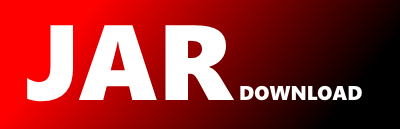
boofcv.abst.feature.describe.SurfPlanar_to_DescribeRegionPoint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of boofcv-feature Show documentation
Show all versions of boofcv-feature Show documentation
BoofCV is an open source Java library for real-time computer vision and robotics applications.
/*
* Copyright (c) 2011-2020, Peter Abeles. All Rights Reserved.
*
* This file is part of BoofCV (http://boofcv.org).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package boofcv.abst.feature.describe;
import boofcv.alg.feature.describe.DescribePointSurfPlanar;
import boofcv.alg.transform.ii.GIntegralImageOps;
import boofcv.core.image.GConvertImage;
import boofcv.core.image.GeneralizedImageOps;
import boofcv.struct.feature.BrightFeature;
import boofcv.struct.image.ImageGray;
import boofcv.struct.image.ImageType;
import boofcv.struct.image.Planar;
/**
* Wrapper around {@link DescribePointSurfPlanar} for {@link DescribeRegionPoint}
*
* @param Image band type
* @param Integral image type
*
* @author Peter Abeles
*/
public class SurfPlanar_to_DescribeRegionPoint, II extends ImageGray>
implements DescribeRegionPoint,BrightFeature>
{
DescribePointSurfPlanar alg;
T gray;
II grayII;
Planar bandII;
ImageType> imageType;
final double canonicalRadius;
public SurfPlanar_to_DescribeRegionPoint(DescribePointSurfPlanar alg,
Class imageType, Class integralType ) {
this.alg = alg;
gray = GeneralizedImageOps.createSingleBand(imageType, 1, 1);
grayII = GeneralizedImageOps.createSingleBand(integralType,1,1);
bandII = new Planar<>(integralType, 1, 1, alg.getNumBands());
this.imageType = ImageType.pl(alg.getNumBands(), imageType);
canonicalRadius = alg.getDescribe().getCanonicalWidth()/2.0;
}
@Override
public void setImage(Planar image) {
gray.reshape(image.width,image.height);
grayII.reshape(image.width,image.height);
bandII.reshape(image.width,image.height);
GConvertImage.average(image, gray);
GIntegralImageOps.transform(gray, grayII);
for( int i = 0; i < image.getNumBands(); i++)
GIntegralImageOps.transform(image.getBand(i), bandII.getBand(i));
alg.setImage(grayII,bandII);
}
@Override
public boolean process(double x, double y, double orientation, double radius, BrightFeature description) {
double scale = radius/canonicalRadius;
alg.describe(x,y,orientation, scale, description);
return true;
}
@Override
public boolean isScalable() {
return true;
}
@Override
public boolean isOriented() {
return true;
}
@Override
public ImageType> getImageType() {
return imageType;
}
@Override
public double getCanonicalWidth() {
return alg.getDescribe().getCanonicalWidth();
}
@Override
public BrightFeature createDescription() {
return alg.createDescription();
}
@Override
public Class getDescriptionType() {
return BrightFeature.class;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy