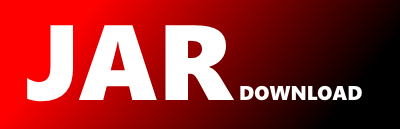
org.bklab.flow.factory.ComboBoxFactory Maven / Gradle / Ivy
package org.bklab.flow.factory;
import com.vaadin.flow.component.ComponentEventListener;
import com.vaadin.flow.component.ItemLabelGenerator;
import com.vaadin.flow.component.combobox.ComboBox;
import com.vaadin.flow.component.combobox.GeneratedVaadinComboBox;
import com.vaadin.flow.component.combobox.dataview.ComboBoxDataView;
import com.vaadin.flow.component.combobox.dataview.ComboBoxLazyDataView;
import com.vaadin.flow.component.combobox.dataview.ComboBoxListDataView;
import com.vaadin.flow.data.provider.ListDataProvider;
import com.vaadin.flow.data.renderer.Renderer;
import com.vaadin.flow.function.SerializableFunction;
import org.bklab.flow.FlowFactory;
import org.bklab.flow.base.*;
import java.util.Collection;
public class ComboBoxFactory extends FlowFactory, ComboBoxFactory> implements
GeneratedVaadinComboBoxFactory, ComboBoxFactory>,
HasSizeFactory, ComboBoxFactory>,
HasValidationFactory, ComboBoxFactory>,
HasDataViewFactory, ComboBox, ComboBoxFactory>,
HasListDataViewFactory, ComboBox, ComboBoxFactory>,
HasLazyDataViewFactory, ComboBox, ComboBoxFactory>,
HasHelperFactory, ComboBoxFactory> {
public ComboBoxFactory() {
super(new ComboBox<>());
}
public ComboBoxFactory(String label) {
super(new ComboBox<>(label));
}
public ComboBoxFactory(String label, Collection items) {
super(new ComboBox<>(label, items));
}
@SafeVarargs
public ComboBoxFactory(String label, T... items) {
super(new ComboBox<>(label, items));
}
public ComboBoxFactory(ComboBox component) {
super(component);
}
public ComboBoxFactory value(T value) {
get().setValue(value);
return this;
}
public ComboBoxFactory opened(boolean opened) {
get().setOpened(opened);
return this;
}
public ComboBoxFactory required(boolean required) {
get().setRequired(required);
return this;
}
public ComboBoxFactory placeholder(String placeholder) {
get().setPlaceholder(placeholder);
return this;
}
public ComboBoxFactory pageSize(int pageSize) {
get().setPageSize(pageSize);
return this;
}
public ComboBoxFactory pattern(String pattern) {
get().setPattern(pattern);
return this;
}
public ComboBoxFactory autofocus(boolean autofocus) {
get().setAutofocus(autofocus);
return this;
}
public ComboBoxFactory label(String label) {
get().setLabel(label);
return this;
}
@SafeVarargs
public final ComboBoxFactory items(ComboBox.ItemFilter comboBoxItemFilterT, T... t) {
get().setItems(comboBoxItemFilterT, t);
return this;
}
public ComboBoxFactory items(ComboBox.ItemFilter comboBoxItemFilterT, Collection collectionT) {
get().setItems(comboBoxItemFilterT, collectionT);
return this;
}
public ComboBoxFactory items(Collection items) {
get().setItems(items);
return this;
}
public ComboBoxFactory itemsAndSelectFirst(Collection items) {
get().setItems(items);
if (items != null) items.stream().findFirst().ifPresent(get()::setValue);
return this;
}
public ComboBoxFactory dataProvider(ComboBox.FetchItemsCallback fetchItems, SerializableFunction sizeCallback) {
get().setDataProvider(fetchItems, sizeCallback);
return this;
}
public ComboBoxFactory dataProvider(ComboBox.ItemFilter itemFilter, ListDataProvider listDataProvider) {
get().setDataProvider(itemFilter, listDataProvider);
return this;
}
public ComboBoxFactory dataProvider(ListDataProvider dataProvider) {
get().setDataProvider(dataProvider);
return this;
}
public ComboBoxFactory errorMessage(String errorMessage) {
get().setErrorMessage(errorMessage);
return this;
}
public ComboBoxFactory invalid(boolean invalid) {
get().setInvalid(invalid);
return this;
}
public ComboBoxFactory renderer(Renderer renderer) {
get().setRenderer(renderer);
return this;
}
public ComboBoxFactory customValueSetListener(ComponentEventListener>> listener) {
get().addCustomValueSetListener(listener);
return this;
}
public ComboBoxFactory itemLabelGenerator(ItemLabelGenerator itemLabelGenerator) {
get().setItemLabelGenerator(itemLabelGenerator);
return this;
}
public ComboBoxFactory clearButtonVisible(boolean clearButtonVisible) {
get().setClearButtonVisible(clearButtonVisible);
return this;
}
public ComboBoxFactory requiredIndicatorVisible(boolean requiredIndicatorVisible) {
get().setRequiredIndicatorVisible(requiredIndicatorVisible);
return this;
}
public ComboBoxFactory preventInvalidInput(boolean preventInvalidInput) {
get().setPreventInvalidInput(preventInvalidInput);
return this;
}
public ComboBoxFactory allowCustomValue(boolean allowCustomValue) {
get().setAllowCustomValue(allowCustomValue);
return this;
}
public ComboBoxFactory lumoSmall() {
get().getElement().setAttribute("theme", "small");
get().getStyle().set("margin-top", "auto 0").set("margin-bottom", "auto 0");
return this;
}
public ComboBoxFactory lumoSmall30pxHeight() {
get().getElement().setAttribute("theme", "small");
get().getStyle().set("height", "30px")
.set("margin-top", "auto 0").set("margin-bottom", "auto 0");
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy