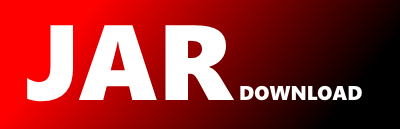
boofcv.factory.feature.detdesc.FactoryDetectDescribe Maven / Gradle / Ivy
Show all versions of boofcv-feature Show documentation
/*
* Copyright (c) 2011-2018, Peter Abeles. All Rights Reserved.
*
* This file is part of BoofCV (http://boofcv.org).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package boofcv.factory.feature.detdesc;
import boofcv.abst.feature.describe.ConfigSiftDescribe;
import boofcv.abst.feature.describe.ConfigSiftScaleSpace;
import boofcv.abst.feature.describe.ConfigSurfDescribe;
import boofcv.abst.feature.describe.DescribeRegionPoint;
import boofcv.abst.feature.detdesc.*;
import boofcv.abst.feature.detect.extract.NonMaxLimiter;
import boofcv.abst.feature.detect.extract.NonMaxSuppression;
import boofcv.abst.feature.detect.interest.ConfigFastHessian;
import boofcv.abst.feature.detect.interest.ConfigSiftDetector;
import boofcv.abst.feature.detect.interest.InterestPointDetector;
import boofcv.abst.feature.orientation.*;
import boofcv.alg.feature.describe.DescribePointSift;
import boofcv.alg.feature.describe.DescribePointSurf;
import boofcv.alg.feature.describe.DescribePointSurfMod;
import boofcv.alg.feature.describe.DescribePointSurfPlanar;
import boofcv.alg.feature.detdesc.CompleteSift;
import boofcv.alg.feature.detdesc.DetectDescribeSurfPlanar;
import boofcv.alg.feature.detect.interest.FastHessianFeatureDetector;
import boofcv.alg.feature.detect.interest.SiftScaleSpace;
import boofcv.alg.feature.orientation.OrientationHistogramSift;
import boofcv.alg.transform.ii.GIntegralImageOps;
import boofcv.factory.feature.describe.FactoryDescribePointAlgs;
import boofcv.factory.feature.detect.extract.FactoryFeatureExtractor;
import boofcv.factory.feature.detect.interest.FactoryInterestPointAlgs;
import boofcv.factory.feature.orientation.FactoryOrientationAlgs;
import boofcv.struct.feature.BrightFeature;
import boofcv.struct.feature.TupleDesc;
import boofcv.struct.image.GrayF32;
import boofcv.struct.image.ImageGray;
import boofcv.struct.image.ImageMultiBand;
import boofcv.struct.image.ImageType;
import javax.annotation.Nullable;
/**
* Creates instances of {@link DetectDescribePoint} for different feature detectors/describers.
*
* @author Peter Abeles
*/
public class FactoryDetectDescribe {
/**
* Creates a new SIFT feature detector and describer.
*
* @see CompleteSift
*
* @param config Configuration for the SIFT detector and descriptor.
* @return SIFT
*/
public static >
DetectDescribePoint sift( @Nullable ConfigCompleteSift config )
{
if( config == null )
config = new ConfigCompleteSift();
ConfigSiftScaleSpace configSS = config.scaleSpace;
ConfigSiftDetector configDetector = config.detector;
ConfigSiftOrientation configOri = config.orientation;
ConfigSiftDescribe configDesc = config.describe;
SiftScaleSpace scaleSpace = new SiftScaleSpace(
configSS.firstOctave,configSS.lastOctave,configSS.numScales,configSS.sigma0);
OrientationHistogramSift orientation = new OrientationHistogramSift<>(
configOri.histogramSize,configOri.sigmaEnlarge,GrayF32.class);
DescribePointSift describe = new DescribePointSift<>(
configDesc.widthSubregion,configDesc.widthGrid, configDesc.numHistogramBins,
configDesc.sigmaToPixels, configDesc.weightingSigmaFraction,
configDesc.maxDescriptorElementValue,GrayF32.class);
NonMaxSuppression nns = FactoryFeatureExtractor.nonmax(configDetector.extract);
NonMaxLimiter nonMax = new NonMaxLimiter(nns,configDetector.maxFeaturesPerScale);
CompleteSift dds = new CompleteSift(scaleSpace,configDetector.edgeR,nonMax,orientation,describe);
return new DetectDescribe_CompleteSift<>(dds);
}
/**
*
* Creates a SURF descriptor. SURF descriptors are invariant to illumination, orientation, and scale.
* BoofCV provides two variants. Creates a variant which is designed for speed at the cost of some stability.
* Different descriptors are created for color and gray-scale images.
*
*
*
* [1] Add tech report when its finished. See SURF performance web page for now.
*
*
* @see FastHessianFeatureDetector
* @see DescribePointSurf
* @see DescribePointSurfPlanar
*
* @param configDetector Configuration for SURF detector
* @param configDesc Configuration for SURF descriptor
* @param configOrientation Configuration for orientation
* @return SURF detector and descriptor
*/
public static , II extends ImageGray>
DetectDescribePoint surfFast( @Nullable ConfigFastHessian configDetector ,
@Nullable ConfigSurfDescribe.Speed configDesc,
@Nullable ConfigAverageIntegral configOrientation,
Class imageType) {
Class integralType = GIntegralImageOps.getIntegralType(imageType);
FastHessianFeatureDetector detector = FactoryInterestPointAlgs.fastHessian(configDetector);
DescribePointSurf describe = FactoryDescribePointAlgs.surfSpeed(configDesc, integralType);
OrientationIntegral orientation = FactoryOrientationAlgs.average_ii(configOrientation, integralType);
return new WrapDetectDescribeSurf<>(detector, orientation, describe);
}
/**
*
* Color version of SURF stable. Features are detected in a gray scale image, but the descriptors are
* computed using a color image. Each band in the page adds to the descriptor length. See
* {@link DetectDescribeSurfPlanar} for details.
*
*
* @see FastHessianFeatureDetector
* @see DescribePointSurf
* @see DescribePointSurfPlanar
*
* @param configDetector Configuration for SURF detector
* @param configDesc Configuration for SURF descriptor
* @param configOrientation Configuration for orientation
* @return SURF detector and descriptor
*/
public static , II extends ImageGray>
DetectDescribePoint surfColorFast( @Nullable ConfigFastHessian configDetector ,
@Nullable ConfigSurfDescribe.Speed configDesc,
@Nullable ConfigAverageIntegral configOrientation,
ImageType imageType) {
Class bandType = imageType.getImageClass();
Class integralType = GIntegralImageOps.getIntegralType(bandType);
FastHessianFeatureDetector detector = FactoryInterestPointAlgs.fastHessian(configDetector);
DescribePointSurf describe = FactoryDescribePointAlgs.surfSpeed(configDesc, integralType);
OrientationIntegral orientation = FactoryOrientationAlgs.average_ii(configOrientation, integralType);
if( imageType.getFamily() == ImageType.Family.PLANAR) {
DescribePointSurfPlanar describeMulti =
new DescribePointSurfPlanar<>(describe, imageType.getNumBands());
DetectDescribeSurfPlanar deteDesc =
new DetectDescribeSurfPlanar<>(detector, orientation, describeMulti);
return new SurfPlanar_to_DetectDescribePoint( deteDesc,bandType,integralType );
} else {
throw new IllegalArgumentException("Image type not supported");
}
}
/**
*
* Creates a SURF descriptor. SURF descriptors are invariant to illumination, orientation, and scale.
* BoofCV provides two variants. Creates a variant which is designed for stability. Different descriptors are
* created for color and gray-scale images.
*
*
*
* [1] Add tech report when its finished. See SURF performance web page for now.
*
*
* @see DescribePointSurfPlanar
* @see FastHessianFeatureDetector
* @see boofcv.alg.feature.describe.DescribePointSurfMod
*
* @param configDetector Configuration for SURF detector. Null for default.
* @param configDescribe Configuration for SURF descriptor. Null for default.
* @param configOrientation Configuration for region orientation. Null for default.
* @param imageType Specify type of input image.
* @return SURF detector and descriptor
*/
public static , II extends ImageGray>
DetectDescribePoint surfStable( @Nullable ConfigFastHessian configDetector,
@Nullable ConfigSurfDescribe.Stability configDescribe,
@Nullable ConfigSlidingIntegral configOrientation,
Class imageType ) {
Class integralType = GIntegralImageOps.getIntegralType(imageType);
FastHessianFeatureDetector detector = FactoryInterestPointAlgs.fastHessian(configDetector);
DescribePointSurfMod describe = FactoryDescribePointAlgs.surfStability(configDescribe, integralType);
OrientationIntegral orientation = FactoryOrientationAlgs.sliding_ii(configOrientation, integralType);
return new WrapDetectDescribeSurf( detector, orientation, describe );
}
/**
*
* Color version of SURF stable feature. Features are detected in a gray scale image, but the descriptors are
* computed using a color image. Each band in the page adds to the descriptor length. See
* {@link DetectDescribeSurfPlanar} for details.
*
*
* @see DescribePointSurfPlanar
* @see FastHessianFeatureDetector
* @see boofcv.alg.feature.describe.DescribePointSurfMod
*
* @param configDetector Configuration for SURF detector. Null for default.
* @param configDescribe Configuration for SURF descriptor. Null for default.
* @param configOrientation Configuration for region orientation. Null for default.
* @param imageType Specify type of color input image.
* @return SURF detector and descriptor
*/
public static , II extends ImageGray>
DetectDescribePoint surfColorStable( @Nullable ConfigFastHessian configDetector,
@Nullable ConfigSurfDescribe.Stability configDescribe,
@Nullable ConfigSlidingIntegral configOrientation,
ImageType imageType ) {
Class bandType = imageType.getImageClass();
Class integralType = GIntegralImageOps.getIntegralType(bandType);
FastHessianFeatureDetector detector = FactoryInterestPointAlgs.fastHessian(configDetector);
DescribePointSurfMod describe = FactoryDescribePointAlgs.surfStability(configDescribe, integralType);
OrientationIntegral orientation = FactoryOrientationAlgs.sliding_ii(configOrientation, integralType);
if( imageType.getFamily() == ImageType.Family.PLANAR) {
DescribePointSurfPlanar describeMulti =
new DescribePointSurfPlanar<>(describe, imageType.getNumBands());
DetectDescribeSurfPlanar deteDesc =
new DetectDescribeSurfPlanar<>(detector, orientation, describeMulti);
return new SurfPlanar_to_DetectDescribePoint( deteDesc,bandType,integralType );
} else {
throw new IllegalArgumentException("Image type not supported");
}
}
/**
* Given independent algorithms for feature detection, orientation, and describing, create a new
* {@link DetectDescribePoint}.
*
* @param detector Feature detector
* @param orientation Orientation estimation. Optionally, can be null.
* @param describe Feature descriptor
* @return {@link DetectDescribePoint}.
*/
public static , D extends TupleDesc>
DetectDescribePoint fuseTogether( InterestPointDetector detector,
@Nullable OrientationImage orientation,
DescribeRegionPoint describe) {
return new DetectDescribeFusion<>(detector, orientation, describe);
}
}