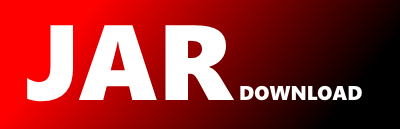
boofcv.alg.transform.ii.impl.ImplIntegralImageConvolve Maven / Gradle / Ivy
Show all versions of boofcv-ip Show documentation
/*
* Copyright (c) 2011-2019, Peter Abeles. All Rights Reserved.
*
* This file is part of BoofCV (http://boofcv.org).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package boofcv.alg.transform.ii.impl;
import boofcv.alg.transform.ii.IntegralKernel;
import boofcv.struct.ImageRectangle;
import boofcv.struct.image.GrayF32;
import boofcv.struct.image.GrayF64;
import boofcv.struct.image.GrayS32;
import boofcv.struct.image.GrayS64;
import javax.annotation.Generated;
import static boofcv.alg.transform.ii.impl.ImplIntegralImageOps.block_zero;
//CONCURRENT_INLINE import boofcv.concurrency.BoofConcurrency;
/**
*
* Compute the integral image for different types of input images.
*
*
*
* DO NOT MODIFY. This code was automatically generated by GenerateImplIntegralImageConvolve.
*
* @author Peter Abeles
*/
@Generated("boofcv.alg.transform.ii.impl.GenerateImplIntegralImageConvolve")
public class ImplIntegralImageConvolve {
public static void convolve( GrayF32 integral ,
IntegralKernel kernel,
GrayF32 output )
{
//CONCURRENT_BELOW BoofConcurrency.loopFor(0, integral.height, y -> {
for( int y = 0; y < integral.height; y++ ) {
for( int x = 0; x < integral.width; x++ ) {
float total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
}
//CONCURRENT_ABOVE });
}
public static void convolveBorder( GrayF32 integral ,
IntegralKernel kernel,
GrayF32 output , int borderX , int borderY )
{
//CONCURRENT_BELOW BoofConcurrency.loopFor(0, integral.width, x -> {
for( int x = 0; x < integral.width; x++ ) {
for( int y = 0; y < borderY; y++ ) {
float total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
for( int y = integral.height-borderY; y < integral.height; y++ ) {
float total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
}
//CONCURRENT_ABOVE });
int endY = integral.height-borderY;
//CONCURRENT_BELOW BoofConcurrency.loopFor(borderY, endY, y -> {
for( int y = borderY; y < endY; y++ ) {
for( int x = 0; x < borderX; x++ ) {
float total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
for( int x = integral.width-borderX; x < integral.width; x++ ) {
float total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
}
//CONCURRENT_ABOVE });
}
public static void convolve( GrayS32 integral ,
IntegralKernel kernel,
GrayS32 output )
{
//CONCURRENT_BELOW BoofConcurrency.loopFor(0, integral.height, y -> {
for( int y = 0; y < integral.height; y++ ) {
for( int x = 0; x < integral.width; x++ ) {
int total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
}
//CONCURRENT_ABOVE });
}
public static void convolveBorder( GrayS32 integral ,
IntegralKernel kernel,
GrayS32 output , int borderX , int borderY )
{
//CONCURRENT_BELOW BoofConcurrency.loopFor(0, integral.width, x -> {
for( int x = 0; x < integral.width; x++ ) {
for( int y = 0; y < borderY; y++ ) {
int total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
for( int y = integral.height-borderY; y < integral.height; y++ ) {
int total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
}
//CONCURRENT_ABOVE });
int endY = integral.height-borderY;
//CONCURRENT_BELOW BoofConcurrency.loopFor(borderY, endY, y -> {
for( int y = borderY; y < endY; y++ ) {
for( int x = 0; x < borderX; x++ ) {
int total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
for( int x = integral.width-borderX; x < integral.width; x++ ) {
int total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
}
//CONCURRENT_ABOVE });
}
public static void convolve( GrayF64 integral ,
IntegralKernel kernel,
GrayF64 output )
{
//CONCURRENT_BELOW BoofConcurrency.loopFor(0, integral.height, y -> {
for( int y = 0; y < integral.height; y++ ) {
for( int x = 0; x < integral.width; x++ ) {
double total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
}
//CONCURRENT_ABOVE });
}
public static void convolveBorder( GrayF64 integral ,
IntegralKernel kernel,
GrayF64 output , int borderX , int borderY )
{
//CONCURRENT_BELOW BoofConcurrency.loopFor(0, integral.width, x -> {
for( int x = 0; x < integral.width; x++ ) {
for( int y = 0; y < borderY; y++ ) {
double total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
for( int y = integral.height-borderY; y < integral.height; y++ ) {
double total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
}
//CONCURRENT_ABOVE });
int endY = integral.height-borderY;
//CONCURRENT_BELOW BoofConcurrency.loopFor(borderY, endY, y -> {
for( int y = borderY; y < endY; y++ ) {
for( int x = 0; x < borderX; x++ ) {
double total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
for( int x = integral.width-borderX; x < integral.width; x++ ) {
double total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
}
//CONCURRENT_ABOVE });
}
public static void convolve( GrayS64 integral ,
IntegralKernel kernel,
GrayS64 output )
{
//CONCURRENT_BELOW BoofConcurrency.loopFor(0, integral.height, y -> {
for( int y = 0; y < integral.height; y++ ) {
for( int x = 0; x < integral.width; x++ ) {
long total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
}
//CONCURRENT_ABOVE });
}
public static void convolveBorder( GrayS64 integral ,
IntegralKernel kernel,
GrayS64 output , int borderX , int borderY )
{
//CONCURRENT_BELOW BoofConcurrency.loopFor(0, integral.width, x -> {
for( int x = 0; x < integral.width; x++ ) {
for( int y = 0; y < borderY; y++ ) {
long total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
for( int y = integral.height-borderY; y < integral.height; y++ ) {
long total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
}
//CONCURRENT_ABOVE });
int endY = integral.height-borderY;
//CONCURRENT_BELOW BoofConcurrency.loopFor(borderY, endY, y -> {
for( int y = borderY; y < endY; y++ ) {
for( int x = 0; x < borderX; x++ ) {
long total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
for( int x = integral.width-borderX; x < integral.width; x++ ) {
long total = 0;
for( int i = 0; i < kernel.blocks.length; i++ ) {
ImageRectangle b = kernel.blocks[i];
total += block_zero(integral,x+b.x0,y+b.y0,x+b.x1,y+b.y1)*kernel.scales[i];
}
output.set(x,y,total);
}
}
//CONCURRENT_ABOVE });
}
}