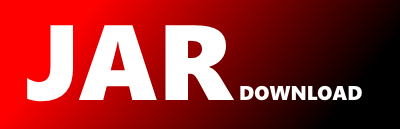
org.bithill.selenium.driver.WebDriverHandle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aport Show documentation
Show all versions of aport Show documentation
web UI testing made easier
package org.bithill.selenium.driver;
import java.util.HashMap;
import java.util.Map;
import org.bithill.selenium.resolving.Resolvable;
import org.openqa.selenium.JavascriptExecutor;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.support.ui.FluentWait;
import org.openqa.selenium.support.ui.WebDriverWait;
import static java.util.Collections.unmodifiableMap;
/**
* Class for holding a Webdriver instance and derived objects.
*/
public class WebDriverHandle
{
/** duration of the default explicit wait used by {@link Resolvable} during resolve */
public static final long DEFAULT_TIMEOUT_SECONDS = 6;
/** name of the default explicit wait*/
public static final String DEFAULT_WAIT = "default";
/** name of the default explicit wait for list resolving */
public static final String DEFAULT_LIST_WAIT = "list default";
/** Map with default properties:
*
* -
* seleniumUrl -- URL of a Selenium hub running on local machine, ignored when !this.isRemote().
*
*
**/
public static final Map DEFAULTS;
static
{
final String SELENIUM_ON_LOCALHOST = "http://127.0.0.1:4444";
int defaultsCapacity = 2;
Map defaultsInternal = new HashMap<>(defaultsCapacity);
defaultsInternal.put("selenium.url", SELENIUM_ON_LOCALHOST);
defaultsInternal.put("selenium.hub.url", SELENIUM_ON_LOCALHOST + "/wd/hub");
DEFAULTS = unmodifiableMap(defaultsInternal);
}
private WebDriver driver;
public WebDriver getDriver() { return driver; }
private boolean remote;
/** @return true if the driver instance is {@link org.openqa.selenium.remote.RemoteWebDriver remote}
* (i.e. typically connected via Selenium hub),
* false for local drivers (FirefoxDriver, ChromeDriver,...) */
public boolean isRemote() { return remote; }
@SuppressWarnings("unchecked")
private Map info = new HashMap<>();
/** @return map of key-value pairs with driver-related information */
public Map getInfo() { return info; }
/** sets map of key-value pairs with driver-related information
* @param info info map to set */
public void setInfo(Map info) { this.info = info; }
public JavascriptExecutor getJs() { return (JavascriptExecutor) getDriver(); }
private Map> waits;
/** @return Collection of reusable named {@link WebDriverWait WebDriverWaits}. */
public Map> getWaits() { return this.waits; }
public WebDriverHandle(WebDriver driver, boolean remote, Map> waits)
{
this.driver = driver;
this.remote = remote;
this.waits = waits;
addDefaultWaits();
}
public WebDriverHandle(WebDriver driver, boolean remote)
{
this.driver = driver;
this.remote = remote;
this.waits = new HashMap<>(1);
addDefaultWaits();
}
/** Add default waits to the internal waits collection. */
protected void addDefaultWaits()
{
waits.putIfAbsent(DEFAULT_WAIT , new WebDriverWait(getDriver(), DEFAULT_TIMEOUT_SECONDS));
waits.putIfAbsent(DEFAULT_LIST_WAIT, new WebDriverWait(getDriver(), DEFAULT_TIMEOUT_SECONDS));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy