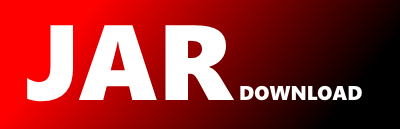
org.bithill.selenium.driver.FasterWebDriverWait Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aport Show documentation
Show all versions of aport Show documentation
web UI testing made easier
package org.bithill.selenium.driver;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.NotFoundException;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.support.ui.Clock;
import org.openqa.selenium.support.ui.FluentWait;
import org.openqa.selenium.support.ui.Sleeper;
import org.openqa.selenium.support.ui.SystemClock;
import org.openqa.selenium.support.ui.WebDriverWait;
/** Copy of {@link WebDriverWait} which using milliseconds for time out */
public class FasterWebDriverWait extends FluentWait {
private final static long DEFAULT_SLEEP_TIMEOUT = 10;
/**
* Wait will ignore instances of NotFoundException that are encountered (thrown) by default in
* the 'until' condition, and immediately propagate all others. You can add more to the ignore
* list by calling ignoring(exceptions to add).
*
* @param driver The WebDriver instance to pass to the expected conditions
* @param timeOutInMillis The timeout in milliseconds when an expectation is called
* @see WebDriverWait#ignoring(Class)
*/
public FasterWebDriverWait(WebDriver driver, long timeOutInMillis) {
this(driver, new SystemClock(), Sleeper.SYSTEM_SLEEPER, timeOutInMillis, DEFAULT_SLEEP_TIMEOUT);
}
/**
* Wait will ignore instances of NotFoundException that are encountered (thrown) by default in
* the 'until' condition, and immediately propagate all others. You can add more to the ignore
* list by calling ignoring(exceptions to add).
*
* @param driver The WebDriver instance to pass to the expected conditions
* @param timeOutInMillis The timeout in milliseconds when an expectation is called
* @param sleepInMillis The duration in milliseconds to sleep between polls.
* @see WebDriverWait#ignoring(Class)
*/
public FasterWebDriverWait(WebDriver driver, long timeOutInMillis, long sleepInMillis) {
this(driver, new SystemClock(), Sleeper.SYSTEM_SLEEPER, timeOutInMillis, sleepInMillis);
}
/**
* @param driver The WebDriver instance to pass to the expected conditions
* @param clock The clock to use when measuring the timeout
* @param sleeper Object used to make the current thread go to sleep.
* @param timeOutInMillis The timeout in milliseconds when an expectation is
* @param sleepTimeOut The timeout used whilst sleeping. Defaults to 500ms called.
*/
public FasterWebDriverWait(WebDriver driver, Clock clock, Sleeper sleeper, long timeOutInMillis, long sleepTimeOut) {
super(driver, clock, sleeper);
withTimeout(timeOutInMillis, TimeUnit.MILLISECONDS);
pollingEvery(sleepTimeOut, TimeUnit.MILLISECONDS);
ignoring(NotFoundException.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy