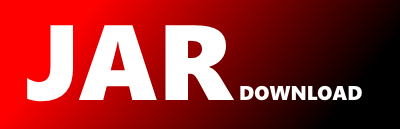
boofcv.alg.feature.associate.AssociateNearestNeighbor_MT Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of boofcv-feature Show documentation
Show all versions of boofcv-feature Show documentation
BoofCV is an open source Java library for real-time computer vision and robotics applications.
/*
* Copyright (c) 2011-2020, Peter Abeles. All Rights Reserved.
*
* This file is part of BoofCV (http://boofcv.org).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package boofcv.alg.feature.associate;
import boofcv.concurrency.BoofConcurrency;
import boofcv.concurrency.IntRangeConsumer;
import boofcv.struct.feature.AssociatedIndex;
import org.ddogleg.nn.NearestNeighbor;
import org.ddogleg.nn.NnData;
import org.ddogleg.struct.FastAccess;
import org.ddogleg.struct.FastQueue;
import java.util.ArrayList;
import java.util.List;
/**
* Parallel associate version of {@link AssociateNearestNeighbor_ST}.
*
* @author Peter Abeles
*/
public class AssociateNearestNeighbor_MT
extends AssociateNearestNeighbor
{
// Nearest Neighbor algorithm and storage for the results
private final List available = new ArrayList<>();
public AssociateNearestNeighbor_MT(NearestNeighbor alg) {
super(alg);
}
@Override
public void setSource(FastAccess listSrc) {
this.sizeSrc = listSrc.size;
alg.setPoints((List)listSrc.toList(),true);
}
@Override
public void setDestination(FastAccess listDst) {
this.listDst = listDst;
}
@Override
public void associate() {
matchesAll.resize(listDst.size);
matchesAll.reset();
if( scoreRatioThreshold >= 1.0 ) {
BoofConcurrency.loopBlocks(0,listDst.size,new InnerConsumer() {
@Override
public void innerAccept(Helper h, int index0, int index1) {
for (int i = index0; i < index1; i++) {
if (!h.search.findNearest(listDst.data[i], maxDistance, h.result))
continue;
h.matches.grow().setAssociation(h.result.index, i, h.result.distance);
}
}
});
} else {
BoofConcurrency.loopBlocks(0,listDst.size,new InnerConsumer() {
@Override
public void innerAccept(Helper h, int index0, int index1) {
for (int i = index0; i < index1; i++) {
h.search.findNearest(listDst.data[i], maxDistance, 2, h.result2);
if (h.result2.size == 1) {
NnData r = h.result2.getTail();
h.matches.grow().setAssociation(r.index, i, r.distance);
} else if (h.result2.size == 2) {
NnData r0 = h.result2.get(0);
NnData r1 = h.result2.get(1);
// ensure that r0 is the closest
if (r0.distance > r1.distance) {
NnData tmp = r0;
r0 = r1;
r1 = tmp;
}
double foundRatio = ratioUsesSqrt ? Math.sqrt(r0.distance) / Math.sqrt(r1.distance) : r0.distance / r1.distance;
if (foundRatio <= scoreRatioThreshold) {
h.matches.grow().setAssociation(r0.index, i, r0.distance);
}
} else if (h.result2.size != 0) {
throw new RuntimeException("BUG! 0,1,2 are acceptable not " + h.result2.size);
}
}
}
});
}
}
/**
* Consumes a block of matches
*/
private abstract class InnerConsumer implements IntRangeConsumer {
@Override
public void accept(int index0, int index1) {
// Recycle a helper if possible
Helper h;
synchronized (available) {
if( available.isEmpty() ) {
h = new Helper();
} else {
h = available.remove(available.size()-1);
h.initialize();
}
}
// do the for loop inside of this and pass in the helper for this block
innerAccept(h,index0,index1);
// synchronize the data again
synchronized (matchesAll) {
for (int i = 0; i < h.matches.size; i++)
{
AssociatedIndex a = h.matches.get(i);
matchesAll.grow().set(a);
}
}
// Put the helper back into the available list
synchronized (available) {
available.add(h);
}
}
public abstract void innerAccept(Helper h, int index0, int index1);
}
/**
* Contains data structures for a specific thread.
*/
private class Helper {
NearestNeighbor.Search search;
FastQueue matches = new FastQueue<>(10, AssociatedIndex::new);
private NnData result = new NnData<>();
private FastQueue> result2 = new FastQueue(NnData::new);
Helper() {
search = alg.createSearch();
}
public void initialize() {
matches.reset();
result2.reset();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy