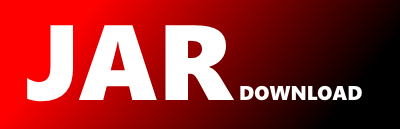
org.bklab.flow.dialog.ModalDialog Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-vaadin-flow Show documentation
Show all versions of fluent-vaadin-flow Show documentation
Broderick Labs for fluent vaadin flow. Inherits common Vaadin components.
package org.bklab.flow.dialog;
import com.vaadin.flow.component.ClickEvent;
import com.vaadin.flow.component.Component;
import com.vaadin.flow.component.ComponentEventListener;
import com.vaadin.flow.component.Html;
import com.vaadin.flow.component.button.Button;
import com.vaadin.flow.component.dependency.CssImport;
import com.vaadin.flow.component.dialog.Dialog;
import com.vaadin.flow.component.html.Div;
import com.vaadin.flow.component.icon.VaadinIcon;
import org.bklab.flow.components.button.FluentButton;
import org.bklab.flow.factory.ButtonFactory;
import org.bklab.flow.factory.DialogFactory;
import org.bklab.flow.factory.DivFactory;
import org.bklab.flow.layout.ToolBar;
import org.bklab.flow.text.TitleLabel;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
@CssImport(value = "./styles/org/bklab/component/dialog/modal-dialog.css")
@CssImport(value = "./styles/org/bklab/component/dialog/vaadin-dialog-overlay.css", themeFor = "vaadin-dialog-overlay")
public class ModalDialog extends Dialog {
private final ToolBar toolBar = new ToolBar();
private final Div content = new Div();
private final ToolBar footer = new ToolBar();
private final TitleLabel title = new TitleLabel("提示");
public ModalDialog() {
add(toolBar, content, footer);
toolBar.addClassName("modal-dialog-toolbar");
content.addClassName("modal-dialog-content");
footer.addClassName("modal-dialog-footer");
toolBar.left(title).right(createCloseButton());
setDraggable(true);
setModal(true);
setResizable(true);
}
public ModalDialog title(String title) {
this.title.removeAll();
this.title.setText(title);
return this;
}
public ModalDialog title(Component... components) {
this.title.removeAll();
this.title.add(components);
return this;
}
public ModalDialog addButton(BiConsumer buttonModalConsumer) {
buttonModalConsumer.accept(new ButtonFactory().lumoSmall(), this);
return this;
}
public ModalDialog addButton(Consumer buttonModalConsumer) {
FluentButton fluentButton = new FluentButton();
footerRight(fluentButton);
buttonModalConsumer.accept(fluentButton);
return this;
}
public ModalDialog addButton(Button button) {
footerRight(button);
return this;
}
public ModalDialog addPrimaryButton(Consumer buttonModalConsumer) {
FluentButton fluentButton = new FluentButton().primary();
footerRight(fluentButton);
buttonModalConsumer.accept(fluentButton);
return this;
}
public ModalDialog addPrimaryButton(String text, ComponentEventListener> listener) {
footerRight(new FluentButton(text, listener));
return this;
}
public ModalDialog addPrimaryButton(VaadinIcon icon, String text, ComponentEventListener> listener) {
footerRight(new FluentButton(icon, text, listener));
return this;
}
public ModalDialog addSaveButton(ComponentEventListener> listener) {
footerRight(FluentButton.saveButton().primary().asFactory().clickListener(listener).get());
return this;
}
public ModalDialog addUpdateButton(ComponentEventListener> listener) {
footerRight(FluentButton.updateButton().primary().asFactory().clickListener(listener).get());
return this;
}
public ModalDialog addSaveButton(boolean updateMode, ComponentEventListener> listener) {
return updateMode ? addUpdateButton(listener) : addSaveButton(listener);
}
public ModalDialog addCloseButton() {
return addCloseButton("关闭");
}
public ModalDialog height(String height) {
setHeight(height);
return this;
}
public ModalDialog width(String width) {
setWidth(width);
return this;
}
public ModalDialog width(String minWidth, String width, String maxWidth) {
setMinWidth(minWidth);
setWidth(width);
setMaxWidth(maxWidth);
return this;
}
public ModalDialog height(String minHeight, String height, String maxHeight) {
setMinHeight(minHeight);
setHeight(height);
setMaxHeight(maxHeight);
return this;
}
public ModalDialog addCancelButton() {
return footerRight(FluentButton.cancelButton().asFactory().clickListener(event -> close()).get());
}
public ModalDialog addCloseButton(String text) {
return footerRight(FluentButton.cancelButton().asFactory().text(text).clickListener(event -> close()).get());
}
public ModalDialog toolBarRight(Component... components) {
toolBar.right(components);
return this;
}
public ModalDialog toolBarMiddle(Component... components) {
toolBar.middle(components);
return this;
}
public ModalDialog toolBarLeft(Component... components) {
toolBar.left(components);
return this;
}
public ModalDialog content(Component... components) {
content.add(components);
return this;
}
public ModalDialog content(String text) {
content.add(text);
return this;
}
public ModalDialog footerRight(Component... components) {
footer.right(components);
return this;
}
public ModalDialog footerLeft(Component... components) {
footer.left(components);
return this;
}
public ModalDialog footerMiddle(Component... components) {
footer.middle(components);
return this;
}
public ToolBar getToolBar() {
return toolBar;
}
public Div getContent() {
return content;
}
public ToolBar getFooter() {
return footer;
}
public TitleLabel getTitle() {
return title;
}
public ModalDialog noFooter() {
this.footer.setVisible(false);
this.content.addClassName("modal-dialog-no-footer");
return this;
}
public ModalDialog hasFooter() {
this.footer.setVisible(true);
this.content.removeClassName("modal-dialog-no-footer");
return this;
}
public DialogFactory asFactory() {
return new DialogFactory(this);
}
private Div createCloseButton() {
return new DivFactory(new Html(" ")).clickListener(e -> close()).className("dialog-modal-close-button").get();
}
public ModalDialog size(String width, String height) {
this.setWidth(width);
this.setHeight(height);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy