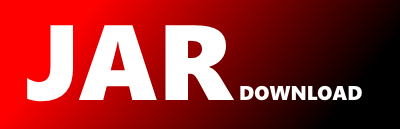
boofcv.factory.feature.detect.interest.FactoryDetectPoint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of boofcv-feature Show documentation
Show all versions of boofcv-feature Show documentation
BoofCV is an open source Java library for real-time computer vision and robotics applications.
/*
* Copyright (c) 2011-2018, Peter Abeles. All Rights Reserved.
*
* This file is part of BoofCV (http://boofcv.org).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package boofcv.factory.feature.detect.interest;
import boofcv.abst.feature.detect.extract.NonMaxSuppression;
import boofcv.abst.feature.detect.intensity.*;
import boofcv.abst.feature.detect.interest.ConfigFast;
import boofcv.abst.feature.detect.interest.ConfigGeneralDetector;
import boofcv.abst.filter.blur.BlurStorageFilter;
import boofcv.alg.feature.detect.intensity.FastCornerIntensity;
import boofcv.alg.feature.detect.intensity.GradientCornerIntensity;
import boofcv.alg.feature.detect.intensity.HessianBlobIntensity;
import boofcv.alg.feature.detect.interest.GeneralFeatureDetector;
import boofcv.factory.feature.detect.extract.FactoryFeatureExtractor;
import boofcv.factory.feature.detect.intensity.FactoryIntensityPoint;
import boofcv.factory.feature.detect.intensity.FactoryIntensityPointAlg;
import boofcv.factory.filter.blur.FactoryBlurFilter;
import boofcv.struct.image.ImageGray;
import boofcv.struct.image.ImageType;
import javax.annotation.Nullable;
/**
*
* Creates instances of {@link GeneralFeatureDetector}, which detects the location of
* point features inside an image.
*
*
*
* NOTE: Sometimes the image border is ignored and some times it is not. If feature intensities are not
* computed along the image border then it will be full of zeros. In that case the ignore border region
* needs to be increased for non-max suppression or else it might generate a false positive.
*
*
* @author Peter Abeles
*/
public class FactoryDetectPoint {
/**
* Detects Harris corners.
*
* @param configDetector Configuration for feature detector.
* @param weighted Is a Gaussian weight applied to the sample region? False is much faster.
* @param derivType Type of derivative image.
* @see boofcv.alg.feature.detect.intensity.HarrisCornerIntensity
*/
public static , D extends ImageGray>
GeneralFeatureDetector createHarris( @Nullable ConfigGeneralDetector configDetector,
boolean weighted, Class derivType) {
if( configDetector == null)
configDetector = new ConfigGeneralDetector();
GradientCornerIntensity cornerIntensity =
FactoryIntensityPointAlg.harris(configDetector.radius, 0.04f, weighted, derivType);
return createGeneral(cornerIntensity, configDetector);
}
/**
* Detects Shi-Tomasi corners.
*
* @param configDetector Configuration for feature detector.
* @param weighted Is a Gaussian weight applied to the sample region? False is much faster.
* @param derivType Type of derivative image.
* @see boofcv.alg.feature.detect.intensity.ShiTomasiCornerIntensity
*/
public static , D extends ImageGray>
GeneralFeatureDetector createShiTomasi( @Nullable ConfigGeneralDetector configDetector,
boolean weighted, Class derivType) {
if( configDetector == null)
configDetector = new ConfigGeneralDetector();
GradientCornerIntensity cornerIntensity =
FactoryIntensityPointAlg.shiTomasi(configDetector.radius, weighted, derivType);
return createGeneral(cornerIntensity, configDetector);
}
/**
* Detects Kitchen and Rosenfeld corners.
*
* @param configDetector Configuration for feature detector.
* @param derivType Type of derivative image.
* @see boofcv.alg.feature.detect.intensity.KitRosCornerIntensity
*/
public static , D extends ImageGray>
GeneralFeatureDetector createKitRos(@Nullable ConfigGeneralDetector configDetector, Class derivType) {
if( configDetector == null)
configDetector = new ConfigGeneralDetector();
GeneralFeatureIntensity intensity = new WrapperKitRosCornerIntensity<>(derivType);
return createGeneral(intensity, configDetector);
}
/**
* Creates a Fast corner detector.
*
* @param configFast Configuration for FAST feature detector
* @param configDetector Configuration for feature extractor.
* @param imageType Type of input image.
* @see FastCornerIntensity
*/
@SuppressWarnings("UnnecessaryLocalVariable")
public static , D extends ImageGray>
GeneralFeatureDetector createFast( @Nullable ConfigFast configFast ,
ConfigGeneralDetector configDetector , Class imageType) {
if( configFast == null )
configFast = new ConfigFast();
configFast.checkValidity();
ConfigGeneralDetector d = configDetector;
FastCornerIntensity alg = FactoryIntensityPointAlg.fast(configFast.pixelTol, configFast.minContinuous, imageType);
GeneralFeatureIntensity intensity = new WrapperFastCornerIntensity<>(alg);
ConfigGeneralDetector configExtract =
new ConfigGeneralDetector(d.maxFeatures,d.radius,d.threshold,0,true,false,true);
return createGeneral(intensity, configExtract);
}
/**
* Creates a median filter corner detector.
*
* @param configDetector Configuration for feature detector.
* @param imageType Type of input image.
* @see boofcv.alg.feature.detect.intensity.MedianCornerIntensity
*/
public static , D extends ImageGray>
GeneralFeatureDetector createMedian(@Nullable ConfigGeneralDetector configDetector, Class imageType) {
if( configDetector == null)
configDetector = new ConfigGeneralDetector();
BlurStorageFilter medianFilter = FactoryBlurFilter.median(ImageType.single(imageType), configDetector.radius);
GeneralFeatureIntensity intensity = new WrapperMedianCornerIntensity<>(medianFilter, imageType);
return createGeneral(intensity, configDetector);
}
/**
* Creates a Hessian based blob detector.
*
* @param type The type of Hessian based blob detector to use. DETERMINANT often works well.
* @param configDetector Configuration for feature detector.
* @param derivType Type of derivative image.
* @see HessianBlobIntensity
*/
public static , D extends ImageGray>
GeneralFeatureDetector createHessian(HessianBlobIntensity.Type type,
@Nullable ConfigGeneralDetector configDetector, Class derivType) {
if( configDetector == null)
configDetector = new ConfigGeneralDetector();
GeneralFeatureIntensity intensity = FactoryIntensityPoint.hessian(type, derivType);
return createGeneral(intensity, configDetector);
}
public static , D extends ImageGray>
GeneralFeatureDetector createGeneral(GradientCornerIntensity cornerIntensity,
ConfigGeneralDetector config) {
GeneralFeatureIntensity intensity = new WrapperGradientCornerIntensity<>(cornerIntensity);
return createGeneral(intensity, config);
}
public static , D extends ImageGray>
GeneralFeatureDetector createGeneral(GeneralFeatureIntensity intensity,
ConfigGeneralDetector config ) {
// create a copy since it's going to modify the detector config
ConfigGeneralDetector foo = new ConfigGeneralDetector();
foo.setTo(config);
config = foo;
config.ignoreBorder += config.radius;
NonMaxSuppression extractor = FactoryFeatureExtractor.nonmax(config);
GeneralFeatureDetector det = new GeneralFeatureDetector<>(intensity, extractor);
det.setMaxFeatures(config.maxFeatures);
return det;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy