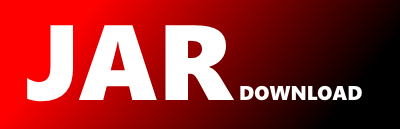
aQute.lib.exceptions.PredicateWithException Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of org.bndtools.templating.gitrepo Show documentation
Show all versions of org.bndtools.templating.gitrepo Show documentation
org.bndtools.templating.gitrepo
package aQute.lib.exceptions;
import static java.util.Objects.requireNonNull;
import java.util.function.BooleanSupplier;
import java.util.function.Predicate;
/**
* Predicate interface that allows exceptions.
*
* @param the type of the argument
*/
@FunctionalInterface
public interface PredicateWithException {
boolean test(T t) throws Exception;
default Predicate orElseThrow() {
return t -> {
try {
return test(t);
} catch (Exception e) {
throw Exceptions.duck(e);
}
};
}
default Predicate orElse(boolean orElse) {
return t -> {
try {
return test(t);
} catch (Exception e) {
return orElse;
}
};
}
default Predicate orElseGet(BooleanSupplier orElseGet) {
requireNonNull(orElseGet);
return t -> {
try {
return test(t);
} catch (Exception e) {
return orElseGet.getAsBoolean();
}
};
}
static Predicate asPredicate(PredicateWithException unchecked) {
return unchecked.orElseThrow();
}
static Predicate asPredicateOrElse(PredicateWithException unchecked, boolean orElse) {
return unchecked.orElse(orElse);
}
static Predicate asPredicateOrElseGet(PredicateWithException unchecked, BooleanSupplier orElseGet) {
return unchecked.orElseGet(orElseGet);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy