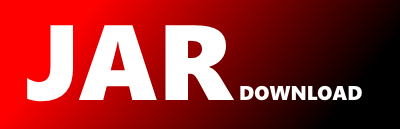
boofcv.factory.feature.detect.line.FactoryDetectLine Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of boofcv-feature Show documentation
Show all versions of boofcv-feature Show documentation
BoofCV is an open source Java library for real-time computer vision and robotics applications.
/*
* Copyright (c) 2011-2019, Peter Abeles. All Rights Reserved.
*
* This file is part of BoofCV (http://boofcv.org).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package boofcv.factory.feature.detect.line;
import boofcv.abst.feature.detect.line.*;
import boofcv.abst.filter.binary.InputToBinary;
import boofcv.alg.feature.detect.line.HoughTransformBinary;
import boofcv.alg.feature.detect.line.HoughTransformGradient;
import boofcv.alg.filter.derivative.GImageDerivativeOps;
import boofcv.factory.filter.binary.ConfigThreshold;
import boofcv.factory.filter.binary.FactoryThresholdBinary;
import boofcv.factory.filter.binary.ThresholdType;
import boofcv.struct.image.ImageGray;
import javax.annotation.Nullable;
/**
* Factory for creating high level implementations of {@link DetectLine} and {@Link DetectLineSegment}. For more access
* to internal data structures see {@link FactoryDetectLineAlgs}.
*
* @author Peter Abeles
*/
public class FactoryDetectLine {
/**
* Detects line segments inside an image using the {@link DetectLineSegmentsGridRansac} algorithm.
*
* @see DetectLineSegmentsGridRansac
*
* @param config Configuration for line detector
* @param imageType Type of single band input image.
* @return Line segment detector
*/
public static >
DetectLineSegment lineRansac( @Nullable ConfigLineRansac config,
Class imageType ) {
Class derivType = GImageDerivativeOps.getDerivativeType(imageType);
return FactoryDetectLineAlgs.lineRansac(config,imageType,derivType);
}
/**
* Detects lines using a foot of norm parametrization and sub images to reduce degenerate
* configurations, see {@link DetectLineHoughFootSubimage} for details.
*
* @see DetectLineHoughFootSubimage
*
* @param config Configuration for line detector. If null then default will be used.
* @param imageType input image type
* @return Line detector.
*/
public static >
DetectLine houghLineFootSub(@Nullable ConfigHoughFootSubimage config , Class imageType ) {
Class derivType = GImageDerivativeOps.getDerivativeType(imageType);
DetectEdgeLines alg = FactoryDetectLineAlgs.houghFootSub(config,derivType);
return new DetectEdgeLinesToLines(alg,imageType,derivType);
}
/**
* Used to find edges along the side of objects. Image gradient is found and thresholded. The pixel coordinate
* of each active pixel and its gradient is then used to estimate the parameter of a line.
* Uses {@link HoughTransformGradient} with {@link boofcv.alg.feature.detect.line.HoughParametersFootOfNorm}
*
* @param configHough Configuration for hough binary transform.
* @param configParam Configuration for polar parameters
* @param imageType type of input image
* @return Line detector.
*/
public static >
DetectLine houghLineFoot(@Nullable ConfigHoughGradient configHough ,
@Nullable ConfigParamFoot configParam , Class imageType )
{
if( configHough == null )
configHough = new ConfigHoughGradient();
if( configParam == null )
configParam = new ConfigParamFoot();
Class derivType = GImageDerivativeOps.getDerivativeType(imageType);
HoughTransformGradient hough = FactoryDetectLineAlgs.houghLineFoot(configHough,configParam,derivType);
HoughGradient_to_DetectLine detector = new HoughGradient_to_DetectLine(hough,imageType);
detector.setThresholdEdge(configHough.thresholdEdge);
return detector;
}
/**
* Used to find edges along the side of objects. Image gradient is found and thresholded. The pixel coordinate
* of each active pixel and its gradient is then used to estimate the parameter of a line.
* Uses {@link HoughTransformGradient} with {@link boofcv.alg.feature.detect.line.HoughParametersPolar}
*
* @param configHough Configuration for hough binary transform.
* @param configParam Configuration for polar parameters
* @param imageType type of input image
* @return Line detector.
*/
public static >
DetectLine houghLinePolar( @Nullable ConfigHoughGradient configHough ,
@Nullable ConfigParamPolar configParam ,
Class imageType )
{
if( configHough == null )
configHough = new ConfigHoughGradient();
if( configParam == null )
configParam = new ConfigParamPolar();
Class derivType = GImageDerivativeOps.getDerivativeType(imageType);
HoughTransformGradient hough = FactoryDetectLineAlgs.houghLinePolar(configHough,configParam,derivType);
HoughGradient_to_DetectLine detector = new HoughGradient_to_DetectLine(hough,imageType);
detector.setThresholdEdge(configHough.thresholdEdge);
return detector;
}
/**
* Line detector which converts image into a binary one and assumes every pixel with a value of 1 belongs
* to a line. Uses {@link HoughTransformBinary} with {@link boofcv.alg.feature.detect.line.HoughParametersPolar}
*
* @param configHough Configuration for hough binary transform.
* @param configParam Configuration for polar parameters
* @param configThreshold Configuration for image thresholding
* @param imageType type of input image
* @return Line detector.
*/
public static >
DetectLine houghLinePolar(@Nullable ConfigHoughBinary configHough ,
@Nullable ConfigParamPolar configParam ,
@Nullable ConfigThreshold configThreshold ,
Class imageType )
{
if( configHough == null )
configHough = new ConfigHoughBinary();
if( configParam == null )
configParam = new ConfigParamPolar();
if( configThreshold == null )
configThreshold = ConfigThreshold.global(ThresholdType.GLOBAL_OTSU);
HoughTransformBinary hough = FactoryDetectLineAlgs.houghLinePolar(configHough,configParam);
InputToBinary thresholder = FactoryThresholdBinary.threshold(configThreshold,imageType);
return new HoughBinary_to_DetectLine<>(hough,thresholder);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy