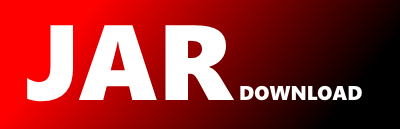
boofcv.abst.fiducial.AztecCodePreciseDetector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of boofcv-recognition Show documentation
Show all versions of boofcv-recognition Show documentation
BoofCV is an open source Java library for real-time computer vision and robotics applications.
The newest version!
/*
* Copyright (c) 2022, Peter Abeles. All Rights Reserved.
*
* This file is part of BoofCV (http://boofcv.org).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package boofcv.abst.fiducial;
import boofcv.abst.filter.binary.InputToBinary;
import boofcv.alg.fiducial.aztec.AztecCode;
import boofcv.alg.fiducial.aztec.AztecDecoderImage;
import boofcv.alg.fiducial.aztec.AztecFinderPatternDetector;
import boofcv.alg.fiducial.aztec.AztecPyramid;
import boofcv.alg.shapes.polygon.DetectPolygonBinaryGrayRefine;
import boofcv.misc.BoofMiscOps;
import boofcv.struct.image.GrayU8;
import boofcv.struct.image.ImageGray;
import lombok.Getter;
import org.ddogleg.struct.DogArray;
import org.ddogleg.struct.VerbosePrint;
import org.jetbrains.annotations.Nullable;
import java.io.PrintStream;
import java.util.ArrayList;
import java.util.List;
import java.util.Set;
/**
* An Aztec Code detector which is designed to detect finder pattern corners to a high degree of precision.
*/
public class AztecCodePreciseDetector> implements AztecCodeDetector, VerbosePrint {
/** Converts input image into a binary image */
@Getter InputToBinary inputToBinary;
/** Detects pyramidal finder patterns */
@Getter AztecFinderPatternDetector detectorPyramids;
/** Decodes a marker given its finder pattern */
@Getter AztecDecoderImage decoder;
// Storage for thresholded input image
@Getter GrayU8 binary = new GrayU8(1, 1);
// Specifies type of input image
Class imageType;
// Storage for all markers, successful and failures
final DogArray allMarkers = new DogArray<>(AztecCode::new, AztecCode::reset);
// Successfully decoded markers in the image
final List detected = new ArrayList<>();
// Candidate markers which could not be decoded
final List failed = new ArrayList<>();
@Nullable PrintStream verbose = null;
public AztecCodePreciseDetector( InputToBinary inputToBinary,
DetectPolygonBinaryGrayRefine squareDetector,
Class imageType ) {
this.imageType = imageType;
this.inputToBinary = inputToBinary;
this.detectorPyramids = new AztecFinderPatternDetector<>(squareDetector);
this.decoder = new AztecDecoderImage<>(imageType);
}
@Override public void process( T gray ) {
// Reset and initialize
allMarkers.reset();
detected.clear();
failed.clear();
// Detect finder patterns
inputToBinary.process(gray, binary);
detectorPyramids.process(gray, binary);
// Attempt to decode candidate markers
List pyramids = detectorPyramids.getFound().toList();
if (verbose != null) verbose.println("Total pyramids found: " + pyramids.size());
for (int locatorIdx = 0; locatorIdx < pyramids.size(); locatorIdx++) {
AztecPyramid pyramid = pyramids.get(locatorIdx);
if (verbose != null) verbose.println("Considering pyramid at: " + pyramid.get(0).center);
AztecCode marker = allMarkers.grow();
if (decoder.process(pyramid, gray, marker)) {
detected.add(marker);
} else {
failed.add(marker);
}
}
}
@Override public List getDetections() {return detected;}
@Override public List getFailures() {return failed;}
@Override public Class getImageType() {return imageType;}
@Override public void setVerbose( @Nullable PrintStream out, @Nullable Set configuration ) {
this.verbose = BoofMiscOps.addPrefix(this, out);
BoofMiscOps.verboseChildren(out, configuration, decoder, detectorPyramids);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy