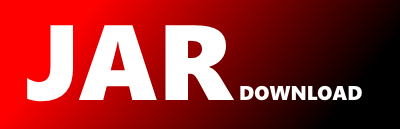
org.bluestemsoftware.open.eoa.plugin.compile.CompilationFailureException Maven / Gradle / Ivy
/**
* Copyright 2008 Bluestem Software LLC. All Rights Reserved.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License version 2 as
* published by the Free Software Foundation.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
*/
package org.bluestemsoftware.open.eoa.plugin.compile;
/*
* Adapted from org.apache.maven.plugin.CompilerMojo which was released under the following
* license:
*
* Copyright 2001-2005 The Apache Software Foundation.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file
* except in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the
* License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
import java.util.Iterator;
import java.util.List;
import org.apache.maven.plugin.MojoFailureException;
import org.codehaus.plexus.compiler.CompilerError;
/**
* @author Jason van Zyl
*/
public class CompilationFailureException extends MojoFailureException {
private static final long serialVersionUID = 1L;
private static final String LS = System.getProperty("line.separator");
public CompilationFailureException(List> messages) {
super(null, shortMessage(messages), longMessage(messages));
}
public static String longMessage(List> messages) {
StringBuffer sb = new StringBuffer();
for (Iterator> it = messages.iterator(); it.hasNext();) {
CompilerError compilerError = (CompilerError)it.next();
sb.append(compilerError).append(LS);
}
return sb.toString();
}
/**
* Short message will have the error message if there's only one, useful for errors forking
* the compiler
*
* @param messages
* @return the short error message
*/
public static String shortMessage(List> messages) {
StringBuffer sb = new StringBuffer();
sb.append("Compilation failure");
if (messages.size() == 1) {
sb.append(LS);
CompilerError compilerError = (CompilerError)messages.get(0);
sb.append(compilerError).append(LS);
}
return sb.toString();
}
}