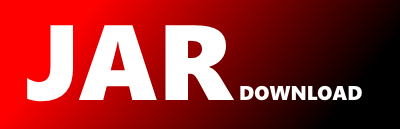
org.bithill.selenium.resolving.FieldOfResolvable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aport Show documentation
Show all versions of aport Show documentation
web UI testing made easier
package org.bithill.selenium.resolving;
import javax.annotation.Nullable;
import java.lang.reflect.Field;
import java.util.Arrays;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.ui.Select;
/**
* Functions for checking attributes of a field in a Resolvable.
*/
class FieldOfResolvable
{
// ---------- checking field annotations ----------------------------------
/** Checks if the given field is Resolvable.
*
* @param field field to check
* @return true if the field's class is Resolvable, false else
*/
protected static boolean isResolvedByAnnotated(Field field)
{
return field.isAnnotationPresent(ResolveBy.class);
}
/** Checks if the given field is container Resolvable.
*
* @param field field to check
* @return true if the field's class is Resolvable and isResolvable is set to true, false else
*/
protected static boolean isContainerResolvable(Field field)
{
ResolveBy resolveBy = field.getAnnotation(ResolveBy.class);
return resolveBy != null && resolveBy.isContainer();
}
/** Checks if the given field is Nullable.
*
* @param field field to check
* @return true if the field's class is Nullable, false else
*/
protected static boolean isNullableAnnotated(Field field)
{
return field.isAnnotationPresent(Nullable.class);
}
/** Checks if the given field is in at least one of the given groups.
*
* @param field field to check
* @param groups list of groups
* @return true if the is in one of given groups, false else
*/
protected static boolean isGroupMember(Field field, String[] groups)
{
boolean result = false;
Set elementGroups = new HashSet<>(Arrays.asList(field.getAnnotation(ResolveBy.class).groups()));
for (String requestedGroup : groups)
{
if (elementGroups.contains(requestedGroup))
{
result = true;
break;
}
}
return result;
}
// ---------- checking field type -----------------------------------------
/** Checks if the given field is Resolvable.
*
* @param field field to check
* @return true if the field's class is Resolvable, false else
*/
protected static boolean isResolvable(Field field)
{
return Resolvable.class.isAssignableFrom(field.getType());
}
/** Checks if the given field is {@link WebElement}.
*
* @param field field to check
* @return true if the field's class is WebElement, false else
*/
protected static boolean isWebElement(Field field)
{
return WebElement.class.isAssignableFrom(field.getType());
}
/** Checks if the given field is {@link Select}.
*
* @param field field to check
* @return true if the field's class is Select, false else
*/
protected static boolean isSelect(Field field)
{
return Select.class.isAssignableFrom(field.getType());
}
/** Checks if the given field is {@link List}.
*
* @param field field to check
* @return true if the field's class is List, false else
*/
protected static boolean isList(Field field)
{
return List.class.isAssignableFrom(field.getType());
}
/** Checks if the given field is {@link ResolvableList}.
*
* @param field field to check
* @return true if the field's class is ResolvableList, false else
*/
protected static boolean isResolvableList(Field field)
{
return ResolvableList.class.isAssignableFrom(field.getType());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy