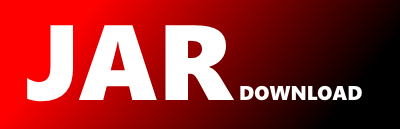
org.bklab.flow.factory.GridFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-vaadin-flow Show documentation
Show all versions of fluent-vaadin-flow Show documentation
Broderick Labs for fluent vaadin flow. Inherits common Vaadin components.
package org.bklab.flow.factory;
import com.vaadin.flow.component.Component;
import com.vaadin.flow.component.ComponentEventListener;
import com.vaadin.flow.component.grid.*;
import com.vaadin.flow.component.grid.dataview.GridDataView;
import com.vaadin.flow.component.grid.dataview.GridLazyDataView;
import com.vaadin.flow.component.grid.dataview.GridListDataView;
import com.vaadin.flow.component.grid.dnd.GridDragEndEvent;
import com.vaadin.flow.component.grid.dnd.GridDragStartEvent;
import com.vaadin.flow.component.grid.dnd.GridDropEvent;
import com.vaadin.flow.component.grid.dnd.GridDropMode;
import com.vaadin.flow.data.event.SortEvent;
import com.vaadin.flow.data.provider.DataGenerator;
import com.vaadin.flow.data.provider.DataProvider;
import com.vaadin.flow.data.renderer.Renderer;
import com.vaadin.flow.data.selection.SelectionListener;
import com.vaadin.flow.function.SerializableFunction;
import com.vaadin.flow.function.SerializablePredicate;
import com.vaadin.flow.function.ValueProvider;
import org.bklab.flow.FlowFactory;
import org.bklab.flow.base.*;
import java.util.List;
import java.util.Map;
public class GridFactory extends FlowFactory, GridFactory> implements
HasStyleFactory, GridFactory>,
HasListDataViewFactory, Grid, GridFactory>,
HasDataViewFactory, Grid, GridFactory>,
HasLazyDataViewFactory, Grid, GridFactory>,
HasSizeFactory, GridFactory>,
FocusableFactory, GridFactory>,
HasThemeFactory, GridFactory>,
HasDataGeneratorsFactory, GridFactory>,
SortEventSortNotifierFactory, GridSortOrder, GridFactory> {
public GridFactory() {
this(new Grid<>());
}
public GridFactory(Grid component) {
super(component);
}
public GridFactory(int pageSize) {
this(new Grid<>(pageSize));
}
public GridFactory(Class beanType, boolean autoCreateColumns) {
this(new Grid<>(beanType, autoCreateColumns));
}
public GridFactory(Class beanType) {
this(beanType, true);
}
public GridFactory columnAutoWidth() {
get().getColumns().forEach(c -> c.setAutoWidth(true));
return this;
}
public GridFactory columnAlignCenter() {
get().getColumns().forEach(c -> c.setTextAlign(ColumnTextAlign.CENTER));
return this;
}
public GridFactory columnAlignEnd() {
get().getColumns().forEach(c -> c.setTextAlign(ColumnTextAlign.END));
return this;
}
public GridFactory columnAlignStart() {
get().getColumns().forEach(c -> c.setTextAlign(ColumnTextAlign.START));
return this;
}
public GridFactory columnAlignStart(String... keys) {
for (String key : keys) {
get().getColumnByKey(key).setTextAlign(ColumnTextAlign.START);
}
return this;
}
public GridFactory columnAlignCenter(String... keys) {
for (String key : keys) {
get().getColumnByKey(key).setTextAlign(ColumnTextAlign.CENTER);
}
return this;
}
public GridFactory columnAlignEnd(String... keys) {
for (String key : keys) {
get().getColumnByKey(key).setTextAlign(ColumnTextAlign.END);
}
return this;
}
public GridFactory sort(List> sort) {
get().sort(sort);
return this;
}
public GridFactory select(T select) {
get().select(select);
return this;
}
@Deprecated
public GridFactory dataProvider(DataProvider dataProvider) {
get().setDataProvider(dataProvider);
return this;
}
public GridFactory columnResizeListener(ComponentEventListener> columnResizeListener) {
get().addColumnResizeListener(columnResizeListener);
return this;
}
public GridFactory classNameGenerator(SerializableFunction classNameGenerator) {
get().setClassNameGenerator(classNameGenerator);
return this;
}
public GridFactory removeThemeVariants(GridVariant... removeThemeVariants) {
get().removeThemeVariants(removeThemeVariants);
return this;
}
public GridFactory dragStartListener(ComponentEventListener> dragStartListener) {
get().addDragStartListener(dragStartListener);
return this;
}
public GridFactory selectionDragDetails(int draggedItemsCount, Map dragData) {
get().setSelectionDragDetails(draggedItemsCount, dragData);
return this;
}
public GridFactory columnReorderListener(ComponentEventListener> listener) {
get().addColumnReorderListener(listener);
return this;
}
public GridFactory columnReorderingAllowed(boolean columnReorderingAllowed) {
get().setColumnReorderingAllowed(columnReorderingAllowed);
return this;
}
public GridFactory selectionListener(SelectionListener, T> listener) {
get().addSelectionListener(listener);
return this;
}
public GridFactory recalculateColumnWidths() {
get().recalculateColumnWidths();
return this;
}
public GridFactory detailsVisibleOnClick(boolean detailsVisibleOnClick) {
get().setDetailsVisibleOnClick(detailsVisibleOnClick);
return this;
}
public GridFactory verticalScrollingEnabled(boolean verticalScrollingEnabled) {
get().setVerticalScrollingEnabled(verticalScrollingEnabled);
return this;
}
public GridFactory itemClickListener(ComponentEventListener> itemClickListener) {
get().addItemClickListener(itemClickListener);
return this;
}
public GridFactory dragDataGenerator(String type, SerializableFunction dragDataGenerator) {
get().setDragDataGenerator(type, dragDataGenerator);
return this;
}
public GridFactory itemDetailsRenderer(Renderer itemDetailsRenderer) {
get().setItemDetailsRenderer(itemDetailsRenderer);
return this;
}
public GridFactory itemDoubleClickListener(ComponentEventListener> itemDoubleClickListener) {
get().addItemDoubleClickListener(itemDoubleClickListener);
return this;
}
public GridFactory dataGenerator(DataGenerator dataGenerator) {
get().addDataGenerator(dataGenerator);
return this;
}
public GridFactory sortListener(ComponentEventListener, GridSortOrder>> listener) {
get().addSortListener(listener);
return this;
}
public GridFactory column(Renderer renderer, String... sortingProperties) {
get().addColumn(renderer, sortingProperties);
return this;
}
public GridFactory column(Renderer column) {
get().addColumn(column);
return this;
}
public GridFactory column(String column) {
get().addColumn(column);
return this;
}
public > GridFactory column(ValueProvider valueProvider, String... sortingProperties) {
get().addColumn(valueProvider, sortingProperties);
return this;
}
public GridFactory column(ValueProvider column) {
get().addColumn(column);
return this;
}
public GridFactory setColumns(String... columns) {
get().setColumns(columns);
return this;
}
public GridFactory componentColumn(ValueProvider componentColumn) {
get().addComponentColumn(componentColumn);
return this;
}
public GridFactory columns(String... propertyNames) {
get().addColumns(propertyNames);
return this;
}
public GridFactory appendHeaderRow() {
get().appendHeaderRow();
return this;
}
public GridFactory sortableColumns(String... sortableColumns) {
get().setSortableColumns(sortableColumns);
return this;
}
public GridFactory appendFooterRow() {
get().appendFooterRow();
return this;
}
public GridFactory themeVariants(GridVariant... themeVariants) {
get().addThemeVariants(themeVariants);
return this;
}
public GridFactory prependHeaderRow() {
get().prependHeaderRow();
return this;
}
public GridFactory prependFooterRow() {
get().prependFooterRow();
return this;
}
public GridFactory asSingleSelect() {
get().asSingleSelect();
return this;
}
public GridFactory asMultiSelect() {
get().asMultiSelect();
return this;
}
public GridFactory deselectAll() {
get().deselectAll();
return this;
}
public GridFactory pageSize(int pageSize) {
get().setPageSize(pageSize);
return this;
}
public GridFactory selectionMode(Grid.SelectionMode selectionMode) {
get().setSelectionMode(selectionMode);
return this;
}
public GridFactory deselect(T deselect) {
get().deselect(deselect);
return this;
}
@SafeVarargs
public final GridFactory removeColumns(Grid.Column... removeColumns) {
get().removeColumns(removeColumns);
return this;
}
public GridFactory removeColumnByKey(String removeColumnByKey) {
get().removeColumnByKey(removeColumnByKey);
return this;
}
public GridFactory removeAllColumns() {
get().removeAllColumns();
return this;
}
public GridFactory detailsVisible(T item, boolean visible) {
get().setDetailsVisible(item, visible);
return this;
}
public GridFactory removeColumn(Grid.Column removeColumn) {
get().removeColumn(removeColumn);
return this;
}
public GridFactory multiSort(boolean multiSort) {
get().setMultiSort(multiSort);
return this;
}
public GridFactory contextMenu() {
get().addContextMenu();
return this;
}
public GridFactory valueProvider(String property, ValueProvider valueProvider) {
get().addValueProvider(property, valueProvider);
return this;
}
public GridFactory heightByRows(boolean heightByRows) {
get().setHeightByRows(heightByRows);
return this;
}
public GridFactory dragEndListener(ComponentEventListener> dragEndListener) {
get().addDragEndListener(dragEndListener);
return this;
}
public GridFactory dragFilter(SerializablePredicate dragFilter) {
get().setDragFilter(dragFilter);
return this;
}
public GridFactory dropMode(GridDropMode dropMode) {
get().setDropMode(dropMode);
return this;
}
public GridFactory rowsDraggable(boolean rowsDraggable) {
get().setRowsDraggable(rowsDraggable);
return this;
}
public GridFactory dropFilter(SerializablePredicate dropFilter) {
get().setDropFilter(dropFilter);
return this;
}
public GridFactory dropListener(ComponentEventListener> dropListener) {
get().addDropListener(dropListener);
return this;
}
public GridFactory scrollToStart() {
get().scrollToStart();
return this;
}
@SafeVarargs
public final GridFactory columnOrder(Grid.Column... columns) {
get().setColumnOrder(columns);
return this;
}
public GridFactory columnOrder(List> columns) {
get().setColumnOrder(columns);
return this;
}
public GridFactory scrollToIndex(int scrollToIndex) {
get().scrollToIndex(scrollToIndex);
return this;
}
public GridFactory scrollToEnd() {
get().scrollToEnd();
return this;
}
public GridFactory lumoNoBorder() {
get().addThemeVariants(GridVariant.LUMO_NO_BORDER);
return this;
}
public GridFactory lumoNoRowBorders() {
get().addThemeVariants(GridVariant.LUMO_NO_ROW_BORDERS);
return this;
}
public GridFactory lumoColumnBorders() {
get().addThemeVariants(GridVariant.LUMO_COLUMN_BORDERS);
return this;
}
public GridFactory lumoRowStripes() {
get().addThemeVariants(GridVariant.LUMO_ROW_STRIPES);
return this;
}
public GridFactory lumoCompact() {
get().addThemeVariants(GridVariant.LUMO_COMPACT);
return this;
}
public GridFactory lumoWrapCellContent() {
get().addThemeVariants(GridVariant.LUMO_WRAP_CELL_CONTENT);
return this;
}
public GridFactory materialColumnDividers() {
get().addThemeVariants(GridVariant.MATERIAL_COLUMN_DIVIDERS);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy