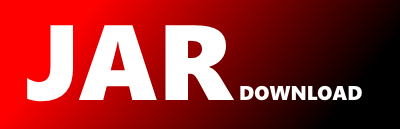
org.bouncycastle.crypto.general.Utils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bc-fips-debug Show documentation
Show all versions of bc-fips-debug Show documentation
The FIPS 140-2 Bouncy Castle Crypto package is a Java implementation of cryptographic algorithms certified to FIPS 140-2 level 1. This jar contains the debug version JCE provider and low-level API for the BC-FJA version 1.0.2.3, FIPS Certificate #3514. Please note the debug jar is not certified.
package org.bouncycastle.crypto.general;
import java.security.SecureRandom;
import org.bouncycastle.crypto.Algorithm;
import org.bouncycastle.crypto.CryptoServicesRegistrar;
import org.bouncycastle.crypto.IllegalKeyException;
import org.bouncycastle.crypto.fips.FipsUnapprovedOperationError;
import org.bouncycastle.crypto.internal.ValidatedSymmetricKey;
import org.bouncycastle.crypto.internal.params.KeyParameter;
import org.bouncycastle.crypto.internal.params.KeyParameterImpl;
class Utils
{
static final SecureRandom testRandom = new SecureRandom();
static void approveModeCheck(Algorithm algorithm)
{
if (CryptoServicesRegistrar.isInApprovedOnlyMode())
{
throw new FipsUnapprovedOperationError("Attempt to use unapproved algorithm in approved only mode", algorithm);
}
}
static KeyParameter getKeyParameter(ValidatedSymmetricKey sKey)
{
return new KeyParameterImpl(sKey.getKeyBytes());
}
static void checkKeyAlgorithm(ValidatedSymmetricKey key, Algorithm generalAlgorithm, Algorithm paramAlgorithm)
{
Algorithm keyAlgorithm = key.getAlgorithm();
if (!keyAlgorithm.equals(generalAlgorithm))
{
if (!keyAlgorithm.equals(paramAlgorithm))
{
throw new IllegalKeyException("Key not for appropriate algorithm");
}
}
}
static int bitsToBytes(int bits)
{
return (bits + 7) / 8;
}
static int getDefaultMacSize(Algorithm algorithm, int blockSize)
{
if (algorithm.getName().endsWith("GMAC") || algorithm.getName().endsWith("/CMAC")
|| algorithm.getName().endsWith("GCM") || algorithm.getName().endsWith("OCB")
|| algorithm.getName().endsWith("ISO979ALG3"))
{
return blockSize;
}
return blockSize / 2;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy