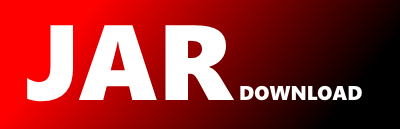
org.bluestemsoftware.open.eoa.plugin.util.FileUtils Maven / Gradle / Ivy
/**
* Copyright 2008 Bluestem Software LLC. All Rights Reserved.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License version 2 as
* published by the Free Software Foundation.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*
*/
package org.bluestemsoftware.open.eoa.plugin.util;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.Enumeration;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
public class FileUtils {
public static void unpackArchive(File archive, File outputDir, boolean isDestinationTemporary) throws Exception {
outputDir.mkdir();
if (isDestinationTemporary) {
outputDir.deleteOnExit();
}
ZipFile zipFile = new ZipFile(archive);
try {
Enumeration> entries = zipFile.entries();
while (entries.hasMoreElements()) {
ZipEntry entry = (ZipEntry)entries.nextElement();
File file = new File(outputDir, File.separator + entry.getName());
if (entry.isDirectory()) {
mkdirs(file);
} else {
InputStream in = null;
OutputStream out = null;
try {
in = zipFile.getInputStream(entry);
out = new BufferedOutputStream(new FileOutputStream(file));
byte[] buffer = new byte[4096];
for (int length = 0; length >= 0; length = in.read(buffer)) {
out.write(buffer, 0, length);
}
} finally {
if (in != null) {
in.close();
}
if (out != null) {
out.close();
}
}
if (isDestinationTemporary) {
file.deleteOnExit();
}
}
}
} finally {
if (zipFile != null) {
zipFile.close();
}
}
}
public static File copyDir(File source, File target, boolean isDestinationTemporary) throws Exception {
target.mkdir();
if (isDestinationTemporary) {
target.deleteOnExit();
}
File[] entries = source.listFiles();
for (int i = 0; i < entries.length; i++) {
File entry = entries[i];
File file = new File(target, File.separator + entry.getName());
if (entry.isDirectory()) {
copyDir(entry, file, isDestinationTemporary);
} else {
InputStream in = null;
OutputStream out = null;
try {
in = new FileInputStream(entry);
out = new BufferedOutputStream(new FileOutputStream(file));
byte[] buffer = new byte[4096];
for (int length = 0; length >= 0; length = in.read(buffer)) {
out.write(buffer, 0, length);
}
} finally {
if (in != null) {
in.close();
}
if (out != null) {
out.close();
}
}
if (isDestinationTemporary) {
file.deleteOnExit();
}
}
}
return target;
}
public static boolean deleteFile(File file) {
boolean result = true;
if (file.exists()) {
if (file.isDirectory()) {
File[] contents = file.listFiles();
if (contents != null) {
for (int i = 0; i < contents.length; i++) {
File content = contents[i];
if (content.isDirectory()) {
result &= deleteFile(content);
} else {
result &= content.delete();
}
}
}
}
return result & file.delete();
}
return result;
}
private static void mkdirs(File child) {
if (child.exists() == false) {
child.mkdir();
child.deleteOnExit();
}
File parent = child.getParentFile();
while (parent != null && parent.exists() == false) {
parent.mkdir();
parent.deleteOnExit();
parent = parent.getParentFile();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy