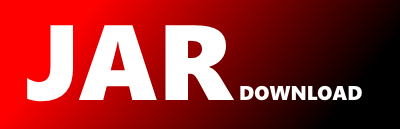
org.bklab.flow.factory.TreeGridFactory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-vaadin-flow Show documentation
Show all versions of fluent-vaadin-flow Show documentation
Broderick Labs for fluent vaadin flow. Inherits common Vaadin components.
package org.bklab.flow.factory;
import com.vaadin.flow.component.Component;
import com.vaadin.flow.component.ComponentEventListener;
import com.vaadin.flow.component.treegrid.CollapseEvent;
import com.vaadin.flow.component.treegrid.ExpandEvent;
import com.vaadin.flow.component.treegrid.TreeGrid;
import com.vaadin.flow.data.provider.DataProvider;
import com.vaadin.flow.data.provider.hierarchy.HierarchicalDataProvider;
import com.vaadin.flow.function.ValueProvider;
import org.bklab.flow.FlowFactory;
import org.bklab.flow.base.HasHierarchicalDataProviderFactory;
import java.util.Collection;
import java.util.stream.Stream;
public class TreeGridFactory extends FlowFactory, TreeGridFactory> implements
HasHierarchicalDataProviderFactory, TreeGridFactory> {
public TreeGridFactory() {
this(new TreeGrid<>());
}
public TreeGridFactory(Class beanType) {
this(new TreeGrid<>(beanType));
}
public TreeGridFactory(HierarchicalDataProvider dataProvider) {
this(new TreeGrid<>(dataProvider));
}
public TreeGridFactory(TreeGrid tTreeGrid) {
super(tTreeGrid);
}
@SafeVarargs
public final TreeGridFactory expand(T... expand) {
get().expand(expand);
return this;
}
public TreeGridFactory expand(Collection expand) {
get().expand(expand);
return this;
}
public TreeGridFactory columns(String hierarchyPropertyName, ValueProvider valueProvider, Collection propertyNames) {
get().setColumns(hierarchyPropertyName, valueProvider, propertyNames);
return this;
}
public TreeGridFactory hierarchyColumn(String propertyName, ValueProvider valueProvider) {
get().setHierarchyColumn(propertyName, valueProvider);
return this;
}
public TreeGridFactory hierarchyColumn(String hierarchyColumn) {
get().setHierarchyColumn(hierarchyColumn);
return this;
}
public TreeGridFactory expandRecursively(Stream items, int depth) {
get().expandRecursively(items, depth);
return this;
}
public TreeGridFactory expandRecursively(Collection items, int depth) {
get().expandRecursively(items, depth);
return this;
}
public TreeGridFactory collapse(Collection collapse) {
get().collapse(collapse);
return this;
}
@SafeVarargs
public final TreeGridFactory collapse(T... collapse) {
get().collapse(collapse);
return this;
}
public TreeGridFactory dataProvider(DataProvider dataProvider) {
get().setDataProvider(dataProvider);
return this;
}
public TreeGridFactory expandListener(ComponentEventListener>> expandListener) {
get().addExpandListener(expandListener);
return this;
}
public TreeGridFactory hierarchyColumn(ValueProvider hierarchyColumn) {
get().addHierarchyColumn(hierarchyColumn);
return this;
}
public TreeGridFactory uniqueKeyDataGenerator(String propertyName, ValueProvider uniqueKeyProvider) {
get().setUniqueKeyDataGenerator(propertyName, uniqueKeyProvider);
return this;
}
public TreeGridFactory collapseListener(ComponentEventListener>> collapseListener) {
get().addCollapseListener(collapseListener);
return this;
}
public TreeGridFactory componentHierarchyColumn(ValueProvider componentHierarchyColumn) {
get().addComponentHierarchyColumn(componentHierarchyColumn);
return this;
}
public TreeGridFactory collapseRecursively(Stream items, int depth) {
get().collapseRecursively(items, depth);
return this;
}
public TreeGridFactory collapseRecursively(Collection items, int depth) {
get().collapseRecursively(items, depth);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy