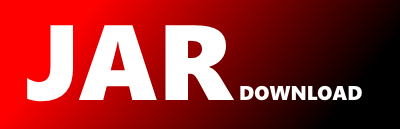
org.bluestemsoftware.open.eoa.plugin.install.InstallMojo Maven / Gradle / Ivy
/**
* Copyright 2008 Bluestem Software LLC. All Rights Reserved.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License version 2 as
* published by the Free Software Foundation.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
*/
package org.bluestemsoftware.open.eoa.plugin.install;
/*
* Adapted from org.apache.maven.plugin.install.InstallMojo which was released under the following
* license:
*
* Copyright 2001-2005 The Apache Software Foundation.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file
* except in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the
* License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND,
* either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
import java.io.File;
import java.util.Iterator;
import java.util.List;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.artifact.installer.ArtifactInstallationException;
import org.apache.maven.artifact.metadata.ArtifactMetadata;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.project.artifact.ProjectArtifactMetadata;
import org.bluestemsoftware.open.eoa.plugin.util.FileUtils;
/**
* Installs project's main artifact in local repository.
*
* @goal install
* @phase install
*/
public class InstallMojo extends AbstractInstallMojo {
/**
* @parameter expression="${project.packaging}"
* @required
* @readonly
*/
protected String packaging;
/**
* @parameter expression="${project.file}"
* @required
* @readonly
*/
private File pomFile;
/**
* Whether to update the metadata to make the artifact as release.
*
* @parameter expression="${updateReleaseInfo}" default-value="false"
*/
private boolean updateReleaseInfo;
/**
* @parameter expression="${project.artifact}"
* @required
* @readonly
*/
private Artifact artifact;
/**
* @parameter expression="${project.attachedArtifacts}
* @required
* @readonly
*/
private List> attachedArtifacts;
public void execute() throws MojoExecutionException {
// TODO: push into transformation
boolean isPomArtifact = "pom".equals(packaging);
ArtifactMetadata metadata = null;
if (updateReleaseInfo) {
artifact.setRelease(true);
}
try {
if (isPomArtifact) {
installer.install(pomFile, artifact, localRepository);
} else {
metadata = new ProjectArtifactMetadata(artifact, pomFile);
artifact.addMetadata(metadata);
File file = artifact.getFile();
// Here, we have a temporary solution to MINSTALL-3 (isDirectory() is true if
// it went through compile
// but not package). We are designing in a proper solution for Maven 2.1
if (file != null && !file.isDirectory()) {
installer.install(file, artifact, localRepository);
expandArtifact(file); // added TWW
if (createChecksum) {
// create checksums for pom and artifact
File pom = new File(localRepository.getBasedir(), localRepository
.pathOfLocalRepositoryMetadata(metadata, localRepository));
installCheckSum(pom, true);
installCheckSum(file, artifact, false);
}
} else if (!attachedArtifacts.isEmpty()) {
getLog().info("No primary artifact to install, installing attached artifacts instead.");
} else {
throw new MojoExecutionException(
"The packaging for this project did not assign a file to the build artifact");
}
}
for (Iterator> i = attachedArtifacts.iterator(); i.hasNext();) {
Artifact attached = (Artifact)i.next();
installer.install(attached.getFile(), attached, localRepository);
if (createChecksum) {
installCheckSum(attached.getFile(), attached, false);
}
}
} catch (ArtifactInstallationException e) {
throw new MojoExecutionException(e.getMessage(), e);
}
}
/*
* added method. TWW
*/
private void expandArtifact(File source) throws MojoExecutionException {
// create an expanded image of artifact within repository
// which may be repository used by alakai, i.e. which
// will significantly decrease system start-up/shutdown
// time
String localPath = localRepository.pathOf(artifact);
localPath = localPath.substring(0, localPath.lastIndexOf("."));
localPath = localPath + ".expanded";
File destination = new File(localRepository.getBasedir(), localPath);
if (!destination.getParentFile().exists()) {
destination.getParentFile().mkdirs();
}
if (destination.exists()) {
FileUtils.deleteFile(destination);
}
try {
FileUtils.unpackArchive(source, destination, false);
} catch (Exception ex) {
throw new MojoExecutionException("Error expanding artifact. " + ex);
}
}
}