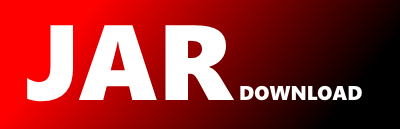
boofcv.alg.cloud.PointCloudUtils_F32 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of boofcv-geo Show documentation
Show all versions of boofcv-geo Show documentation
BoofCV is an open source Java library for real-time computer vision and robotics applications.
/*
* Copyright (c) 2021, Peter Abeles. All Rights Reserved.
*
* This file is part of BoofCV (http://boofcv.org).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package boofcv.alg.cloud;
import javax.annotation.Generated;
import boofcv.misc.BoofLambdas;
import boofcv.struct.Point3dRgbI_F32;
import georegression.helper.KdTreePoint3D_F32;
import georegression.struct.point.Point3D_F32;
import org.ddogleg.nn.FactoryNearestNeighbor;
import org.ddogleg.nn.NearestNeighbor;
import org.ddogleg.nn.NnData;
import org.ddogleg.struct.DogArray;
import org.ddogleg.struct.DogArray_I32;
import org.jetbrains.annotations.Nullable;
import java.util.List;
/**
* Various utility functions for working with point clouds with a float type of float.
*
* @author Peter Abeles
*/
@Generated("boofcv.alg.cloud.PointCloudUtils_F64")
public class PointCloudUtils_F32 {
/**
* Creates a new list of points while filtering out points
*/
public static DogArray filter( AccessPointIndex accessPoint,
AccessColorIndex accessColor,
int size,
BoofLambdas.FilterInt filter,
@Nullable DogArray output ) {
if (output == null)
output = new DogArray<>(Point3dRgbI_F32::new);
output.reset();
// Guess how much memory is needed
output.reserve(size/5);
Point3dRgbI_F32 p = output.grow();
for (int pointIdx = 0; pointIdx < size; pointIdx++) {
if (!filter.keep(pointIdx))
continue;
accessPoint.getPoint(pointIdx, p);
p.rgb = accessColor.getRGB(pointIdx);
p = output.grow();
}
output.removeTail();
return output;
}
/**
* Automatically rescales the point cloud based so that it has a standard deviation of 'target'
*
* @param cloud The point cloud
* @param target The desired standard deviation of the cloud. Try 100
* @return The selected scale factor
*/
public static float autoScale( List cloud, float target ) {
Point3D_F32 mean = new Point3D_F32();
Point3D_F32 stdev = new Point3D_F32();
statistics(cloud, mean, stdev);
float scale = target/Math.max(Math.max(stdev.x, stdev.y), stdev.z);
int N = cloud.size();
for (int i = 0; i < N; i++) {
cloud.get(i).scale(scale);
}
return scale;
}
/**
* Computes the mean and standard deviation of each axis in the point cloud computed in dependently
*
* @param cloud (Input) Cloud
* @param mean (Output) mean of each axis
* @param stdev (Output) standard deviation of each axis
*/
public static void statistics( List cloud, Point3D_F32 mean, Point3D_F32 stdev ) {
final int N = cloud.size();
for (int i = 0; i < N; i++) {
Point3D_F32 p = cloud.get(i);
mean.x += p.x/N;
mean.y += p.y/N;
mean.z += p.z/N;
}
for (int i = 0; i < N; i++) {
Point3D_F32 p = cloud.get(i);
float dx = p.x - mean.x;
float dy = p.y - mean.y;
float dz = p.z - mean.z;
stdev.x += dx*dx/N;
stdev.y += dy*dy/N;
stdev.z += dz*dz/N;
}
stdev.x = (float)Math.sqrt(stdev.x);
stdev.y = (float)Math.sqrt(stdev.y);
stdev.z = (float)Math.sqrt(stdev.z);
}
/**
* Prunes points from the point cloud if they have very few neighbors
*
* @param cloud Point cloud
* @param minNeighbors Minimum number of neighbors for it to not be pruned
* @param radius search distance for neighbors
*/
public static void prune( List cloud, int minNeighbors, float radius ) {
if (minNeighbors < 0)
throw new IllegalArgumentException("minNeighbors must be >= 0");
NearestNeighbor nn = FactoryNearestNeighbor.kdtree(new KdTreePoint3D_F32());
NearestNeighbor.Search search = nn.createSearch();
nn.setPoints(cloud, false);
DogArray> results = new DogArray<>(NnData::new);
// It will always find itself
minNeighbors += 1;
// distance is Euclidean squared
radius *= radius;
for (int i = cloud.size() - 1; i >= 0; i--) {
search.findNearest(cloud.get(i), radius, minNeighbors, results);
if (results.size < minNeighbors) {
cloud.remove(i);
}
}
}
/**
* Prunes points from the point cloud if they have very few neighbors
*
* @param cloud Point cloud
* @param colors Color of each point.
* @param minNeighbors Minimum number of neighbors for it to not be pruned
* @param radius search distance for neighbors
*/
public static void prune( List cloud, DogArray_I32 colors, int minNeighbors, float radius ) {
if (minNeighbors < 0)
throw new IllegalArgumentException("minNeighbors must be >= 0");
NearestNeighbor nn = FactoryNearestNeighbor.kdtree(new KdTreePoint3D_F32());
NearestNeighbor.Search search = nn.createSearch();
nn.setPoints(cloud, false);
DogArray> results = new DogArray<>(NnData::new);
// It will always find itself
minNeighbors += 1;
// distance is Euclidean squared
radius *= radius;
for (int i = cloud.size() - 1; i >= 0; i--) {
search.findNearest(cloud.get(i), radius, minNeighbors, results);
if (results.size < minNeighbors) {
cloud.remove(i);
colors.remove(i);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy