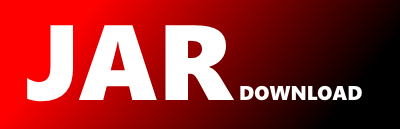
org.bouncycastle.crypto.fips.Utils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bc-fips-debug Show documentation
Show all versions of bc-fips-debug Show documentation
The FIPS 140-2 Bouncy Castle Crypto package is a Java implementation of cryptographic algorithms certified to FIPS 140-2 level 1. This jar contains the debug version JCE provider and low-level API for the BC-FJA version 1.0.2.3, FIPS Certificate #3514. Please note the debug jar is not certified.
package org.bouncycastle.crypto.fips;
import java.security.AccessController;
import java.security.Permission;
import java.security.PrivilegedAction;
import java.security.Provider;
import java.security.SecureRandom;
import java.security.SecureRandomSpi;
import java.util.concurrent.atomic.AtomicLong;
import java.util.logging.Logger;
import org.bouncycastle.crypto.Algorithm;
import org.bouncycastle.crypto.CryptoServicesRegistrar;
import org.bouncycastle.crypto.internal.ValidatedSymmetricKey;
import org.bouncycastle.crypto.internal.params.AEADParameters;
import org.bouncycastle.crypto.internal.params.KeyParameter;
import org.bouncycastle.crypto.internal.params.KeyParameterImpl;
import org.bouncycastle.crypto.internal.params.ParametersWithIV;
import org.bouncycastle.jcajce.provider.BouncyCastleFipsProvider;
import org.bouncycastle.math.ec.ECCurve;
import org.bouncycastle.util.Pack;
import org.bouncycastle.util.Properties;
class Utils
{
// DRBG used by KAT tests
static final SecureRandom testRandom = new TestSecureRandom();
private static class TestSecureRandom
extends SecureRandom
{
public TestSecureRandom()
{
super(new RandomSpi(), new RandomProvider());
}
private static class RandomSpi
extends SecureRandomSpi
{
private final AtomicLong counter = new AtomicLong(System.currentTimeMillis());
@Override
protected void engineSetSeed(byte[] bytes)
{
// ignore
}
@Override
protected void engineNextBytes(byte[] bytes)
{
SHA256Digest digest = new SHA256Digest();
byte[] digestBuf = new byte[digest.getDigestSize()];
byte[] counterBytes = new byte[8];
int required = bytes.length;
int offset = 0;
while (required > 0)
{
Pack.longToBigEndian(counter.getAndIncrement(), counterBytes, 0);
digest.update(counterBytes, 0, counterBytes.length);
digest.doFinal(digestBuf, 0);
if (required > digestBuf.length)
{
System.arraycopy(digestBuf, 0, bytes, offset, digestBuf.length);
}
else
{
System.arraycopy(digestBuf, 0, bytes, offset, required);
}
offset += digestBuf.length;
required -= digestBuf.length;
}
}
@Override
protected byte[] engineGenerateSeed(int numBytes)
{
byte[] rv = new byte[numBytes];
engineNextBytes(rv);
return rv;
}
}
private static class RandomProvider
extends Provider
{
RandomProvider()
{
super("BCFIPS_TEST_RNG", 1.0, "BCFIPS Test Secure Random Provider");
}
}
}
static void validateRandom(SecureRandom random, String message)
{
if (!(random instanceof FipsSecureRandom) && !(random.getProvider() instanceof BouncyCastleFipsProvider))
{
throw new FipsUnapprovedOperationError(message);
}
}
static void validateRandom(SecureRandom random, FipsAlgorithm algorithm, String message)
{
if (!(random instanceof FipsSecureRandom) && !(random.getProvider() instanceof BouncyCastleFipsProvider))
{
throw new FipsUnapprovedOperationError(message, algorithm);
}
}
static void validateRandom(SecureRandom random, int securityStrength, FipsAlgorithm algorithm, String message)
{
if (random instanceof FipsSecureRandom)
{
if (((FipsSecureRandom)random).getSecurityStrength() < securityStrength)
{
throw new FipsUnapprovedOperationError("FIPS SecureRandom security strength not as high as required for operation", algorithm);
}
}
else if (random.getProvider() instanceof BouncyCastleFipsProvider)
{
if (((BouncyCastleFipsProvider)random.getProvider()).getDefaultRandomSecurityStrength() < securityStrength)
{
throw new FipsUnapprovedOperationError("FIPS SecureRandom security strength not as high as required for operation", algorithm);
}
}
else
{
throw new FipsUnapprovedOperationError(message, algorithm);
}
}
static void validateKeyGenRandom(SecureRandom random, int securityStrength, FipsAlgorithm algorithm)
{
validateRandom(random, securityStrength, algorithm, "Attempt to create key with unapproved RNG");
}
static void validateKeyPairGenRandom(SecureRandom random, int securityStrength, FipsAlgorithm algorithm)
{
validateRandom(random, securityStrength, algorithm, "Attempt to create key pair with unapproved RNG");
}
static void checkPermission(final Permission permission)
{
final SecurityManager securityManager = System.getSecurityManager();
if (securityManager != null)
{
AccessController.doPrivileged(new PrivilegedAction
© 2015 - 2025 Weber Informatics LLC | Privacy Policy