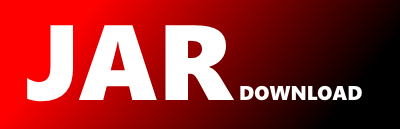
org.bonitasoft.engine.api.PlatformAPIAccessor Maven / Gradle / Ivy
/**
* Copyright (C) 2019 Bonitasoft S.A.
* Bonitasoft, 32 rue Gustave Eiffel - 38000 Grenoble
* This library is free software; you can redistribute it and/or modify it under the terms
* of the GNU Lesser General Public License as published by the Free Software Foundation
* version 2.1 of the License.
* This library is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY;
* without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
* See the GNU Lesser General Public License for more details.
* You should have received a copy of the GNU Lesser General Public License along with this
* program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth
* Floor, Boston, MA 02110-1301, USA.
**/
package org.bonitasoft.engine.api;
import java.io.IOException;
import java.lang.reflect.Proxy;
import java.util.Map;
import org.bonitasoft.engine.api.impl.ClientInterceptor;
import org.bonitasoft.engine.api.impl.LocalServerAPIFactory;
import org.bonitasoft.engine.api.internal.ServerAPI;
import org.bonitasoft.engine.exception.BonitaHomeNotSetException;
import org.bonitasoft.engine.exception.ServerAPIException;
import org.bonitasoft.engine.exception.UnknownAPITypeException;
import org.bonitasoft.engine.session.InvalidSessionException;
import org.bonitasoft.engine.session.PlatformSession;
import org.bonitasoft.engine.util.APITypeManager;
/**
* Accessor class that retrieve Platform APIs
*
* All APIs given by this class are relevant to the platform only.
*
* - {@link PlatformAPI}
* - {@link PlatformCommandAPI}
* - {@link PlatformLoginAPI}
*
*
* @author Matthieu Chaffotte
*/
public class PlatformAPIAccessor {
static ServerAPI getServerAPI() throws BonitaHomeNotSetException, ServerAPIException, UnknownAPITypeException {
try {
final ApiAccessType apiType = APITypeManager.getAPIType();
Map parameters;
switch (apiType) {
case LOCAL:
return LocalServerAPIFactory.getServerAPI();
case HTTP:
parameters = APITypeManager.getAPITypeParameters();
return new HTTPServerAPI(parameters);
default:
throw new UnknownAPITypeException("Unsupported API Type: " + apiType);
}
} catch (IOException e) {
throw new ServerAPIException(e);
}
}
/**
* Reload the configuration of the Bonita home from the file system
* It allows to change in runtime the Bonita engine your client application uses
*/
public static void refresh() {
APITypeManager.refresh();
}
/**
* @return the {@link PlatformLoginAPI}
* @throws BonitaHomeNotSetException
* @throws ServerAPIException
* @throws UnknownAPITypeException
*/
public static PlatformLoginAPI getPlatformLoginAPI()
throws BonitaHomeNotSetException, ServerAPIException, UnknownAPITypeException {
return getAPI(PlatformLoginAPI.class);
}
static T getAPI(final Class clazz, final PlatformSession session)
throws BonitaHomeNotSetException, ServerAPIException,
UnknownAPITypeException {
final ServerAPI serverAPI = getServerAPI();
final ClientInterceptor sessionInterceptor = new ClientInterceptor(clazz.getName(), serverAPI, session);
return (T) Proxy.newProxyInstance(APIAccessor.class.getClassLoader(), new Class[] { clazz },
sessionInterceptor);
}
static T getAPI(final Class clazz) throws BonitaHomeNotSetException, ServerAPIException,
UnknownAPITypeException {
final ServerAPI serverAPI = getServerAPI();
final ClientInterceptor sessionInterceptor = new ClientInterceptor(clazz.getName(), serverAPI);
return (T) Proxy.newProxyInstance(APIAccessor.class.getClassLoader(), new Class[] { clazz },
sessionInterceptor);
}
/**
* @param session
* a {@link PlatformSession} created using the {@link PlatformLoginAPI}
* @return
* the {@link PlatformAPI}
* @throws InvalidSessionException
* @throws BonitaHomeNotSetException
* @throws ServerAPIException
* @throws UnknownAPITypeException
*/
public static PlatformAPI getPlatformAPI(final PlatformSession session)
throws BonitaHomeNotSetException, ServerAPIException, UnknownAPITypeException {
return getAPI(PlatformAPI.class, session);
}
/**
* @param session
* a {@link PlatformSession} created using the {@link PlatformLoginAPI}
* @return
* the {@link PlatformCommandAPI}
* @throws BonitaHomeNotSetException
* @throws ServerAPIException
* @throws UnknownAPITypeException
* @throws InvalidSessionException
*/
public static PlatformCommandAPI getPlatformCommandAPI(final PlatformSession session)
throws BonitaHomeNotSetException, ServerAPIException,
UnknownAPITypeException {
return getAPI(PlatformCommandAPI.class, session);
}
public static TemporaryContentAPI getTemporaryContentAPI()
throws BonitaHomeNotSetException, ServerAPIException, UnknownAPITypeException {
return getAPI(TemporaryContentAPI.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy