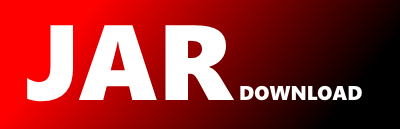
org.bklab.flow.textfield.AutoComplete Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fluent-vaadin-flow Show documentation
Show all versions of fluent-vaadin-flow Show documentation
Broderick Labs for fluent vaadin flow. Inherits common Vaadin components.
package org.bklab.flow.textfield;
import com.github.appreciated.ironoverlay.IronOverlay;
import com.github.appreciated.ironoverlay.IronOverlayBuilder;
import com.github.appreciated.ironoverlay.VerticalOrientation;
import com.vaadin.flow.component.AbstractField;
import com.vaadin.flow.component.html.Div;
import com.vaadin.flow.component.listbox.ListBox;
import com.vaadin.flow.component.textfield.TextField;
import com.vaadin.flow.data.value.ValueChangeMode;
import org.bklab.flow.factory.ListBoxFactory;
import org.bklab.flow.factory.TextFieldFactory;
import java.util.Collection;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Function;
public class AutoComplete extends Div {
private final IronOverlay ironOverlay;
private final TextField textField;
private final ListBox listBox = new ListBoxFactory().lumoSmall().widthFull().get();
Function> suggestGenerator;
private Function itemLabelGenerator = Objects::toString;
private T value;
{
this.ironOverlay = IronOverlayBuilder.get().with(listBox)
.withVerticalAlign(VerticalOrientation.TOP).build();
}
public AutoComplete() {
this(new TextFieldFactory().lumoSmall().widthFull().get());
}
public AutoComplete(TextField textField) {
this.textField = textField;
}
public AutoComplete build() {
add(textField, ironOverlay);
listBox.addValueChangeListener(this::handleSelectChanged);
textField.addValueChangeListener(e -> {
items(suggestGenerator.apply(
Optional.ofNullable(e.getValue()).orElse("")
));
ironOverlay.open();
});
textField.setValueChangeMode(ValueChangeMode.ON_CHANGE);
textField.setValueChangeTimeout(500);
return this;
}
public AutoComplete suggestGenerator(Function> suggestGenerator) {
this.suggestGenerator = suggestGenerator;
return this;
}
private void handleSelectChanged(AbstractField.ComponentValueChangeEvent, T> event) {
T value = event.getValue();
if (value == null) {
textField.clear();
return;
}
textField.setValue(itemLabelGenerator.apply(value));
ironOverlay.close();
this.value = value;
}
@SafeVarargs
public final AutoComplete items(T... items) {
this.listBox.setItems(items);
return this;
}
public AutoComplete items(Collection items) {
this.listBox.setItems(items);
return this;
}
public AutoComplete value(T value) {
this.value = value;
if (value == null) {
textField.clear();
return this;
}
textField.setValue(itemLabelGenerator.apply(value));
return this;
}
public T getValue() {
return value;
}
public AutoComplete itemLabelGenerator(Function itemLabelGenerator) {
this.itemLabelGenerator = itemLabelGenerator;
return this;
}
public IronOverlay getIronOverlay() {
return ironOverlay;
}
public TextField getTextField() {
return textField;
}
public ListBox getListBox() {
return listBox;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy