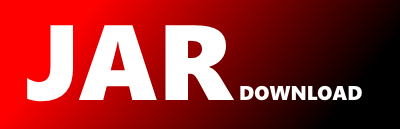
aQute.lib.exceptions.BiFunctionWithException Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.bndtools.templating.gitrepo Show documentation
Show all versions of org.bndtools.templating.gitrepo Show documentation
org.bndtools.templating.gitrepo
package aQute.lib.exceptions;
import static java.util.Objects.requireNonNull;
import java.util.function.BiFunction;
import java.util.function.Supplier;
/**
* BiFunction interface that allows exceptions.
*
* @param the type 1 of the argument
* @param the type 2 of the argument
* @param the result type
*/
@FunctionalInterface
public interface BiFunctionWithException {
R apply(T t, U u) throws Exception;
default BiFunction orElseThrow() {
return (t, u) -> {
try {
return apply(t, u);
} catch (Exception e) {
throw Exceptions.duck(e);
}
};
}
default BiFunction orElse(R orElse) {
return (t, u) -> {
try {
return apply(t, u);
} catch (Exception e) {
return orElse;
}
};
}
default BiFunction orElseGet(Supplier extends R> orElseGet) {
requireNonNull(orElseGet);
return (t, u) -> {
try {
return apply(t, u);
} catch (Exception e) {
return orElseGet.get();
}
};
}
static BiFunction asBiFunction(BiFunctionWithException unchecked) {
return unchecked.orElseThrow();
}
static BiFunction asBiFunctionOrElse(BiFunctionWithException unchecked, R orElse) {
return unchecked.orElse(orElse);
}
static BiFunction asBiFunctionOrElseGet(BiFunctionWithException unchecked,
Supplier extends R> orElseGet) {
return unchecked.orElseGet(orElseGet);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy