out the next key in the chain and
* should just treat it as a regular ORIGINAL type key.
*
*
* required bytes chain_code = 1;
*/
com.google.protobuf.ByteString getChainCode();
/**
*
* The path through the key tree. Each number is encoded in the standard form: high bit set for private derivation
* and high bit unset for public derivation.
*
*
* repeated uint32 path = 2;
*/
java.util.List getPathList();
/**
*
* The path through the key tree. Each number is encoded in the standard form: high bit set for private derivation
* and high bit unset for public derivation.
*
*
* repeated uint32 path = 2;
*/
int getPathCount();
/**
*
* The path through the key tree. Each number is encoded in the standard form: high bit set for private derivation
* and high bit unset for public derivation.
*
*
* repeated uint32 path = 2;
*/
int getPath(int index);
/**
*
* How many children of this key have been issued, that is, given to the user when they requested a fresh key?
* For the parents of keys being handed out, this is always less than the true number of children: the difference is
* called the lookahead zone. These keys are put into Bloom filters so we can spot transactions made by clones of
* this wallet - for instance when restoring from backup or if the seed was shared between devices.
* If this field is missing it means we're not issuing subkeys of this key to users.
*
*
* optional uint32 issued_subkeys = 3;
*/
boolean hasIssuedSubkeys();
/**
*
* How many children of this key have been issued, that is, given to the user when they requested a fresh key?
* For the parents of keys being handed out, this is always less than the true number of children: the difference is
* called the lookahead zone. These keys are put into Bloom filters so we can spot transactions made by clones of
* this wallet - for instance when restoring from backup or if the seed was shared between devices.
* If this field is missing it means we're not issuing subkeys of this key to users.
*
*
* optional uint32 issued_subkeys = 3;
*/
int getIssuedSubkeys();
/**
* optional uint32 lookahead_size = 4;
*/
boolean hasLookaheadSize();
/**
* optional uint32 lookahead_size = 4;
*/
int getLookaheadSize();
/**
*
**
* Flag indicating that this key is a root of a following chain. This chain is following the next non-following chain.
* Following/followed chains concept is used for married keychains, where the set of keys combined together to produce
* a single P2SH multisignature address
*
*
* optional bool isFollowing = 5;
*/
boolean hasIsFollowing();
/**
*
**
* Flag indicating that this key is a root of a following chain. This chain is following the next non-following chain.
* Following/followed chains concept is used for married keychains, where the set of keys combined together to produce
* a single P2SH multisignature address
*
*
* optional bool isFollowing = 5;
*/
boolean getIsFollowing();
/**
*
* Number of signatures required to spend. This field is needed only for married keychains to reconstruct KeyChain
* and represents the N value from N-of-M CHECKMULTISIG script. For regular single keychains it will always be 1.
*
*
* optional uint32 sigsRequiredToSpend = 6 [default = 1];
*/
boolean hasSigsRequiredToSpend();
/**
*
* Number of signatures required to spend. This field is needed only for married keychains to reconstruct KeyChain
* and represents the N value from N-of-M CHECKMULTISIG script. For regular single keychains it will always be 1.
*
*
* optional uint32 sigsRequiredToSpend = 6 [default = 1];
*/
int getSigsRequiredToSpend();
}
/**
*
**
* Data attached to a Key message that defines the data needed by the BIP32 deterministic key hierarchy algorithm.
*
*
* Protobuf type {@code wallet.DeterministicKey}
*/
public static final class DeterministicKey extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:wallet.DeterministicKey)
DeterministicKeyOrBuilder {
private static final long serialVersionUID = 0L;
// Use DeterministicKey.newBuilder() to construct.
private DeterministicKey(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DeterministicKey() {
chainCode_ = com.google.protobuf.ByteString.EMPTY;
path_ = java.util.Collections.emptyList();
issuedSubkeys_ = 0;
lookaheadSize_ = 0;
isFollowing_ = false;
sigsRequiredToSpend_ = 1;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DeterministicKey(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
bitField0_ |= 0x00000001;
chainCode_ = input.readBytes();
break;
}
case 16: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
path_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
path_.add(input.readUInt32());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002) && input.getBytesUntilLimit() > 0) {
path_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
while (input.getBytesUntilLimit() > 0) {
path_.add(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 24: {
bitField0_ |= 0x00000002;
issuedSubkeys_ = input.readUInt32();
break;
}
case 32: {
bitField0_ |= 0x00000004;
lookaheadSize_ = input.readUInt32();
break;
}
case 40: {
bitField0_ |= 0x00000008;
isFollowing_ = input.readBool();
break;
}
case 48: {
bitField0_ |= 0x00000010;
sigsRequiredToSpend_ = input.readUInt32();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
path_ = java.util.Collections.unmodifiableList(path_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_DeterministicKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_DeterministicKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoinj.wallet.Protos.DeterministicKey.class, org.bitcoinj.wallet.Protos.DeterministicKey.Builder.class);
}
private int bitField0_;
public static final int CHAIN_CODE_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString chainCode_;
/**
*
* Random data that allows us to extend a key. Without this, we can't figure out the next key in the chain and
* should just treat it as a regular ORIGINAL type key.
*
*
* required bytes chain_code = 1;
*/
public boolean hasChainCode() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* Random data that allows us to extend a key. Without this, we can't figure out the next key in the chain and
* should just treat it as a regular ORIGINAL type key.
*
*
* required bytes chain_code = 1;
*/
public com.google.protobuf.ByteString getChainCode() {
return chainCode_;
}
public static final int PATH_FIELD_NUMBER = 2;
private java.util.List path_;
/**
*
* The path through the key tree. Each number is encoded in the standard form: high bit set for private derivation
* and high bit unset for public derivation.
*
*
* repeated uint32 path = 2;
*/
public java.util.List
getPathList() {
return path_;
}
/**
*
* The path through the key tree. Each number is encoded in the standard form: high bit set for private derivation
* and high bit unset for public derivation.
*
*
* repeated uint32 path = 2;
*/
public int getPathCount() {
return path_.size();
}
/**
*
* The path through the key tree. Each number is encoded in the standard form: high bit set for private derivation
* and high bit unset for public derivation.
*
*
* repeated uint32 path = 2;
*/
public int getPath(int index) {
return path_.get(index);
}
public static final int ISSUED_SUBKEYS_FIELD_NUMBER = 3;
private int issuedSubkeys_;
/**
*
* How many children of this key have been issued, that is, given to the user when they requested a fresh key?
* For the parents of keys being handed out, this is always less than the true number of children: the difference is
* called the lookahead zone. These keys are put into Bloom filters so we can spot transactions made by clones of
* this wallet - for instance when restoring from backup or if the seed was shared between devices.
* If this field is missing it means we're not issuing subkeys of this key to users.
*
*
* optional uint32 issued_subkeys = 3;
*/
public boolean hasIssuedSubkeys() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* How many children of this key have been issued, that is, given to the user when they requested a fresh key?
* For the parents of keys being handed out, this is always less than the true number of children: the difference is
* called the lookahead zone. These keys are put into Bloom filters so we can spot transactions made by clones of
* this wallet - for instance when restoring from backup or if the seed was shared between devices.
* If this field is missing it means we're not issuing subkeys of this key to users.
*
*
* optional uint32 issued_subkeys = 3;
*/
public int getIssuedSubkeys() {
return issuedSubkeys_;
}
public static final int LOOKAHEAD_SIZE_FIELD_NUMBER = 4;
private int lookaheadSize_;
/**
* optional uint32 lookahead_size = 4;
*/
public boolean hasLookaheadSize() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
* optional uint32 lookahead_size = 4;
*/
public int getLookaheadSize() {
return lookaheadSize_;
}
public static final int ISFOLLOWING_FIELD_NUMBER = 5;
private boolean isFollowing_;
/**
*
**
* Flag indicating that this key is a root of a following chain. This chain is following the next non-following chain.
* Following/followed chains concept is used for married keychains, where the set of keys combined together to produce
* a single P2SH multisignature address
*
*
* optional bool isFollowing = 5;
*/
public boolean hasIsFollowing() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
**
* Flag indicating that this key is a root of a following chain. This chain is following the next non-following chain.
* Following/followed chains concept is used for married keychains, where the set of keys combined together to produce
* a single P2SH multisignature address
*
*
* optional bool isFollowing = 5;
*/
public boolean getIsFollowing() {
return isFollowing_;
}
public static final int SIGSREQUIREDTOSPEND_FIELD_NUMBER = 6;
private int sigsRequiredToSpend_;
/**
*
* Number of signatures required to spend. This field is needed only for married keychains to reconstruct KeyChain
* and represents the N value from N-of-M CHECKMULTISIG script. For regular single keychains it will always be 1.
*
*
* optional uint32 sigsRequiredToSpend = 6 [default = 1];
*/
public boolean hasSigsRequiredToSpend() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* Number of signatures required to spend. This field is needed only for married keychains to reconstruct KeyChain
* and represents the N value from N-of-M CHECKMULTISIG script. For regular single keychains it will always be 1.
*
*
* optional uint32 sigsRequiredToSpend = 6 [default = 1];
*/
public int getSigsRequiredToSpend() {
return sigsRequiredToSpend_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasChainCode()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, chainCode_);
}
for (int i = 0; i < path_.size(); i++) {
output.writeUInt32(2, path_.get(i));
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeUInt32(3, issuedSubkeys_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeUInt32(4, lookaheadSize_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBool(5, isFollowing_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
output.writeUInt32(6, sigsRequiredToSpend_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, chainCode_);
}
{
int dataSize = 0;
for (int i = 0; i < path_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(path_.get(i));
}
size += dataSize;
size += 1 * getPathList().size();
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(3, issuedSubkeys_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(4, lookaheadSize_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, isFollowing_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(6, sigsRequiredToSpend_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.bitcoinj.wallet.Protos.DeterministicKey)) {
return super.equals(obj);
}
org.bitcoinj.wallet.Protos.DeterministicKey other = (org.bitcoinj.wallet.Protos.DeterministicKey) obj;
boolean result = true;
result = result && (hasChainCode() == other.hasChainCode());
if (hasChainCode()) {
result = result && getChainCode()
.equals(other.getChainCode());
}
result = result && getPathList()
.equals(other.getPathList());
result = result && (hasIssuedSubkeys() == other.hasIssuedSubkeys());
if (hasIssuedSubkeys()) {
result = result && (getIssuedSubkeys()
== other.getIssuedSubkeys());
}
result = result && (hasLookaheadSize() == other.hasLookaheadSize());
if (hasLookaheadSize()) {
result = result && (getLookaheadSize()
== other.getLookaheadSize());
}
result = result && (hasIsFollowing() == other.hasIsFollowing());
if (hasIsFollowing()) {
result = result && (getIsFollowing()
== other.getIsFollowing());
}
result = result && (hasSigsRequiredToSpend() == other.hasSigsRequiredToSpend());
if (hasSigsRequiredToSpend()) {
result = result && (getSigsRequiredToSpend()
== other.getSigsRequiredToSpend());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasChainCode()) {
hash = (37 * hash) + CHAIN_CODE_FIELD_NUMBER;
hash = (53 * hash) + getChainCode().hashCode();
}
if (getPathCount() > 0) {
hash = (37 * hash) + PATH_FIELD_NUMBER;
hash = (53 * hash) + getPathList().hashCode();
}
if (hasIssuedSubkeys()) {
hash = (37 * hash) + ISSUED_SUBKEYS_FIELD_NUMBER;
hash = (53 * hash) + getIssuedSubkeys();
}
if (hasLookaheadSize()) {
hash = (37 * hash) + LOOKAHEAD_SIZE_FIELD_NUMBER;
hash = (53 * hash) + getLookaheadSize();
}
if (hasIsFollowing()) {
hash = (37 * hash) + ISFOLLOWING_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsFollowing());
}
if (hasSigsRequiredToSpend()) {
hash = (37 * hash) + SIGSREQUIREDTOSPEND_FIELD_NUMBER;
hash = (53 * hash) + getSigsRequiredToSpend();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.bitcoinj.wallet.Protos.DeterministicKey parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoinj.wallet.Protos.DeterministicKey parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.DeterministicKey parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoinj.wallet.Protos.DeterministicKey parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.DeterministicKey parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoinj.wallet.Protos.DeterministicKey parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.DeterministicKey parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.bitcoinj.wallet.Protos.DeterministicKey parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.DeterministicKey parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.bitcoinj.wallet.Protos.DeterministicKey parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.DeterministicKey parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.bitcoinj.wallet.Protos.DeterministicKey parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.bitcoinj.wallet.Protos.DeterministicKey prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* Data attached to a Key message that defines the data needed by the BIP32 deterministic key hierarchy algorithm.
*
*
* Protobuf type {@code wallet.DeterministicKey}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:wallet.DeterministicKey)
org.bitcoinj.wallet.Protos.DeterministicKeyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_DeterministicKey_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_DeterministicKey_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoinj.wallet.Protos.DeterministicKey.class, org.bitcoinj.wallet.Protos.DeterministicKey.Builder.class);
}
// Construct using org.bitcoinj.wallet.Protos.DeterministicKey.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
chainCode_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
path_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
issuedSubkeys_ = 0;
bitField0_ = (bitField0_ & ~0x00000004);
lookaheadSize_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
isFollowing_ = false;
bitField0_ = (bitField0_ & ~0x00000010);
sigsRequiredToSpend_ = 1;
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_DeterministicKey_descriptor;
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.DeterministicKey getDefaultInstanceForType() {
return org.bitcoinj.wallet.Protos.DeterministicKey.getDefaultInstance();
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.DeterministicKey build() {
org.bitcoinj.wallet.Protos.DeterministicKey result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.DeterministicKey buildPartial() {
org.bitcoinj.wallet.Protos.DeterministicKey result = new org.bitcoinj.wallet.Protos.DeterministicKey(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.chainCode_ = chainCode_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
path_ = java.util.Collections.unmodifiableList(path_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.path_ = path_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000002;
}
result.issuedSubkeys_ = issuedSubkeys_;
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000004;
}
result.lookaheadSize_ = lookaheadSize_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000008;
}
result.isFollowing_ = isFollowing_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000010;
}
result.sigsRequiredToSpend_ = sigsRequiredToSpend_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.bitcoinj.wallet.Protos.DeterministicKey) {
return mergeFrom((org.bitcoinj.wallet.Protos.DeterministicKey)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.bitcoinj.wallet.Protos.DeterministicKey other) {
if (other == org.bitcoinj.wallet.Protos.DeterministicKey.getDefaultInstance()) return this;
if (other.hasChainCode()) {
setChainCode(other.getChainCode());
}
if (!other.path_.isEmpty()) {
if (path_.isEmpty()) {
path_ = other.path_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensurePathIsMutable();
path_.addAll(other.path_);
}
onChanged();
}
if (other.hasIssuedSubkeys()) {
setIssuedSubkeys(other.getIssuedSubkeys());
}
if (other.hasLookaheadSize()) {
setLookaheadSize(other.getLookaheadSize());
}
if (other.hasIsFollowing()) {
setIsFollowing(other.getIsFollowing());
}
if (other.hasSigsRequiredToSpend()) {
setSigsRequiredToSpend(other.getSigsRequiredToSpend());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasChainCode()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.bitcoinj.wallet.Protos.DeterministicKey parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.bitcoinj.wallet.Protos.DeterministicKey) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString chainCode_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Random data that allows us to extend a key. Without this, we can't figure out the next key in the chain and
* should just treat it as a regular ORIGINAL type key.
*
*
* required bytes chain_code = 1;
*/
public boolean hasChainCode() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
*
* Random data that allows us to extend a key. Without this, we can't figure out the next key in the chain and
* should just treat it as a regular ORIGINAL type key.
*
*
* required bytes chain_code = 1;
*/
public com.google.protobuf.ByteString getChainCode() {
return chainCode_;
}
/**
*
* Random data that allows us to extend a key. Without this, we can't figure out the next key in the chain and
* should just treat it as a regular ORIGINAL type key.
*
*
* required bytes chain_code = 1;
*/
public Builder setChainCode(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
chainCode_ = value;
onChanged();
return this;
}
/**
*
* Random data that allows us to extend a key. Without this, we can't figure out the next key in the chain and
* should just treat it as a regular ORIGINAL type key.
*
*
* required bytes chain_code = 1;
*/
public Builder clearChainCode() {
bitField0_ = (bitField0_ & ~0x00000001);
chainCode_ = getDefaultInstance().getChainCode();
onChanged();
return this;
}
private java.util.List path_ = java.util.Collections.emptyList();
private void ensurePathIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
path_ = new java.util.ArrayList(path_);
bitField0_ |= 0x00000002;
}
}
/**
*
* The path through the key tree. Each number is encoded in the standard form: high bit set for private derivation
* and high bit unset for public derivation.
*
*
* repeated uint32 path = 2;
*/
public java.util.List
getPathList() {
return java.util.Collections.unmodifiableList(path_);
}
/**
*
* The path through the key tree. Each number is encoded in the standard form: high bit set for private derivation
* and high bit unset for public derivation.
*
*
* repeated uint32 path = 2;
*/
public int getPathCount() {
return path_.size();
}
/**
*
* The path through the key tree. Each number is encoded in the standard form: high bit set for private derivation
* and high bit unset for public derivation.
*
*
* repeated uint32 path = 2;
*/
public int getPath(int index) {
return path_.get(index);
}
/**
*
* The path through the key tree. Each number is encoded in the standard form: high bit set for private derivation
* and high bit unset for public derivation.
*
*
* repeated uint32 path = 2;
*/
public Builder setPath(
int index, int value) {
ensurePathIsMutable();
path_.set(index, value);
onChanged();
return this;
}
/**
*
* The path through the key tree. Each number is encoded in the standard form: high bit set for private derivation
* and high bit unset for public derivation.
*
*
* repeated uint32 path = 2;
*/
public Builder addPath(int value) {
ensurePathIsMutable();
path_.add(value);
onChanged();
return this;
}
/**
*
* The path through the key tree. Each number is encoded in the standard form: high bit set for private derivation
* and high bit unset for public derivation.
*
*
* repeated uint32 path = 2;
*/
public Builder addAllPath(
java.lang.Iterable extends java.lang.Integer> values) {
ensurePathIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, path_);
onChanged();
return this;
}
/**
*
* The path through the key tree. Each number is encoded in the standard form: high bit set for private derivation
* and high bit unset for public derivation.
*
*
* repeated uint32 path = 2;
*/
public Builder clearPath() {
path_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
private int issuedSubkeys_ ;
/**
*
* How many children of this key have been issued, that is, given to the user when they requested a fresh key?
* For the parents of keys being handed out, this is always less than the true number of children: the difference is
* called the lookahead zone. These keys are put into Bloom filters so we can spot transactions made by clones of
* this wallet - for instance when restoring from backup or if the seed was shared between devices.
* If this field is missing it means we're not issuing subkeys of this key to users.
*
*
* optional uint32 issued_subkeys = 3;
*/
public boolean hasIssuedSubkeys() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* How many children of this key have been issued, that is, given to the user when they requested a fresh key?
* For the parents of keys being handed out, this is always less than the true number of children: the difference is
* called the lookahead zone. These keys are put into Bloom filters so we can spot transactions made by clones of
* this wallet - for instance when restoring from backup or if the seed was shared between devices.
* If this field is missing it means we're not issuing subkeys of this key to users.
*
*
* optional uint32 issued_subkeys = 3;
*/
public int getIssuedSubkeys() {
return issuedSubkeys_;
}
/**
*
* How many children of this key have been issued, that is, given to the user when they requested a fresh key?
* For the parents of keys being handed out, this is always less than the true number of children: the difference is
* called the lookahead zone. These keys are put into Bloom filters so we can spot transactions made by clones of
* this wallet - for instance when restoring from backup or if the seed was shared between devices.
* If this field is missing it means we're not issuing subkeys of this key to users.
*
*
* optional uint32 issued_subkeys = 3;
*/
public Builder setIssuedSubkeys(int value) {
bitField0_ |= 0x00000004;
issuedSubkeys_ = value;
onChanged();
return this;
}
/**
*
* How many children of this key have been issued, that is, given to the user when they requested a fresh key?
* For the parents of keys being handed out, this is always less than the true number of children: the difference is
* called the lookahead zone. These keys are put into Bloom filters so we can spot transactions made by clones of
* this wallet - for instance when restoring from backup or if the seed was shared between devices.
* If this field is missing it means we're not issuing subkeys of this key to users.
*
*
* optional uint32 issued_subkeys = 3;
*/
public Builder clearIssuedSubkeys() {
bitField0_ = (bitField0_ & ~0x00000004);
issuedSubkeys_ = 0;
onChanged();
return this;
}
private int lookaheadSize_ ;
/**
* optional uint32 lookahead_size = 4;
*/
public boolean hasLookaheadSize() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
* optional uint32 lookahead_size = 4;
*/
public int getLookaheadSize() {
return lookaheadSize_;
}
/**
* optional uint32 lookahead_size = 4;
*/
public Builder setLookaheadSize(int value) {
bitField0_ |= 0x00000008;
lookaheadSize_ = value;
onChanged();
return this;
}
/**
* optional uint32 lookahead_size = 4;
*/
public Builder clearLookaheadSize() {
bitField0_ = (bitField0_ & ~0x00000008);
lookaheadSize_ = 0;
onChanged();
return this;
}
private boolean isFollowing_ ;
/**
*
**
* Flag indicating that this key is a root of a following chain. This chain is following the next non-following chain.
* Following/followed chains concept is used for married keychains, where the set of keys combined together to produce
* a single P2SH multisignature address
*
*
* optional bool isFollowing = 5;
*/
public boolean hasIsFollowing() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
**
* Flag indicating that this key is a root of a following chain. This chain is following the next non-following chain.
* Following/followed chains concept is used for married keychains, where the set of keys combined together to produce
* a single P2SH multisignature address
*
*
* optional bool isFollowing = 5;
*/
public boolean getIsFollowing() {
return isFollowing_;
}
/**
*
**
* Flag indicating that this key is a root of a following chain. This chain is following the next non-following chain.
* Following/followed chains concept is used for married keychains, where the set of keys combined together to produce
* a single P2SH multisignature address
*
*
* optional bool isFollowing = 5;
*/
public Builder setIsFollowing(boolean value) {
bitField0_ |= 0x00000010;
isFollowing_ = value;
onChanged();
return this;
}
/**
*
**
* Flag indicating that this key is a root of a following chain. This chain is following the next non-following chain.
* Following/followed chains concept is used for married keychains, where the set of keys combined together to produce
* a single P2SH multisignature address
*
*
* optional bool isFollowing = 5;
*/
public Builder clearIsFollowing() {
bitField0_ = (bitField0_ & ~0x00000010);
isFollowing_ = false;
onChanged();
return this;
}
private int sigsRequiredToSpend_ = 1;
/**
*
* Number of signatures required to spend. This field is needed only for married keychains to reconstruct KeyChain
* and represents the N value from N-of-M CHECKMULTISIG script. For regular single keychains it will always be 1.
*
*
* optional uint32 sigsRequiredToSpend = 6 [default = 1];
*/
public boolean hasSigsRequiredToSpend() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
*
* Number of signatures required to spend. This field is needed only for married keychains to reconstruct KeyChain
* and represents the N value from N-of-M CHECKMULTISIG script. For regular single keychains it will always be 1.
*
*
* optional uint32 sigsRequiredToSpend = 6 [default = 1];
*/
public int getSigsRequiredToSpend() {
return sigsRequiredToSpend_;
}
/**
*
* Number of signatures required to spend. This field is needed only for married keychains to reconstruct KeyChain
* and represents the N value from N-of-M CHECKMULTISIG script. For regular single keychains it will always be 1.
*
*
* optional uint32 sigsRequiredToSpend = 6 [default = 1];
*/
public Builder setSigsRequiredToSpend(int value) {
bitField0_ |= 0x00000020;
sigsRequiredToSpend_ = value;
onChanged();
return this;
}
/**
*
* Number of signatures required to spend. This field is needed only for married keychains to reconstruct KeyChain
* and represents the N value from N-of-M CHECKMULTISIG script. For regular single keychains it will always be 1.
*
*
* optional uint32 sigsRequiredToSpend = 6 [default = 1];
*/
public Builder clearSigsRequiredToSpend() {
bitField0_ = (bitField0_ & ~0x00000020);
sigsRequiredToSpend_ = 1;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:wallet.DeterministicKey)
}
// @@protoc_insertion_point(class_scope:wallet.DeterministicKey)
private static final org.bitcoinj.wallet.Protos.DeterministicKey DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.bitcoinj.wallet.Protos.DeterministicKey();
}
public static org.bitcoinj.wallet.Protos.DeterministicKey getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public DeterministicKey parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DeterministicKey(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.DeterministicKey getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface KeyOrBuilder extends
// @@protoc_insertion_point(interface_extends:wallet.Key)
com.google.protobuf.MessageOrBuilder {
/**
* required .wallet.Key.Type type = 1;
*/
boolean hasType();
/**
* required .wallet.Key.Type type = 1;
*/
org.bitcoinj.wallet.Protos.Key.Type getType();
/**
*
* Either the private EC key bytes (without any ASN.1 wrapping), or the deterministic root seed.
* If the secret is encrypted, or this is a "watching entry" then this is missing.
*
*
* optional bytes secret_bytes = 2;
*/
boolean hasSecretBytes();
/**
*
* Either the private EC key bytes (without any ASN.1 wrapping), or the deterministic root seed.
* If the secret is encrypted, or this is a "watching entry" then this is missing.
*
*
* optional bytes secret_bytes = 2;
*/
com.google.protobuf.ByteString getSecretBytes();
/**
*
* If the secret data is encrypted, then secret_bytes is missing and this field is set.
*
*
* optional .wallet.EncryptedData encrypted_data = 6;
*/
boolean hasEncryptedData();
/**
*
* If the secret data is encrypted, then secret_bytes is missing and this field is set.
*
*
* optional .wallet.EncryptedData encrypted_data = 6;
*/
org.bitcoinj.wallet.Protos.EncryptedData getEncryptedData();
/**
*
* If the secret data is encrypted, then secret_bytes is missing and this field is set.
*
*
* optional .wallet.EncryptedData encrypted_data = 6;
*/
org.bitcoinj.wallet.Protos.EncryptedDataOrBuilder getEncryptedDataOrBuilder();
/**
*
* The public EC key derived from the private key. We allow both to be stored to avoid mobile clients having to
* do lots of slow EC math on startup. For DETERMINISTIC_MNEMONIC entries this is missing.
*
*
* optional bytes public_key = 3;
*/
boolean hasPublicKey();
/**
*
* The public EC key derived from the private key. We allow both to be stored to avoid mobile clients having to
* do lots of slow EC math on startup. For DETERMINISTIC_MNEMONIC entries this is missing.
*
*
* optional bytes public_key = 3;
*/
com.google.protobuf.ByteString getPublicKey();
/**
*
* User-provided label associated with the key.
*
*
* optional string label = 4;
*/
boolean hasLabel();
/**
*
* User-provided label associated with the key.
*
*
* optional string label = 4;
*/
java.lang.String getLabel();
/**
*
* User-provided label associated with the key.
*
*
* optional string label = 4;
*/
com.google.protobuf.ByteString
getLabelBytes();
/**
*
* Timestamp stored as millis since epoch. Useful for skipping block bodies before this point. The reason it's
* optional is that keys derived from a parent don't have this data.
*
*
* optional int64 creation_timestamp = 5;
*/
boolean hasCreationTimestamp();
/**
*
* Timestamp stored as millis since epoch. Useful for skipping block bodies before this point. The reason it's
* optional is that keys derived from a parent don't have this data.
*
*
* optional int64 creation_timestamp = 5;
*/
long getCreationTimestamp();
/**
* optional .wallet.DeterministicKey deterministic_key = 7;
*/
boolean hasDeterministicKey();
/**
* optional .wallet.DeterministicKey deterministic_key = 7;
*/
org.bitcoinj.wallet.Protos.DeterministicKey getDeterministicKey();
/**
* optional .wallet.DeterministicKey deterministic_key = 7;
*/
org.bitcoinj.wallet.Protos.DeterministicKeyOrBuilder getDeterministicKeyOrBuilder();
/**
*
* The seed for a deterministic key hierarchy. Derived from the mnemonic,
* but cached here for quick startup. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* optional bytes deterministic_seed = 8;
*/
boolean hasDeterministicSeed();
/**
*
* The seed for a deterministic key hierarchy. Derived from the mnemonic,
* but cached here for quick startup. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* optional bytes deterministic_seed = 8;
*/
com.google.protobuf.ByteString getDeterministicSeed();
/**
*
* Encrypted version of the seed
*
*
* optional .wallet.EncryptedData encrypted_deterministic_seed = 9;
*/
boolean hasEncryptedDeterministicSeed();
/**
*
* Encrypted version of the seed
*
*
* optional .wallet.EncryptedData encrypted_deterministic_seed = 9;
*/
org.bitcoinj.wallet.Protos.EncryptedData getEncryptedDeterministicSeed();
/**
*
* Encrypted version of the seed
*
*
* optional .wallet.EncryptedData encrypted_deterministic_seed = 9;
*/
org.bitcoinj.wallet.Protos.EncryptedDataOrBuilder getEncryptedDeterministicSeedOrBuilder();
/**
*
* The path to the root. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* repeated uint32 account_path = 10 [packed = true];
*/
java.util.List getAccountPathList();
/**
*
* The path to the root. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* repeated uint32 account_path = 10 [packed = true];
*/
int getAccountPathCount();
/**
*
* The path to the root. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* repeated uint32 account_path = 10 [packed = true];
*/
int getAccountPath(int index);
/**
*
* Type of addresses (aka output scripts) to generate for receiving.
*
*
* optional .wallet.Key.OutputScriptType output_script_type = 11;
*/
boolean hasOutputScriptType();
/**
*
* Type of addresses (aka output scripts) to generate for receiving.
*
*
* optional .wallet.Key.OutputScriptType output_script_type = 11;
*/
org.bitcoinj.wallet.Protos.Key.OutputScriptType getOutputScriptType();
}
/**
*
**
* A key used to control Bitcoin spending.
* Either the private key, the public key or both may be present. It is recommended that
* if the private key is provided that the public key is provided too because deriving it is slow.
* If only the public key is provided, the key can only be used to watch the blockchain and verify
* transactions, and not for spending.
*
*
* Protobuf type {@code wallet.Key}
*/
public static final class Key extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:wallet.Key)
KeyOrBuilder {
private static final long serialVersionUID = 0L;
// Use Key.newBuilder() to construct.
private Key(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Key() {
type_ = 1;
secretBytes_ = com.google.protobuf.ByteString.EMPTY;
publicKey_ = com.google.protobuf.ByteString.EMPTY;
label_ = "";
creationTimestamp_ = 0L;
deterministicSeed_ = com.google.protobuf.ByteString.EMPTY;
accountPath_ = java.util.Collections.emptyList();
outputScriptType_ = 1;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Key(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
org.bitcoinj.wallet.Protos.Key.Type value = org.bitcoinj.wallet.Protos.Key.Type.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(1, rawValue);
} else {
bitField0_ |= 0x00000001;
type_ = rawValue;
}
break;
}
case 18: {
bitField0_ |= 0x00000002;
secretBytes_ = input.readBytes();
break;
}
case 26: {
bitField0_ |= 0x00000008;
publicKey_ = input.readBytes();
break;
}
case 34: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000010;
label_ = bs;
break;
}
case 40: {
bitField0_ |= 0x00000020;
creationTimestamp_ = input.readInt64();
break;
}
case 50: {
org.bitcoinj.wallet.Protos.EncryptedData.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
subBuilder = encryptedData_.toBuilder();
}
encryptedData_ = input.readMessage(org.bitcoinj.wallet.Protos.EncryptedData.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(encryptedData_);
encryptedData_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 58: {
org.bitcoinj.wallet.Protos.DeterministicKey.Builder subBuilder = null;
if (((bitField0_ & 0x00000040) == 0x00000040)) {
subBuilder = deterministicKey_.toBuilder();
}
deterministicKey_ = input.readMessage(org.bitcoinj.wallet.Protos.DeterministicKey.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(deterministicKey_);
deterministicKey_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000040;
break;
}
case 66: {
bitField0_ |= 0x00000080;
deterministicSeed_ = input.readBytes();
break;
}
case 74: {
org.bitcoinj.wallet.Protos.EncryptedData.Builder subBuilder = null;
if (((bitField0_ & 0x00000100) == 0x00000100)) {
subBuilder = encryptedDeterministicSeed_.toBuilder();
}
encryptedDeterministicSeed_ = input.readMessage(org.bitcoinj.wallet.Protos.EncryptedData.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(encryptedDeterministicSeed_);
encryptedDeterministicSeed_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000100;
break;
}
case 80: {
if (!((mutable_bitField0_ & 0x00000200) == 0x00000200)) {
accountPath_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000200;
}
accountPath_.add(input.readUInt32());
break;
}
case 82: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000200) == 0x00000200) && input.getBytesUntilLimit() > 0) {
accountPath_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000200;
}
while (input.getBytesUntilLimit() > 0) {
accountPath_.add(input.readUInt32());
}
input.popLimit(limit);
break;
}
case 88: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
org.bitcoinj.wallet.Protos.Key.OutputScriptType value = org.bitcoinj.wallet.Protos.Key.OutputScriptType.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(11, rawValue);
} else {
bitField0_ |= 0x00000200;
outputScriptType_ = rawValue;
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000200) == 0x00000200)) {
accountPath_ = java.util.Collections.unmodifiableList(accountPath_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_Key_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_Key_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoinj.wallet.Protos.Key.class, org.bitcoinj.wallet.Protos.Key.Builder.class);
}
/**
* Protobuf enum {@code wallet.Key.Type}
*/
public enum Type
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
** Unencrypted - Original bitcoin secp256k1 curve
*
*
* ORIGINAL = 1;
*/
ORIGINAL(1),
/**
*
** Encrypted with Scrypt and AES - Original bitcoin secp256k1 curve
*
*
* ENCRYPTED_SCRYPT_AES = 2;
*/
ENCRYPTED_SCRYPT_AES(2),
/**
*
**
* Not really a key, but rather contains the mnemonic phrase for a deterministic key hierarchy in the private_key field.
* The label and public_key fields are missing. Creation timestamp will exist.
*
*
* DETERMINISTIC_MNEMONIC = 3;
*/
DETERMINISTIC_MNEMONIC(3),
/**
*
**
* A key that was derived deterministically. Note that the root seed that created it may NOT be present in the
* wallet, for the case of watching wallets. A deterministic key may or may not have the private key bytes present.
* However the public key bytes and the deterministic_key field are guaranteed to exist. In a wallet where there
* is a path from this key up to a key that has (possibly encrypted) private bytes, it's expected that the private
* key can be rederived on the fly.
*
*
* DETERMINISTIC_KEY = 4;
*/
DETERMINISTIC_KEY(4),
;
/**
*
** Unencrypted - Original bitcoin secp256k1 curve
*
*
* ORIGINAL = 1;
*/
public static final int ORIGINAL_VALUE = 1;
/**
*
** Encrypted with Scrypt and AES - Original bitcoin secp256k1 curve
*
*
* ENCRYPTED_SCRYPT_AES = 2;
*/
public static final int ENCRYPTED_SCRYPT_AES_VALUE = 2;
/**
*
**
* Not really a key, but rather contains the mnemonic phrase for a deterministic key hierarchy in the private_key field.
* The label and public_key fields are missing. Creation timestamp will exist.
*
*
* DETERMINISTIC_MNEMONIC = 3;
*/
public static final int DETERMINISTIC_MNEMONIC_VALUE = 3;
/**
*
**
* A key that was derived deterministically. Note that the root seed that created it may NOT be present in the
* wallet, for the case of watching wallets. A deterministic key may or may not have the private key bytes present.
* However the public key bytes and the deterministic_key field are guaranteed to exist. In a wallet where there
* is a path from this key up to a key that has (possibly encrypted) private bytes, it's expected that the private
* key can be rederived on the fly.
*
*
* DETERMINISTIC_KEY = 4;
*/
public static final int DETERMINISTIC_KEY_VALUE = 4;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Type valueOf(int value) {
return forNumber(value);
}
public static Type forNumber(int value) {
switch (value) {
case 1: return ORIGINAL;
case 2: return ENCRYPTED_SCRYPT_AES;
case 3: return DETERMINISTIC_MNEMONIC;
case 4: return DETERMINISTIC_KEY;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Type> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Type findValueByNumber(int number) {
return Type.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.bitcoinj.wallet.Protos.Key.getDescriptor().getEnumTypes().get(0);
}
private static final Type[] VALUES = values();
public static Type valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private Type(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:wallet.Key.Type)
}
/**
* Protobuf enum {@code wallet.Key.OutputScriptType}
*/
public enum OutputScriptType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* P2PKH = 1;
*/
P2PKH(1),
/**
* P2WPKH = 2;
*/
P2WPKH(2),
;
/**
* P2PKH = 1;
*/
public static final int P2PKH_VALUE = 1;
/**
* P2WPKH = 2;
*/
public static final int P2WPKH_VALUE = 2;
public final int getNumber() {
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static OutputScriptType valueOf(int value) {
return forNumber(value);
}
public static OutputScriptType forNumber(int value) {
switch (value) {
case 1: return P2PKH;
case 2: return P2WPKH;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
OutputScriptType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public OutputScriptType findValueByNumber(int number) {
return OutputScriptType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.bitcoinj.wallet.Protos.Key.getDescriptor().getEnumTypes().get(1);
}
private static final OutputScriptType[] VALUES = values();
public static OutputScriptType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private OutputScriptType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:wallet.Key.OutputScriptType)
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private int type_;
/**
* required .wallet.Key.Type type = 1;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .wallet.Key.Type type = 1;
*/
public org.bitcoinj.wallet.Protos.Key.Type getType() {
@SuppressWarnings("deprecation")
org.bitcoinj.wallet.Protos.Key.Type result = org.bitcoinj.wallet.Protos.Key.Type.valueOf(type_);
return result == null ? org.bitcoinj.wallet.Protos.Key.Type.ORIGINAL : result;
}
public static final int SECRET_BYTES_FIELD_NUMBER = 2;
private com.google.protobuf.ByteString secretBytes_;
/**
*
* Either the private EC key bytes (without any ASN.1 wrapping), or the deterministic root seed.
* If the secret is encrypted, or this is a "watching entry" then this is missing.
*
*
* optional bytes secret_bytes = 2;
*/
public boolean hasSecretBytes() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Either the private EC key bytes (without any ASN.1 wrapping), or the deterministic root seed.
* If the secret is encrypted, or this is a "watching entry" then this is missing.
*
*
* optional bytes secret_bytes = 2;
*/
public com.google.protobuf.ByteString getSecretBytes() {
return secretBytes_;
}
public static final int ENCRYPTED_DATA_FIELD_NUMBER = 6;
private org.bitcoinj.wallet.Protos.EncryptedData encryptedData_;
/**
*
* If the secret data is encrypted, then secret_bytes is missing and this field is set.
*
*
* optional .wallet.EncryptedData encrypted_data = 6;
*/
public boolean hasEncryptedData() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* If the secret data is encrypted, then secret_bytes is missing and this field is set.
*
*
* optional .wallet.EncryptedData encrypted_data = 6;
*/
public org.bitcoinj.wallet.Protos.EncryptedData getEncryptedData() {
return encryptedData_ == null ? org.bitcoinj.wallet.Protos.EncryptedData.getDefaultInstance() : encryptedData_;
}
/**
*
* If the secret data is encrypted, then secret_bytes is missing and this field is set.
*
*
* optional .wallet.EncryptedData encrypted_data = 6;
*/
public org.bitcoinj.wallet.Protos.EncryptedDataOrBuilder getEncryptedDataOrBuilder() {
return encryptedData_ == null ? org.bitcoinj.wallet.Protos.EncryptedData.getDefaultInstance() : encryptedData_;
}
public static final int PUBLIC_KEY_FIELD_NUMBER = 3;
private com.google.protobuf.ByteString publicKey_;
/**
*
* The public EC key derived from the private key. We allow both to be stored to avoid mobile clients having to
* do lots of slow EC math on startup. For DETERMINISTIC_MNEMONIC entries this is missing.
*
*
* optional bytes public_key = 3;
*/
public boolean hasPublicKey() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* The public EC key derived from the private key. We allow both to be stored to avoid mobile clients having to
* do lots of slow EC math on startup. For DETERMINISTIC_MNEMONIC entries this is missing.
*
*
* optional bytes public_key = 3;
*/
public com.google.protobuf.ByteString getPublicKey() {
return publicKey_;
}
public static final int LABEL_FIELD_NUMBER = 4;
private volatile java.lang.Object label_;
/**
*
* User-provided label associated with the key.
*
*
* optional string label = 4;
*/
public boolean hasLabel() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* User-provided label associated with the key.
*
*
* optional string label = 4;
*/
public java.lang.String getLabel() {
java.lang.Object ref = label_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
label_ = s;
}
return s;
}
}
/**
*
* User-provided label associated with the key.
*
*
* optional string label = 4;
*/
public com.google.protobuf.ByteString
getLabelBytes() {
java.lang.Object ref = label_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
label_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CREATION_TIMESTAMP_FIELD_NUMBER = 5;
private long creationTimestamp_;
/**
*
* Timestamp stored as millis since epoch. Useful for skipping block bodies before this point. The reason it's
* optional is that keys derived from a parent don't have this data.
*
*
* optional int64 creation_timestamp = 5;
*/
public boolean hasCreationTimestamp() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
*
* Timestamp stored as millis since epoch. Useful for skipping block bodies before this point. The reason it's
* optional is that keys derived from a parent don't have this data.
*
*
* optional int64 creation_timestamp = 5;
*/
public long getCreationTimestamp() {
return creationTimestamp_;
}
public static final int DETERMINISTIC_KEY_FIELD_NUMBER = 7;
private org.bitcoinj.wallet.Protos.DeterministicKey deterministicKey_;
/**
* optional .wallet.DeterministicKey deterministic_key = 7;
*/
public boolean hasDeterministicKey() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .wallet.DeterministicKey deterministic_key = 7;
*/
public org.bitcoinj.wallet.Protos.DeterministicKey getDeterministicKey() {
return deterministicKey_ == null ? org.bitcoinj.wallet.Protos.DeterministicKey.getDefaultInstance() : deterministicKey_;
}
/**
* optional .wallet.DeterministicKey deterministic_key = 7;
*/
public org.bitcoinj.wallet.Protos.DeterministicKeyOrBuilder getDeterministicKeyOrBuilder() {
return deterministicKey_ == null ? org.bitcoinj.wallet.Protos.DeterministicKey.getDefaultInstance() : deterministicKey_;
}
public static final int DETERMINISTIC_SEED_FIELD_NUMBER = 8;
private com.google.protobuf.ByteString deterministicSeed_;
/**
*
* The seed for a deterministic key hierarchy. Derived from the mnemonic,
* but cached here for quick startup. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* optional bytes deterministic_seed = 8;
*/
public boolean hasDeterministicSeed() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
*
* The seed for a deterministic key hierarchy. Derived from the mnemonic,
* but cached here for quick startup. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* optional bytes deterministic_seed = 8;
*/
public com.google.protobuf.ByteString getDeterministicSeed() {
return deterministicSeed_;
}
public static final int ENCRYPTED_DETERMINISTIC_SEED_FIELD_NUMBER = 9;
private org.bitcoinj.wallet.Protos.EncryptedData encryptedDeterministicSeed_;
/**
*
* Encrypted version of the seed
*
*
* optional .wallet.EncryptedData encrypted_deterministic_seed = 9;
*/
public boolean hasEncryptedDeterministicSeed() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
*
* Encrypted version of the seed
*
*
* optional .wallet.EncryptedData encrypted_deterministic_seed = 9;
*/
public org.bitcoinj.wallet.Protos.EncryptedData getEncryptedDeterministicSeed() {
return encryptedDeterministicSeed_ == null ? org.bitcoinj.wallet.Protos.EncryptedData.getDefaultInstance() : encryptedDeterministicSeed_;
}
/**
*
* Encrypted version of the seed
*
*
* optional .wallet.EncryptedData encrypted_deterministic_seed = 9;
*/
public org.bitcoinj.wallet.Protos.EncryptedDataOrBuilder getEncryptedDeterministicSeedOrBuilder() {
return encryptedDeterministicSeed_ == null ? org.bitcoinj.wallet.Protos.EncryptedData.getDefaultInstance() : encryptedDeterministicSeed_;
}
public static final int ACCOUNT_PATH_FIELD_NUMBER = 10;
private java.util.List accountPath_;
/**
*
* The path to the root. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* repeated uint32 account_path = 10 [packed = true];
*/
public java.util.List
getAccountPathList() {
return accountPath_;
}
/**
*
* The path to the root. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* repeated uint32 account_path = 10 [packed = true];
*/
public int getAccountPathCount() {
return accountPath_.size();
}
/**
*
* The path to the root. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* repeated uint32 account_path = 10 [packed = true];
*/
public int getAccountPath(int index) {
return accountPath_.get(index);
}
private int accountPathMemoizedSerializedSize = -1;
public static final int OUTPUT_SCRIPT_TYPE_FIELD_NUMBER = 11;
private int outputScriptType_;
/**
*
* Type of addresses (aka output scripts) to generate for receiving.
*
*
* optional .wallet.Key.OutputScriptType output_script_type = 11;
*/
public boolean hasOutputScriptType() {
return ((bitField0_ & 0x00000200) == 0x00000200);
}
/**
*
* Type of addresses (aka output scripts) to generate for receiving.
*
*
* optional .wallet.Key.OutputScriptType output_script_type = 11;
*/
public org.bitcoinj.wallet.Protos.Key.OutputScriptType getOutputScriptType() {
@SuppressWarnings("deprecation")
org.bitcoinj.wallet.Protos.Key.OutputScriptType result = org.bitcoinj.wallet.Protos.Key.OutputScriptType.valueOf(outputScriptType_);
return result == null ? org.bitcoinj.wallet.Protos.Key.OutputScriptType.P2PKH : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasType()) {
memoizedIsInitialized = 0;
return false;
}
if (hasEncryptedData()) {
if (!getEncryptedData().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasDeterministicKey()) {
if (!getDeterministicKey().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
if (hasEncryptedDeterministicSeed()) {
if (!getEncryptedDeterministicSeed().isInitialized()) {
memoizedIsInitialized = 0;
return false;
}
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeEnum(1, type_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeBytes(2, secretBytes_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
output.writeBytes(3, publicKey_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, label_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
output.writeInt64(5, creationTimestamp_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
output.writeMessage(6, getEncryptedData());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
output.writeMessage(7, getDeterministicKey());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
output.writeBytes(8, deterministicSeed_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
output.writeMessage(9, getEncryptedDeterministicSeed());
}
if (getAccountPathList().size() > 0) {
output.writeUInt32NoTag(82);
output.writeUInt32NoTag(accountPathMemoizedSerializedSize);
}
for (int i = 0; i < accountPath_.size(); i++) {
output.writeUInt32NoTag(accountPath_.get(i));
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
output.writeEnum(11, outputScriptType_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(2, secretBytes_);
}
if (((bitField0_ & 0x00000008) == 0x00000008)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(3, publicKey_);
}
if (((bitField0_ & 0x00000010) == 0x00000010)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, label_);
}
if (((bitField0_ & 0x00000020) == 0x00000020)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, creationTimestamp_);
}
if (((bitField0_ & 0x00000004) == 0x00000004)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getEncryptedData());
}
if (((bitField0_ & 0x00000040) == 0x00000040)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, getDeterministicKey());
}
if (((bitField0_ & 0x00000080) == 0x00000080)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(8, deterministicSeed_);
}
if (((bitField0_ & 0x00000100) == 0x00000100)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getEncryptedDeterministicSeed());
}
{
int dataSize = 0;
for (int i = 0; i < accountPath_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(accountPath_.get(i));
}
size += dataSize;
if (!getAccountPathList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
accountPathMemoizedSerializedSize = dataSize;
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(11, outputScriptType_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.bitcoinj.wallet.Protos.Key)) {
return super.equals(obj);
}
org.bitcoinj.wallet.Protos.Key other = (org.bitcoinj.wallet.Protos.Key) obj;
boolean result = true;
result = result && (hasType() == other.hasType());
if (hasType()) {
result = result && type_ == other.type_;
}
result = result && (hasSecretBytes() == other.hasSecretBytes());
if (hasSecretBytes()) {
result = result && getSecretBytes()
.equals(other.getSecretBytes());
}
result = result && (hasEncryptedData() == other.hasEncryptedData());
if (hasEncryptedData()) {
result = result && getEncryptedData()
.equals(other.getEncryptedData());
}
result = result && (hasPublicKey() == other.hasPublicKey());
if (hasPublicKey()) {
result = result && getPublicKey()
.equals(other.getPublicKey());
}
result = result && (hasLabel() == other.hasLabel());
if (hasLabel()) {
result = result && getLabel()
.equals(other.getLabel());
}
result = result && (hasCreationTimestamp() == other.hasCreationTimestamp());
if (hasCreationTimestamp()) {
result = result && (getCreationTimestamp()
== other.getCreationTimestamp());
}
result = result && (hasDeterministicKey() == other.hasDeterministicKey());
if (hasDeterministicKey()) {
result = result && getDeterministicKey()
.equals(other.getDeterministicKey());
}
result = result && (hasDeterministicSeed() == other.hasDeterministicSeed());
if (hasDeterministicSeed()) {
result = result && getDeterministicSeed()
.equals(other.getDeterministicSeed());
}
result = result && (hasEncryptedDeterministicSeed() == other.hasEncryptedDeterministicSeed());
if (hasEncryptedDeterministicSeed()) {
result = result && getEncryptedDeterministicSeed()
.equals(other.getEncryptedDeterministicSeed());
}
result = result && getAccountPathList()
.equals(other.getAccountPathList());
result = result && (hasOutputScriptType() == other.hasOutputScriptType());
if (hasOutputScriptType()) {
result = result && outputScriptType_ == other.outputScriptType_;
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasType()) {
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
}
if (hasSecretBytes()) {
hash = (37 * hash) + SECRET_BYTES_FIELD_NUMBER;
hash = (53 * hash) + getSecretBytes().hashCode();
}
if (hasEncryptedData()) {
hash = (37 * hash) + ENCRYPTED_DATA_FIELD_NUMBER;
hash = (53 * hash) + getEncryptedData().hashCode();
}
if (hasPublicKey()) {
hash = (37 * hash) + PUBLIC_KEY_FIELD_NUMBER;
hash = (53 * hash) + getPublicKey().hashCode();
}
if (hasLabel()) {
hash = (37 * hash) + LABEL_FIELD_NUMBER;
hash = (53 * hash) + getLabel().hashCode();
}
if (hasCreationTimestamp()) {
hash = (37 * hash) + CREATION_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCreationTimestamp());
}
if (hasDeterministicKey()) {
hash = (37 * hash) + DETERMINISTIC_KEY_FIELD_NUMBER;
hash = (53 * hash) + getDeterministicKey().hashCode();
}
if (hasDeterministicSeed()) {
hash = (37 * hash) + DETERMINISTIC_SEED_FIELD_NUMBER;
hash = (53 * hash) + getDeterministicSeed().hashCode();
}
if (hasEncryptedDeterministicSeed()) {
hash = (37 * hash) + ENCRYPTED_DETERMINISTIC_SEED_FIELD_NUMBER;
hash = (53 * hash) + getEncryptedDeterministicSeed().hashCode();
}
if (getAccountPathCount() > 0) {
hash = (37 * hash) + ACCOUNT_PATH_FIELD_NUMBER;
hash = (53 * hash) + getAccountPathList().hashCode();
}
if (hasOutputScriptType()) {
hash = (37 * hash) + OUTPUT_SCRIPT_TYPE_FIELD_NUMBER;
hash = (53 * hash) + outputScriptType_;
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.bitcoinj.wallet.Protos.Key parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoinj.wallet.Protos.Key parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.Key parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoinj.wallet.Protos.Key parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.Key parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoinj.wallet.Protos.Key parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.Key parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.bitcoinj.wallet.Protos.Key parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.Key parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.bitcoinj.wallet.Protos.Key parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.Key parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.bitcoinj.wallet.Protos.Key parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.bitcoinj.wallet.Protos.Key prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
**
* A key used to control Bitcoin spending.
* Either the private key, the public key or both may be present. It is recommended that
* if the private key is provided that the public key is provided too because deriving it is slow.
* If only the public key is provided, the key can only be used to watch the blockchain and verify
* transactions, and not for spending.
*
*
* Protobuf type {@code wallet.Key}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:wallet.Key)
org.bitcoinj.wallet.Protos.KeyOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_Key_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_Key_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoinj.wallet.Protos.Key.class, org.bitcoinj.wallet.Protos.Key.Builder.class);
}
// Construct using org.bitcoinj.wallet.Protos.Key.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getEncryptedDataFieldBuilder();
getDeterministicKeyFieldBuilder();
getEncryptedDeterministicSeedFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
type_ = 1;
bitField0_ = (bitField0_ & ~0x00000001);
secretBytes_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
if (encryptedDataBuilder_ == null) {
encryptedData_ = null;
} else {
encryptedDataBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
publicKey_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000008);
label_ = "";
bitField0_ = (bitField0_ & ~0x00000010);
creationTimestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000020);
if (deterministicKeyBuilder_ == null) {
deterministicKey_ = null;
} else {
deterministicKeyBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
deterministicSeed_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000080);
if (encryptedDeterministicSeedBuilder_ == null) {
encryptedDeterministicSeed_ = null;
} else {
encryptedDeterministicSeedBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
accountPath_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
outputScriptType_ = 1;
bitField0_ = (bitField0_ & ~0x00000400);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_Key_descriptor;
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.Key getDefaultInstanceForType() {
return org.bitcoinj.wallet.Protos.Key.getDefaultInstance();
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.Key build() {
org.bitcoinj.wallet.Protos.Key result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.Key buildPartial() {
org.bitcoinj.wallet.Protos.Key result = new org.bitcoinj.wallet.Protos.Key(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.type_ = type_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.secretBytes_ = secretBytes_;
if (((from_bitField0_ & 0x00000004) == 0x00000004)) {
to_bitField0_ |= 0x00000004;
}
if (encryptedDataBuilder_ == null) {
result.encryptedData_ = encryptedData_;
} else {
result.encryptedData_ = encryptedDataBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) == 0x00000008)) {
to_bitField0_ |= 0x00000008;
}
result.publicKey_ = publicKey_;
if (((from_bitField0_ & 0x00000010) == 0x00000010)) {
to_bitField0_ |= 0x00000010;
}
result.label_ = label_;
if (((from_bitField0_ & 0x00000020) == 0x00000020)) {
to_bitField0_ |= 0x00000020;
}
result.creationTimestamp_ = creationTimestamp_;
if (((from_bitField0_ & 0x00000040) == 0x00000040)) {
to_bitField0_ |= 0x00000040;
}
if (deterministicKeyBuilder_ == null) {
result.deterministicKey_ = deterministicKey_;
} else {
result.deterministicKey_ = deterministicKeyBuilder_.build();
}
if (((from_bitField0_ & 0x00000080) == 0x00000080)) {
to_bitField0_ |= 0x00000080;
}
result.deterministicSeed_ = deterministicSeed_;
if (((from_bitField0_ & 0x00000100) == 0x00000100)) {
to_bitField0_ |= 0x00000100;
}
if (encryptedDeterministicSeedBuilder_ == null) {
result.encryptedDeterministicSeed_ = encryptedDeterministicSeed_;
} else {
result.encryptedDeterministicSeed_ = encryptedDeterministicSeedBuilder_.build();
}
if (((bitField0_ & 0x00000200) == 0x00000200)) {
accountPath_ = java.util.Collections.unmodifiableList(accountPath_);
bitField0_ = (bitField0_ & ~0x00000200);
}
result.accountPath_ = accountPath_;
if (((from_bitField0_ & 0x00000400) == 0x00000400)) {
to_bitField0_ |= 0x00000200;
}
result.outputScriptType_ = outputScriptType_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.bitcoinj.wallet.Protos.Key) {
return mergeFrom((org.bitcoinj.wallet.Protos.Key)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.bitcoinj.wallet.Protos.Key other) {
if (other == org.bitcoinj.wallet.Protos.Key.getDefaultInstance()) return this;
if (other.hasType()) {
setType(other.getType());
}
if (other.hasSecretBytes()) {
setSecretBytes(other.getSecretBytes());
}
if (other.hasEncryptedData()) {
mergeEncryptedData(other.getEncryptedData());
}
if (other.hasPublicKey()) {
setPublicKey(other.getPublicKey());
}
if (other.hasLabel()) {
bitField0_ |= 0x00000010;
label_ = other.label_;
onChanged();
}
if (other.hasCreationTimestamp()) {
setCreationTimestamp(other.getCreationTimestamp());
}
if (other.hasDeterministicKey()) {
mergeDeterministicKey(other.getDeterministicKey());
}
if (other.hasDeterministicSeed()) {
setDeterministicSeed(other.getDeterministicSeed());
}
if (other.hasEncryptedDeterministicSeed()) {
mergeEncryptedDeterministicSeed(other.getEncryptedDeterministicSeed());
}
if (!other.accountPath_.isEmpty()) {
if (accountPath_.isEmpty()) {
accountPath_ = other.accountPath_;
bitField0_ = (bitField0_ & ~0x00000200);
} else {
ensureAccountPathIsMutable();
accountPath_.addAll(other.accountPath_);
}
onChanged();
}
if (other.hasOutputScriptType()) {
setOutputScriptType(other.getOutputScriptType());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasType()) {
return false;
}
if (hasEncryptedData()) {
if (!getEncryptedData().isInitialized()) {
return false;
}
}
if (hasDeterministicKey()) {
if (!getDeterministicKey().isInitialized()) {
return false;
}
}
if (hasEncryptedDeterministicSeed()) {
if (!getEncryptedDeterministicSeed().isInitialized()) {
return false;
}
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.bitcoinj.wallet.Protos.Key parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.bitcoinj.wallet.Protos.Key) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int type_ = 1;
/**
* required .wallet.Key.Type type = 1;
*/
public boolean hasType() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required .wallet.Key.Type type = 1;
*/
public org.bitcoinj.wallet.Protos.Key.Type getType() {
@SuppressWarnings("deprecation")
org.bitcoinj.wallet.Protos.Key.Type result = org.bitcoinj.wallet.Protos.Key.Type.valueOf(type_);
return result == null ? org.bitcoinj.wallet.Protos.Key.Type.ORIGINAL : result;
}
/**
* required .wallet.Key.Type type = 1;
*/
public Builder setType(org.bitcoinj.wallet.Protos.Key.Type value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value.getNumber();
onChanged();
return this;
}
/**
* required .wallet.Key.Type type = 1;
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = 1;
onChanged();
return this;
}
private com.google.protobuf.ByteString secretBytes_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Either the private EC key bytes (without any ASN.1 wrapping), or the deterministic root seed.
* If the secret is encrypted, or this is a "watching entry" then this is missing.
*
*
* optional bytes secret_bytes = 2;
*/
public boolean hasSecretBytes() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Either the private EC key bytes (without any ASN.1 wrapping), or the deterministic root seed.
* If the secret is encrypted, or this is a "watching entry" then this is missing.
*
*
* optional bytes secret_bytes = 2;
*/
public com.google.protobuf.ByteString getSecretBytes() {
return secretBytes_;
}
/**
*
* Either the private EC key bytes (without any ASN.1 wrapping), or the deterministic root seed.
* If the secret is encrypted, or this is a "watching entry" then this is missing.
*
*
* optional bytes secret_bytes = 2;
*/
public Builder setSecretBytes(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
secretBytes_ = value;
onChanged();
return this;
}
/**
*
* Either the private EC key bytes (without any ASN.1 wrapping), or the deterministic root seed.
* If the secret is encrypted, or this is a "watching entry" then this is missing.
*
*
* optional bytes secret_bytes = 2;
*/
public Builder clearSecretBytes() {
bitField0_ = (bitField0_ & ~0x00000002);
secretBytes_ = getDefaultInstance().getSecretBytes();
onChanged();
return this;
}
private org.bitcoinj.wallet.Protos.EncryptedData encryptedData_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.bitcoinj.wallet.Protos.EncryptedData, org.bitcoinj.wallet.Protos.EncryptedData.Builder, org.bitcoinj.wallet.Protos.EncryptedDataOrBuilder> encryptedDataBuilder_;
/**
*
* If the secret data is encrypted, then secret_bytes is missing and this field is set.
*
*
* optional .wallet.EncryptedData encrypted_data = 6;
*/
public boolean hasEncryptedData() {
return ((bitField0_ & 0x00000004) == 0x00000004);
}
/**
*
* If the secret data is encrypted, then secret_bytes is missing and this field is set.
*
*
* optional .wallet.EncryptedData encrypted_data = 6;
*/
public org.bitcoinj.wallet.Protos.EncryptedData getEncryptedData() {
if (encryptedDataBuilder_ == null) {
return encryptedData_ == null ? org.bitcoinj.wallet.Protos.EncryptedData.getDefaultInstance() : encryptedData_;
} else {
return encryptedDataBuilder_.getMessage();
}
}
/**
*
* If the secret data is encrypted, then secret_bytes is missing and this field is set.
*
*
* optional .wallet.EncryptedData encrypted_data = 6;
*/
public Builder setEncryptedData(org.bitcoinj.wallet.Protos.EncryptedData value) {
if (encryptedDataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
encryptedData_ = value;
onChanged();
} else {
encryptedDataBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* If the secret data is encrypted, then secret_bytes is missing and this field is set.
*
*
* optional .wallet.EncryptedData encrypted_data = 6;
*/
public Builder setEncryptedData(
org.bitcoinj.wallet.Protos.EncryptedData.Builder builderForValue) {
if (encryptedDataBuilder_ == null) {
encryptedData_ = builderForValue.build();
onChanged();
} else {
encryptedDataBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* If the secret data is encrypted, then secret_bytes is missing and this field is set.
*
*
* optional .wallet.EncryptedData encrypted_data = 6;
*/
public Builder mergeEncryptedData(org.bitcoinj.wallet.Protos.EncryptedData value) {
if (encryptedDataBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004) &&
encryptedData_ != null &&
encryptedData_ != org.bitcoinj.wallet.Protos.EncryptedData.getDefaultInstance()) {
encryptedData_ =
org.bitcoinj.wallet.Protos.EncryptedData.newBuilder(encryptedData_).mergeFrom(value).buildPartial();
} else {
encryptedData_ = value;
}
onChanged();
} else {
encryptedDataBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* If the secret data is encrypted, then secret_bytes is missing and this field is set.
*
*
* optional .wallet.EncryptedData encrypted_data = 6;
*/
public Builder clearEncryptedData() {
if (encryptedDataBuilder_ == null) {
encryptedData_ = null;
onChanged();
} else {
encryptedDataBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
*
* If the secret data is encrypted, then secret_bytes is missing and this field is set.
*
*
* optional .wallet.EncryptedData encrypted_data = 6;
*/
public org.bitcoinj.wallet.Protos.EncryptedData.Builder getEncryptedDataBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getEncryptedDataFieldBuilder().getBuilder();
}
/**
*
* If the secret data is encrypted, then secret_bytes is missing and this field is set.
*
*
* optional .wallet.EncryptedData encrypted_data = 6;
*/
public org.bitcoinj.wallet.Protos.EncryptedDataOrBuilder getEncryptedDataOrBuilder() {
if (encryptedDataBuilder_ != null) {
return encryptedDataBuilder_.getMessageOrBuilder();
} else {
return encryptedData_ == null ?
org.bitcoinj.wallet.Protos.EncryptedData.getDefaultInstance() : encryptedData_;
}
}
/**
*
* If the secret data is encrypted, then secret_bytes is missing and this field is set.
*
*
* optional .wallet.EncryptedData encrypted_data = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.bitcoinj.wallet.Protos.EncryptedData, org.bitcoinj.wallet.Protos.EncryptedData.Builder, org.bitcoinj.wallet.Protos.EncryptedDataOrBuilder>
getEncryptedDataFieldBuilder() {
if (encryptedDataBuilder_ == null) {
encryptedDataBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.bitcoinj.wallet.Protos.EncryptedData, org.bitcoinj.wallet.Protos.EncryptedData.Builder, org.bitcoinj.wallet.Protos.EncryptedDataOrBuilder>(
getEncryptedData(),
getParentForChildren(),
isClean());
encryptedData_ = null;
}
return encryptedDataBuilder_;
}
private com.google.protobuf.ByteString publicKey_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The public EC key derived from the private key. We allow both to be stored to avoid mobile clients having to
* do lots of slow EC math on startup. For DETERMINISTIC_MNEMONIC entries this is missing.
*
*
* optional bytes public_key = 3;
*/
public boolean hasPublicKey() {
return ((bitField0_ & 0x00000008) == 0x00000008);
}
/**
*
* The public EC key derived from the private key. We allow both to be stored to avoid mobile clients having to
* do lots of slow EC math on startup. For DETERMINISTIC_MNEMONIC entries this is missing.
*
*
* optional bytes public_key = 3;
*/
public com.google.protobuf.ByteString getPublicKey() {
return publicKey_;
}
/**
*
* The public EC key derived from the private key. We allow both to be stored to avoid mobile clients having to
* do lots of slow EC math on startup. For DETERMINISTIC_MNEMONIC entries this is missing.
*
*
* optional bytes public_key = 3;
*/
public Builder setPublicKey(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
publicKey_ = value;
onChanged();
return this;
}
/**
*
* The public EC key derived from the private key. We allow both to be stored to avoid mobile clients having to
* do lots of slow EC math on startup. For DETERMINISTIC_MNEMONIC entries this is missing.
*
*
* optional bytes public_key = 3;
*/
public Builder clearPublicKey() {
bitField0_ = (bitField0_ & ~0x00000008);
publicKey_ = getDefaultInstance().getPublicKey();
onChanged();
return this;
}
private java.lang.Object label_ = "";
/**
*
* User-provided label associated with the key.
*
*
* optional string label = 4;
*/
public boolean hasLabel() {
return ((bitField0_ & 0x00000010) == 0x00000010);
}
/**
*
* User-provided label associated with the key.
*
*
* optional string label = 4;
*/
public java.lang.String getLabel() {
java.lang.Object ref = label_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
label_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* User-provided label associated with the key.
*
*
* optional string label = 4;
*/
public com.google.protobuf.ByteString
getLabelBytes() {
java.lang.Object ref = label_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
label_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* User-provided label associated with the key.
*
*
* optional string label = 4;
*/
public Builder setLabel(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
label_ = value;
onChanged();
return this;
}
/**
*
* User-provided label associated with the key.
*
*
* optional string label = 4;
*/
public Builder clearLabel() {
bitField0_ = (bitField0_ & ~0x00000010);
label_ = getDefaultInstance().getLabel();
onChanged();
return this;
}
/**
*
* User-provided label associated with the key.
*
*
* optional string label = 4;
*/
public Builder setLabelBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
label_ = value;
onChanged();
return this;
}
private long creationTimestamp_ ;
/**
*
* Timestamp stored as millis since epoch. Useful for skipping block bodies before this point. The reason it's
* optional is that keys derived from a parent don't have this data.
*
*
* optional int64 creation_timestamp = 5;
*/
public boolean hasCreationTimestamp() {
return ((bitField0_ & 0x00000020) == 0x00000020);
}
/**
*
* Timestamp stored as millis since epoch. Useful for skipping block bodies before this point. The reason it's
* optional is that keys derived from a parent don't have this data.
*
*
* optional int64 creation_timestamp = 5;
*/
public long getCreationTimestamp() {
return creationTimestamp_;
}
/**
*
* Timestamp stored as millis since epoch. Useful for skipping block bodies before this point. The reason it's
* optional is that keys derived from a parent don't have this data.
*
*
* optional int64 creation_timestamp = 5;
*/
public Builder setCreationTimestamp(long value) {
bitField0_ |= 0x00000020;
creationTimestamp_ = value;
onChanged();
return this;
}
/**
*
* Timestamp stored as millis since epoch. Useful for skipping block bodies before this point. The reason it's
* optional is that keys derived from a parent don't have this data.
*
*
* optional int64 creation_timestamp = 5;
*/
public Builder clearCreationTimestamp() {
bitField0_ = (bitField0_ & ~0x00000020);
creationTimestamp_ = 0L;
onChanged();
return this;
}
private org.bitcoinj.wallet.Protos.DeterministicKey deterministicKey_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.bitcoinj.wallet.Protos.DeterministicKey, org.bitcoinj.wallet.Protos.DeterministicKey.Builder, org.bitcoinj.wallet.Protos.DeterministicKeyOrBuilder> deterministicKeyBuilder_;
/**
* optional .wallet.DeterministicKey deterministic_key = 7;
*/
public boolean hasDeterministicKey() {
return ((bitField0_ & 0x00000040) == 0x00000040);
}
/**
* optional .wallet.DeterministicKey deterministic_key = 7;
*/
public org.bitcoinj.wallet.Protos.DeterministicKey getDeterministicKey() {
if (deterministicKeyBuilder_ == null) {
return deterministicKey_ == null ? org.bitcoinj.wallet.Protos.DeterministicKey.getDefaultInstance() : deterministicKey_;
} else {
return deterministicKeyBuilder_.getMessage();
}
}
/**
* optional .wallet.DeterministicKey deterministic_key = 7;
*/
public Builder setDeterministicKey(org.bitcoinj.wallet.Protos.DeterministicKey value) {
if (deterministicKeyBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
deterministicKey_ = value;
onChanged();
} else {
deterministicKeyBuilder_.setMessage(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .wallet.DeterministicKey deterministic_key = 7;
*/
public Builder setDeterministicKey(
org.bitcoinj.wallet.Protos.DeterministicKey.Builder builderForValue) {
if (deterministicKeyBuilder_ == null) {
deterministicKey_ = builderForValue.build();
onChanged();
} else {
deterministicKeyBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .wallet.DeterministicKey deterministic_key = 7;
*/
public Builder mergeDeterministicKey(org.bitcoinj.wallet.Protos.DeterministicKey value) {
if (deterministicKeyBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040) &&
deterministicKey_ != null &&
deterministicKey_ != org.bitcoinj.wallet.Protos.DeterministicKey.getDefaultInstance()) {
deterministicKey_ =
org.bitcoinj.wallet.Protos.DeterministicKey.newBuilder(deterministicKey_).mergeFrom(value).buildPartial();
} else {
deterministicKey_ = value;
}
onChanged();
} else {
deterministicKeyBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000040;
return this;
}
/**
* optional .wallet.DeterministicKey deterministic_key = 7;
*/
public Builder clearDeterministicKey() {
if (deterministicKeyBuilder_ == null) {
deterministicKey_ = null;
onChanged();
} else {
deterministicKeyBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000040);
return this;
}
/**
* optional .wallet.DeterministicKey deterministic_key = 7;
*/
public org.bitcoinj.wallet.Protos.DeterministicKey.Builder getDeterministicKeyBuilder() {
bitField0_ |= 0x00000040;
onChanged();
return getDeterministicKeyFieldBuilder().getBuilder();
}
/**
* optional .wallet.DeterministicKey deterministic_key = 7;
*/
public org.bitcoinj.wallet.Protos.DeterministicKeyOrBuilder getDeterministicKeyOrBuilder() {
if (deterministicKeyBuilder_ != null) {
return deterministicKeyBuilder_.getMessageOrBuilder();
} else {
return deterministicKey_ == null ?
org.bitcoinj.wallet.Protos.DeterministicKey.getDefaultInstance() : deterministicKey_;
}
}
/**
* optional .wallet.DeterministicKey deterministic_key = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.bitcoinj.wallet.Protos.DeterministicKey, org.bitcoinj.wallet.Protos.DeterministicKey.Builder, org.bitcoinj.wallet.Protos.DeterministicKeyOrBuilder>
getDeterministicKeyFieldBuilder() {
if (deterministicKeyBuilder_ == null) {
deterministicKeyBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.bitcoinj.wallet.Protos.DeterministicKey, org.bitcoinj.wallet.Protos.DeterministicKey.Builder, org.bitcoinj.wallet.Protos.DeterministicKeyOrBuilder>(
getDeterministicKey(),
getParentForChildren(),
isClean());
deterministicKey_ = null;
}
return deterministicKeyBuilder_;
}
private com.google.protobuf.ByteString deterministicSeed_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* The seed for a deterministic key hierarchy. Derived from the mnemonic,
* but cached here for quick startup. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* optional bytes deterministic_seed = 8;
*/
public boolean hasDeterministicSeed() {
return ((bitField0_ & 0x00000080) == 0x00000080);
}
/**
*
* The seed for a deterministic key hierarchy. Derived from the mnemonic,
* but cached here for quick startup. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* optional bytes deterministic_seed = 8;
*/
public com.google.protobuf.ByteString getDeterministicSeed() {
return deterministicSeed_;
}
/**
*
* The seed for a deterministic key hierarchy. Derived from the mnemonic,
* but cached here for quick startup. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* optional bytes deterministic_seed = 8;
*/
public Builder setDeterministicSeed(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000080;
deterministicSeed_ = value;
onChanged();
return this;
}
/**
*
* The seed for a deterministic key hierarchy. Derived from the mnemonic,
* but cached here for quick startup. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* optional bytes deterministic_seed = 8;
*/
public Builder clearDeterministicSeed() {
bitField0_ = (bitField0_ & ~0x00000080);
deterministicSeed_ = getDefaultInstance().getDeterministicSeed();
onChanged();
return this;
}
private org.bitcoinj.wallet.Protos.EncryptedData encryptedDeterministicSeed_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.bitcoinj.wallet.Protos.EncryptedData, org.bitcoinj.wallet.Protos.EncryptedData.Builder, org.bitcoinj.wallet.Protos.EncryptedDataOrBuilder> encryptedDeterministicSeedBuilder_;
/**
*
* Encrypted version of the seed
*
*
* optional .wallet.EncryptedData encrypted_deterministic_seed = 9;
*/
public boolean hasEncryptedDeterministicSeed() {
return ((bitField0_ & 0x00000100) == 0x00000100);
}
/**
*
* Encrypted version of the seed
*
*
* optional .wallet.EncryptedData encrypted_deterministic_seed = 9;
*/
public org.bitcoinj.wallet.Protos.EncryptedData getEncryptedDeterministicSeed() {
if (encryptedDeterministicSeedBuilder_ == null) {
return encryptedDeterministicSeed_ == null ? org.bitcoinj.wallet.Protos.EncryptedData.getDefaultInstance() : encryptedDeterministicSeed_;
} else {
return encryptedDeterministicSeedBuilder_.getMessage();
}
}
/**
*
* Encrypted version of the seed
*
*
* optional .wallet.EncryptedData encrypted_deterministic_seed = 9;
*/
public Builder setEncryptedDeterministicSeed(org.bitcoinj.wallet.Protos.EncryptedData value) {
if (encryptedDeterministicSeedBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
encryptedDeterministicSeed_ = value;
onChanged();
} else {
encryptedDeterministicSeedBuilder_.setMessage(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
*
* Encrypted version of the seed
*
*
* optional .wallet.EncryptedData encrypted_deterministic_seed = 9;
*/
public Builder setEncryptedDeterministicSeed(
org.bitcoinj.wallet.Protos.EncryptedData.Builder builderForValue) {
if (encryptedDeterministicSeedBuilder_ == null) {
encryptedDeterministicSeed_ = builderForValue.build();
onChanged();
} else {
encryptedDeterministicSeedBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000100;
return this;
}
/**
*
* Encrypted version of the seed
*
*
* optional .wallet.EncryptedData encrypted_deterministic_seed = 9;
*/
public Builder mergeEncryptedDeterministicSeed(org.bitcoinj.wallet.Protos.EncryptedData value) {
if (encryptedDeterministicSeedBuilder_ == null) {
if (((bitField0_ & 0x00000100) == 0x00000100) &&
encryptedDeterministicSeed_ != null &&
encryptedDeterministicSeed_ != org.bitcoinj.wallet.Protos.EncryptedData.getDefaultInstance()) {
encryptedDeterministicSeed_ =
org.bitcoinj.wallet.Protos.EncryptedData.newBuilder(encryptedDeterministicSeed_).mergeFrom(value).buildPartial();
} else {
encryptedDeterministicSeed_ = value;
}
onChanged();
} else {
encryptedDeterministicSeedBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000100;
return this;
}
/**
*
* Encrypted version of the seed
*
*
* optional .wallet.EncryptedData encrypted_deterministic_seed = 9;
*/
public Builder clearEncryptedDeterministicSeed() {
if (encryptedDeterministicSeedBuilder_ == null) {
encryptedDeterministicSeed_ = null;
onChanged();
} else {
encryptedDeterministicSeedBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000100);
return this;
}
/**
*
* Encrypted version of the seed
*
*
* optional .wallet.EncryptedData encrypted_deterministic_seed = 9;
*/
public org.bitcoinj.wallet.Protos.EncryptedData.Builder getEncryptedDeterministicSeedBuilder() {
bitField0_ |= 0x00000100;
onChanged();
return getEncryptedDeterministicSeedFieldBuilder().getBuilder();
}
/**
*
* Encrypted version of the seed
*
*
* optional .wallet.EncryptedData encrypted_deterministic_seed = 9;
*/
public org.bitcoinj.wallet.Protos.EncryptedDataOrBuilder getEncryptedDeterministicSeedOrBuilder() {
if (encryptedDeterministicSeedBuilder_ != null) {
return encryptedDeterministicSeedBuilder_.getMessageOrBuilder();
} else {
return encryptedDeterministicSeed_ == null ?
org.bitcoinj.wallet.Protos.EncryptedData.getDefaultInstance() : encryptedDeterministicSeed_;
}
}
/**
*
* Encrypted version of the seed
*
*
* optional .wallet.EncryptedData encrypted_deterministic_seed = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.bitcoinj.wallet.Protos.EncryptedData, org.bitcoinj.wallet.Protos.EncryptedData.Builder, org.bitcoinj.wallet.Protos.EncryptedDataOrBuilder>
getEncryptedDeterministicSeedFieldBuilder() {
if (encryptedDeterministicSeedBuilder_ == null) {
encryptedDeterministicSeedBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.bitcoinj.wallet.Protos.EncryptedData, org.bitcoinj.wallet.Protos.EncryptedData.Builder, org.bitcoinj.wallet.Protos.EncryptedDataOrBuilder>(
getEncryptedDeterministicSeed(),
getParentForChildren(),
isClean());
encryptedDeterministicSeed_ = null;
}
return encryptedDeterministicSeedBuilder_;
}
private java.util.List accountPath_ = java.util.Collections.emptyList();
private void ensureAccountPathIsMutable() {
if (!((bitField0_ & 0x00000200) == 0x00000200)) {
accountPath_ = new java.util.ArrayList(accountPath_);
bitField0_ |= 0x00000200;
}
}
/**
*
* The path to the root. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* repeated uint32 account_path = 10 [packed = true];
*/
public java.util.List
getAccountPathList() {
return java.util.Collections.unmodifiableList(accountPath_);
}
/**
*
* The path to the root. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* repeated uint32 account_path = 10 [packed = true];
*/
public int getAccountPathCount() {
return accountPath_.size();
}
/**
*
* The path to the root. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* repeated uint32 account_path = 10 [packed = true];
*/
public int getAccountPath(int index) {
return accountPath_.get(index);
}
/**
*
* The path to the root. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* repeated uint32 account_path = 10 [packed = true];
*/
public Builder setAccountPath(
int index, int value) {
ensureAccountPathIsMutable();
accountPath_.set(index, value);
onChanged();
return this;
}
/**
*
* The path to the root. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* repeated uint32 account_path = 10 [packed = true];
*/
public Builder addAccountPath(int value) {
ensureAccountPathIsMutable();
accountPath_.add(value);
onChanged();
return this;
}
/**
*
* The path to the root. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* repeated uint32 account_path = 10 [packed = true];
*/
public Builder addAllAccountPath(
java.lang.Iterable extends java.lang.Integer> values) {
ensureAccountPathIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, accountPath_);
onChanged();
return this;
}
/**
*
* The path to the root. Only applicable to a DETERMINISTIC_MNEMONIC key entry.
*
*
* repeated uint32 account_path = 10 [packed = true];
*/
public Builder clearAccountPath() {
accountPath_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
private int outputScriptType_ = 1;
/**
*
* Type of addresses (aka output scripts) to generate for receiving.
*
*
* optional .wallet.Key.OutputScriptType output_script_type = 11;
*/
public boolean hasOutputScriptType() {
return ((bitField0_ & 0x00000400) == 0x00000400);
}
/**
*
* Type of addresses (aka output scripts) to generate for receiving.
*
*
* optional .wallet.Key.OutputScriptType output_script_type = 11;
*/
public org.bitcoinj.wallet.Protos.Key.OutputScriptType getOutputScriptType() {
@SuppressWarnings("deprecation")
org.bitcoinj.wallet.Protos.Key.OutputScriptType result = org.bitcoinj.wallet.Protos.Key.OutputScriptType.valueOf(outputScriptType_);
return result == null ? org.bitcoinj.wallet.Protos.Key.OutputScriptType.P2PKH : result;
}
/**
*
* Type of addresses (aka output scripts) to generate for receiving.
*
*
* optional .wallet.Key.OutputScriptType output_script_type = 11;
*/
public Builder setOutputScriptType(org.bitcoinj.wallet.Protos.Key.OutputScriptType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000400;
outputScriptType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Type of addresses (aka output scripts) to generate for receiving.
*
*
* optional .wallet.Key.OutputScriptType output_script_type = 11;
*/
public Builder clearOutputScriptType() {
bitField0_ = (bitField0_ & ~0x00000400);
outputScriptType_ = 1;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:wallet.Key)
}
// @@protoc_insertion_point(class_scope:wallet.Key)
private static final org.bitcoinj.wallet.Protos.Key DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.bitcoinj.wallet.Protos.Key();
}
public static org.bitcoinj.wallet.Protos.Key getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Key parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Key(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.Key getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ScriptOrBuilder extends
// @@protoc_insertion_point(interface_extends:wallet.Script)
com.google.protobuf.MessageOrBuilder {
/**
* required bytes program = 1;
*/
boolean hasProgram();
/**
* required bytes program = 1;
*/
com.google.protobuf.ByteString getProgram();
/**
*
* Timestamp stored as millis since epoch. Useful for skipping block bodies before this point
* when watching for scripts on the blockchain.
*
*
* required int64 creation_timestamp = 2;
*/
boolean hasCreationTimestamp();
/**
*
* Timestamp stored as millis since epoch. Useful for skipping block bodies before this point
* when watching for scripts on the blockchain.
*
*
* required int64 creation_timestamp = 2;
*/
long getCreationTimestamp();
}
/**
* Protobuf type {@code wallet.Script}
*/
public static final class Script extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:wallet.Script)
ScriptOrBuilder {
private static final long serialVersionUID = 0L;
// Use Script.newBuilder() to construct.
private Script(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Script() {
program_ = com.google.protobuf.ByteString.EMPTY;
creationTimestamp_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Script(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
bitField0_ |= 0x00000001;
program_ = input.readBytes();
break;
}
case 16: {
bitField0_ |= 0x00000002;
creationTimestamp_ = input.readInt64();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_Script_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_Script_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoinj.wallet.Protos.Script.class, org.bitcoinj.wallet.Protos.Script.Builder.class);
}
private int bitField0_;
public static final int PROGRAM_FIELD_NUMBER = 1;
private com.google.protobuf.ByteString program_;
/**
* required bytes program = 1;
*/
public boolean hasProgram() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes program = 1;
*/
public com.google.protobuf.ByteString getProgram() {
return program_;
}
public static final int CREATION_TIMESTAMP_FIELD_NUMBER = 2;
private long creationTimestamp_;
/**
*
* Timestamp stored as millis since epoch. Useful for skipping block bodies before this point
* when watching for scripts on the blockchain.
*
*
* required int64 creation_timestamp = 2;
*/
public boolean hasCreationTimestamp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Timestamp stored as millis since epoch. Useful for skipping block bodies before this point
* when watching for scripts on the blockchain.
*
*
* required int64 creation_timestamp = 2;
*/
public long getCreationTimestamp() {
return creationTimestamp_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
if (!hasProgram()) {
memoizedIsInitialized = 0;
return false;
}
if (!hasCreationTimestamp()) {
memoizedIsInitialized = 0;
return false;
}
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
output.writeBytes(1, program_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
output.writeInt64(2, creationTimestamp_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(1, program_);
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, creationTimestamp_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.bitcoinj.wallet.Protos.Script)) {
return super.equals(obj);
}
org.bitcoinj.wallet.Protos.Script other = (org.bitcoinj.wallet.Protos.Script) obj;
boolean result = true;
result = result && (hasProgram() == other.hasProgram());
if (hasProgram()) {
result = result && getProgram()
.equals(other.getProgram());
}
result = result && (hasCreationTimestamp() == other.hasCreationTimestamp());
if (hasCreationTimestamp()) {
result = result && (getCreationTimestamp()
== other.getCreationTimestamp());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasProgram()) {
hash = (37 * hash) + PROGRAM_FIELD_NUMBER;
hash = (53 * hash) + getProgram().hashCode();
}
if (hasCreationTimestamp()) {
hash = (37 * hash) + CREATION_TIMESTAMP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCreationTimestamp());
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.bitcoinj.wallet.Protos.Script parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoinj.wallet.Protos.Script parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.Script parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoinj.wallet.Protos.Script parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.Script parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.bitcoinj.wallet.Protos.Script parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.Script parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.bitcoinj.wallet.Protos.Script parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.Script parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.bitcoinj.wallet.Protos.Script parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.bitcoinj.wallet.Protos.Script parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.bitcoinj.wallet.Protos.Script parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.bitcoinj.wallet.Protos.Script prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code wallet.Script}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:wallet.Script)
org.bitcoinj.wallet.Protos.ScriptOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_Script_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_Script_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.bitcoinj.wallet.Protos.Script.class, org.bitcoinj.wallet.Protos.Script.Builder.class);
}
// Construct using org.bitcoinj.wallet.Protos.Script.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
program_ = com.google.protobuf.ByteString.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
creationTimestamp_ = 0L;
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.bitcoinj.wallet.Protos.internal_static_wallet_Script_descriptor;
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.Script getDefaultInstanceForType() {
return org.bitcoinj.wallet.Protos.Script.getDefaultInstance();
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.Script build() {
org.bitcoinj.wallet.Protos.Script result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.bitcoinj.wallet.Protos.Script buildPartial() {
org.bitcoinj.wallet.Protos.Script result = new org.bitcoinj.wallet.Protos.Script(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) == 0x00000001)) {
to_bitField0_ |= 0x00000001;
}
result.program_ = program_;
if (((from_bitField0_ & 0x00000002) == 0x00000002)) {
to_bitField0_ |= 0x00000002;
}
result.creationTimestamp_ = creationTimestamp_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return (Builder) super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.bitcoinj.wallet.Protos.Script) {
return mergeFrom((org.bitcoinj.wallet.Protos.Script)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.bitcoinj.wallet.Protos.Script other) {
if (other == org.bitcoinj.wallet.Protos.Script.getDefaultInstance()) return this;
if (other.hasProgram()) {
setProgram(other.getProgram());
}
if (other.hasCreationTimestamp()) {
setCreationTimestamp(other.getCreationTimestamp());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
if (!hasProgram()) {
return false;
}
if (!hasCreationTimestamp()) {
return false;
}
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.bitcoinj.wallet.Protos.Script parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.bitcoinj.wallet.Protos.Script) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString program_ = com.google.protobuf.ByteString.EMPTY;
/**
* required bytes program = 1;
*/
public boolean hasProgram() {
return ((bitField0_ & 0x00000001) == 0x00000001);
}
/**
* required bytes program = 1;
*/
public com.google.protobuf.ByteString getProgram() {
return program_;
}
/**
* required bytes program = 1;
*/
public Builder setProgram(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
program_ = value;
onChanged();
return this;
}
/**
* required bytes program = 1;
*/
public Builder clearProgram() {
bitField0_ = (bitField0_ & ~0x00000001);
program_ = getDefaultInstance().getProgram();
onChanged();
return this;
}
private long creationTimestamp_ ;
/**
*
* Timestamp stored as millis since epoch. Useful for skipping block bodies before this point
* when watching for scripts on the blockchain.
*
*
* required int64 creation_timestamp = 2;
*/
public boolean hasCreationTimestamp() {
return ((bitField0_ & 0x00000002) == 0x00000002);
}
/**
*
* Timestamp stored as millis since epoch. Useful for skipping block bodies before this point
* when watching for scripts on the blockchain.
*
*
* required int64 creation_timestamp = 2;
*/
public long getCreationTimestamp() {
return creationTimestamp_;
}
/**
*
* Timestamp stored as millis since epoch. Useful for skipping block bodies before this point
* when watching for scripts on the blockchain.
*
*
* required int64 creation_timestamp = 2;
*/
public Builder setCreationTimestamp(long value) {
bitField0_ |= 0x00000002;
creationTimestamp_ = value;
onChanged();
return this;
}
/**
*
* Timestamp stored as millis since epoch. Useful for skipping block bodies before this point
* when watching for scripts on the blockchain.
*
*
* required int64 creation_timestamp = 2;
*/
public Builder clearCreationTimestamp() {
bitField0_ = (bitField0_ & ~0x00000002);
creationTimestamp_ = 0L;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:wallet.Script)
}
// @@protoc_insertion_point(class_scope:wallet.Script)
private static final org.bitcoinj.wallet.Protos.Script DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.bitcoinj.wallet.Protos.Script();
}
public static org.bitcoinj.wallet.Protos.Script getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser