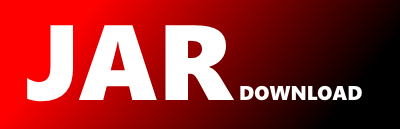
org.bithill.selenium.condition.ElementConditions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aport Show documentation
Show all versions of aport Show documentation
web UI testing made easier
package org.bithill.selenium.condition;
import java.util.List;
import java.util.regex.Pattern;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.ui.ExpectedCondition;
/** Collection of {@link ExpectedCondition}s for {@link WebElement}. */
public class ElementConditions
{
/**
* Condition met when an element contains text matching given pattern.
*
* @param locator locator of the element, element must be displayed
* @param pattern regular expression to match
* @return the matching element
*/
public static ExpectedCondition textMatches(final By locator, final Pattern pattern)
{
return new ExpectedCondition()
{
private String currentText = null;
@Override
public WebElement apply(WebDriver driver)
{
WebElement element = driver.findElement(locator);
if (element.isDisplayed())
{
currentText = element.getText();
if (pattern.matcher(currentText).matches())
{
return element;
}
}
return null;
}
public String toString()
{
return String.format("Current text '%s' does no match pattern '%s'.",
currentText, pattern.toString());
}
};
}
/**
* Condition met when a child (descendant) {@link WebElement element} matching given locator is visible.
*
* @param parentElement parent WebElement, expected to be valid and visible
* @param childElementLocator locator of child WebElement
* @return visible child WebElement
*/
public static ExpectedCondition visibilityOfNestedElement
(final WebElement parentElement, final By childElementLocator)
{
return new ExpectedCondition()
{
@Override
public WebElement apply(WebDriver driver)
{
WebElement element = parentElement.findElement(childElementLocator);
if (element.isDisplayed())
{
return element;
}
return null;
}
@Override
public String toString()
{
return String.format("Element '%s' was not found visible inside it's :parent element '%s'.",
childElementLocator, parentElement);
}
};
}
/**
* Condition met when all child (descendant) {@link WebElement elements} matching given locator are visible.
*
* @param parentElement parent WebElement, expected to be valid and visible
* @param childElementLocator locator of child WebElement
* @return list of visible child WebElements
*/
public static ExpectedCondition> visibilityOfAllNestedElementsLocatedBy
(final WebElement parentElement, final By childElementLocator)
{
return new ExpectedCondition>()
{
@Override
public List apply(WebDriver webDriver)
{
List foundChildren = parentElement.findElements(childElementLocator);
boolean allChildrenAreVisible = foundChildren.stream().allMatch(WebElement::isDisplayed);
return !foundChildren.isEmpty() && allChildrenAreVisible ? foundChildren : null;
}
@Override
public String toString()
{
return String.format("All elements '%s' were not found visible inside it's :parent element '%s'.",
childElementLocator, parentElement);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy