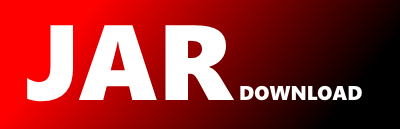
org.bouncycastle.openpgp.operator.PGPKeyConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bcpg-jdk14 Show documentation
Show all versions of bcpg-jdk14 Show documentation
The Bouncy Castle Java API for handling the OpenPGP protocol. This jar contains the OpenPGP API for JDK 1.4. The APIs can be used in conjunction with a JCE/JCA provider such as the one provided with the Bouncy Castle Cryptography APIs.
The newest version!
package org.bouncycastle.openpgp.operator;
import java.io.IOException;
import java.math.BigInteger;
import org.bouncycastle.asn1.ASN1ObjectIdentifier;
import org.bouncycastle.asn1.DEROctetString;
import org.bouncycastle.asn1.cryptlib.CryptlibObjectIdentifiers;
import org.bouncycastle.asn1.edec.EdECObjectIdentifiers;
import org.bouncycastle.asn1.pkcs.PrivateKeyInfo;
import org.bouncycastle.asn1.sec.SECObjectIdentifiers;
import org.bouncycastle.asn1.teletrust.TeleTrusTObjectIdentifiers;
import org.bouncycastle.asn1.x509.AlgorithmIdentifier;
import org.bouncycastle.bcpg.HashAlgorithmTags;
import org.bouncycastle.bcpg.SymmetricKeyAlgorithmTags;
import org.bouncycastle.openpgp.PGPAlgorithmParameters;
import org.bouncycastle.openpgp.PGPKdfParameters;
import org.bouncycastle.util.BigIntegers;
public abstract class PGPKeyConverter
{
protected PGPKeyConverter()
{
}
/**
* Reference:
* RFC9580 - OpenPGP
*
* This class provides information about the recommended algorithms to use
* depending on the key version and curve type in OpenPGP keys.
*
*
* For OpenPGP keys using the specified curves, the following algorithms are recommended:
*
* Recommended Algorithms for OpenPGP Keys
*
* Curve
* Hash Algorithm
* Symmetric Algorithm
*
*
* NIST P-256
* SHA2-256
* AES-128
*
*
* NIST P-384
* SHA2-384
* AES-192
*
*
* NIST P-521
* SHA2-512
* AES-256
*
*
* brainpoolP256r1
* SHA2-256
* AES-128
*
*
* brainpoolP384r1
* SHA2-384
* AES-192
*
*
* brainpoolP512r1
* SHA2-512
* AES-256
*
*
* Curve25519Legacy
* SHA2-256
* AES-128
*
*
* Curve448Legacy (not in RFC Draft)
* SHA2-512
* AES-256
*
*
*/
protected PGPKdfParameters implGetKdfParameters(ASN1ObjectIdentifier curveID, PGPAlgorithmParameters algorithmParameters)
{
if (null == algorithmParameters)
{
if (curveID.equals(SECObjectIdentifiers.secp256r1) || curveID.equals(TeleTrusTObjectIdentifiers.brainpoolP256r1)
|| curveID.equals(CryptlibObjectIdentifiers.curvey25519) || curveID.equals(EdECObjectIdentifiers.id_X25519))
{
return new PGPKdfParameters(HashAlgorithmTags.SHA256, SymmetricKeyAlgorithmTags.AES_128);
}
else if (curveID.equals(SECObjectIdentifiers.secp384r1) || curveID.equals(TeleTrusTObjectIdentifiers.brainpoolP384r1))
{
return new PGPKdfParameters(HashAlgorithmTags.SHA384, SymmetricKeyAlgorithmTags.AES_192);
}
else if (curveID.equals(SECObjectIdentifiers.secp521r1) || curveID.equals(TeleTrusTObjectIdentifiers.brainpoolP512r1)
|| curveID.equals(EdECObjectIdentifiers.id_X448))
{
return new PGPKdfParameters(HashAlgorithmTags.SHA512, SymmetricKeyAlgorithmTags.AES_256);
}
else
{
throw new IllegalArgumentException("unknown curve");
}
}
return (PGPKdfParameters)algorithmParameters;
}
protected PrivateKeyInfo getPrivateKeyInfo(ASN1ObjectIdentifier algorithm, int keySize, byte[] key)
throws IOException
{
return (new PrivateKeyInfo(new AlgorithmIdentifier(algorithm),
new DEROctetString(BigIntegers.asUnsignedByteArray(keySize, new BigInteger(1, key)))));
}
protected PrivateKeyInfo getPrivateKeyInfo(ASN1ObjectIdentifier algorithm, byte[] key)
throws IOException
{
return (new PrivateKeyInfo(new AlgorithmIdentifier(algorithm), new DEROctetString(key)));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy