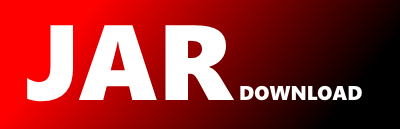
bndtools.utils.HierarchicalLabel Maven / Gradle / Ivy
The newest version!
package bndtools.utils;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.function.Function;
/**
* This class uses a list labels to internally store the different hierarchical
* labels. It provides methods to get the number of layers, retrieve a label by
* position, retrieve the first and last labels to e.g. build a menu with
* submenues.
*
*
* public static void main(String[] args) {
* HierarchicalLabel label = new HierarchicalLabel("layer1/layer2/layer3/layer4", (l) -> new Action(l.getLast()));
*
* System.out.println("Number of layers: " + label.getNumLevels());
* System.out.println("Label at position 2: " + label.getByPosition(2));
* System.out.println("First label: " + label.getFirst());
* System.out.println("Last label: " + label.getLast());
*
* }
*
*/
public class HierarchicalLabel {
private static final String DELIMITER_FOR_HIERARCHY = " :: ";
private final List labels;
private Function, T> leafActionCallback;
boolean enabled = true;
boolean checked = false;
String description = null;
/**
* @param compoundLabel a string consisting of multiple parts which is parse
* by a regex into 4 parts. E.g.
* Example Strings:
*
* -
*
* "-!MyLabel{This is a description}"
*
*
* - Group 1:
-
=> enabled
is
* false
.
* - Group 2:
!
=> checked
is
* true
.
* - Group 3:
MyLabel
=> Label text is
* "MyLabel".
* - Group 4:
This is a description
=>
* Description is "This is a description".
*
*
* -
*
* "!MyLabel"
*
*
* - Group 1: Not present =>
enabled
is not
* affected (remains whatever value it previously had).
* - Group 2:
!
=> checked
is
* true
.
* - Group 3:
MyLabel
=> Label text is
* "MyLabel".
* - Group 4: Not present => Description is
*
null
.
*
*
* -
*
* "MyLabel{Description here}"
*
*
* - Group 1: Not present =>
enabled
is not
* affected.
* - Group 2: Not present =>
checked
is not
* affected.
* - Group 3:
MyLabel
=> Label text is
* "MyLabel".
* - Group 4:
Description here
=> Description
* is "Description here".
*
*
*
*
* These example strings illustrate how the regex pattern breaks
* down and captures each part of the input string.
*
* @param leafNodeAction a callback to create an Action from the outside
*/
public HierarchicalLabel(String compoundLabel, Function, T> leafNodeAction) {
this.leafActionCallback = leafNodeAction;
if (compoundLabel == null || compoundLabel.trim()
.isEmpty()) {
throw new IllegalArgumentException("Label string cannot be null or empty.");
}
LabelParser parsedEntry = new LabelParser(compoundLabel);
this.enabled = parsedEntry.isEnabled();
this.checked = parsedEntry.isChecked();
this.description = parsedEntry.getDescription();
String label = parsedEntry.getLabel();
this.labels = new ArrayList<>(Arrays.asList(label.split(DELIMITER_FOR_HIERARCHY)));
}
public T getLeafAction() {
return leafActionCallback.apply(this);
}
public int getNumLLevels() {
return labels.size();
}
public String getByPosition(int position) {
if (position < 0 || position >= labels.size()) {
throw new IllegalArgumentException("Position out of bounds.");
}
return labels.get(position);
}
public String getFirst() {
return labels.get(0);
}
/**
* @return the last entry (most right)
*/
public String getLeaf() {
return labels.get(labels.size() - 1);
}
public List getLabels() {
return labels;
}
public String getDescription() {
return description;
}
public boolean isEnabled() {
return enabled;
}
public boolean isChecked() {
return checked;
}
@Override
public String toString() {
return String.join(DELIMITER_FOR_HIERARCHY, labels);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy