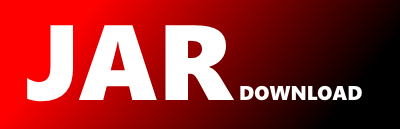
org.bluestemsoftware.open.eoa.plugin.surefire.IntegrationTestMojo Maven / Gradle / Ivy
/**
* Copyright 2008 Bluestem Software LLC. All Rights Reserved.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License version 2 as
* published by the Free Software Foundation.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
*/
package org.bluestemsoftware.open.eoa.plugin.surefire;
import java.io.File;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.bluestemsoftware.open.eoa.plugin.util.FileFilterImpl;
/**
* Executes integration tests. Note that plexus component descriptor included within plugin
* dist binds integration-test phase within default lifecycle to this mojo's it-test goal.
*
* @goal it-test
* @phase integration-test
*/
public class IntegrationTestMojo extends AbstractSurefireMojo {
/**
* Identifies suite(s) to be processed. Default is 'all'.
*
* @parameter expression="${maven.surefire.it.suite}" default-value="all"
*/
private String suiteName;
/**
* Name of system property, which if set, signals forked jvm, i.e. system it tests fork, to
* wait for a jwdp attachment via port 5005.
*
* @parameter expression="maven.surefire.it.debug"
* @required
* @readonly
*/
private String debugPropertyName;
/**
* Name of system property, which if set, signals forked jvm, i.e. client it tests fork, to
* wait for a jwdp attachment via port 5005.
*
* @parameter expression="maven.surefire.it.client.debug"
* @required
* @readonly
*/
private String clientDebugPropertyName;
private File system;
/*
* (non-Javadoc)
* @see org.bluestemsoftware.open.eoa.plugin.surefire.AbstractSurefireMojo#isIntegrationTest()
*/
protected boolean isIntegrationTest() {
return true;
}
/*
* (non-Javadoc)
* @see org.bluestemsoftware.open.eoa.plugin.surefire.AbstractSurefireMojo#execute()
*/
public void execute() throws MojoExecutionException, MojoFailureException {
if (skip) {
getLog().info("tests skipped. not compiling sources");
return;
}
System.setProperty("basedir", basedir.getAbsolutePath());
File it = new File(basedir, "target/it/");
if (!it.exists() || !it.isDirectory()) {
getLog().info("no tests to execute");
return;
}
System.out.println(LINE_BREAK + "executing integration tests");
if (suiteName.equals("all")) {
File[] suites = it.listFiles(new FileFilterImpl());
for (int i = 0; i < suites.length; i++) {
File suite = suites[i];
if (!suite.isDirectory()) {
throw new MojoExecutionException("Invalid directory structure.");
}
System.out.println(LINE_BREAK + "processing " + suite.getName());
system = new File(suite, "system/");
if (!system.exists() || !system.isDirectory()) {
throw new MojoExecutionException("Invalid directory structure.");
}
super.execute();
}
} else {
File suite = new File(it, suiteName + "/");
if (!suite.exists() || !suite.isDirectory()) {
throw new MojoExecutionException("Value '"
+ suiteName
+ "' specified for parameter 'maven.surefire.it.suite' is invalid."
+ " Directory '"
+ suite.getAbsolutePath()
+ "' does not exist.");
} else {
System.out.println(LINE_BREAK + "processing " + suite.getName());
system = new File(suite, "system/");
if (!system.exists() || !system.isDirectory()) {
throw new MojoExecutionException("Invalid directory structure.");
}
super.execute();
}
}
}
/*
* (non-Javadoc)
* @see org.bluestemsoftware.open.eoa.plugin.surefire.AbstractSurefireMojo#getTestClassesDirectory()
*/
protected File getSystemTestClassesDirectory() {
return new File(system, "test-classes/");
}
/*
* (non-Javadoc)
* @see org.bluestemsoftware.open.eoa.plugin.surefire.AbstractSurefireMojo#getClientTestClassesDirectory()
*/
protected File getClientTestClassesDirectory() {
File suite = system.getParentFile();
File client = new File(suite, "client/test-classes/");
if (client.exists() && client.isDirectory()) {
return client;
} else {
return null;
}
}
/*
* (non-Javadoc)
* @see org.bluestemsoftware.open.eoa.plugin.surefire.AbstractSurefireMojo#getTestReportsDirectory()
*/
protected File getTestReportsDirectory() {
String suite = system.getParentFile().getName();
String relativePath = "it/" + suite + "/system/";
return new File(reportsDirectory, relativePath);
}
/*
* defined as a paramater for plugin documentation only. property name is used within
* surefire booter to set debug option when running jvm
*/
protected String getDebugPropertyName() {
return debugPropertyName;
}
/*
* defined as a paramater for plugin documentation only. property name is used within
* surefire booter to set debug option when running jvm
*/
protected String getClientDebugPropertyName() {
return clientDebugPropertyName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy