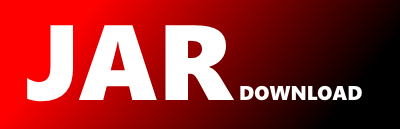
org.bithill.selenium.resolving.Page Maven / Gradle / Ivy
Show all versions of aport Show documentation
package org.bithill.selenium.resolving;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.Date;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.bithill.selenium.driver.WebDriverHandle;
import org.bithill.selenium.site.MinimalSiteConfig;
import org.bithill.selenium.site.Site;
import org.bithill.selenium.site.SiteConfig;
import org.openqa.selenium.OutputType;
import org.openqa.selenium.TakesScreenshot;
/** An abstraction of a tested page. */
public abstract class Page extends Resolvable implements AutoCloseable
{
protected final Logger log = LogManager.getLogger(Page.class);
/** Configuration for the web site the page is part of. */
protected SiteConfig siteConfig;
public SiteConfig getSiteConfig() { return this.siteConfig; }
/** Page URL parameters - an URL relative to a Site URL or parameters to substitute to Site URL template. */
private String[] pageUrlParams = new String[0];
protected String[] getPageUrlParams () { return pageUrlParams; }
public void setPageUrlParams (String... pageUrlParams) { this.pageUrlParams = pageUrlParams; }
public Page(WebDriverHandle driverHandle)
{
super(driverHandle);
getSiteConfigFromAnnotation();
}
/** Creates a SiteConfig - if not specified, an instance of SiteConfigMinimal is used. */
private void getSiteConfigFromAnnotation()
{
Site site = getClass().getAnnotation(Site.class);
if (site != null)
{
try
{
siteConfig = site.config().newInstance();
}
catch (InstantiationException | IllegalAccessException ex)
{
log.error(ex);
}
}
else
{
log.info("Class '{}' does not have SiteConfig.", this.getClass());
siteConfig = new MinimalSiteConfig();
}
}
/**
* Opens the web page associated with this Page class.
*
* @return an instance of Page sub-class
*/
public Page open()
{
getDriverHandle().getDriver().get(pageUrl());
checkOpened();
return this;
}
/**
* Provides Page URL.
*
* @return Page URL as application of {@link #pageUrlParams} to Site URL/
* By default it only concatenates pageUrlParams to Site URL.
*/
protected String pageUrl()
{
StringBuilder pageUrl = new StringBuilder(siteConfig.getUrl());
for(String pageUrlParam : pageUrlParams)
{
pageUrl.append(pageUrlParam);
}
return pageUrl.toString();
}
@Override
public void close()
{
getDriver().close();
}
/**
* Checks that the desired pages is opened.
* The page is considered successfully open when this methods does not throw an exception.
*
* @throws InvalidPageException when the page check fails
* @return the reference to the Page when check is successful
*/
protected abstract Page checkOpened();
/**
* Takes a screenshot in PNG format and saves it to disk.
*
* The target directory is set via SiteConfig.
* The used WebDriver must implement TakesScreenshot interface.
*
* @param screenshotFileName path to a resulting screenshot file
*/
public void takeScreenshot(String screenshotFileName)
{
File screenshotDir = new File(siteConfig.getScreenshotDir());
if (!screenshotDir.exists())
{
log.info("Target directory '{}' does not exist. Creating a new one.", screenshotDir.getPath());
boolean directoryCreationSucceeded = screenshotDir.mkdir();
if (!directoryCreationSucceeded)
{
log.error("Failed to create directory for screenshots: " + screenshotDir);
}
else
{
File screenshotFile = new File(screenshotDir, screenshotFileName);
try
{
log.trace("Taking screenshot.");
byte[] screenshotData = ((TakesScreenshot) getDriver()).getScreenshotAs(OutputType.BYTES);
log.debug("Saving screenshot to file '{}'.", screenshotFile);
try (FileOutputStream imageOutputStream = new FileOutputStream(screenshotFile))
{
imageOutputStream.write(screenshotData);
}
}
catch (ClassCastException ex)
{
log.warn("Used driver '{}' does not support taking of screenshots.", getDriver().getClass());
}
catch (IOException ex)
{
log.error("Unable to write screenshot to file '{}'.", screenshotFile.getPath());
}
}
}
}
/**
* Takes a screenshot in PNG format and saves it to the current directory directory.
* The screenshot is named after the Page class and time-stamped.
*/
public void takeScreenshot()
{
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd_hh-mm-ss");
String fileName = String.format("%s_%s.png", this.getClass().getSimpleName(), dateFormat.format(new Date()));
takeScreenshot(fileName);
}
}