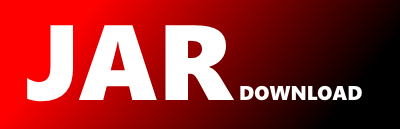
boofcv.alg.segmentation.fh04.impl.FhEdgeWeights4_PLU8 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of boofcv-feature Show documentation
Show all versions of boofcv-feature Show documentation
BoofCV is an open source Java library for real-time computer vision and robotics applications.
/*
* Copyright (c) 2011-2017, Peter Abeles. All Rights Reserved.
*
* This file is part of BoofCV (http://boofcv.org).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package boofcv.alg.segmentation.fh04.impl;
import boofcv.alg.segmentation.fh04.FhEdgeWeights;
import boofcv.struct.image.GrayU8;
import boofcv.struct.image.ImageType;
import boofcv.struct.image.Planar;
import org.ddogleg.struct.FastQueue;
import static boofcv.alg.segmentation.fh04.SegmentFelzenszwalbHuttenlocher04.Edge;
/**
* Computes edge weight as the F-norm different in pixel value for {@link Planar} images.
* A 4-connect neighborhood is considered.
*
*
* WARNING: Do not modify. Automatically generated by {@link GenerateFhEdgeWeights_PL}.
*
*
* @author Peter Abeles
*/
public class FhEdgeWeights4_PLU8 implements FhEdgeWeights> {
int pixelColor[];
int numBands;
public FhEdgeWeights4_PLU8(int numBands) {
this.numBands = numBands;
pixelColor = new int[numBands];
}
@Override
public void process(Planar input,
FastQueue edges) {
edges.reset();
int w = input.width-1;
int h = input.height-1;
// First consider the inner pixels
for( int y = 0; y < h; y++ ) {
int indexSrc = input.startIndex + y*input.stride + 0;
int indexDst = + y*input.width + 0;
for( int x = 0; x < w; x++ , indexSrc++ , indexDst++ ) {
int weight1=0,weight2=0;
for( int i = 0; i < numBands; i++ ) {
GrayU8 band = input.getBand(i);
int color0 = band.data[indexSrc]& 0xFF; // (x,y)
int color1 = band.data[indexSrc+1]& 0xFF; // (x+1,y)
int color2 = band.data[indexSrc+input.stride]& 0xFF; // (x,y+1)
int diff1 = color0-color1;
int diff2 = color0-color2;
weight1 += diff1*diff1;
weight2 += diff2*diff2;
}
Edge e1 = edges.grow();
Edge e2 = edges.grow();
e1.sortValue = (float)Math.sqrt(weight1);
e1.indexA = indexDst;
e1.indexB = indexDst+1;
e2.sortValue = (float)Math.sqrt(weight2);
e2.indexA = indexDst;
e2.indexB = indexDst+input.width;
}
}
// Handle border pixels
for( int y = 0; y < h; y++ ) {
checkAround(w,y,input,edges);
}
for( int x = 0; x < w; x++ ) {
checkAround(x,h,input,edges);
}
}
private void checkAround( int x , int y ,
Planar input ,
FastQueue edges )
{
int indexSrc = input.startIndex + y*input.stride + x;
int indexA = y*input.width + x;
for( int i = 0; i < numBands; i++ ) {
GrayU8 band = input.getBand(i);
pixelColor[i] = band.data[indexSrc]& 0xFF;
}
check(x+1, y, pixelColor,indexA,input,edges);
check(x ,y+1,pixelColor,indexA,input,edges);
}
private void check( int x , int y , int color0[] , int indexA,
Planar input ,
FastQueue edges ) {
if( !input.isInBounds(x,y) )
return;
int indexSrc = input.startIndex + y*input.stride + x;
int indexB = + y*input.width + x;
float weight = 0;
for( int i = 0; i < numBands; i++ ) {
GrayU8 band = input.getBand(i);
int color = band.data[indexSrc]& 0xFF;
int diff = color0[i]-color;
weight += diff*diff;
}
Edge e1 = edges.grow();
e1.sortValue = (float)Math.sqrt(weight);
e1.indexA = indexA;
e1.indexB = indexB;
}
@Override
public ImageType> getInputType() {
return ImageType.pl(3,GrayU8.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy