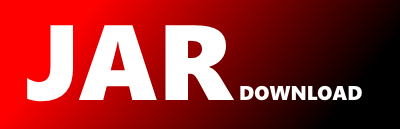
org.bouncycastle.bcpg.AEADUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bcpg-jdk14 Show documentation
Show all versions of bcpg-jdk14 Show documentation
The Bouncy Castle Java API for handling the OpenPGP protocol. This jar contains the OpenPGP API for JDK 1.4. The APIs can be used in conjunction with a JCE/JCA provider such as the one provided with the Bouncy Castle Cryptography APIs.
The newest version!
package org.bouncycastle.bcpg;
public class AEADUtils
implements AEADAlgorithmTags
{
private AEADUtils()
{
}
/**
* Return the length of the IV used by the given AEAD algorithm in octets.
*
* @param aeadAlgorithmTag AEAD algorithm identifier
* @return length of the IV
*/
public static int getIVLength(int aeadAlgorithmTag)
{
switch (aeadAlgorithmTag)
{
case EAX:
return 16;
case OCB:
return 15;
case GCM:
return 12;
default:
throw new IllegalArgumentException("Invalid AEAD algorithm tag: " + aeadAlgorithmTag);
}
}
/**
* Return the length of the authentication tag used by the given AEAD algorithm in octets.
*
* @param aeadAlgorithmTag AEAD algorithm identifier
* @return length of the auth tag
*/
public static int getAuthTagLength(int aeadAlgorithmTag)
{
switch (aeadAlgorithmTag)
{
case EAX:
case OCB:
case GCM:
return 16;
default:
throw new IllegalArgumentException("Invalid AEAD algorithm tag: " + aeadAlgorithmTag);
}
}
/**
* Split a given byte array containing m
bytes of key and n-8 bytes of IV into
* two separate byte arrays.
* m is the key length of the cipher algorithm, while n is the IV length of the AEAD algorithm.
* Note, that the IV is filled with n-8 bytes only, the remainder is left as 0s.
* Return an array of both arrays with the key and index 0 and the IV at index 1.
*
* @param messageKeyAndIv m+n-8 bytes of concatenated message key and IV
* @param cipherAlgo symmetric cipher algorithm
* @param aeadAlgo AEAD algorithm
* @return array of arrays containing message key and IV
*/
public static byte[][] splitMessageKeyAndIv(byte[] messageKeyAndIv, int cipherAlgo, int aeadAlgo)
{
int keyLen = SymmetricKeyUtils.getKeyLengthInOctets(cipherAlgo);
int ivLen = AEADUtils.getIVLength(aeadAlgo);
byte[] messageKey = new byte[keyLen];
byte[] iv = new byte[ivLen];
System.arraycopy(messageKeyAndIv, 0, messageKey, 0, messageKey.length);
System.arraycopy(messageKeyAndIv, messageKey.length, iv, 0, ivLen - 8);
return new byte[][]{messageKey, iv};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy