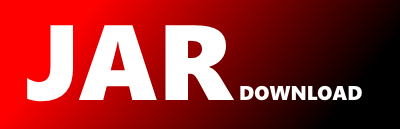
org.bithill.selenium.condition.SelectConditions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aport Show documentation
Show all versions of aport Show documentation
web UI testing made easier
package org.bithill.selenium.condition;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.ui.ExpectedCondition;
import org.openqa.selenium.support.ui.Select;
/** Collection of {@link ExpectedCondition}s for {@link Select}. */
public class SelectConditions
{
/**
* Condition met when a <select> has at least given count of <option>s.
*
* @param locator locator of the select
* @param minValuesCount minimal number of options required
* @return the matching select
*/
public static ExpectedCondition
© 2015 - 2025 Weber Informatics LLC | Privacy Policy