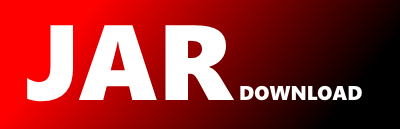
org.blackdread.camerabinding.jna.EdsdkLibrary Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of camera-binding Show documentation
Show all versions of camera-binding Show documentation
Bindings of C header files in java of EDSDK
package org.blackdread.camerabinding.jna;
import com.sun.jna.Native;
import com.sun.jna.NativeLong;
import com.sun.jna.Pointer;
import com.sun.jna.PointerType;
import com.sun.jna.ptr.ByReference;
import com.sun.jna.ptr.IntByReference;
import com.sun.jna.ptr.LongByReference;
import com.sun.jna.ptr.NativeLongByReference;
import com.sun.jna.ptr.PointerByReference;
import com.sun.jna.ptr.ShortByReference;
import com.sun.jna.win32.StdCallLibrary;
import org.blackdread.camerabinding.annotation.ImplicitRetain;
import java.nio.ByteBuffer;
import java.nio.IntBuffer;
/**
* JNA Wrapper for library Edsdk
* This file was autogenerated by JNAerator,
* a tool written by Olivier Chafik that uses a few opensource projects..
* For help, please visit NativeLibs4Java , Rococoa, or JNA.
*/
public interface EdsdkLibrary extends StdCallLibrary {
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsDataType {
/**
* native declaration : sdk-header\EDSDKTypes.h:136
*/
public static final int kEdsDataType_Unknown = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:137
*/
public static final int kEdsDataType_Bool = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:138
*/
public static final int kEdsDataType_String = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:139
*/
public static final int kEdsDataType_Int8 = 3;
/**
* native declaration : sdk-header\EDSDKTypes.h:140
*/
public static final int kEdsDataType_UInt8 = 6;
/**
* native declaration : sdk-header\EDSDKTypes.h:141
*/
public static final int kEdsDataType_Int16 = 4;
/**
* native declaration : sdk-header\EDSDKTypes.h:142
*/
public static final int kEdsDataType_UInt16 = 7;
/**
* native declaration : sdk-header\EDSDKTypes.h:143
*/
public static final int kEdsDataType_Int32 = 8;
/**
* native declaration : sdk-header\EDSDKTypes.h:144
*/
public static final int kEdsDataType_UInt32 = 9;
/**
* native declaration : sdk-header\EDSDKTypes.h:145
*/
public static final int kEdsDataType_Int64 = 10;
/**
* native declaration : sdk-header\EDSDKTypes.h:146
*/
public static final int kEdsDataType_UInt64 = 11;
/**
* native declaration : sdk-header\EDSDKTypes.h:147
*/
public static final int kEdsDataType_Float = 12;
/**
* native declaration : sdk-header\EDSDKTypes.h:148
*/
public static final int kEdsDataType_Double = 13;
/**
* native declaration : sdk-header\EDSDKTypes.h:149
*/
public static final int kEdsDataType_ByteBlock = 14;
/**
* native declaration : sdk-header\EDSDKTypes.h:150
*/
public static final int kEdsDataType_Rational = 20;
/**
* native declaration : sdk-header\EDSDKTypes.h:151
*/
public static final int kEdsDataType_Point = 21;
/**
* native declaration : sdk-header\EDSDKTypes.h:152
*/
public static final int kEdsDataType_Rect = 22;
/**
* native declaration : sdk-header\EDSDKTypes.h:153
*/
public static final int kEdsDataType_Time = 23;
/**
* native declaration : sdk-header\EDSDKTypes.h:155
*/
public static final int kEdsDataType_Bool_Array = 30;
/**
* native declaration : sdk-header\EDSDKTypes.h:156
*/
public static final int kEdsDataType_Int8_Array = 31;
/**
* native declaration : sdk-header\EDSDKTypes.h:157
*/
public static final int kEdsDataType_Int16_Array = 32;
/**
* native declaration : sdk-header\EDSDKTypes.h:158
*/
public static final int kEdsDataType_Int32_Array = 33;
/**
* native declaration : sdk-header\EDSDKTypes.h:159
*/
public static final int kEdsDataType_UInt8_Array = 34;
/**
* native declaration : sdk-header\EDSDKTypes.h:160
*/
public static final int kEdsDataType_UInt16_Array = 35;
/**
* native declaration : sdk-header\EDSDKTypes.h:161
*/
public static final int kEdsDataType_UInt32_Array = 36;
/**
* native declaration : sdk-header\EDSDKTypes.h:162
*/
public static final int kEdsDataType_Rational_Array = 37;
/**
* native declaration : sdk-header\EDSDKTypes.h:164
*/
public static final int kEdsDataType_FocusInfo = 101;
/**
* native declaration : sdk-header\EDSDKTypes.h:165
*/
public static final int kEdsDataType_PictureStyleDesc = 102;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsEvfAf {
/**
* native declaration : sdk-header\EDSDKTypes.h:333
*/
public static final int kEdsCameraCommand_EvfAf_OFF = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:334
*/
public static final int kEdsCameraCommand_EvfAf_ON = 1;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsShutterButton {
/**
* native declaration : sdk-header\EDSDKTypes.h:339
*/
public static final int kEdsCameraCommand_ShutterButton_OFF = 0x00000000;
/**
* native declaration : sdk-header\EDSDKTypes.h:340
*/
public static final int kEdsCameraCommand_ShutterButton_Halfway = 0x00000001;
/**
* native declaration : sdk-header\EDSDKTypes.h:341
*/
public static final int kEdsCameraCommand_ShutterButton_Completely = 0x00000003;
/**
* native declaration : sdk-header\EDSDKTypes.h:342
*/
public static final int kEdsCameraCommand_ShutterButton_Halfway_NonAF = 0x00010001;
/**
* native declaration : sdk-header\EDSDKTypes.h:343
*/
public static final int kEdsCameraCommand_ShutterButton_Completely_NonAF = 0x00010003;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsEvfDriveLens {
/**
* native declaration : sdk-header\EDSDKTypes.h:542
*/
public static final int kEdsEvfDriveLens_Near1 = 0x00000001;
/**
* native declaration : sdk-header\EDSDKTypes.h:543
*/
public static final int kEdsEvfDriveLens_Near2 = 0x00000002;
/**
* native declaration : sdk-header\EDSDKTypes.h:544
*/
public static final int kEdsEvfDriveLens_Near3 = 0x00000003;
/**
* native declaration : sdk-header\EDSDKTypes.h:545
*/
public static final int kEdsEvfDriveLens_Far1 = 0x00008001;
/**
* native declaration : sdk-header\EDSDKTypes.h:546
*/
public static final int kEdsEvfDriveLens_Far2 = 0x00008002;
/**
* native declaration : sdk-header\EDSDKTypes.h:547
*/
public static final int kEdsEvfDriveLens_Far3 = 0x00008003;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsEvfDepthOfFieldPreview {
/**
* native declaration : sdk-header\EDSDKTypes.h:556
*/
public static final int kEdsEvfDepthOfFieldPreview_OFF = 0x00000000;
/**
* native declaration : sdk-header\EDSDKTypes.h:557
*/
public static final int kEdsEvfDepthOfFieldPreview_ON = 0x00000001;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsSeekOrigin {
/**
* native declaration : sdk-header\EDSDKTypes.h:566
*/
public static final int kEdsSeek_Cur = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:567
*/
public static final int kEdsSeek_Begin = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:568
*/
public static final int kEdsSeek_End = 2;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsAccess {
/**
* native declaration : sdk-header\EDSDKTypes.h:577
*/
public static final int kEdsAccess_Read = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:578
*/
public static final int kEdsAccess_Write = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:579
*/
public static final int kEdsAccess_ReadWrite = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:580
*/
public static final int kEdsAccess_Error = -1;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsFileCreateDisposition {
/**
* native declaration : sdk-header\EDSDKTypes.h:589
*/
public static final int kEdsFileCreateDisposition_CreateNew = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:590
*/
public static final int kEdsFileCreateDisposition_CreateAlways = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:591
*/
public static final int kEdsFileCreateDisposition_OpenExisting = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:592
*/
public static final int kEdsFileCreateDisposition_OpenAlways = 3;
/**
* native declaration : sdk-header\EDSDKTypes.h:593
*/
public static final int kEdsFileCreateDisposition_TruncateExsisting = 4;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsImageType {
/**
* native declaration : sdk-header\EDSDKTypes.h:604
*/
public static final int kEdsImageType_Unknown = 0x00000000;
/**
* native declaration : sdk-header\EDSDKTypes.h:605
*/
public static final int kEdsImageType_Jpeg = 0x00000001;
/**
* native declaration : sdk-header\EDSDKTypes.h:606
*/
public static final int kEdsImageType_CRW = 0x00000002;
/**
* native declaration : sdk-header\EDSDKTypes.h:607
*/
public static final int kEdsImageType_RAW = 0x00000004;
/**
* native declaration : sdk-header\EDSDKTypes.h:608
*/
public static final int kEdsImageType_CR2 = 0x00000006;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsImageSize {
/**
* native declaration : sdk-header\EDSDKTypes.h:617
*/
public static final int kEdsImageSize_Large = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:618
*/
public static final int kEdsImageSize_Middle = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:619
*/
public static final int kEdsImageSize_Small = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:620
*/
public static final int kEdsImageSize_Middle1 = 5;
/**
* native declaration : sdk-header\EDSDKTypes.h:621
*/
public static final int kEdsImageSize_Middle2 = 6;
/**
* native declaration : sdk-header\EDSDKTypes.h:622
*/
public static final int kEdsImageSize_Small1 = 14;
/**
* native declaration : sdk-header\EDSDKTypes.h:623
*/
public static final int kEdsImageSize_Small2 = 15;
/**
* native declaration : sdk-header\EDSDKTypes.h:624
*/
public static final int kEdsImageSize_Small3 = 16;
/**
* native declaration : sdk-header\EDSDKTypes.h:625
*/
public static final int kEdsImageSize_Unknown = -1;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsCompressQuality {
/**
* native declaration : sdk-header\EDSDKTypes.h:634
*/
public static final int kEdsCompressQuality_Normal = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:635
*/
public static final int kEdsCompressQuality_Fine = 3;
/**
* native declaration : sdk-header\EDSDKTypes.h:636
*/
public static final int kEdsCompressQuality_Lossless = 4;
/**
* native declaration : sdk-header\EDSDKTypes.h:637
*/
public static final int kEdsCompressQuality_SuperFine = 5;
/**
* native declaration : sdk-header\EDSDKTypes.h:638
*/
public static final int kEdsCompressQuality_Unknown = -1;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsImageQuality {
/**
* Jpeg Large
* native declaration : sdk-header\EDSDKTypes.h:649
*/
public static final int EdsImageQuality_LJ = 0x0010ff0f;
/**
* Jpeg Middle1
* native declaration : sdk-header\EDSDKTypes.h:650
*/
public static final int EdsImageQuality_M1J = 0x0510ff0f;
/**
* Jpeg Middle2
* native declaration : sdk-header\EDSDKTypes.h:651
*/
public static final int EdsImageQuality_M2J = 0x0610ff0f;
/**
* Jpeg Small
* native declaration : sdk-header\EDSDKTypes.h:652
*/
public static final int EdsImageQuality_SJ = 0x0210ff0f;
/**
* Jpeg Large Fine
* native declaration : sdk-header\EDSDKTypes.h:653
*/
public static final int EdsImageQuality_LJF = 0x0013ff0f;
/**
* Jpeg Large Normal
* native declaration : sdk-header\EDSDKTypes.h:654
*/
public static final int EdsImageQuality_LJN = 0x0012ff0f;
/**
* Jpeg Middle Fine
* native declaration : sdk-header\EDSDKTypes.h:655
*/
public static final int EdsImageQuality_MJF = 0x0113ff0f;
/**
* Jpeg Middle Normal
* native declaration : sdk-header\EDSDKTypes.h:656
*/
public static final int EdsImageQuality_MJN = 0x0112ff0f;
/**
* Jpeg Small Fine
* native declaration : sdk-header\EDSDKTypes.h:657
*/
public static final int EdsImageQuality_SJF = 0x0213ff0f;
/**
* Jpeg Small Normal
* native declaration : sdk-header\EDSDKTypes.h:658
*/
public static final int EdsImageQuality_SJN = 0x0212ff0f;
/**
* Jpeg Small1 Fine
* native declaration : sdk-header\EDSDKTypes.h:659
*/
public static final int EdsImageQuality_S1JF = 0x0E13ff0f;
/**
* Jpeg Small1 Normal
* native declaration : sdk-header\EDSDKTypes.h:660
*/
public static final int EdsImageQuality_S1JN = 0x0E12ff0f;
/**
* Jpeg Small2
* native declaration : sdk-header\EDSDKTypes.h:661
*/
public static final int EdsImageQuality_S2JF = 0x0F13ff0f;
/**
* Jpeg Small3
* native declaration : sdk-header\EDSDKTypes.h:662
*/
public static final int EdsImageQuality_S3JF = 0x1013ff0f;
/**
* RAW
* native declaration : sdk-header\EDSDKTypes.h:665
*/
public static final int EdsImageQuality_LR = 0x0064ff0f;
/**
* RAW + Jpeg Large Fine
* native declaration : sdk-header\EDSDKTypes.h:666
*/
public static final int EdsImageQuality_LRLJF = 0x00640013;
/**
* RAW + Jpeg Large Normal
* native declaration : sdk-header\EDSDKTypes.h:667
*/
public static final int EdsImageQuality_LRLJN = 0x00640012;
/**
* RAW + Jpeg Middle Fine
* native declaration : sdk-header\EDSDKTypes.h:668
*/
public static final int EdsImageQuality_LRMJF = 0x00640113;
/**
* RAW + Jpeg Middle Normal
* native declaration : sdk-header\EDSDKTypes.h:669
*/
public static final int EdsImageQuality_LRMJN = 0x00640112;
/**
* RAW + Jpeg Small Fine
* native declaration : sdk-header\EDSDKTypes.h:670
*/
public static final int EdsImageQuality_LRSJF = 0x00640213;
/**
* RAW + Jpeg Small Normal
* native declaration : sdk-header\EDSDKTypes.h:671
*/
public static final int EdsImageQuality_LRSJN = 0x00640212;
/**
* RAW + Jpeg Small1 Fine
* native declaration : sdk-header\EDSDKTypes.h:672
*/
public static final int EdsImageQuality_LRS1JF = 0x00640E13;
/**
* RAW + Jpeg Small1 Normal
* native declaration : sdk-header\EDSDKTypes.h:673
*/
public static final int EdsImageQuality_LRS1JN = 0x00640E12;
/**
* RAW + Jpeg Small2
* native declaration : sdk-header\EDSDKTypes.h:674
*/
public static final int EdsImageQuality_LRS2JF = 0x00640F13;
/**
* RAW + Jpeg Small3
* native declaration : sdk-header\EDSDKTypes.h:675
*/
public static final int EdsImageQuality_LRS3JF = 0x00641013;
/**
* RAW + Jpeg Large
* native declaration : sdk-header\EDSDKTypes.h:677
*/
public static final int EdsImageQuality_LRLJ = 0x00640010;
/**
* RAW + Jpeg Middle1
* native declaration : sdk-header\EDSDKTypes.h:678
*/
public static final int EdsImageQuality_LRM1J = 0x00640510;
/**
* RAW + Jpeg Middle2
* native declaration : sdk-header\EDSDKTypes.h:679
*/
public static final int EdsImageQuality_LRM2J = 0x00640610;
/**
* RAW + Jpeg Small
* native declaration : sdk-header\EDSDKTypes.h:680
*/
public static final int EdsImageQuality_LRSJ = 0x00640210;
/**
* MRAW(SRAW1)
* native declaration : sdk-header\EDSDKTypes.h:683
*/
public static final int EdsImageQuality_MR = 0x0164ff0f;
/**
* MRAW(SRAW1) + Jpeg Large Fine
* native declaration : sdk-header\EDSDKTypes.h:684
*/
public static final int EdsImageQuality_MRLJF = 0x01640013;
/**
* MRAW(SRAW1) + Jpeg Large Normal
* native declaration : sdk-header\EDSDKTypes.h:685
*/
public static final int EdsImageQuality_MRLJN = 0x01640012;
/**
* MRAW(SRAW1) + Jpeg Middle Fine
* native declaration : sdk-header\EDSDKTypes.h:686
*/
public static final int EdsImageQuality_MRMJF = 0x01640113;
/**
* MRAW(SRAW1) + Jpeg Middle Normal
* native declaration : sdk-header\EDSDKTypes.h:687
*/
public static final int EdsImageQuality_MRMJN = 0x01640112;
/**
* MRAW(SRAW1) + Jpeg Small Fine
* native declaration : sdk-header\EDSDKTypes.h:688
*/
public static final int EdsImageQuality_MRSJF = 0x01640213;
/**
* MRAW(SRAW1) + Jpeg Small Normal
* native declaration : sdk-header\EDSDKTypes.h:689
*/
public static final int EdsImageQuality_MRSJN = 0x01640212;
/**
* MRAW(SRAW1) + Jpeg Small1 Fine
* native declaration : sdk-header\EDSDKTypes.h:690
*/
public static final int EdsImageQuality_MRS1JF = 0x01640E13;
/**
* MRAW(SRAW1) + Jpeg Small1 Normal
* native declaration : sdk-header\EDSDKTypes.h:691
*/
public static final int EdsImageQuality_MRS1JN = 0x01640E12;
/**
* MRAW(SRAW1) + Jpeg Small2
* native declaration : sdk-header\EDSDKTypes.h:692
*/
public static final int EdsImageQuality_MRS2JF = 0x01640F13;
/**
* MRAW(SRAW1) + Jpeg Small3
* native declaration : sdk-header\EDSDKTypes.h:693
*/
public static final int EdsImageQuality_MRS3JF = 0x01641013;
/**
* MRAW(SRAW1) + Jpeg Large
* native declaration : sdk-header\EDSDKTypes.h:695
*/
public static final int EdsImageQuality_MRLJ = 0x01640010;
/**
* MRAW(SRAW1) + Jpeg Middle1
* native declaration : sdk-header\EDSDKTypes.h:696
*/
public static final int EdsImageQuality_MRM1J = 0x01640510;
/**
* MRAW(SRAW1) + Jpeg Middle2
* native declaration : sdk-header\EDSDKTypes.h:697
*/
public static final int EdsImageQuality_MRM2J = 0x01640610;
/**
* MRAW(SRAW1) + Jpeg Small
* native declaration : sdk-header\EDSDKTypes.h:698
*/
public static final int EdsImageQuality_MRSJ = 0x01640210;
/**
* SRAW(SRAW2)
* native declaration : sdk-header\EDSDKTypes.h:701
*/
public static final int EdsImageQuality_SR = 0x0264ff0f;
/**
* SRAW(SRAW2) + Jpeg Large Fine
* native declaration : sdk-header\EDSDKTypes.h:702
*/
public static final int EdsImageQuality_SRLJF = 0x02640013;
/**
* SRAW(SRAW2) + Jpeg Large Normal
* native declaration : sdk-header\EDSDKTypes.h:703
*/
public static final int EdsImageQuality_SRLJN = 0x02640012;
/**
* SRAW(SRAW2) + Jpeg Middle Fine
* native declaration : sdk-header\EDSDKTypes.h:704
*/
public static final int EdsImageQuality_SRMJF = 0x02640113;
/**
* SRAW(SRAW2) + Jpeg Middle Normal
* native declaration : sdk-header\EDSDKTypes.h:705
*/
public static final int EdsImageQuality_SRMJN = 0x02640112;
/**
* SRAW(SRAW2) + Jpeg Small Fine
* native declaration : sdk-header\EDSDKTypes.h:706
*/
public static final int EdsImageQuality_SRSJF = 0x02640213;
/**
* SRAW(SRAW2) + Jpeg Small Normal
* native declaration : sdk-header\EDSDKTypes.h:707
*/
public static final int EdsImageQuality_SRSJN = 0x02640212;
/**
* SRAW(SRAW2) + Jpeg Small1 Fine
* native declaration : sdk-header\EDSDKTypes.h:708
*/
public static final int EdsImageQuality_SRS1JF = 0x02640E13;
/**
* SRAW(SRAW2) + Jpeg Small1 Normal
* native declaration : sdk-header\EDSDKTypes.h:709
*/
public static final int EdsImageQuality_SRS1JN = 0x02640E12;
/**
* SRAW(SRAW2) + Jpeg Small2
* native declaration : sdk-header\EDSDKTypes.h:710
*/
public static final int EdsImageQuality_SRS2JF = 0x02640F13;
/**
* SRAW(SRAW2) + Jpeg Small3
* native declaration : sdk-header\EDSDKTypes.h:711
*/
public static final int EdsImageQuality_SRS3JF = 0x02641013;
/**
* SRAW(SRAW2) + Jpeg Large
* native declaration : sdk-header\EDSDKTypes.h:713
*/
public static final int EdsImageQuality_SRLJ = 0x02640010;
/**
* SRAW(SRAW2) + Jpeg Middle1
* native declaration : sdk-header\EDSDKTypes.h:714
*/
public static final int EdsImageQuality_SRM1J = 0x02640510;
/**
* SRAW(SRAW2) + Jpeg Middle2
* native declaration : sdk-header\EDSDKTypes.h:715
*/
public static final int EdsImageQuality_SRM2J = 0x02640610;
/**
* SRAW(SRAW2) + Jpeg Small
* native declaration : sdk-header\EDSDKTypes.h:716
*/
public static final int EdsImageQuality_SRSJ = 0x02640210;
/**
* CRAW
* native declaration : sdk-header\EDSDKTypes.h:719
*/
public static final int EdsImageQuality_CR = 0x0063ff0f;
/**
* CRAW + Jpeg Large Fine
* native declaration : sdk-header\EDSDKTypes.h:720
*/
public static final int EdsImageQuality_CRLJF = 0x00630013;
/**
* CRAW + Jpeg Middle Fine
* native declaration : sdk-header\EDSDKTypes.h:721
*/
public static final int EdsImageQuality_CRMJF = 0x00630113;
/**
* CRAW + Jpeg Middle1 Fine
* native declaration : sdk-header\EDSDKTypes.h:722
*/
public static final int EdsImageQuality_CRM1JF = 0x00630513;
/**
* CRAW + Jpeg Middle2 Fine
* native declaration : sdk-header\EDSDKTypes.h:723
*/
public static final int EdsImageQuality_CRM2JF = 0x00630613;
/**
* CRAW + Jpeg Small Fine
* native declaration : sdk-header\EDSDKTypes.h:724
*/
public static final int EdsImageQuality_CRSJF = 0x00630213;
/**
* CRAW + Jpeg Small1 Fine
* native declaration : sdk-header\EDSDKTypes.h:725
*/
public static final int EdsImageQuality_CRS1JF = 0x00630E13;
/**
* CRAW + Jpeg Small2 Fine
* native declaration : sdk-header\EDSDKTypes.h:726
*/
public static final int EdsImageQuality_CRS2JF = 0x00630F13;
/**
* CRAW + Jpeg Small3 Fine
* native declaration : sdk-header\EDSDKTypes.h:727
*/
public static final int EdsImageQuality_CRS3JF = 0x00631013;
/**
* CRAW + Jpeg Large Normal
* native declaration : sdk-header\EDSDKTypes.h:728
*/
public static final int EdsImageQuality_CRLJN = 0x00630012;
/**
* CRAW + Jpeg Middle Normal
* native declaration : sdk-header\EDSDKTypes.h:729
*/
public static final int EdsImageQuality_CRMJN = 0x00630112;
/**
* CRAW + Jpeg Middle1 Normal
* native declaration : sdk-header\EDSDKTypes.h:730
*/
public static final int EdsImageQuality_CRM1JN = 0x00630512;
/**
* CRAW + Jpeg Middle2 Normal
* native declaration : sdk-header\EDSDKTypes.h:731
*/
public static final int EdsImageQuality_CRM2JN = 0x00630612;
/**
* CRAW + Jpeg Small Normal
* native declaration : sdk-header\EDSDKTypes.h:732
*/
public static final int EdsImageQuality_CRSJN = 0x00630212;
/**
* CRAW + Jpeg Small1 Normal
* native declaration : sdk-header\EDSDKTypes.h:733
*/
public static final int EdsImageQuality_CRS1JN = 0x00630E12;
/**
* CRAW + Jpeg Large
* native declaration : sdk-header\EDSDKTypes.h:735
*/
public static final int EdsImageQuality_CRLJ = 0x00630010;
/**
* CRAW + Jpeg Middle1
* native declaration : sdk-header\EDSDKTypes.h:736
*/
public static final int EdsImageQuality_CRM1J = 0x00630510;
/**
* CRAW + Jpeg Middle2
* native declaration : sdk-header\EDSDKTypes.h:737
*/
public static final int EdsImageQuality_CRM2J = 0x00630610;
/**
* CRAW + Jpeg Small
* native declaration : sdk-header\EDSDKTypes.h:738
*/
public static final int EdsImageQuality_CRSJ = 0x00630210;
/**
* native declaration : sdk-header\EDSDKTypes.h:740
*/
public static final int EdsImageQuality_Unknown = -1;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsImageQualityForLegacy {
/**
* Jpeg Large
* native declaration : sdk-header\EDSDKTypes.h:745
*/
public static final int kEdsImageQualityForLegacy_LJ = 0x001f000f;
/**
* Jpeg Middle1
* native declaration : sdk-header\EDSDKTypes.h:746
*/
public static final int kEdsImageQualityForLegacy_M1J = 0x051f000f;
/**
* Jpeg Middle2
* native declaration : sdk-header\EDSDKTypes.h:747
*/
public static final int kEdsImageQualityForLegacy_M2J = 0x061f000f;
/**
* Jpeg Small
* native declaration : sdk-header\EDSDKTypes.h:748
*/
public static final int kEdsImageQualityForLegacy_SJ = 0x021f000f;
/**
* Jpeg Large Fine
* native declaration : sdk-header\EDSDKTypes.h:749
*/
public static final int kEdsImageQualityForLegacy_LJF = 0x00130000;
/**
* Jpeg Large Normal
* native declaration : sdk-header\EDSDKTypes.h:750
*/
public static final int kEdsImageQualityForLegacy_LJN = 0x00120000;
/**
* Jpeg Middle Fine
* native declaration : sdk-header\EDSDKTypes.h:751
*/
public static final int kEdsImageQualityForLegacy_MJF = 0x01130000;
/**
* Jpeg Middle Normal
* native declaration : sdk-header\EDSDKTypes.h:752
*/
public static final int kEdsImageQualityForLegacy_MJN = 0x01120000;
/**
* Jpeg Small Fine
* native declaration : sdk-header\EDSDKTypes.h:753
*/
public static final int kEdsImageQualityForLegacy_SJF = 0x02130000;
/**
* Jpeg Small Normal
* native declaration : sdk-header\EDSDKTypes.h:754
*/
public static final int kEdsImageQualityForLegacy_SJN = 0x02120000;
/**
* RAW
* native declaration : sdk-header\EDSDKTypes.h:756
*/
public static final int kEdsImageQualityForLegacy_LR = 0x00240000;
/**
* RAW + Jpeg Large Fine
* native declaration : sdk-header\EDSDKTypes.h:757
*/
public static final int kEdsImageQualityForLegacy_LRLJF = 0x00240013;
/**
* RAW + Jpeg Large Normal
* native declaration : sdk-header\EDSDKTypes.h:758
*/
public static final int kEdsImageQualityForLegacy_LRLJN = 0x00240012;
/**
* RAW + Jpeg Middle Fine
* native declaration : sdk-header\EDSDKTypes.h:759
*/
public static final int kEdsImageQualityForLegacy_LRMJF = 0x00240113;
/**
* RAW + Jpeg Middle Normal
* native declaration : sdk-header\EDSDKTypes.h:760
*/
public static final int kEdsImageQualityForLegacy_LRMJN = 0x00240112;
/**
* RAW + Jpeg Small Fine
* native declaration : sdk-header\EDSDKTypes.h:761
*/
public static final int kEdsImageQualityForLegacy_LRSJF = 0x00240213;
/**
* RAW + Jpeg Small Normal
* native declaration : sdk-header\EDSDKTypes.h:762
*/
public static final int kEdsImageQualityForLegacy_LRSJN = 0x00240212;
/**
* RAW
* native declaration : sdk-header\EDSDKTypes.h:764
*/
public static final int kEdsImageQualityForLegacy_LR2 = 0x002f000f;
/**
* RAW + Jpeg Large
* native declaration : sdk-header\EDSDKTypes.h:765
*/
public static final int kEdsImageQualityForLegacy_LR2LJ = 0x002f001f;
/**
* RAW + Jpeg Middle1
* native declaration : sdk-header\EDSDKTypes.h:766
*/
public static final int kEdsImageQualityForLegacy_LR2M1J = 0x002f051f;
/**
* RAW + Jpeg Middle2
* native declaration : sdk-header\EDSDKTypes.h:767
*/
public static final int kEdsImageQualityForLegacy_LR2M2J = 0x002f061f;
/**
* RAW + Jpeg Small
* native declaration : sdk-header\EDSDKTypes.h:768
*/
public static final int kEdsImageQualityForLegacy_LR2SJ = 0x002f021f;
/**
* native declaration : sdk-header\EDSDKTypes.h:770
*/
public static final int kEdsImageQualityForLegacy_Unknown = -1;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsImageSource {
/**
* native declaration : sdk-header\EDSDKTypes.h:779
*/
public static final int kEdsImageSrc_FullView = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:780
*/
public static final int kEdsImageSrc_Thumbnail = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:781
*/
public static final int kEdsImageSrc_Preview = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:782
*/
public static final int kEdsImageSrc_RAWThumbnail = 3;
/**
* native declaration : sdk-header\EDSDKTypes.h:783
*/
public static final int kEdsImageSrc_RAWFullView = 4;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsTargetImageType {
/**
* native declaration : sdk-header\EDSDKTypes.h:793
*/
public static final int kEdsTargetImageType_Unknown = 0x00000000;
/**
* native declaration : sdk-header\EDSDKTypes.h:794
*/
public static final int kEdsTargetImageType_Jpeg = 0x00000001;
/**
* native declaration : sdk-header\EDSDKTypes.h:795
*/
public static final int kEdsTargetImageType_TIFF = 0x00000007;
/**
* native declaration : sdk-header\EDSDKTypes.h:796
*/
public static final int kEdsTargetImageType_TIFF16 = 0x00000008;
/**
* native declaration : sdk-header\EDSDKTypes.h:797
*/
public static final int kEdsTargetImageType_RGB = 0x00000009;
/**
* native declaration : sdk-header\EDSDKTypes.h:798
*/
public static final int kEdsTargetImageType_RGB16 = 0x0000000A;
/**
* native declaration : sdk-header\EDSDKTypes.h:799
*/
public static final int kEdsTargetImageType_DIB = 0x0000000B;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsProgressOption {
/**
* native declaration : sdk-header\EDSDKTypes.h:808
*/
public static final int kEdsProgressOption_NoReport = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:809
*/
public static final int kEdsProgressOption_Done = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:810
*/
public static final int kEdsProgressOption_Periodically = 2;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsFileAttributes {
/**
* native declaration : sdk-header\EDSDKTypes.h:820
*/
public static final int kEdsFileAttribute_Normal = 0x00000000;
/**
* native declaration : sdk-header\EDSDKTypes.h:821
*/
public static final int kEdsFileAttribute_ReadOnly = 0x00000001;
/**
* native declaration : sdk-header\EDSDKTypes.h:822
*/
public static final int kEdsFileAttribute_Hidden = 0x00000002;
/**
* native declaration : sdk-header\EDSDKTypes.h:823
*/
public static final int kEdsFileAttribute_System = 0x00000004;
/**
* native declaration : sdk-header\EDSDKTypes.h:824
*/
public static final int kEdsFileAttribute_Archive = 0x00000020;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsBatteryLevel2 {
/**
* native declaration : sdk-header\EDSDKTypes.h:834
*/
public static final int kEdsBatteryLevel2_Empty = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:835
*/
public static final int kEdsBatteryLevel2_Low = 9;
/**
* native declaration : sdk-header\EDSDKTypes.h:836
*/
public static final int kEdsBatteryLevel2_Half = 49;
/**
* native declaration : sdk-header\EDSDKTypes.h:837
*/
public static final int kEdsBatteryLevel2_Normal = 80;
/**
* native declaration : sdk-header\EDSDKTypes.h:838
*/
public static final int kEdsBatteryLevel2_Hi = 69;
/**
* native declaration : sdk-header\EDSDKTypes.h:839
*/
public static final int kEdsBatteryLevel2_Quarter = 19;
/**
* native declaration : sdk-header\EDSDKTypes.h:840
*/
public static final int kEdsBatteryLevel2_Error = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:841
*/
public static final int kEdsBatteryLevel2_BCLevel = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:842
*/
public static final int kEdsBatteryLevel2_AC = -1;
/**
* native declaration : sdk-header\EDSDKTypes.h:843
*/
public static final int kEdsBatteryLevel2_Unknown = 0xFFFFFFFE;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsSaveTo {
/**
* native declaration : sdk-header\EDSDKTypes.h:851
*/
public static final int kEdsSaveTo_Camera = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:852
*/
public static final int kEdsSaveTo_Host = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:853
*/
public static final int kEdsSaveTo_Both = (int) EdsSaveTo.kEdsSaveTo_Camera | (int) EdsSaveTo.kEdsSaveTo_Host;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsStorageType {
/**
* native declaration : sdk-header\EDSDKTypes.h:862
*/
public static final int kEdsStorageType_Non = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:863
*/
public static final int kEdsStorageType_CF = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:864
*/
public static final int kEdsStorageType_SD = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:865
*/
public static final int kEdsStorageType_HD = 4;
/**
* native declaration : sdk-header\EDSDKTypes.h:866
*/
public static final int kEdsStorageType_CFast = 5;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsWhiteBalance {
/**
* native declaration : sdk-header\EDSDKTypes.h:875
*/
public static final int kEdsWhiteBalance_Auto = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:876
*/
public static final int kEdsWhiteBalance_Daylight = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:877
*/
public static final int kEdsWhiteBalance_Cloudy = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:878
*/
public static final int kEdsWhiteBalance_Tangsten = 3;
/**
* native declaration : sdk-header\EDSDKTypes.h:879
*/
public static final int kEdsWhiteBalance_Fluorescent = 4;
/**
* native declaration : sdk-header\EDSDKTypes.h:880
*/
public static final int kEdsWhiteBalance_Strobe = 5;
/**
* native declaration : sdk-header\EDSDKTypes.h:881
*/
public static final int kEdsWhiteBalance_WhitePaper = 6;
/**
* native declaration : sdk-header\EDSDKTypes.h:882
*/
public static final int kEdsWhiteBalance_Shade = 8;
/**
* native declaration : sdk-header\EDSDKTypes.h:883
*/
public static final int kEdsWhiteBalance_ColorTemp = 9;
/**
* native declaration : sdk-header\EDSDKTypes.h:884
*/
public static final int kEdsWhiteBalance_PCSet1 = 10;
/**
* native declaration : sdk-header\EDSDKTypes.h:885
*/
public static final int kEdsWhiteBalance_PCSet2 = 11;
/**
* native declaration : sdk-header\EDSDKTypes.h:886
*/
public static final int kEdsWhiteBalance_PCSet3 = 12;
/**
* native declaration : sdk-header\EDSDKTypes.h:887
*/
public static final int kEdsWhiteBalance_WhitePaper2 = 15;
/**
* native declaration : sdk-header\EDSDKTypes.h:888
*/
public static final int kEdsWhiteBalance_WhitePaper3 = 16;
/**
* native declaration : sdk-header\EDSDKTypes.h:889
*/
public static final int kEdsWhiteBalance_WhitePaper4 = 18;
/**
* native declaration : sdk-header\EDSDKTypes.h:890
*/
public static final int kEdsWhiteBalance_WhitePaper5 = 19;
/**
* native declaration : sdk-header\EDSDKTypes.h:891
*/
public static final int kEdsWhiteBalance_PCSet4 = 20;
/**
* native declaration : sdk-header\EDSDKTypes.h:892
*/
public static final int kEdsWhiteBalance_PCSet5 = 21;
/**
* native declaration : sdk-header\EDSDKTypes.h:893
*/
public static final int kEdsWhiteBalance_AwbWhite = 23;
/**
* native declaration : sdk-header\EDSDKTypes.h:894
*/
public static final int kEdsWhiteBalance_Click = -1;
/**
* native declaration : sdk-header\EDSDKTypes.h:895
*/
public static final int kEdsWhiteBalance_Pasted = -2;
/**
* @deprecated got it from my camera... do not use. Put it here for now to let tests pass
*/
public static final int kEdsWhiteBalance_UnknownSelf = 32768;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsPhotoEffect {
/**
* native declaration : sdk-header\EDSDKTypes.h:904
*/
public static final int kEdsPhotoEffect_Off = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:905
*/
public static final int kEdsPhotoEffect_Monochrome = 5;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsColorMatrix {
/**
* native declaration : sdk-header\EDSDKTypes.h:914
*/
public static final int kEdsColorMatrix_Custom = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:915
*/
public static final int kEdsColorMatrix_1 = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:916
*/
public static final int kEdsColorMatrix_2 = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:917
*/
public static final int kEdsColorMatrix_3 = 3;
/**
* native declaration : sdk-header\EDSDKTypes.h:918
*/
public static final int kEdsColorMatrix_4 = 4;
/**
* native declaration : sdk-header\EDSDKTypes.h:919
*/
public static final int kEdsColorMatrix_5 = 5;
/**
* native declaration : sdk-header\EDSDKTypes.h:920
*/
public static final int kEdsColorMatrix_6 = 6;
/**
* native declaration : sdk-header\EDSDKTypes.h:921
*/
public static final int kEdsColorMatrix_7 = 7;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsFilterEffect {
/**
* native declaration : sdk-header\EDSDKTypes.h:930
*/
public static final int kEdsFilterEffect_None = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:931
*/
public static final int kEdsFilterEffect_Yellow = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:932
*/
public static final int kEdsFilterEffect_Orange = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:933
*/
public static final int kEdsFilterEffect_Red = 3;
/**
* native declaration : sdk-header\EDSDKTypes.h:934
*/
public static final int kEdsFilterEffect_Green = 4;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsTonigEffect {
/**
* native declaration : sdk-header\EDSDKTypes.h:943
*/
public static final int kEdsTonigEffect_None = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:944
*/
public static final int kEdsTonigEffect_Sepia = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:945
*/
public static final int kEdsTonigEffect_Blue = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:946
*/
public static final int kEdsTonigEffect_Purple = 3;
/**
* native declaration : sdk-header\EDSDKTypes.h:947
*/
public static final int kEdsTonigEffect_Green = 4;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsColorSpace {
/**
* native declaration : sdk-header\EDSDKTypes.h:956
*/
public static final int kEdsColorSpace_sRGB = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:957
*/
public static final int kEdsColorSpace_AdobeRGB = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:958
*/
public static final int kEdsColorSpace_Unknown = -1;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsPictureStyle {
/**
* native declaration : sdk-header\EDSDKTypes.h:967
*/
public static final int kEdsPictureStyle_Standard = 0x0081;
/**
* native declaration : sdk-header\EDSDKTypes.h:968
*/
public static final int kEdsPictureStyle_Portrait = 0x0082;
/**
* native declaration : sdk-header\EDSDKTypes.h:969
*/
public static final int kEdsPictureStyle_Landscape = 0x0083;
/**
* native declaration : sdk-header\EDSDKTypes.h:970
*/
public static final int kEdsPictureStyle_Neutral = 0x0084;
/**
* native declaration : sdk-header\EDSDKTypes.h:971
*/
public static final int kEdsPictureStyle_Faithful = 0x0085;
/**
* native declaration : sdk-header\EDSDKTypes.h:972
*/
public static final int kEdsPictureStyle_Monochrome = 0x0086;
/**
* native declaration : sdk-header\EDSDKTypes.h:973
*/
public static final int kEdsPictureStyle_Auto = 0x0087;
/**
* native declaration : sdk-header\EDSDKTypes.h:974
*/
public static final int kEdsPictureStyle_FineDetail = 0x0088;
/**
* native declaration : sdk-header\EDSDKTypes.h:975
*/
public static final int kEdsPictureStyle_User1 = 0x0021;
/**
* native declaration : sdk-header\EDSDKTypes.h:976
*/
public static final int kEdsPictureStyle_User2 = 0x0022;
/**
* native declaration : sdk-header\EDSDKTypes.h:977
*/
public static final int kEdsPictureStyle_User3 = 0x0023;
/**
* native declaration : sdk-header\EDSDKTypes.h:978
*/
public static final int kEdsPictureStyle_PC1 = 0x0041;
/**
* native declaration : sdk-header\EDSDKTypes.h:979
*/
public static final int kEdsPictureStyle_PC2 = 0x0042;
/**
* native declaration : sdk-header\EDSDKTypes.h:980
*/
public static final int kEdsPictureStyle_PC3 = 0x0043;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsTransferOption {
/**
* native declaration : sdk-header\EDSDKTypes.h:989
*/
public static final int kEdsTransferOption_ByDirectTransfer = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:990
*/
public static final int kEdsTransferOption_ByRelease = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:991
*/
public static final int kEdsTransferOption_ToDesktop = 0x00000100;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsAEMode {
/**
* native declaration : sdk-header\EDSDKTypes.h:1000
*/
public static final int kEdsAEMode_Program = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:1001
*/
public static final int kEdsAEMode_Tv = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:1002
*/
public static final int kEdsAEMode_Av = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:1003
*/
public static final int kEdsAEMode_Manual = 3;
/**
* native declaration : sdk-header\EDSDKTypes.h:1004
*/
public static final int kEdsAEMode_Bulb = 4;
/**
* native declaration : sdk-header\EDSDKTypes.h:1005
*/
public static final int kEdsAEMode_A_DEP = 5;
/**
* native declaration : sdk-header\EDSDKTypes.h:1006
*/
public static final int kEdsAEMode_DEP = 6;
/**
* native declaration : sdk-header\EDSDKTypes.h:1007
*/
public static final int kEdsAEMode_Custom = 7;
/**
* native declaration : sdk-header\EDSDKTypes.h:1008
*/
public static final int kEdsAEMode_Lock = 8;
/**
* native declaration : sdk-header\EDSDKTypes.h:1009
*/
public static final int kEdsAEMode_Green = 9;
/**
* native declaration : sdk-header\EDSDKTypes.h:1010
*/
public static final int kEdsAEMode_NightPortrait = 10;
/**
* native declaration : sdk-header\EDSDKTypes.h:1011
*/
public static final int kEdsAEMode_Sports = 11;
/**
* native declaration : sdk-header\EDSDKTypes.h:1012
*/
public static final int kEdsAEMode_Portrait = 12;
/**
* native declaration : sdk-header\EDSDKTypes.h:1013
*/
public static final int kEdsAEMode_Landscape = 13;
/**
* native declaration : sdk-header\EDSDKTypes.h:1014
*/
public static final int kEdsAEMode_Closeup = 14;
/**
* native declaration : sdk-header\EDSDKTypes.h:1015
*/
public static final int kEdsAEMode_FlashOff = 15;
/**
* native declaration : sdk-header\EDSDKTypes.h:1016
*/
public static final int kEdsAEMode_CreativeAuto = 19;
/**
* native declaration : sdk-header\EDSDKTypes.h:1017
*/
public static final int kEdsAEMode_Movie = 20;
/**
* native declaration : sdk-header\EDSDKTypes.h:1018
*/
public static final int kEdsAEMode_PhotoInMovie = 21;
/**
* native declaration : sdk-header\EDSDKTypes.h:1019
*/
public static final int kEdsAEMode_SceneIntelligentAuto = 22;
/**
* native declaration : sdk-header\EDSDKTypes.h:1020
*/
public static final int kEdsAEMode_SCN = 25;
/**
* native declaration : sdk-header\EDSDKTypes.h:1021
*/
public static final int kEdsAEMode_NightScenes = 23;
/**
* native declaration : sdk-header\EDSDKTypes.h:1022
*/
public static final int kEdsAEMode_BacklitScenes = 24;
/**
* native declaration : sdk-header\EDSDKTypes.h:1023
*/
public static final int kEdsAEMode_Children = 26;
/**
* native declaration : sdk-header\EDSDKTypes.h:1024
*/
public static final int kEdsAEMode_Food = 27;
/**
* native declaration : sdk-header\EDSDKTypes.h:1025
*/
public static final int kEdsAEMode_CandlelightPortraits = 28;
/**
* native declaration : sdk-header\EDSDKTypes.h:1026
*/
public static final int kEdsAEMode_CreativeFilter = 29;
/**
* native declaration : sdk-header\EDSDKTypes.h:1027
*/
public static final int kEdsAEMode_RoughMonoChrome = 30;
/**
* native declaration : sdk-header\EDSDKTypes.h:1028
*/
public static final int kEdsAEMode_SoftFocus = 31;
/**
* native declaration : sdk-header\EDSDKTypes.h:1029
*/
public static final int kEdsAEMode_ToyCamera = 32;
/**
* native declaration : sdk-header\EDSDKTypes.h:1030
*/
public static final int kEdsAEMode_Fisheye = 33;
/**
* native declaration : sdk-header\EDSDKTypes.h:1031
*/
public static final int kEdsAEMode_WaterColor = 34;
/**
* native declaration : sdk-header\EDSDKTypes.h:1032
*/
public static final int kEdsAEMode_Miniature = 35;
/**
* native declaration : sdk-header\EDSDKTypes.h:1033
*/
public static final int kEdsAEMode_Hdr_Standard = 36;
/**
* native declaration : sdk-header\EDSDKTypes.h:1034
*/
public static final int kEdsAEMode_Hdr_Vivid = 37;
/**
* native declaration : sdk-header\EDSDKTypes.h:1035
*/
public static final int kEdsAEMode_Hdr_Bold = 38;
/**
* native declaration : sdk-header\EDSDKTypes.h:1036
*/
public static final int kEdsAEMode_Hdr_Embossed = 39;
/**
* native declaration : sdk-header\EDSDKTypes.h:1037
*/
public static final int kEdsAEMode_Movie_Fantasy = 40;
/**
* native declaration : sdk-header\EDSDKTypes.h:1038
*/
public static final int kEdsAEMode_Movie_Old = 41;
/**
* native declaration : sdk-header\EDSDKTypes.h:1039
*/
public static final int kEdsAEMode_Movie_Memory = 42;
/**
* native declaration : sdk-header\EDSDKTypes.h:1040
*/
public static final int kEdsAEMode_Movie_DirectMono = 43;
/**
* native declaration : sdk-header\EDSDKTypes.h:1041
*/
public static final int kEdsAEMode_Movie_Mini = 44;
/**
* native declaration : sdk-header\EDSDKTypes.h:1042
*/
public static final int kEdsAEMode_PanningAssist = 45;
/**
* native declaration : sdk-header\EDSDKTypes.h:1043
*/
public static final int kEdsAEMode_GroupPhoto = 46;
/**
* native declaration : sdk-header\EDSDKTypes.h:1044
*/
public static final int kEdsAEMode_Myself = 50;
/**
* native declaration : sdk-header\EDSDKTypes.h:1045
*/
public static final int kEdsAEMode_SmoothSkin = 52;
/**
* native declaration : sdk-header\EDSDKTypes.h:1046
*/
public static final int kEdsAEMode_Flexible = 55;
/**
* native declaration : sdk-header\EDSDKTypes.h:1047
*/
public static final int kEdsAEMode_Unknown = -1;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsBracket {
/**
* native declaration : sdk-header\EDSDKTypes.h:1056
*/
public static final int kEdsBracket_AEB = 0x01;
/**
* native declaration : sdk-header\EDSDKTypes.h:1057
*/
public static final int kEdsBracket_ISOB = 0x02;
/**
* native declaration : sdk-header\EDSDKTypes.h:1058
*/
public static final int kEdsBracket_WBB = 0x04;
/**
* native declaration : sdk-header\EDSDKTypes.h:1059
*/
public static final int kEdsBracket_FEB = 0x08;
/**
* native declaration : sdk-header\EDSDKTypes.h:1060
*/
public static final int kEdsBracket_Unknown = -1;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsEvfOutputDevice {
/**
* native declaration : sdk-header\EDSDKTypes.h:1069
*/
public static final int kEdsEvfOutputDevice_TFT = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:1070
*/
public static final int kEdsEvfOutputDevice_PC = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:1071
*/
public static final int kEdsEvfOutputDevice_MOBILE = 4;
/**
* native declaration : sdk-header\EDSDKTypes.h:1072
*/
public static final int kEdsEvfOutputDevice_MOBILE2 = 8;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsEvfZoom {
/**
* native declaration : sdk-header\EDSDKTypes.h:1080
*/
public static final int kEdsEvfZoom_Fit = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:1081
*/
public static final int kEdsEvfZoom_x5 = 5;
/**
* native declaration : sdk-header\EDSDKTypes.h:1082
*/
public static final int kEdsEvfZoom_x10 = 10;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsEvfAFMode {
/**
* native declaration : sdk-header\EDSDKTypes.h:1090
*/
public static final int Evf_AFMode_Quick = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:1091
*/
public static final int Evf_AFMode_Live = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:1092
*/
public static final int Evf_AFMode_LiveFace = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:1093
*/
public static final int Evf_AFMode_LiveMulti = 3;
/**
* native declaration : sdk-header\EDSDKTypes.h:1094
*/
public static final int Evf_AFMode_LiveZone = 4;
/**
* native declaration : sdk-header\EDSDKTypes.h:1095
*/
public static final int Evf_AFMode_LiveSingleExpandCross = 5;
/**
* native declaration : sdk-header\EDSDKTypes.h:1096
*/
public static final int Evf_AFMode_LiveSingleExpandSurround = 6;
/**
* native declaration : sdk-header\EDSDKTypes.h:1097
*/
public static final int Evf_AFMode_LiveZoneLargeH = 7;
/**
* native declaration : sdk-header\EDSDKTypes.h:1098
*/
public static final int Evf_AFMode_LiveZoneLargeV = 8;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsStroboMode {
/**
* native declaration : sdk-header\EDSDKTypes.h:1100
*/
public static final int kEdsStroboModeInternal = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:1101
*/
public static final int kEdsStroboModeExternalETTL = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h:1102
*/
public static final int kEdsStroboModeExternalATTL = 2;
/**
* native declaration : sdk-header\EDSDKTypes.h:1103
*/
public static final int kEdsStroboModeExternalTTL = 3;
/**
* native declaration : sdk-header\EDSDKTypes.h:1104
*/
public static final int kEdsStroboModeExternalAuto = 4;
/**
* native declaration : sdk-header\EDSDKTypes.h:1105
*/
public static final int kEdsStroboModeExternalManual = 5;
/**
* native declaration : sdk-header\EDSDKTypes.h:1106
*/
public static final int kEdsStroboModeManual = 6;
}
/**
* native declaration : sdk-header\EDSDKTypes.h
* enum values
*/
public static interface EdsETTL2Mode {
/**
* native declaration : sdk-header\EDSDKTypes.h:1114
*/
public static final int kEdsETTL2ModeEvaluative = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h:1115
*/
public static final int kEdsETTL2ModeAverage = 1;
}
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ISSPECIFIC_MASK = 0x80000000;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_COMPONENTID_MASK = 0x7F000000;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_RESERVED_MASK = 0x00FF0000;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERRORID_MASK = 0x0000FFFF;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_CMP_ID_CLIENT_COMPONENTID = 0x01000000;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_CMP_ID_LLSDK_COMPONENTID = 0x02000000;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_CMP_ID_HLSDK_COMPONENTID = 0x03000000;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_OK = 0x00000000;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_UNIMPLEMENTED = 0x00000001;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INTERNAL_ERROR = 0x00000002;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_MEM_ALLOC_FAILED = 0x00000003;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_MEM_FREE_FAILED = 0x00000004;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_OPERATION_CANCELLED = 0x00000005;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INCOMPATIBLE_VERSION = 0x00000006;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_NOT_SUPPORTED = 0x00000007;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_UNEXPECTED_EXCEPTION = 0x00000008;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_PROTECTION_VIOLATION = 0x00000009;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_MISSING_SUBCOMPONENT = 0x0000000A;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_SELECTION_UNAVAILABLE = 0x0000000B;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_FILE_IO_ERROR = 0x00000020;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_FILE_TOO_MANY_OPEN = 0x00000021;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_FILE_NOT_FOUND = 0x00000022;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_FILE_OPEN_ERROR = 0x00000023;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_FILE_CLOSE_ERROR = 0x00000024;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_FILE_SEEK_ERROR = 0x00000025;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_FILE_TELL_ERROR = 0x00000026;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_FILE_READ_ERROR = 0x00000027;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_FILE_WRITE_ERROR = 0x00000028;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_FILE_PERMISSION_ERROR = 0x00000029;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_FILE_DISK_FULL_ERROR = 0x0000002A;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_FILE_ALREADY_EXISTS = 0x0000002B;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_FILE_FORMAT_UNRECOGNIZED = 0x0000002C;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_FILE_DATA_CORRUPT = 0x0000002D;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_FILE_NAMING_NA = 0x0000002E;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DIR_NOT_FOUND = 0x00000040;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DIR_IO_ERROR = 0x00000041;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DIR_ENTRY_NOT_FOUND = 0x00000042;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DIR_ENTRY_EXISTS = 0x00000043;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DIR_NOT_EMPTY = 0x00000044;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_PROPERTIES_UNAVAILABLE = 0x00000050;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_PROPERTIES_MISMATCH = 0x00000051;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_PROPERTIES_NOT_LOADED = 0x00000053;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INVALID_PARAMETER = 0x00000060;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INVALID_HANDLE = 0x00000061;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INVALID_POINTER = 0x00000062;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INVALID_INDEX = 0x00000063;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INVALID_LENGTH = 0x00000064;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INVALID_FN_POINTER = 0x00000065;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INVALID_SORT_FN = 0x00000066;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DEVICE_NOT_FOUND = 0x00000080;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DEVICE_BUSY = 0x00000081;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DEVICE_INVALID = 0x00000082;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DEVICE_EMERGENCY = 0x00000083;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DEVICE_MEMORY_FULL = 0x00000084;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DEVICE_INTERNAL_ERROR = 0x00000085;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DEVICE_INVALID_PARAMETER = 0x00000086;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DEVICE_NO_DISK = 0x00000087;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DEVICE_DISK_ERROR = 0x00000088;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DEVICE_CF_GATE_CHANGED = 0x00000089;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DEVICE_DIAL_CHANGED = 0x0000008A;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DEVICE_NOT_INSTALLED = 0x0000008B;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DEVICE_STAY_AWAKE = 0x0000008C;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DEVICE_NOT_RELEASED = 0x0000008D;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STREAM_IO_ERROR = 0x000000A0;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STREAM_NOT_OPEN = 0x000000A1;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STREAM_ALREADY_OPEN = 0x000000A2;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STREAM_OPEN_ERROR = 0x000000A3;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STREAM_CLOSE_ERROR = 0x000000A4;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STREAM_SEEK_ERROR = 0x000000A5;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STREAM_TELL_ERROR = 0x000000A6;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STREAM_READ_ERROR = 0x000000A7;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STREAM_WRITE_ERROR = 0x000000A8;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STREAM_PERMISSION_ERROR = 0x000000A9;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STREAM_COULDNT_BEGIN_THREAD = 0x000000AA;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STREAM_BAD_OPTIONS = 0x000000AB;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STREAM_END_OF_STREAM = 0x000000AC;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_COMM_PORT_IS_IN_USE = 0x000000C0;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_COMM_DISCONNECTED = 0x000000C1;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_COMM_DEVICE_INCOMPATIBLE = 0x000000C2;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_COMM_BUFFER_FULL = 0x000000C3;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_COMM_USB_BUS_ERR = 0x000000C4;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_USB_DEVICE_LOCK_ERROR = 0x000000D0;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_USB_DEVICE_UNLOCK_ERROR = 0x000000D1;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STI_UNKNOWN_ERROR = 0x000000E0;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STI_INTERNAL_ERROR = 0x000000E1;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STI_DEVICE_CREATE_ERROR = 0x000000E2;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_STI_DEVICE_RELEASE_ERROR = 0x000000E3;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DEVICE_NOT_LAUNCHED = 0x000000E4;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_ENUM_NA = 0x000000F0;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INVALID_FN_CALL = 0x000000F1;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_HANDLE_NOT_FOUND = 0x000000F2;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INVALID_ID = 0x000000F3;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_WAIT_TIMEOUT_ERROR = 0x000000F4;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_SESSION_NOT_OPEN = 0x00002003;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INVALID_TRANSACTIONID = 0x00002004;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INCOMPLETE_TRANSFER = 0x00002007;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INVALID_STRAGEID = 0x00002008;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_DEVICEPROP_NOT_SUPPORTED = 0x0000200A;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INVALID_OBJECTFORMATCODE = 0x0000200B;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_SELF_TEST_FAILED = 0x00002011;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_PARTIAL_DELETION = 0x00002012;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_SPECIFICATION_BY_FORMAT_UNSUPPORTED = 0x00002014;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_NO_VALID_OBJECTINFO = 0x00002015;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INVALID_CODE_FORMAT = 0x00002016;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_UNKNOWN_VENDOR_CODE = 0x00002017;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_CAPTURE_ALREADY_TERMINATED = 0x00002018;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_PTP_DEVICE_BUSY = 0x00002019;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INVALID_PARENTOBJECT = 0x0000201A;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INVALID_DEVICEPROP_FORMAT = 0x0000201B;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_INVALID_DEVICEPROP_VALUE = 0x0000201C;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_SESSION_ALREADY_OPEN = 0x0000201E;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_TRANSACTION_CANCELLED = 0x0000201F;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_SPECIFICATION_OF_DESTINATION_UNSUPPORTED = 0x00002020;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_NOT_CAMERA_SUPPORT_SDK_VERSION = 0x00002021;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_UNKNOWN_COMMAND = 0x0000A001;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_OPERATION_REFUSED = 0x0000A005;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_LENS_COVER_CLOSE = 0x0000A006;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_LOW_BATTERY = 0x0000A101;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_OBJECT_NOTREADY = 0x0000A102;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_CANNOT_MAKE_OBJECT = 0x0000A104;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_MEMORYSTATUS_NOTREADY = 0x0000A106;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_TAKE_PICTURE_AF_NG = 0x00008D01;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_TAKE_PICTURE_RESERVED = 0x00008D02;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_TAKE_PICTURE_MIRROR_UP_NG = 0x00008D03;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_TAKE_PICTURE_SENSOR_CLEANING_NG = 0x00008D04;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_TAKE_PICTURE_SILENCE_NG = 0x00008D05;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_TAKE_PICTURE_NO_CARD_NG = 0x00008D06;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_TAKE_PICTURE_CARD_NG = 0x00008D07;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_TAKE_PICTURE_CARD_PROTECT_NG = 0x00008D08;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_TAKE_PICTURE_MOVIE_CROP_NG = 0x00008D09;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_TAKE_PICTURE_STROBO_CHARGE_NG = 0x00008D0A;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_TAKE_PICTURE_NO_LENS_NG = 0x00008D0B;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_TAKE_PICTURE_SPECIAL_MOVIE_MODE_NG = 0x00008D0C;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_TAKE_PICTURE_LV_REL_PROHIBIT_MODE_NG = 0x00008D0D;
/**
* native declaration : sdk-header\EDSDKErrors.h
*/
public static final int EDS_ERR_LAST_GENERIC_ERROR_PLUS_ONE = 0x000000F5;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int EDS_MAX_NAME = 256;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int EDS_TRANSFER_BLOCK_SIZE = 512;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int NULL = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int FALSE = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int TRUE = 1;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Unknown = 0x0000ffff;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_ProductName = 0x00000002;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_OwnerName = 0x00000004;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_MakerName = 0x00000005;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_DateTime = 0x00000006;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_FirmwareVersion = 0x00000007;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_BatteryLevel = 0x00000008;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_CFn = 0x00000009;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_SaveTo = 0x0000000b;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_CurrentStorage = 0x0000000c;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_CurrentFolder = 0x0000000d;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_MyMenu = 0x0000000e;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_BatteryQuality = 0x00000010;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_BodyIDEx = 0x00000015;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_HDDirectoryStructure = 0x00000020;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_ImageQuality = 0x00000100;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_JpegQuality = 0x00000101;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Orientation = 0x00000102;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_ICCProfile = 0x00000103;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_FocusInfo = 0x00000104;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_DigitalExposure = 0x00000105;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_WhiteBalance = 0x00000106;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_ColorTemperature = 0x00000107;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_WhiteBalanceShift = 0x00000108;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Contrast = 0x00000109;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_ColorSaturation = 0x0000010a;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_ColorTone = 0x0000010b;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Sharpness = 0x0000010c;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_ColorSpace = 0x0000010d;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_ToneCurve = 0x0000010e;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_PhotoEffect = 0x0000010f;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_FilterEffect = 0x00000110;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_ToningEffect = 0x00000111;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_ParameterSet = 0x00000112;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_ColorMatrix = 0x00000113;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_PictureStyle = 0x00000114;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_PictureStyleDesc = 0x00000115;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_PictureStyleCaption = 0x00000200;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Linear = 0x00000300;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_ClickWBPoint = 0x00000301;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_WBCoeffs = 0x00000302;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_GPSVersionID = 0x00000800;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_GPSLatitudeRef = 0x00000801;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_GPSLatitude = 0x00000802;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_GPSLongitudeRef = 0x00000803;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_GPSLongitude = 0x00000804;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_GPSAltitudeRef = 0x00000805;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_GPSAltitude = 0x00000806;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_GPSTimeStamp = 0x00000807;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_GPSSatellites = 0x00000808;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_GPSStatus = 0x00000809;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_GPSMapDatum = 0x00000812;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_GPSDateStamp = 0x0000081D;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_AtCapture_Flag = 0x80000000;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_AEMode = 0x00000400;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_DriveMode = 0x00000401;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_ISOSpeed = 0x00000402;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_MeteringMode = 0x00000403;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_AFMode = 0x00000404;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Av = 0x00000405;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Tv = 0x00000406;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_ExposureCompensation = 0x00000407;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_FlashCompensation = 0x00000408;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_FocalLength = 0x00000409;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_AvailableShots = 0x0000040a;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Bracket = 0x0000040b;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_WhiteBalanceBracket = 0x0000040c;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_LensName = 0x0000040d;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_AEBracket = 0x0000040e;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_FEBracket = 0x0000040f;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_ISOBracket = 0x00000410;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_NoiseReduction = 0x00000411;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_FlashOn = 0x00000412;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_RedEye = 0x00000413;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_FlashMode = 0x00000414;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_LensStatus = 0x00000416;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Artist = 0x00000418;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Copyright = 0x00000419;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_DepthOfField = 0x0000041b;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_EFCompensation = 0x0000041e;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_AEModeSelect = 0x00000436;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_OutputDevice = 0x00000500;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_Mode = 0x00000501;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_WhiteBalance = 0x00000502;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_ColorTemperature = 0x00000503;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_DepthOfFieldPreview = 0x00000504;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_Zoom = 0x00000507;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_ZoomPosition = 0x00000508;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_FocusAid = 0x00000509;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_Histogram = 0x0000050A;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_ImagePosition = 0x0000050B;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_HistogramStatus = 0x0000050C;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_AFMode = 0x0000050E;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Record = 0x00000510;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_HistogramY = 0x00000515;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_HistogramR = 0x00000516;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_HistogramG = 0x00000517;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_HistogramB = 0x00000518;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_CoordinateSystem = 0x00000540;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_ZoomRect = 0x00000541;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropID_Evf_ImageClipRect = 0x00000545;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsCameraCommand_TakePicture = 0x00000000;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsCameraCommand_ExtendShutDownTimer = 0x00000001;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsCameraCommand_BulbStart = 0x00000002;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsCameraCommand_BulbEnd = 0x00000003;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsCameraCommand_DoEvfAf = 0x00000102;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsCameraCommand_DriveLensEvf = 0x00000103;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsCameraCommand_DoClickWBEvf = 0x00000104;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsCameraCommand_PressShutterButton = 0x00000004;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsCameraStatusCommand_UILock = 0x00000000;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsCameraStatusCommand_UIUnLock = 0x00000001;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsCameraStatusCommand_EnterDirectTransfer = 0x00000002;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsCameraStatusCommand_ExitDirectTransfer = 0x00000003;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropertyEvent_All = 0x00000100;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropertyEvent_PropertyChanged = 0x00000101;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsPropertyEvent_PropertyDescChanged = 0x00000102;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsObjectEvent_All = 0x00000200;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsObjectEvent_VolumeInfoChanged = 0x00000201;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsObjectEvent_VolumeUpdateItems = 0x00000202;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsObjectEvent_FolderUpdateItems = 0x00000203;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsObjectEvent_DirItemCreated = 0x00000204;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsObjectEvent_DirItemRemoved = 0x00000205;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsObjectEvent_DirItemInfoChanged = 0x00000206;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsObjectEvent_DirItemContentChanged = 0x00000207;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsObjectEvent_DirItemRequestTransfer = 0x00000208;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsObjectEvent_DirItemRequestTransferDT = 0x00000209;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsObjectEvent_DirItemCancelTransferDT = 0x0000020a;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsObjectEvent_VolumeAdded = 0x0000020c;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsObjectEvent_VolumeRemoved = 0x0000020d;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsStateEvent_All = 0x00000300;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsStateEvent_Shutdown = 0x00000301;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsStateEvent_JobStatusChanged = 0x00000302;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsStateEvent_WillSoonShutDown = 0x00000303;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsStateEvent_ShutDownTimerUpdate = 0x00000304;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsStateEvent_CaptureError = 0x00000305;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsStateEvent_InternalError = 0x00000306;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsStateEvent_AfResult = 0x00000309;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public static final int kEdsStateEvent_BulbExposureTime = 0x00000310;
/**
* native declaration : sdk-header\EDSDK.h
*/
public static final int oldif = 0;
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public interface EdsProgressCallback extends StdCallCallback {
NativeLong apply(NativeLong inPercent, Pointer inContext, IntByReference outCancel);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
// @Deprecated
// NativeLong apply(NativeLong inPercent, EdsVoid inContext, IntByReference outCancel);
}
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public interface EdsCameraAddedHandler extends StdCallCallback {
NativeLong apply(Pointer inContext);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
// @Deprecated
// NativeLong apply(EdsVoid inContext);
}
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public interface EdsPropertyEventHandler extends StdCallCallback {
NativeLong apply(NativeLong inEvent, NativeLong inPropertyID, NativeLong inParam, Pointer inContext);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
// @Deprecated
// NativeLong apply(NativeLong inEvent, NativeLong inPropertyID, NativeLong inParam, EdsVoid inContext);
}
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public interface EdsObjectEventHandler extends StdCallCallback {
NativeLong apply(NativeLong inEvent, EdsBaseRef inRef, Pointer inContext);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
// @Deprecated
// NativeLong apply(NativeLong inEvent, EdsBaseRef inRef, EdsVoid inContext);
// NativeLong apply(NativeLong inEvent, Pointer inRef, EdsVoid inContext);
}
/**
* native declaration : sdk-header\EDSDKTypes.h
*/
public interface EdsStateEventHandler extends StdCallCallback {
NativeLong apply(NativeLong inEvent, NativeLong inEventData, Pointer inContext);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
// @Deprecated
// NativeLong apply(NativeLong inEvent, NativeLong inEventData, EdsVoid inContext);
}
/**
* ----------------------------------------------------------------------------
* native declaration : sdk-header\EDSDKTypes.h:1368
*/
public interface EdsReadStream extends StdCallCallback {
NativeLong apply(Pointer inContext, NativeLong inReadSize, Pointer outBuffer, NativeLongByReference outReadSize);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
// @Deprecated
// NativeLong apply(Pointer inContext, NativeLong inReadSize, EdsVoid outBuffer, NativeLongByReference outReadSize);
}
/**
* native declaration : sdk-header\EDSDKTypes.h:1369
*/
public interface EdsWriteStream extends StdCallCallback {
NativeLong apply(Pointer inContext, NativeLong inWriteSize, Pointer inBuffer, NativeLongByReference outWrittenSize);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
// @Deprecated
// NativeLong apply(Pointer inContext, NativeLong inWriteSize, EdsVoid inBuffer, NativeLongByReference outWrittenSize);
}
/**
* native declaration : sdk-header\EDSDKTypes.h:1370
*/
public interface EdsSeekStream extends StdCallCallback {
NativeLong apply(Pointer inContext, NativeLong inSeekOffset, int inSeekOrigin);
}
/**
* native declaration : sdk-header\EDSDKTypes.h:1371
*/
public interface EdsTellStream extends StdCallCallback {
NativeLong apply(Pointer inContext, NativeLongByReference outPosition);
}
/**
* native declaration : sdk-header\EDSDKTypes.h:1372
*/
public interface EdsGetStreamLength extends StdCallCallback {
NativeLong apply(Pointer inContext, NativeLongByReference outLength);
}
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsInitializeSDK
* //
* // Description:
* // Initializes the libraries.
* // When using the EDSDK libraries, you must call this API once
* // before using EDSDK APIs.
* //
* // Parameters:
* // In: None
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsInitializeSDK()
* native declaration : sdk-header\EDSDK.h:63
*
* @return any of the sdk errors
*/
NativeLong EdsInitializeSDK();
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsTerminateSDK
* //
* // Description:
* // Terminates use of the libraries.
* // This function muse be called when ending the SDK.
* // Calling this function releases all resources allocated by the libraries.
* //
* // Parameters:
* // In: None
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsTerminateSDK()
* native declaration : sdk-header\EDSDK.h:80
*
* @return any of the sdk errors
*/
NativeLong EdsTerminateSDK();
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsRetain
* //
* // Description:
* // Increments the reference counter of existing objects.
* //
* // Parameters:
* // In: inRef - The reference for the item.
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsUInt32 EdsRetain(EdsBaseRef)
* native declaration : sdk-header\EDSDK.h:105
*
* @param inRef the reference for the item
* @return any of the sdk errors
*/
NativeLong EdsRetain(EdsBaseRef inRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsRelease
* //
* // Description:
* // Decrements the reference counter to an object.
* // When the reference counter reaches 0, the object is released.
* //
* // Parameters:
* // In: inRef - The reference of the item.
* // Out: None
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsUInt32 EdsRelease(EdsBaseRef)
* native declaration : sdk-header\EDSDK.h:121
*
* @param inRef the reference of the item
* @return any of the sdk errors
*/
NativeLong EdsRelease(EdsBaseRef inRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetChildCount
* //
* // Description:
* // Gets the number of child objects of the designated object.
* // Example: Number of files in a directory
* //
* // Parameters:
* // In: inRef - The reference of the list.
* // Out: outCount - Number of elements in this list.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetChildCount(EdsBaseRef, EdsUInt32*)
* native declaration : sdk-header\EDSDK.h:147
*
* @param inRef the reference of the list
* @param outCount number of elements in this list
* @return any of the sdk errors
*/
NativeLong EdsGetChildCount(EdsBaseRef inRef, NativeLongByReference outCount);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetChildAtIndex
* //
* // Description:
* // Gets an indexed child object of the designated object.
* //
* // Parameters:
* // In: inRef - The reference of the item.
* // inIndex - The index that is passed in, is zero based.
* // Out: outRef - The pointer which receives reference of the
* // specified index .
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetChildAtIndex(EdsBaseRef, EdsInt32, EdsBaseRef*)
* native declaration : sdk-header\EDSDK.h:166
*
* @param inRef the reference of the item
* @param inIndex the index that is passed in, is zero based
* @param outRef the pointer which receives reference of the specified index
* @return any of the sdk errors
*/
NativeLong EdsGetChildAtIndex(EdsBaseRef inRef, NativeLong inIndex, @ImplicitRetain EdsBaseRef.ByReference outRef);
// NativeLong EdsGetChildAtIndex(EdsBaseRef inRef, NativeLong inIndex, PointerByReference outRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetParent
* //
* // Description:
* // Gets the parent object of the designated object.
* //
* // Parameters:
* // In: inRef - The reference of the item.
* // Out: outParentRef - The pointer which receives reference.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetParent(EdsBaseRef, EdsBaseRef*)
* native declaration : sdk-header\EDSDK.h:184
*
* @param inRef the reference of the item
* @param outParentRef the pointer which receives reference
* @return any of the sdk errors
*/
NativeLong EdsGetParent(EdsBaseRef inRef, @ImplicitRetain EdsBaseRef.ByReference outParentRef);
// NativeLong EdsGetParent(EdsBaseRef inRef, PointerByReference outParentRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetPropertySize
* //
* // Description:
* // Gets the byte size and data type of a designated property
* // from a camera object or image object.
* //
* // Parameters:
* // In: inRef - The reference of the item.
* // inPropertyID - The ProprtyID
* // inParam - Additional information of property.
* // We use this parameter in order to specify an index
* // in case there are two or more values over the same ID.
* // Out: outDataType - Pointer to the buffer that is to receive the property
* // type data.
* // outSize - Pointer to the buffer that is to receive the property
* // size.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetPropertySize(EdsBaseRef, EdsPropertyID, EdsInt32, EdsDataType*, EdsUInt32*)
* native declaration : sdk-header\EDSDK.h:218
*
* @param inRef the reference of the item
* @param inPropertyID the ProprtyID
* @param inParam additional information of property
* @param outDataType pointer to the buffer that is to receive the property type data
* @param outSize pointer to the buffer that is to receive the property size
* @return any of the sdk errors
* @deprecated use the safer methods {@link #EdsGetPropertySize(EdsdkLibrary.EdsBaseRef, NativeLong, NativeLong, IntBuffer, NativeLongByReference)} and {@link #EdsGetPropertySize(EdsBaseRef, NativeLong, NativeLong, IntByReference, NativeLongByReference)} instead
*/
@Deprecated
NativeLong EdsGetPropertySize(EdsBaseRef inRef, NativeLong inPropertyID, NativeLong inParam, IntByReference outDataType, NativeLongByReference outSize);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetPropertySize
* //
* // Description:
* // Gets the byte size and data type of a designated property
* // from a camera object or image object.
* //
* // Parameters:
* // In: inRef - The reference of the item.
* // inPropertyID - The ProprtyID
* // inParam - Additional information of property.
* // We use this parameter in order to specify an index
* // in case there are two or more values over the same ID.
* // Out: outDataType - Pointer to the buffer that is to receive the property
* // type data.
* // outSize - Pointer to the buffer that is to receive the property
* // size.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetPropertySize(EdsBaseRef, EdsPropertyID, EdsInt32, EdsDataType*, EdsUInt32*)
* native declaration : sdk-header\EDSDK.h:218
*
* @param inRef the reference of the item
* @param inPropertyID the ProprtyID
* @param inParam additional information of property
* @param outDataType pointer to the buffer that is to receive the property type data
* @param outSize pointer to the buffer that is to receive the property size
* @return any of the sdk errors
*/
NativeLong EdsGetPropertySize(EdsBaseRef inRef, NativeLong inPropertyID, NativeLong inParam, IntBuffer outDataType, NativeLongByReference outSize);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetPropertyData
* //
* // Description:
* // Gets property information from the object designated in inRef.
* //
* // Parameters:
* // In: inRef - The reference of the item.
* // inPropertyID - The ProprtyID
* // inParam - Additional information of property.
* // We use this parameter in order to specify an index
* // in case there are two or more values over the same ID.
* // inPropertySize - The number of bytes of the prepared buffer
* // for receive property-value.
* // Out: outPropertyData - The buffer pointer to receive property-value.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetPropertyData(EdsBaseRef, EdsPropertyID, EdsInt32, EdsUInt32, EdsVoid*)
* native declaration : sdk-header\EDSDK.h:244
*
* @param inRef The reference of the item
* @param inPropertyID The ProprtyID
* @param inParam Additional information of property
* @param inPropertySize The number of bytes of the prepared buffer for receive property-value
* @param outPropertyData The buffer pointer to receive property-value
* @return any of the sdk errors
*/
NativeLong EdsGetPropertyData(EdsBaseRef inRef, NativeLong inPropertyID, NativeLong inParam, NativeLong inPropertySize, Pointer outPropertyData);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
@Deprecated
NativeLong EdsGetPropertyData(EdsBaseRef inRef, NativeLong inPropertyID, NativeLong inParam, NativeLong inPropertySize, EdsVoid outPropertyData);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsSetPropertyData
* //
* // Description:
* // Sets property data for the object designated in inRef.
* //
* // Parameters:
* // In: inRef - The reference of the item.
* // inPropertyID - The ProprtyID
* // inParam - Additional information of property.
* // inPropertySize - The number of bytes of the prepared buffer
* // for set property-value.
* // inPropertyData - The buffer pointer to set property-value.
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsSetPropertyData(EdsBaseRef, EdsPropertyID, EdsInt32, EdsUInt32, const EdsVoid*)
* native declaration : sdk-header\EDSDK.h:269
*
* @param inRef The reference of the item
* @param inPropertyID The ProprtyID
* @param inParam Additional information of property
* @param inPropertySize The number of bytes of the prepared buffer for set property-value
* @param inPropertyData The buffer pointer to set property-value
* @return any of the sdk errors
*/
NativeLong EdsSetPropertyData(EdsBaseRef inRef, NativeLong inPropertyID, NativeLong inParam, NativeLong inPropertySize, Pointer inPropertyData);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
@Deprecated
NativeLong EdsSetPropertyData(EdsBaseRef inRef, NativeLong inPropertyID, NativeLong inParam, NativeLong inPropertySize, EdsVoid inPropertyData);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetPropertyDesc
* //
* // Description:
* // Gets a list of property data that can be set for the object
* // designated in inRef, as well as maximum and minimum values.
* // This API is intended for only some shooting-related properties.
* //
* // Parameters:
* // In: inRef - The reference of the camera.
* // inPropertyID - The Property ID.
* // Out: outPropertyDesc - Array of the value which can be set up.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetPropertyDesc(EdsBaseRef, EdsPropertyID, EdsPropertyDesc*)
* native declaration : sdk-header\EDSDK.h:292
*
* @param inRef The reference of the camera
* @param inPropertyID The Property ID
* @param outPropertyDesc Array of the value which can be set up
* @return any of the sdk errors
*/
NativeLong EdsGetPropertyDesc(EdsBaseRef inRef, NativeLong inPropertyID, EdsPropertyDesc outPropertyDesc);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetCameraList
* //
* // Description:
* // Gets camera list objects.
* //
* // Parameters:
* // In: None
* // Out: outCameraListRef - Pointer to the camera-list.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetCameraList(EdsCameraListRef*)
* native declaration : sdk-header\EDSDK.h:319
*
* @param outCameraListRef Pointer to the camera-list
* @return any of the sdk errors
*/
NativeLong EdsGetCameraList(@ImplicitRetain EdsCameraListRef.ByReference outCameraListRef);
// NativeLong EdsGetCameraList(PointerByReference outCameraListRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetDeviceInfo
* //
* // Description:
* // Gets device information, such as the device name.
* // Because device information of remote cameras is stored
* // on the host computer, you can use this API
* // before the camera object initiates communication
* // (that is, before a session is opened).
* //
* // Parameters:
* // In: inCameraRef - The reference of the camera.
* // Out: outDeviceInfo - Information as device of camera.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetDeviceInfo(EdsCameraRef, EdsDeviceInfo*)
* native declaration : sdk-header\EDSDK.h:348
*
* @param inCameraRef The reference of the camera
* @param outDeviceInfo Information as device of camera
* @return any of the sdk errors
*/
NativeLong EdsGetDeviceInfo(EdsCameraRef inCameraRef, EdsDeviceInfo outDeviceInfo);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsOpenSession
* //
* // Description:
* // Establishes a logical connection with a remote camera.
* // Use this API after getting the camera's EdsCamera object.
* //
* // Parameters:
* // In: inCameraRef - The reference of the camera
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsOpenSession(EdsCameraRef)
* native declaration : sdk-header\EDSDK.h:366
*
* @param inCameraRef The reference of the camera
* @return any of the sdk errors
*/
NativeLong EdsOpenSession(EdsCameraRef inCameraRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsCloseSession
* //
* // Description:
* // Closes a logical connection with a remote camera.
* //
* // Parameters:
* // In: inCameraRef - The reference of the camera
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsCloseSession(EdsCameraRef)
* native declaration : sdk-header\EDSDK.h:382
*
* @param inCameraRef The reference of the camera
* @return any of the sdk errors
*/
NativeLong EdsCloseSession(EdsCameraRef inCameraRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsSendCommand
* //
* // Description:
* // Sends a command such as "Shoot" to a remote camera.
* //
* // Parameters:
* // In: inCameraRef - The reference of the camera which will receive the
* // command.
* // inCommand - Specifies the command to be sent.
* // inParam - Specifies additional command-specific information.
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsSendCommand(EdsCameraRef, EdsCameraCommand, EdsInt32)
* native declaration : sdk-header\EDSDK.h:401
*
* @param inCameraRef The reference of the camera which will receive the command
* @param inCommand Specifies the command to be sent
* @param inParam Specifies additional command-specific information
* @return any of the sdk errors
*/
NativeLong EdsSendCommand(EdsCameraRef inCameraRef, NativeLong inCommand, NativeLong inParam);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsSendStatusCommand
* //
* // Description:
* // Sets the remote camera state or mode.
* //
* // Parameters:
* // In: inCameraRef - The reference of the camera which will receive the
* // command.
* // inStatusCommand - Specifies the command to be sent.
* // inParam - Specifies additional command-specific information.
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsSendStatusCommand(EdsCameraRef, EdsCameraStatusCommand, EdsInt32)
* native declaration : sdk-header\EDSDK.h:422
*
* @param inCameraRef The reference of the camera which will receive the command
* @param inStatusCommand Specifies the command to be sent
* @param inParam Specifies additional command-specific information
* @return any of the sdk errors
*/
NativeLong EdsSendStatusCommand(EdsCameraRef inCameraRef, NativeLong inStatusCommand, NativeLong inParam);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsSetCapacity
* //
* // Description:
* // Sets the remaining HDD capacity on the host computer
* // (excluding the portion from image transfer),
* // as calculated by subtracting the portion from the previous time.
* // Set a reset flag initially and designate the cluster length
* // and number of free clusters.
* // Some type 2 protocol standard cameras can display the number of shots
* // left on the camera based on the available disk capacity
* // of the host computer.
* // For these cameras, after the storage destination is set to the computer,
* // use this API to notify the camera of the available disk capacity
* // of the host computer.
* //
* // Parameters:
* // In: inCameraRef - The reference of the camera which will receive the
* // command.
* // inCapacity - The remaining capacity of a transmission place.
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsSetCapacity(EdsCameraRef, EdsCapacity)
* native declaration : sdk-header\EDSDK.h:453
*
* @param inCameraRef The reference of the camera which will receive the command
* @param inCapacity The remaining capacity of a transmission place
* @return any of the sdk errors
*/
NativeLong EdsSetCapacity(EdsCameraRef inCameraRef, EdsCapacity.ByValue inCapacity);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetVolumeInfo
* //
* // Description:
* // Gets volume information for a memory card in the camera.
* //
* // Parameters:
* // In: inVolumeRef - The reference of the volume.
* // Out: outVolumeInfo - information of the volume.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetVolumeInfo(EdsVolumeRef, EdsVolumeInfo*)
* native declaration : sdk-header\EDSDK.h:479
*
* @param inVolumeRef The reference of the volume
* @param outVolumeInfo information of the volume
* @return any of the sdk errors
*/
NativeLong EdsGetVolumeInfo(EdsVolumeRef inVolumeRef, EdsVolumeInfo outVolumeInfo);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsFormatVolume
* //
* // Description:
* // Formats volumes of memory cards in a camera.
* //
* // Parameters:
* // In: inVolumeRef - The reference of volume .
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsFormatVolume(EdsVolumeRef)
* native declaration : sdk-header\EDSDK.h:495
*
* @param inVolumeRef The reference of volume
* @return any of the sdk errors
*/
NativeLong EdsFormatVolume(EdsVolumeRef inVolumeRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetDirectoryItemInfo
* //
* // Description:
* // Gets information about the directory or file objects
* // on the memory card (volume) in a remote camera.
* //
* // Parameters:
* // In: inDirItemRef - The reference of the directory item.
* // Out: outDirItemInfo - information of the directory item.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetDirectoryItemInfo(EdsDirectoryItemRef, EdsDirectoryItemInfo*)
* native declaration : sdk-header\EDSDK.h:521
*
* @param inDirItemRef The reference of the directory item
* @param outDirItemInfo information of the directory item
* @return any of the sdk errors
*/
NativeLong EdsGetDirectoryItemInfo(EdsDirectoryItemRef inDirItemRef, EdsDirectoryItemInfo outDirItemInfo);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsDeleteDirectoryItem
* //
* // Description:
* // Deletes a camera folder or file.
* // If folders with subdirectories are designated, all files are deleted
* // except protected files.
* // EdsDirectoryItem objects deleted by means of this API are implicitly
* // released by the EDSDK. Thus, there is no need to release them
* // by means of EdsRelease.
* //
* // Parameters:
* // In: inDirItemRef - The reference of the directory item.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsDeleteDirectoryItem(EdsDirectoryItemRef)
* native declaration : sdk-header\EDSDK.h:543
*
* @param inDirItemRef The reference of the directory item
* @return any of the sdk errors
*/
NativeLong EdsDeleteDirectoryItem(EdsDirectoryItemRef inDirItemRef);
/**
* TODO no need NativeLong for inReadSize (EdsUInt64)
* -----------------------------------------------------------------------------
* //
* // Function: EdsDownload
* //
* // Description:
* // Downloads a file on a remote camera
* // (in the camera memory or on a memory card) to the host computer.
* // The downloaded file is sent directly to a file stream created in advance.
* // When dividing the file being retrieved, call this API repeatedly.
* // Also in this case, make the data block size a multiple of 512 (bytes),
* // excluding the final block.
* //
* // Parameters:
* // In: inDirItemRef - The reference of the directory item.
* // inReadSize -
* //
* // Out: outStream - The reference of the stream.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsDownload(EdsDirectoryItemRef, EdsUInt64, EdsStreamRef)
* native declaration : sdk-header\EDSDK.h:566
*
* @param inDirItemRef The reference of the directory item
* @param inReadSize Size to read
* @param outStream The reference of the stream
* @return any of the sdk errors
*/
NativeLong EdsDownload(EdsDirectoryItemRef inDirItemRef, long inReadSize, EdsStreamRef outStream);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsDownloadCancel
* //
* // Description:
* // Must be executed when downloading of a directory item is canceled.
* // Calling this API makes the camera cancel file transmission.
* // It also releases resources.
* // This operation need not be executed when using EdsDownloadThumbnail.
* //
* // Parameters:
* // In: inDirItemRef - The reference of the directory item.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsDownloadCancel(EdsDirectoryItemRef)
* native declaration : sdk-header\EDSDK.h:586
*
* @param inDirItemRef The reference of the directory item
* @return any of the sdk errors
*/
NativeLong EdsDownloadCancel(EdsDirectoryItemRef inDirItemRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsDownloadComplete
* //
* // Description:
* // Must be called when downloading of directory items is complete.
* // Executing this API makes the camera
* // recognize that file transmission is complete.
* // This operation need not be executed when using EdsDownloadThumbnail.
* //
* // Parameters:
* // In: inDirItemRef - The reference of the directory item.
* //
* // Out: outStream - None.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsDownloadComplete(EdsDirectoryItemRef)
* native declaration : sdk-header\EDSDK.h:606
*
* @param inDirItemRef The reference of the directory item
* @return any of the sdk errors
*/
NativeLong EdsDownloadComplete(EdsDirectoryItemRef inDirItemRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsDownloadThumbnail
* //
* // Description:
* // Extracts and downloads thumbnail information from image files in a camera.
* // Thumbnail information in the camera's image files is downloaded
* // to the host computer.
* // Downloaded thumbnails are sent directly to a file stream created in advance.
* //
* // Parameters:
* // In: inDirItemRef - The reference of the directory item.
* //
* // Out: outStream - The reference of the stream.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsDownloadThumbnail(EdsDirectoryItemRef, EdsStreamRef)
* native declaration : sdk-header\EDSDK.h:626
*
* @param inDirItemRef The reference of the directory item
* @param outStream The reference of the stream
* @return any of the sdk errors
*/
NativeLong EdsDownloadThumbnail(EdsDirectoryItemRef inDirItemRef, EdsStreamRef outStream);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetAttribute
* //
* // Description:
* // Gets attributes of files on a camera.
* //
* // Parameters:
* // In: inDirItemRef - The reference of the directory item.
* // Out: outFileAttribute - Indicates the file attributes.
* // As for the file attributes, OR values of the value defined
* // by enum EdsFileAttributes can be retrieved. Thus, when
* // determining the file attributes, you must check
* // if an attribute flag is set for target attributes.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetAttribute(EdsDirectoryItemRef, EdsFileAttributes*)
* native declaration : sdk-header\EDSDK.h:647
*
* @param inDirItemRef The reference of the directory item
* @param outFileAttribute Indicates the file attributes
* @return any of the sdk errors
* @deprecated use the safer methods {@link #EdsGetAttribute(EdsdkLibrary.EdsDirectoryItemRef, IntBuffer)} and {@link #EdsGetAttribute(EdsDirectoryItemRef, IntByReference)} instead
*/
@Deprecated
NativeLong EdsGetAttribute(EdsDirectoryItemRef inDirItemRef, IntByReference outFileAttribute);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetAttribute
* //
* // Description:
* // Gets attributes of files on a camera.
* //
* // Parameters:
* // In: inDirItemRef - The reference of the directory item.
* // Out: outFileAttribute - Indicates the file attributes.
* // As for the file attributes, OR values of the value defined
* // by enum EdsFileAttributes can be retrieved. Thus, when
* // determining the file attributes, you must check
* // if an attribute flag is set for target attributes.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetAttribute(EdsDirectoryItemRef, EdsFileAttributes*)
* native declaration : sdk-header\EDSDK.h:647
*
* @param inDirItemRef The reference of the directory item
* @param outFileAttribute Indicates the file attributes
* @return any of the sdk errors
*/
NativeLong EdsGetAttribute(EdsDirectoryItemRef inDirItemRef, IntBuffer outFileAttribute);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsSetAttribute
* //
* // Description:
* // Changes attributes of files on a camera.
* //
* // Parameters:
* // In: inDirItemRef - The reference of the directory item.
* // inFileAttribute - Indicates the file attributes.
* // As for the file attributes, OR values of the value
* // defined by enum EdsFileAttributes can be retrieved.
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsSetAttribute(EdsDirectoryItemRef, EdsFileAttributes)
* native declaration : sdk-header\EDSDK.h:667
*
* @param inDirItemRef The reference of the directory item
* @param inFileAttribute Indicates the file attributes
* @return any of the sdk errors
*/
NativeLong EdsSetAttribute(EdsDirectoryItemRef inDirItemRef, int inFileAttribute);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsCreateFileStream
* //
* // Description:
* // Creates a new file on a host computer (or opens an existing file)
* // and creates a file stream for access to the file.
* // If a new file is designated before executing this API,
* // the file is actually created following the timing of writing
* // by means of EdsWrite or the like with respect to an open stream.
* //
* // Parameters:
* // In: inFileName - Pointer to a null-terminated string that specifies
* // the file name.
* // inCreateDisposition - Action to take on files that exist,
* // and which action to take when files do not exist.
* // inDesiredAccess - Access to the stream (reading, writing, or both).
* // Out: outStream - The reference of the stream.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsCreateFileStream(const EdsChar*, EdsFileCreateDisposition, EdsAccess, EdsStreamRef*)
* native declaration : sdk-header\EDSDK.h:702
*
* @param inFileName Pointer to a null-terminated string that specifies the file name
* @param inCreateDisposition Action to take on files that exist and which action to take when files do not exist
* @param inDesiredAccess Access to the stream (reading, writing, or both)
* @param outStream The reference of the stream
* @return any of the sdk errors
*/
NativeLong EdsCreateFileStream(ByteBuffer inFileName, int inCreateDisposition, int inDesiredAccess, EdsStreamRef.ByReference outStream);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsCreateFileStream
* //
* // Description:
* // Creates a new file on a host computer (or opens an existing file)
* // and creates a file stream for access to the file.
* // If a new file is designated before executing this API,
* // the file is actually created following the timing of writing
* // by means of EdsWrite or the like with respect to an open stream.
* //
* // Parameters:
* // In: inFileName - Pointer to a null-terminated string that specifies
* // the file name.
* // inCreateDisposition - Action to take on files that exist,
* // and which action to take when files do not exist.
* // inDesiredAccess - Access to the stream (reading, writing, or both).
* // Out: outStream - The reference of the stream.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsCreateFileStream(const EdsChar*, EdsFileCreateDisposition, EdsAccess, EdsStreamRef*)
* native declaration : sdk-header\EDSDK.h:702
*
* @param inFileName Pointer to a null-terminated string that specifies the file name
* @param inCreateDisposition Action to take on files that exist and which action to take when files do not exist
* @param inDesiredAccess Access to the stream (reading, writing, or both)
* @param outStream The reference of the stream
* @return any of the sdk errors
*/
NativeLong EdsCreateFileStream(byte inFileName[], int inCreateDisposition, int inDesiredAccess, EdsStreamRef.ByReference outStream);
/**
* TODO no need NativeLong for inBufferSize (EdsUInt64)
* -----------------------------------------------------------------------------
* //
* // Function: EdsCreateMemoryStream
* //
* // Description:
* // Creates a stream in the memory of a host computer.
* // In the case of writing in excess of the allocated buffer size,
* // the memory is automatically extended.
* //
* // Parameters:
* // In: inBufferSize - The number of bytes of the memory to allocate.
* // Out: outStream - The reference of the stream.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsCreateMemoryStream(EdsUInt64, EdsStreamRef*)
* native declaration : sdk-header\EDSDK.h:724
*
* @param inBufferSize The number of bytes of the memory to allocate
* @param outStream The reference of the stream
* @return any of the sdk errors
*/
NativeLong EdsCreateMemoryStream(long inBufferSize, EdsStreamRef.ByReference outStream);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsCreateStreamEx
* //
* // Description:
* // An extended version of EdsCreateStreamFromFile.
* // Use this function when working with Unicode file names.
* //
* // Parameters:
* // In: inURL (for Macintosh) - Designate CFURLRef.
* // inFileName (for Windows) - Designate the file name.
* // inCreateDisposition - Action to take on files that exist,
* // and which action to take when files do not exist.
* // inDesiredAccess - Access to the stream (reading, writing, or both).
* //
* // Out: outStream - The reference of the stream.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsCreateFileStreamEx(const WCHAR*, EdsFileCreateDisposition, EdsAccess, EdsStreamRef*)
* native declaration : sdk-header\EDSDK.h:748
*
* @param inFileName Designate the file name
* @param inCreateDisposition Action to take on files that exist and which action to take when files do not exist
* @param inDesiredAccess Access to the stream (reading, writing, or both)
* @param outStream The reference of the stream
* @return any of the sdk errors
*/
NativeLong EdsCreateFileStreamEx(short inFileName[], int inCreateDisposition, int inDesiredAccess, EdsStreamRef.ByReference outStream);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsCreateStreamEx
* //
* // Description:
* // An extended version of EdsCreateStreamFromFile.
* // Use this function when working with Unicode file names.
* //
* // Parameters:
* // In: inURL (for Macintosh) - Designate CFURLRef.
* // inFileName (for Windows) - Designate the file name.
* // inCreateDisposition - Action to take on files that exist,
* // and which action to take when files do not exist.
* // inDesiredAccess - Access to the stream (reading, writing, or both).
* //
* // Out: outStream - The reference of the stream.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsCreateFileStreamEx(const WCHAR*, EdsFileCreateDisposition, EdsAccess, EdsStreamRef*)
* native declaration : sdk-header\EDSDK.h:748
*
* @param inFileName Designate the file name
* @param inCreateDisposition Action to take on files that exist and which action to take when files do not exist
* @param inDesiredAccess Access to the stream (reading, writing, or both)
* @param outStream The reference of the stream
* @return any of the sdk errors
* @deprecated use safer method {@link #EdsCreateFileStreamEx(short[], int, int, EdsStreamRef.ByReference)}
*/
@Deprecated
NativeLong EdsCreateFileStreamEx(ShortByReference inFileName, int inCreateDisposition, int inDesiredAccess, EdsStreamRef.ByReference outStream);
/**
* TODO no need NativeLong for inBufferSize (EdsUInt64)
* -----------------------------------------------------------------------------
* //
* // Function: EdsCreateMemoryStreamFromPointer
* //
* // Description:
* // Creates a stream from the memory buffer you prepare.
* // Unlike the buffer size of streams created by means of EdsCreateMemoryStream,
* // the buffer size you prepare for streams created this way does not expand.
* //
* // Parameters:
* // In: inUserBuffer - Pointer to the buffer you have prepared.
* // Streams created by means of this API lead to this buffer.
* // inBufferSize - The number of bytes of the memory to allocate.
* // Out: outStream - The reference of the stream.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsCreateMemoryStreamFromPointer(EdsVoid*, EdsUInt64, EdsStreamRef*)
* native declaration : sdk-header\EDSDK.h:778
*
* @param inUserBuffer Pointer to the buffer you have prepared. Streams created by means of this API lead to this buffer
* @param inBufferSize The number of bytes of the memory to allocate
* @param outStream The reference of the stream
* @return any of the sdk errors
*/
NativeLong EdsCreateMemoryStreamFromPointer(Pointer inUserBuffer, long inBufferSize, EdsStreamRef.ByReference outStream);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
@Deprecated
NativeLong EdsCreateMemoryStreamFromPointer(EdsVoid inUserBuffer, long inBufferSize, EdsStreamRef.ByReference outStream);
// NativeLong EdsCreateMemoryStreamFromPointer(EdsVoid inUserBuffer, long inBufferSize, PointerByReference outStream);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetPointer
* //
* // Description:
* // Gets the pointer to the start address of memory managed by the memory stream.
* // As the EDSDK automatically resizes the buffer, the memory stream provides
* // you with the same access methods as for the file stream.
* // If access is attempted that is excessive with regard to the buffer size
* // for the stream, data before the required buffer size is allocated
* // is copied internally, and new writing occurs.
* // Thus, the buffer pointer might be switched on an unknown timing.
* // Caution in use is therefore advised.
* //
* // Parameters:
* // In: inStream - Designate the memory stream for the pointer to retrieve.
* // Out: outPointer - If successful, returns the pointer to the buffer
* // written in the memory stream.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetPointer(EdsStreamRef, EdsVoid**)
* native declaration : sdk-header\EDSDK.h:805
*
* @param inStream Designate the memory stream for the pointer to retrieve
* @param outPointer If successful, returns the pointer to the buffer written in the memory stream
* @return any of the sdk errors
*/
NativeLong EdsGetPointer(EdsStreamRef inStream, PointerByReference outPointer);
/**
* TODO no need NativeLong for inReadSize (EdsUInt64)
* TODO no need NativeLongByReference for outWrittenSize (EdsUInt64*)
* -----------------------------------------------------------------------------
* //
* // Function: EdsRead
* //
* // Description:
* // Reads data the size of inReadSize into the outBuffer buffer,
* // starting at the current read or write position of the stream.
* // The size of data actually read can be designated in outReadSize.
* //
* // Parameters:
* // In: inStreamRef - The reference of the stream or image.
* // inReadSize - The number of bytes to read.
* // Out: outBuffer - Pointer to the user-supplied buffer that is to receive
* // the data read from the stream.
* // outReadSize - The actually read number of bytes.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsRead(EdsStreamRef, EdsUInt64, EdsVoid*, EdsUInt64*)
* native declaration : sdk-header\EDSDK.h:828
*
* @param inStreamRef The reference of the stream or image
* @param inReadSize The number of bytes to read
* @param outBuffer Pointer to the user-supplied buffer that is to receive the data read from the stream
* @param outReadSize The actually read number of bytes
* @return any of the sdk errors
*/
NativeLong EdsRead(EdsStreamRef inStreamRef, long inReadSize, Pointer outBuffer, LongByReference outReadSize);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
@Deprecated
NativeLong EdsRead(EdsStreamRef inStreamRef, long inReadSize, EdsVoid outBuffer, LongByReference outReadSize);
// NativeLong EdsRead(EdsStreamRef inStreamRef, long inReadSize, EdsVoid outBuffer, NativeLongByReference outReadSize);
// NativeLong EdsRead(EdsStreamRef inStreamRef, long inReadSize, EdsVoid outBuffer, LongBuffer outReadSize);
/**
* TODO no need NativeLong for inWriteSize (EdsUInt64)
* TODO no need NativeLongByReference for outWrittenSize (EdsUInt64*)
* -----------------------------------------------------------------------------
* //
* // Function: EdsWrite
* //
* // Description:
* // Writes data of a designated buffer
* // to the current read or write position of the stream.
* //
* // Parameters:
* // In: inStreamRef - The reference of the stream or image.
* // inWriteSize - The number of bytes to write.
* // inBuffer - A pointer to the user-supplied buffer that contains
* // the data to be written to the stream.
* // Out: outWrittenSize - The actually written-in number of bytes.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsWrite(EdsStreamRef, EdsUInt64, const EdsVoid*, EdsUInt64*)
* native declaration : sdk-header\EDSDK.h:852
*
* @param inStreamRef The reference of the stream or image
* @param inWriteSize The number of bytes to write
* @param inBuffer A pointer to the user-supplied buffer that contains the data to be written to the stream
* @param outWrittenSize The actually written-in number of bytes
* @return any of the sdk errors
*/
NativeLong EdsWrite(EdsStreamRef inStreamRef, long inWriteSize, Pointer inBuffer, LongByReference outWrittenSize);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
@Deprecated
NativeLong EdsWrite(EdsStreamRef inStreamRef, long inWriteSize, EdsVoid inBuffer, LongByReference outWrittenSize);
// NativeLong EdsWrite(EdsStreamRef inStreamRef, long inWriteSize, EdsVoid inBuffer, NativeLongByReference outWrittenSize);
// NativeLong EdsWrite(EdsStreamRef inStreamRef, long inWriteSize, EdsVoid inBuffer, LongBuffer outWrittenSize);
/**
* TODO no need NativeLong for inSeekOffset (EdsInt64)
* -----------------------------------------------------------------------------
* //
* // Function: EdsSeek
* //
* // Description:
* // Moves the read or write position of the stream
* (that is, the file position indicator).
* //
* // Parameters:
* // In: inStreamRef - The reference of the stream or image.
* // inSeekOffset - Number of bytes to move the pointer.
* // inSeekOrigin - Pointer movement mode. Must be one of the following
* // values.
* // kEdsSeek_Cur Move the stream pointer inSeekOffset bytes
* // from the current position in the stream.
* // kEdsSeek_Begin Move the stream pointer inSeekOffset bytes
* // forward from the beginning of the stream.
* // kEdsSeek_End Move the stream pointer inSeekOffset bytes
* // from the end of the stream.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsSeek(EdsStreamRef, EdsInt64, EdsSeekOrigin)
* native declaration : sdk-header\EDSDK.h:881
*
* @param inStreamRef The reference of the stream or image
* @param inSeekOffset Number of bytes to move the pointer
* @param inSeekOrigin Pointer movement mode
* @return any of the sdk errors
*/
NativeLong EdsSeek(EdsStreamRef inStreamRef, long inSeekOffset, int inSeekOrigin);
/**
* TODO no need NativeLongByReference for outPosition (EdsUInt64*)
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetPosition
* //
* // Description:
* // Gets the current read or write position of the stream
* // (that is, the file position indicator).
* //
* // Parameters:
* // In: inStreamRef - The reference of the stream or image.
* // Out: outPosition - The current stream pointer.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetPosition(EdsStreamRef, EdsUInt64*)
* native declaration : sdk-header\EDSDK.h:901
*
* @param inStreamRef The reference of the stream or image
* @param outPosition The current stream pointer
* @return any of the sdk errors
*/
NativeLong EdsGetPosition(EdsStreamRef inStreamRef, LongByReference outPosition);
// NativeLong EdsGetPosition(EdsStreamRef inStreamRef, NativeLongByReference outPosition);
// NativeLong EdsGetPosition(EdsStreamRef inStreamRef, LongBuffer outPosition);
/**
* TODO no need NativeLongByReference for outLength (EdsUInt64*)
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetLength
* //
* // Description:
* // Gets the stream size.
* //
* // Parameters:
* // In: inStreamRef - The reference of the stream or image.
* // Out: outLength - The length of the stream.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetLength(EdsStreamRef, EdsUInt64*)
* native declaration : sdk-header\EDSDK.h:919
*
* @param inStreamRef The reference of the stream or image
* @param outLength The length of the stream
* @return any of the sdk errors
*/
NativeLong EdsGetLength(EdsStreamRef inStreamRef, LongByReference outLength);
// NativeLong EdsGetLength(EdsStreamRef inStreamRef, NativeLongByReference outLength);
// NativeLong EdsGetLength(EdsStreamRef inStreamRef, LongBuffer outLength);
/**
* TODO no need NativeLong for inWriteSize (EdsUInt64)
* -----------------------------------------------------------------------------
* //
* // Function: EdsCopyData
* //
* // Description:
* // Copies data from the copy source stream to the copy destination stream.
* // The read or write position of the data to copy is determined from
* // the current file read or write position of the respective stream.
* // After this API is executed, the read or write positions of the copy source
* // and copy destination streams are moved an amount corresponding to
* // inWriteSize in the positive direction.
* //
* // Parameters:
* // In: inStreamRef - The reference of the stream or image.
* // inWriteSize - The number of bytes to copy.
* // Out: outStreamRef - The reference of the stream or image.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsCopyData(EdsStreamRef, EdsUInt64, EdsStreamRef)
* native declaration : sdk-header\EDSDK.h:943
*
* @param inStreamRef The reference of the stream or image
* @param inWriteSize The number of bytes to copy
* @param outStreamRef The reference of the stream or image
* @return any of the sdk errors
*/
NativeLong EdsCopyData(EdsStreamRef inStreamRef, long inWriteSize, EdsStreamRef outStreamRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsSetProgressCallback
* //
* // Description:
* // Register a progress callback function.
* // An event is received as notification of progress during processing that
* // takes a relatively long time, such as downloading files from a
* // remote camera.
* // If you register the callback function, the EDSDK calls the callback
* // function during execution or on completion of the following APIs.
* // This timing can be used in updating on-screen progress bars, for example.
* //
* // Parameters:
* // In: inRef - The reference of the stream or image.
* // inProgressCallback - Pointer to a progress callback function.
* // inProgressOption - The option about progress is specified.
* // Must be one of the following values.
* // kEdsProgressOption_Done
* // When processing is completed,a callback function
* // is called only at once.
* // kEdsProgressOption_Periodically
* // A callback function is performed periodically.
* // inContext - Application information, passed in the argument
* // when the callback function is called. Any information
* // required for your program may be added.
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsSetProgressCallback(EdsBaseRef, EdsProgressCallback, EdsProgressOption, EdsVoid*)
* native declaration : sdk-header\EDSDK.h:979
*
* @param inRef The reference of the stream or image
* @param inProgressCallback Pointer to a progress callback function
* @param inProgressOption The option about progress is specified
* @param inContext Application information, passed in the argument when the callback function is called. Any information required for your program may be added
* @return any of the sdk errors
*/
NativeLong EdsSetProgressCallback(EdsBaseRef inRef, EdsProgressCallback inProgressCallback, int inProgressOption, Pointer inContext);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
@Deprecated
NativeLong EdsSetProgressCallback(EdsBaseRef inRef, EdsProgressCallback inProgressCallback, int inProgressOption, EdsVoid inContext);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsCreateImageRef
* //
* // Description:
* // Creates an image object from an image file.
* // Without modification, stream objects cannot be worked with as images.
* // Thus, when extracting images from image files,
* // you must use this API to create image objects.
* // The image object created this way can be used to get image information
* // (such as the height and width, number of color components, and
* // resolution), thumbnail image data, and the image data itself.
* //
* // Parameters:
* // In: inStreamRef - The reference of the stream.
* //
* // Out: outImageRef - The reference of the image.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsCreateImageRef(EdsStreamRef, EdsImageRef*)
* native declaration : sdk-header\EDSDK.h:1015
*
* @param inStreamRef The reference of the stream
* @param outImageRef The reference of the image
* @return any of the sdk errors
*/
NativeLong EdsCreateImageRef(EdsStreamRef inStreamRef, EdsImageRef.ByReference outImageRef);
// NativeLong EdsCreateImageRef(EdsStreamRef inStreamRef, PointerByReference outImageRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetImageInfo
* //
* // Description:
* // Gets image information from a designated image object.
* // Here, image information means the image width and height,
* // number of color components, resolution, and effective image area.
* //
* // Parameters:
* // In: inStreamRef - Designate the object for which to get image information.
* // inImageSource - Of the various image data items in the image file,
* // designate the type of image data representing the
* // information you want to get. Designate the image as
* // defined in Enum EdsImageSource.
* //
* // kEdsImageSrc_FullView
* // The image itself (a full-sized image)
* // kEdsImageSrc_Thumbnail
* // A thumbnail image
* // kEdsImageSrc_Preview
* // A preview image
* // kEdsImageSrc_RAWThumbnail
* // A RAW thumbnail image
* // kEdsImageSrc_RAWFullView
* // A RAW full-sized image
* // Out: outImageInfo - Stores the image data information designated
* // in inImageSource.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetImageInfo(EdsImageRef, EdsImageSource, EdsImageInfo*)
* native declaration : sdk-header\EDSDK.h:1050
*
* @param inImageRef Designate the object for which to get image information
* @param inImageSource Designate the type of image data EdsImageSource
* @param outImageInfo Stores the image data information designated in inImageSource
* @return any of the sdk errors
*/
NativeLong EdsGetImageInfo(EdsImageRef inImageRef, int inImageSource, EdsImageInfo outImageInfo);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetImage
* //
* // Description:
* // Gets designated image data from an image file, in the form of a
* // designated rectangle.
* // Returns uncompressed results for JPEGs and processed results
* // in the designated pixel order (RGB, Top-down BGR, and so on) for
* // RAW images.
* // Additionally, by designating the input/output rectangle,
* // it is possible to get reduced, enlarged, or partial images.
* // However, because images corresponding to the designated output rectangle
* // are always returned by the SDK, the SDK does not take the aspect
* // ratio into account.
* // To maintain the aspect ratio, you must keep the aspect ratio in mind
* // when designating the rectangle.
* //
* // Parameters:
* // In:
* // inImageRef - Designate the image object for which to get
* // the image data.
* // inImageSource - Designate the type of image data to get from
* // the image file (thumbnail, preview, and so on).
* // Designate values as defined in Enum EdsImageSource.
* // inImageType - Designate the output image type. Because
* // the output format of EdGetImage may only be RGB, only
* // kEdsTargetImageType_RGB or kEdsTargetImageType_RGB16
* // can be designated.
* // However, image types exceeding the resolution of
* // inImageSource cannot be designated.
* // inSrcRect - Designate the coordinates and size of the rectangle
* // to be retrieved (processed) from the source image.
* // inDstSize - Designate the rectangle size for output.
* //
* // Out:
* // outStreamRef - Designate the memory or file stream for output of
* // the image.
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetImage(EdsImageRef, EdsImageSource, EdsTargetImageType, EdsRect, EdsSize, EdsStreamRef)
* native declaration : sdk-header\EDSDK.h:1095
*
* @param inImageRef Designate the image object for which to get the image data
* @param inImageSource Designate the type of image data to get from the image file (thumbnail, preview, and so on)
* @param inImageType Designate the output image type
* @param inSrcRect Designate the coordinates and size of the rectangle to be retrieved (processed) from the source image
* @param inDstSize Designate the rectangle size for output the image
* @param outStreamRef Designate the memory or file stream for output of
* @return any of the sdk errors
*/
NativeLong EdsGetImage(EdsImageRef inImageRef, int inImageSource, int inImageType, EdsRect.ByValue inSrcRect, EdsSize.ByValue inDstSize, EdsStreamRef outStreamRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsSaveImage
* //
* // Description:
* // Saves as a designated image type after RAW processing.
* // When saving with JPEG compression,
* // the JPEG quality setting applies with respect to EdsOptionRef.
* //
* // Parameters:
* // In:
* // inImageRef - Designate the image object for which to produce the file.
* // inImageType - Designate the image type to produce. Designate the
* // following image types.
* //
* // kEdsTargetImageType - Jpeg JPEG
* // kEdsTargetImageType - TIFF 8-bit TIFF
* // kEdsTargetImageType - TIFF16 16-bit TIFF
* // inSaveSetting - Designate saving options, such as JPEG image quality.
* // Out:
* // outStreamRef - Specifies the output file stream. The memory stream
* // cannot be specified here.
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsSaveImage(EdsImageRef, EdsTargetImageType, EdsSaveImageSetting, EdsStreamRef)
* native declaration : sdk-header\EDSDK.h:1128
*
* @param inImageRef Designate the image object for which to produce the file
* @param inImageType Designate the image type to produce
* @param inSaveSetting Designate saving options, such as JPEG image quality
* @param outStreamRef Specifies the output file stream. The memory stream cannot be specified here
* @return any of the sdk errors
*/
NativeLong EdsSaveImage(EdsImageRef inImageRef, int inImageType, EdsSaveImageSetting.ByValue inSaveSetting, EdsStreamRef outStreamRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsCacheImage
* //
* // Description:
* // Switches a setting on and off for creation of an image cache in the SDK
* // for a designated image object during extraction (processing) of
* // the image data.
* // Creating the cache increases the processing speed, starting from
* // the second time.
* //
* // Parameters:
* // In: inImageRef - The reference of the image.
* // inUseCache - If cache image data or not
* // If set to FALSE, the cached image data will released.
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsCacheImage(EdsImageRef, EdsBool)
* native declaration : sdk-header\EDSDK.h:1154
*
* @param inImageRef The reference of the image
* @param inUseCache If cache image data or not. If set to FALSE, the cached image data will released
* @return any of the sdk errors
*/
NativeLong EdsCacheImage(EdsImageRef inImageRef, int inUseCache);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsReflectImageProperty
* // Description:
* // Incorporates image object property changes
* // (effected by means of EdsSetPropertyData) in the stream.
* //
* // Parameters:
* // In: inImageRef - The reference of the image.
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsReflectImageProperty(EdsImageRef)
* native declaration : sdk-header\EDSDK.h:1171
*
* @param inImageRef The reference of the image
* @return any of the sdk errors
*/
NativeLong EdsReflectImageProperty(EdsImageRef inImageRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsCreateEvfImageRef
* // Description:
* // Creates an object used to get the live view image data set.
* //
* // Parameters:
* // In: inStreamRef - The stream reference which opened to get EVF JPEG image.
* // Out: outEvfImageRef - The EVFData reference.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsCreateEvfImageRef(EdsStreamRef, EdsEvfImageRef*)
* native declaration : sdk-header\EDSDK.h:1187
*
* @param inStreamRef The stream reference which opened to get EVF JPEG image
* @param outEvfImageRef The EVFData reference
* @return any of the sdk errors
*/
NativeLong EdsCreateEvfImageRef(EdsStreamRef inStreamRef, EdsEvfImageRef.ByReference outEvfImageRef);
// NativeLong EdsCreateEvfImageRef(EdsStreamRef inStreamRef, PointerByReference outEvfImageRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsDownloadEvfImage
* // Description:
* // Downloads the live view image data set for a camera currently in live view mode.
* // Live view can be started by using the property ID:kEdsPropertyID_Evf_OutputDevice and
* // data:EdsOutputDevice_PC to call EdsSetPropertyData.
* // In addition to image data, information such as zoom, focus position, and histogram data
* // is included in the image data set. Image data is saved in a stream maintained by EdsEvfImageRef.
* // EdsGetPropertyData can be used to get information such as the zoom, focus position, etc.
* // Although the information of the zoom and focus position can be obtained from EdsEvfImageRef,
* // settings are applied to EdsCameraRef.
* //
* // Parameters:
* // In: inCameraRef - The Camera reference.
* // In: inEvfImageRef - The EVFData reference.
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsDownloadEvfImage(EdsCameraRef, EdsEvfImageRef)
* native declaration : sdk-header\EDSDK.h:1212
*
* @param inCameraRef The Camera reference
* @param inEvfImageRef The EVFData reference
* @return any of the sdk errors
*/
NativeLong EdsDownloadEvfImage(EdsCameraRef inCameraRef, EdsEvfImageRef inEvfImageRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsSetCameraAddedHandler
* //
* // Description:
* // Registers a callback function for when a camera is detected.
* //
* // Parameters:
* // In: inCameraAddedHandler - Pointer to a callback function
* // called when a camera is connected physically
* // inContext - Specifies an application-defined value to be sent to
* // the callback function pointed to by CallBack parameter.
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsSetCameraAddedHandler(EdsCameraAddedHandler, EdsVoid*)
* native declaration : sdk-header\EDSDK.h:1242
*
* @param inCameraAddedHandler Pointer to a callback function called when a camera is connected physically
* @param inContext Specifies an application-defined value to be sent to he callback function pointed to by CallBack parameter
* @return any of the sdk errors
*/
NativeLong EdsSetCameraAddedHandler(EdsCameraAddedHandler inCameraAddedHandler, Pointer inContext);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
@Deprecated
NativeLong EdsSetCameraAddedHandler(EdsCameraAddedHandler inCameraAddedHandler, EdsVoid inContext);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsSetPropertyEventHandler
* //
* // Description:
* // Registers a callback function for receiving status
* // change notification events for property states on a camera.
* //
* // Parameters:
* // In: inCameraRef - Designate the camera object.
* // inEvent - Designate one or all events to be supplemented.
* // inPropertyEventHandler - Designate the pointer to the callback
* // function for receiving property-related camera events.
* // inContext - Designate application information to be passed by
* // means of the callback function. Any data needed for
* // your application can be passed.
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsSetPropertyEventHandler(EdsCameraRef, EdsPropertyEvent, EdsPropertyEventHandler, EdsVoid*)
* native declaration : sdk-header\EDSDK.h:1267
*
* @param inCameraRef Designate the camera object
* @param inEvnet Designate one or all events to be supplemented
* @param inPropertyEventHandler Designate the pointer to the callback function for receiving property-related camera events
* @param inContext Designate application information to be passed by means of the callback function. Any data needed for your application can be passed
* @return any of the sdk errors
*/
NativeLong EdsSetPropertyEventHandler(EdsCameraRef inCameraRef, NativeLong inEvnet, EdsPropertyEventHandler inPropertyEventHandler, Pointer inContext);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
@Deprecated
NativeLong EdsSetPropertyEventHandler(EdsCameraRef inCameraRef, NativeLong inEvnet, EdsPropertyEventHandler inPropertyEventHandler, EdsVoid inContext);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsSetObjectEventHandler
* //
* // Description:
* // Registers a callback function for receiving status
* // change notification events for objects on a remote camera.
* // Here, object means volumes representing memory cards, files and directories,
* // and shot images stored in memory, in particular.
* //
* // Parameters:
* // In: inCameraRef - Designate the camera object.
* // inEvent - Designate one or all events to be supplemented.
* // To designate all events, use kEdsObjectEvent_All.
* // inObjectEventHandler - Designate the pointer to the callback function
* // for receiving object-related camera events.
* // inContext - Passes inContext without modification,
* // as designated as an EdsSetObjectEventHandler argument.
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsSetObjectEventHandler(EdsCameraRef, EdsObjectEvent, EdsObjectEventHandler, EdsVoid*)
* native declaration : sdk-header\EDSDK.h:1296
*
* @param inCameraRef Designate the camera object
* @param inEvnet Designate one or all events to be supplemented. To designate all events, use kEdsObjectEvent_All
* @param inObjectEventHandler Designate the pointer to the callback function for receiving object-related camera events
* @param inContext Passes inContext without modification, as designated as an EdsSetObjectEventHandler argument
* @return any of the sdk errors
*/
NativeLong EdsSetObjectEventHandler(EdsCameraRef inCameraRef, NativeLong inEvnet, EdsObjectEventHandler inObjectEventHandler, Pointer inContext);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
@Deprecated
NativeLong EdsSetObjectEventHandler(EdsCameraRef inCameraRef, NativeLong inEvnet, EdsObjectEventHandler inObjectEventHandler, EdsVoid inContext);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsSetCameraStateEventHandler
* //
* // Description:
* // Registers a callback function for receiving status
* // change notification events for property states on a camera.
* //
* // Parameters:
* // In: inCameraRef - Designate the camera object.
* // inEvent - Designate one or all events to be supplemented.
* // To designate all events, use kEdsStateEvent_All.
* // inStateEventHandler - Designate the pointer to the callback function
* // for receiving events related to camera object states.
* // inContext - Designate application information to be passed
* // by means of the callback function. Any data needed for
* // your application can be passed.
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsSetCameraStateEventHandler(EdsCameraRef, EdsStateEvent, EdsStateEventHandler, EdsVoid*)
* native declaration : sdk-header\EDSDK.h:1324
*
* @param inCameraRef Designate the camera object
* @param inEvnet Designate one or all events to be supplemented. To designate all events, use kEdsStateEvent_All
* @param inStateEventHandler Designate the pointer to the callback function for receiving events related to camera object states
* @param inContext Designate application information to be passed by means of the callback function. Any data needed for your application can be passed
* @return any of the sdk errors
*/
NativeLong EdsSetCameraStateEventHandler(EdsCameraRef inCameraRef, NativeLong inEvnet, EdsStateEventHandler inStateEventHandler, Pointer inContext);
// will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
@Deprecated
NativeLong EdsSetCameraStateEventHandler(EdsCameraRef inCameraRef, NativeLong inEvnet, EdsStateEventHandler inStateEventHandler, EdsVoid inContext);
/**
* ----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsCreateStream(EdsIStream*, EdsStreamRef*)
* native declaration : sdk-header\EDSDK.h:1332
*
* @param inStream In stream
* @param outStreamRef Out stream ref
* @return any of the sdk errors
*/
NativeLong EdsCreateStream(EdsIStream inStream, EdsStreamRef.ByReference outStreamRef);
// NativeLong EdsCreateStream(EdsIStream inStream, PointerByReference outStreamRef);
/**
* -----------------------------------------------------------------------------
* //
* // Function: EdsGetEvent
* //
* // Description:
* // This function acquires an event.
* // In console application, please call this function regularly to acquire
* // the event from a camera.
* //
* // Parameters:
* // In: None
* // Out: None
* //
* // Returns: Any of the sdk errors.
* -----------------------------------------------------------------------------
* Original signature : __attribute__((dllimport)) EdsError EdsGetEvent()
* native declaration : sdk-header\EDSDK.h:1349
*
* @return any of the sdk errors
*/
NativeLong EdsGetEvent();
public abstract class EdsObjectByReference extends ByReference {
public EdsObjectByReference() {
this(null);
}
public EdsObjectByReference(final T r) {
super(Native.POINTER_SIZE);
setValue(r);
}
public void setValue(final T r) {
getPointer().setPointer(0, r != null ? r.getPointer() : null);
}
public abstract T getValue();
}
/**
* will remove as it requires to do "new EdsVoid(new Memory(size expected))", instead we simply have with Pointer "new Memory(size expected)"
*/
@Deprecated
public static class EdsVoid extends PointerType {
public EdsVoid(Pointer address) {
super(address);
}
public EdsVoid() {
super();
}
}
public static class EdsBaseRef extends PointerType {
public EdsBaseRef() {
super();
}
public EdsBaseRef(Pointer address) {
super(address);
}
public static class ByReference extends EdsObjectByReference {
@Override
public EdsBaseRef getValue() {
final Pointer p = getPointer().getPointer(0);
if (p == null) {
return null;
}
return new EdsBaseRef(p);
}
}
}
/**
* This object represents an enumeration of the cameras remotely connected to the host PC by USB interface.
*
* This object can be used to select the camera to be controlled from among the cameras currently connected with
* EDSDK client application.
*
* This object can also be used when getting an EdsCameraRef child object.
*/
public static class EdsCameraListRef extends EdsBaseRef {
public EdsCameraListRef() {
super();
}
public EdsCameraListRef(Pointer address) {
super(address);
}
public static class ByReference extends EdsBaseRef.ByReference {
@Override
public EdsCameraListRef getValue() {
final Pointer p = getPointer().getPointer(0);
if (p == null) {
return null;
}
return new EdsCameraListRef(p);
}
}
}
/**
* This object represents a remotely connected camera.
*
* This object is used to control the camera or to get an
* EdsVolumeRef object when accessing the memory card, which is a child object of the camera.
*/
public static class EdsCameraRef extends EdsBaseRef {
public EdsCameraRef() {
super();
}
public EdsCameraRef(final Pointer address) {
super(address);
}
public static class ByReference extends EdsBaseRef.ByReference {
@Override
public EdsCameraRef getValue() {
final Pointer p = getPointer().getPointer(0);
if (p == null) {
return null;
}
return new EdsCameraRef(p);
}
}
}
/**
* This object represents the memory card inside the camera.
*
* If the camera model allows two memory cards to be installed at once,
* the EdsVolumeRef object represents one memory card each.
*
* This object is used to get an EdsDirectoryItemRef object, which is
* a child object, when performing operations on a file or folder on the memory card.
*/
public static class EdsVolumeRef extends EdsBaseRef {
public EdsVolumeRef() {
super();
}
public EdsVolumeRef(final Pointer address) {
super(address);
}
public static class ByReference extends EdsBaseRef.ByReference {
@Override
public EdsVolumeRef getValue() {
final Pointer p = getPointer().getPointer(0);
if (p == null) {
return null;
}
return new EdsVolumeRef(p);
}
}
}
/**
* This object represents a file or folder on the camera.
*
* When files are downloaded from the camera, each file to
* be downloaded is treated as one of these objects.
*/
public static class EdsDirectoryItemRef extends EdsBaseRef {
public EdsDirectoryItemRef() {
super();
}
public EdsDirectoryItemRef(final Pointer address) {
super(address);
}
public static class ByReference extends EdsBaseRef.ByReference {
@Override
public EdsDirectoryItemRef getValue() {
final Pointer p = getPointer().getPointer(0);
if (p == null) {
return null;
}
return new EdsDirectoryItemRef(p);
}
}
}
/**
* This object represents the file I/O stream.
*
* An open stream on the host PC can be specified as the download
* destination when downloading files in the camera to the host PC.
*
* Streams are also used when loading image files stored on the storage media of
* the host PC into an EDSDK client application. Furthermore,
* EdsStreamRef objects can also be created in memory.
*/
public static class EdsStreamRef extends EdsBaseRef {
public EdsStreamRef() {
super();
}
public EdsStreamRef(final Pointer address) {
super(address);
}
public static class ByReference extends EdsBaseRef.ByReference {
@Override
public EdsStreamRef getValue() {
final Pointer p = getPointer().getPointer(0);
if (p == null) {
return null;
}
return new EdsStreamRef(p);
}
}
}
/**
* This object represents image data.
*
* This data is obtained from image files.
*
* This object is used to retrieve and control information included with an
* image such as thumbnails and parameters.
*/
public static class EdsImageRef extends EdsStreamRef {
public EdsImageRef() {
super();
}
public EdsImageRef(final Pointer address) {
super(address);
}
public static class ByReference extends EdsBaseRef.ByReference {
@Override
public EdsImageRef getValue() {
final Pointer p = getPointer().getPointer(0);
if (p == null) {
return null;
}
return new EdsImageRef(p);
}
}
}
/**
* This object represents PC live view image data.
*
* When using a camera model that supports live view, live view image
* data set can be downloaded from the camera.
*
* Information such as zoom and histogram data is included with image data.
*/
public static class EdsEvfImageRef extends EdsBaseRef {
public EdsEvfImageRef() {
super();
}
public EdsEvfImageRef(final Pointer address) {
super(address);
}
public static class ByReference extends EdsBaseRef.ByReference {
@Override
public EdsEvfImageRef getValue() {
final Pointer p = getPointer().getPointer(0);
if (p == null) {
return null;
}
return new EdsEvfImageRef(p);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy