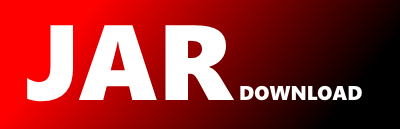
org.bonitasoft.web.client.api.ProcessApi Maven / Gradle / Ivy
/**
* Copyright (C) 2023 BonitaSoft S.A.
* BonitaSoft, 32 rue Gustave Eiffel - 38000 Grenoble
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 2.0 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package org.bonitasoft.web.client.api;
import java.io.File;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.bonitasoft.web.client.invoker.ApiClient;
import org.bonitasoft.web.client.invoker.EncodingUtils;
import org.bonitasoft.web.client.model.ApiResponse;
import org.bonitasoft.web.client.model.Contract;
import org.bonitasoft.web.client.model.DesignProcessDefinition;
import org.bonitasoft.web.client.model.ProcessCreateRequest;
import org.bonitasoft.web.client.model.ProcessDefinition;
import org.bonitasoft.web.client.model.ProcessInstantiationResponse;
import org.bonitasoft.web.client.model.ProcessUpdateRequest;
import org.bonitasoft.web.client.model.UpdateProcessConnectorByProcessIdRequest;
import feign.*;
@jakarta.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen")
public interface ProcessApi extends ApiClient.Api {
/**
* Create the Process
* Create the Process. A process resource is created using the content of a .bar file that has previously been uploaded, using the [processUpload
* servlet](#operation/uploadProcess), to get the process archive path.
*
* @param body Partial Process description (required)
* @return ProcessDefinition
*/
@RequestLine("POST /API/bpm/process")
@Headers({
"Content-Type: application/json",
"Accept: application/json",
})
ProcessDefinition createProcess(ProcessCreateRequest body);
/**
* Create the Process
* Similar to createProcess
but it also returns the http response headers .
* Create the Process. A process resource is created using the content of a .bar file that has previously been uploaded, using the [processUpload
* servlet](#operation/uploadProcess), to get the process archive path.
*
* @param body Partial Process description (required)
* @return A ApiResponse that wraps the response boyd and the http headers.
*/
@RequestLine("POST /API/bpm/process")
@Headers({
"Content-Type: application/json",
"Accept: application/json",
})
ApiResponse createProcessWithHttpInfo(ProcessCreateRequest body);
/**
* Delete the Process by ID
* Delete the single Process for the given ID. **Warning: Beware! Data loss risk!** Deleting a process will automatically delete all its process instances
* (on-going and archived alike). Thus, the operation may take a long time, and fail if the transaction timeout is not large enough. This feature should only be
* used on non-production environments. **Please proceed at your own risk.**
*
* @param id ID of the Process to delete (required)
*/
@RequestLine("DELETE /API/bpm/process/{id}")
@Headers({
"Accept: application/json",
})
void deleteProcessById(@Param("id") String id);
/**
* Delete the Process by ID
* Similar to deleteProcessById
but it also returns the http response headers .
* Delete the single Process for the given ID. **Warning: Beware! Data loss risk!** Deleting a process will automatically delete all its process instances
* (on-going and archived alike). Thus, the operation may take a long time, and fail if the transaction timeout is not large enough. This feature should only be
* used on non-production environments. **Please proceed at your own risk.**
*
* @param id ID of the Process to delete (required)
*/
@RequestLine("DELETE /API/bpm/process/{id}")
@Headers({
"Accept: application/json",
})
ApiResponse deleteProcessByIdWithHttpInfo(@Param("id") String id);
/**
* Delete the Process by IDs
* Delete Process for the given list of ID. **Warning: Beware! Data loss risk!** Deleting a process will automatically delete all its process instances
* (on-going and archived alike). Thus, the operation may take a long time, and fail if the transaction timeout is not large enough. This feature should only be
* used on non-production environments. **Please proceed at your own risk.**
*
* @param requestBody (optional)
*/
@RequestLine("DELETE /API/bpm/process")
@Headers({
"Content-Type: application/json",
"Accept: application/json",
})
void deleteProcessByIds(List requestBody);
/**
* Delete the Process by IDs
* Similar to deleteProcessByIds
but it also returns the http response headers .
* Delete Process for the given list of ID. **Warning: Beware! Data loss risk!** Deleting a process will automatically delete all its process instances
* (on-going and archived alike). Thus, the operation may take a long time, and fail if the transaction timeout is not large enough. This feature should only be
* used on non-production environments. **Please proceed at your own risk.**
*
* @param requestBody (optional)
*/
@RequestLine("DELETE /API/bpm/process")
@Headers({
"Content-Type: application/json",
"Accept: application/json",
})
ApiResponse deleteProcessByIdsWithHttpInfo(List requestBody);
/**
* Finds the Process by ID
* Returns the single Process for the given ID
*
* @param id ID of the Process to return (required)
* @return ProcessDefinition
*/
@RequestLine("GET /API/bpm/process/{id}")
@Headers({
"Accept: application/json",
})
ProcessDefinition getProcessById(@Param("id") String id);
/**
* Finds the Process by ID
* Similar to getProcessById
but it also returns the http response headers .
* Returns the single Process for the given ID
*
* @param id ID of the Process to return (required)
* @return A ApiResponse that wraps the response boyd and the http headers.
*/
@RequestLine("GET /API/bpm/process/{id}")
@Headers({
"Accept: application/json",
})
ApiResponse getProcessByIdWithHttpInfo(@Param("id") String id);
/**
* Finds the Process contract by ID
* Returns the process contract for the given ID
*
* @param id ID of the Process to get the contract from (required)
* @return Contract
*/
@RequestLine("GET /API/bpm/process/{id}/contract")
@Headers({
"Accept: application/json",
})
Contract getProcessContractById(@Param("id") String id);
/**
* Finds the Process contract by ID
* Similar to getProcessContractById
but it also returns the http response headers .
* Returns the process contract for the given ID
*
* @param id ID of the Process to get the contract from (required)
* @return A ApiResponse that wraps the response boyd and the http headers.
*/
@RequestLine("GET /API/bpm/process/{id}/contract")
@Headers({
"Accept: application/json",
})
ApiResponse getProcessContractByIdWithHttpInfo(@Param("id") String id);
/**
* Finds the Process design by ID
* Returns the single Process design for the given ID
*
* @param id ID of the Process to get the design from (required)
* @return DesignProcessDefinition
*/
@RequestLine("GET /API/bpm/process/{id}/design")
@Headers({
"Accept: application/json",
})
DesignProcessDefinition getProcessDesignById(@Param("id") String id);
/**
* Finds the Process design by ID
* Similar to getProcessDesignById
but it also returns the http response headers .
* Returns the single Process design for the given ID
*
* @param id ID of the Process to get the design from (required)
* @return A ApiResponse that wraps the response boyd and the http headers.
*/
@RequestLine("GET /API/bpm/process/{id}/design")
@Headers({
"Accept: application/json",
})
ApiResponse getProcessDesignByIdWithHttpInfo(@Param("id") String id);
/**
* Instanciate the process
* Instanciate the process with the provided contract values.
*
* @param id ID of the process to instanciate (required)
* @param body A JSON object matching process contract. (required)
* @return ProcessInstantiationResponse
*/
@RequestLine("POST /API/bpm/process/{id}/instantiation")
@Headers({
"Content-Type: application/json",
"Accept: application/json",
})
ProcessInstantiationResponse instanciateProcess(@Param("id") String id, Map body);
/**
* Instanciate the process
* Similar to instanciateProcess
but it also returns the http response headers .
* Instanciate the process with the provided contract values.
*
* @param id ID of the process to instanciate (required)
* @param body A JSON object matching process contract. (required)
* @return A ApiResponse that wraps the response boyd and the http headers.
*/
@RequestLine("POST /API/bpm/process/{id}/instantiation")
@Headers({
"Content-Type: application/json",
"Accept: application/json",
})
ApiResponse instanciateProcessWithHttpInfo(@Param("id") String id,
Map body);
/**
* Finds Processes
* Finds Processes with pagination params and filters - can order (default is ASC) on `name`, `version`, `deploymentDate`,
* `deployedBy`, `activationState`, `configurationState`, `processId`, `displayName`,
* `lastUpdateDate`, `categoryId`, `label` - can search on `name`, `displayName` or `version` - can
* filter on `name`, `version`, `deploymentDate`, `deployedBy`, `activationState` with the value DISABLED or
* ENABLED, `configurationState` with the value UNRESOLVED, or RESOLVED, `processId`, `displayName`, `lastUpdateDate`,
* `categoryId`, `label`, `supervisor_id`
*
* @param p index of the page to display (required)
* @param c maximum number of elements to retrieve (required)
* @param f can filter on attributes with the format f={filter\\_name}={filter\\_value} with the name/value pair as url encoded string. (optional)
* @param o can order on attributes (optional)
* @param s can search on attributes (optional)
* @return List<ProcessDefinition>
*/
@RequestLine("GET /API/bpm/process?p={p}&c={c}&f={f}&o={o}&s={s}")
@Headers({
"Accept: application/json",
})
List searchProcesses(@Param("p") Integer p, @Param("c") Integer c, @Param("f") List f,
@Param("o") String o, @Param("s") String s);
/**
* Finds Processes
* Similar to searchProcesses
but it also returns the http response headers .
* Finds Processes with pagination params and filters - can order (default is ASC) on `name`, `version`, `deploymentDate`,
* `deployedBy`, `activationState`, `configurationState`, `processId`, `displayName`,
* `lastUpdateDate`, `categoryId`, `label` - can search on `name`, `displayName` or `version` - can
* filter on `name`, `version`, `deploymentDate`, `deployedBy`, `activationState` with the value DISABLED or
* ENABLED, `configurationState` with the value UNRESOLVED, or RESOLVED, `processId`, `displayName`, `lastUpdateDate`,
* `categoryId`, `label`, `supervisor_id`
*
* @param p index of the page to display (required)
* @param c maximum number of elements to retrieve (required)
* @param f can filter on attributes with the format f={filter\\_name}={filter\\_value} with the name/value pair as url encoded string. (optional)
* @param o can order on attributes (optional)
* @param s can search on attributes (optional)
* @return A ApiResponse that wraps the response boyd and the http headers.
*/
@RequestLine("GET /API/bpm/process?p={p}&c={c}&f={f}&o={o}&s={s}")
@Headers({
"Accept: application/json",
})
ApiResponse> searchProcessesWithHttpInfo(@Param("p") Integer p, @Param("c") Integer c,
@Param("f") List f, @Param("o") String o, @Param("s") String s);
/**
* Finds Processes
* Finds Processes with pagination params and filters - can order (default is ASC) on `name`, `version`, `deploymentDate`,
* `deployedBy`, `activationState`, `configurationState`, `processId`, `displayName`,
* `lastUpdateDate`, `categoryId`, `label` - can search on `name`, `displayName` or `version` - can
* filter on `name`, `version`, `deploymentDate`, `deployedBy`, `activationState` with the value DISABLED or
* ENABLED, `configurationState` with the value UNRESOLVED, or RESOLVED, `processId`, `displayName`, `lastUpdateDate`,
* `categoryId`, `label`, `supervisor_id`
* Note, this is equivalent to the other searchProcesses
method,
* but with the query parameters collected into a single Map parameter. This
* is convenient for services with optional query parameters, especially when
* used with the {@link SearchProcessesQueryParams} class that allows for
* building up this map in a fluent style.
*
* @param queryParams Map of query parameters as name-value pairs
* The following elements may be specified in the query map:
*
* - p - index of the page to display (required)
* - c - maximum number of elements to retrieve (required)
* - f - can filter on attributes with the format f={filter\\_name}={filter\\_value} with the name/value pair as url encoded string.
* (optional)
* - o - can order on attributes (optional)
* - s - can search on attributes (optional)
*
* @return List<ProcessDefinition>
*/
@RequestLine("GET /API/bpm/process?p={p}&c={c}&f={f}&o={o}&s={s}")
@Headers({
"Accept: application/json",
})
List searchProcesses(@QueryMap(encoded = true) SearchProcessesQueryParams queryParams);
/**
* Finds Processes
* Finds Processes with pagination params and filters - can order (default is ASC) on `name`, `version`, `deploymentDate`,
* `deployedBy`, `activationState`, `configurationState`, `processId`, `displayName`,
* `lastUpdateDate`, `categoryId`, `label` - can search on `name`, `displayName` or `version` - can
* filter on `name`, `version`, `deploymentDate`, `deployedBy`, `activationState` with the value DISABLED or
* ENABLED, `configurationState` with the value UNRESOLVED, or RESOLVED, `processId`, `displayName`, `lastUpdateDate`,
* `categoryId`, `label`, `supervisor_id`
* Note, this is equivalent to the other searchProcesses
that receives the query parameters as a map,
* but this one also exposes the Http response headers
*
* @param queryParams Map of query parameters as name-value pairs
* The following elements may be specified in the query map:
*
* - p - index of the page to display (required)
* - c - maximum number of elements to retrieve (required)
* - f - can filter on attributes with the format f={filter\\_name}={filter\\_value} with the name/value pair as url encoded string.
* (optional)
* - o - can order on attributes (optional)
* - s - can search on attributes (optional)
*
* @return List<ProcessDefinition>
*/
@RequestLine("GET /API/bpm/process?p={p}&c={c}&f={f}&o={o}&s={s}")
@Headers({
"Accept: application/json",
})
ApiResponse> searchProcessesWithHttpInfo(
@QueryMap(encoded = true) SearchProcessesQueryParams queryParams);
/**
* A convenience class for generating query parameters for the
* searchProcesses
method in a fluent style.
*/
public static class SearchProcessesQueryParams extends HashMap {
public SearchProcessesQueryParams p(final Integer value) {
put("p", EncodingUtils.encode(value));
return this;
}
public SearchProcessesQueryParams c(final Integer value) {
put("c", EncodingUtils.encode(value));
return this;
}
public SearchProcessesQueryParams f(final List value) {
put("f", EncodingUtils.encodeCollection(value, "multi"));
return this;
}
public SearchProcessesQueryParams o(final String value) {
put("o", EncodingUtils.encode(value));
return this;
}
public SearchProcessesQueryParams s(final String value) {
put("s", EncodingUtils.encode(value));
return this;
}
}
/**
* Update the Process by ID
* Update the Process for the given ID
*
* @param id ID of the Process to return (required)
* @param processUpdateRequest Partial Process description (required)
*/
@RequestLine("PUT /API/bpm/process/{id}")
@Headers({
"Content-Type: application/json",
"Accept: application/json",
})
void updateProcessById(@Param("id") String id, ProcessUpdateRequest processUpdateRequest);
/**
* Update the Process by ID
* Similar to updateProcessById
but it also returns the http response headers .
* Update the Process for the given ID
*
* @param id ID of the Process to return (required)
* @param processUpdateRequest Partial Process description (required)
*/
@RequestLine("PUT /API/bpm/process/{id}")
@Headers({
"Content-Type: application/json",
"Accept: application/json",
})
ApiResponse updateProcessByIdWithHttpInfo(@Param("id") String id, ProcessUpdateRequest processUpdateRequest);
/**
* Update the Process Connector by Process ID
* Update the ProcessConnector for the given ID
*
* @param id ID of the process to update (required)
* @param connectorImplId ID of the Process Connector implementation to update (required)
* @param connectorImplVersion Version of the Process Connector implementation to update (required)
* @param updateProcessConnectorByProcessIdRequest Partial ProcessConnector description (required)
*/
@RequestLine("PUT /API/bpm/processConnector/{id}/{connectorImplId}/{connectorImplVersion}")
@Headers({
"Content-Type: application/json",
"Accept: application/json",
})
void updateProcessConnectorByProcessId(@Param("id") String id, @Param("connectorImplId") String connectorImplId,
@Param("connectorImplVersion") String connectorImplVersion,
UpdateProcessConnectorByProcessIdRequest updateProcessConnectorByProcessIdRequest);
/**
* Update the Process Connector by Process ID
* Similar to updateProcessConnectorByProcessId
but it also returns the http response headers .
* Update the ProcessConnector for the given ID
*
* @param id ID of the process to update (required)
* @param connectorImplId ID of the Process Connector implementation to update (required)
* @param connectorImplVersion Version of the Process Connector implementation to update (required)
* @param updateProcessConnectorByProcessIdRequest Partial ProcessConnector description (required)
*/
@RequestLine("PUT /API/bpm/processConnector/{id}/{connectorImplId}/{connectorImplVersion}")
@Headers({
"Content-Type: application/json",
"Accept: application/json",
})
ApiResponse updateProcessConnectorByProcessIdWithHttpInfo(@Param("id") String id,
@Param("connectorImplId") String connectorImplId,
@Param("connectorImplVersion") String connectorImplVersion,
UpdateProcessConnectorByProcessIdRequest updateProcessConnectorByProcessIdRequest);
/**
* Upload a bar file
* Upload a bar file
*
* @param file (optional)
* @return String
*/
@RequestLine("POST /portal/processUpload")
@Headers({
"Content-Type: multipart/form-data",
"Accept: application/json",
})
String uploadProcess(@Param("file") File file);
/**
* Upload a bar file
* Similar to uploadProcess
but it also returns the http response headers .
* Upload a bar file
*
* @param file (optional)
* @return A ApiResponse that wraps the response boyd and the http headers.
*/
@RequestLine("POST /portal/processUpload")
@Headers({
"Content-Type: multipart/form-data",
"Accept: application/json",
})
ApiResponse uploadProcessWithHttpInfo(@Param("file") File file);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy