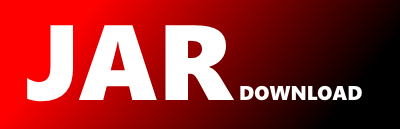
org.bouncycastle.openpgp.ExtendedPGPSecretKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bcpg-lts8on Show documentation
Show all versions of bcpg-lts8on Show documentation
The Bouncy Castle Java APIs for the OpenPGP Protocol. The APIs are designed primarily to be used in conjunction with the BC LTS provider but may also be used with other providers providing cryptographic services.
The newest version!
package org.bouncycastle.openpgp;
import java.util.Collections;
import java.util.List;
import org.bouncycastle.bcpg.SecretKeyPacket;
import org.bouncycastle.openpgp.operator.PBESecretKeyEncryptor;
import org.bouncycastle.openpgp.operator.PGPContentSignerBuilder;
import org.bouncycastle.openpgp.operator.PGPDigestCalculator;
public class ExtendedPGPSecretKey
extends PGPSecretKey
{
private final List headers;
private final List attributes;
public ExtendedPGPSecretKey(List headerList, List attributes, SecretKeyPacket secret, PGPPublicKey pub)
{
super(secret, pub);
headers = Collections.unmodifiableList(headerList);
this.attributes = Collections.unmodifiableList(attributes);
}
ExtendedPGPSecretKey(List headerList, List attributes, PGPPrivateKey privKey, PGPPublicKey pubKey, PGPDigestCalculator checksumCalculator, PBESecretKeyEncryptor keyEncryptor)
throws PGPException
{
super(privKey, pubKey, checksumCalculator, keyEncryptor);
headers = Collections.unmodifiableList(headerList);
this.attributes = Collections.unmodifiableList(attributes);
}
public ExtendedPGPSecretKey(List headerList, List attributes, PGPPrivateKey privKey, PGPPublicKey pubKey, PGPDigestCalculator checksumCalculator, boolean isMasterKey, PBESecretKeyEncryptor keyEncryptor)
throws PGPException
{
super(privKey, pubKey, checksumCalculator, isMasterKey, keyEncryptor);
headers = Collections.unmodifiableList(headerList);
this.attributes = Collections.unmodifiableList(attributes);
}
public ExtendedPGPSecretKey(List headerList, List attributes, int certificationLevel, PGPKeyPair keyPair, String id, PGPSignatureSubpacketVector hashedPcks, PGPSignatureSubpacketVector unhashedPcks, PGPContentSignerBuilder certificationSignerBuilder, PBESecretKeyEncryptor keyEncryptor)
throws PGPException
{
super(certificationLevel, keyPair, id, hashedPcks, unhashedPcks, certificationSignerBuilder, keyEncryptor);
headers = Collections.unmodifiableList(headerList);
this.attributes = Collections.unmodifiableList(attributes);
}
public ExtendedPGPSecretKey(List headerList, List attributes, PGPKeyPair masterKeyPair, PGPKeyPair keyPair, PGPDigestCalculator checksumCalculator, PGPContentSignerBuilder certificationSignerBuilder, PBESecretKeyEncryptor keyEncryptor)
throws PGPException
{
super(masterKeyPair, keyPair, checksumCalculator, certificationSignerBuilder, keyEncryptor);
headers = Collections.unmodifiableList(headerList);
this.attributes = Collections.unmodifiableList(attributes);
}
public ExtendedPGPSecretKey(List headerList, List attributes, PGPKeyPair masterKeyPair, PGPKeyPair keyPair, PGPDigestCalculator checksumCalculator, PGPSignatureSubpacketVector hashedPcks, PGPSignatureSubpacketVector unhashedPcks, PGPContentSignerBuilder certificationSignerBuilder, PBESecretKeyEncryptor keyEncryptor)
throws PGPException
{
super(masterKeyPair, keyPair, checksumCalculator, hashedPcks, unhashedPcks, certificationSignerBuilder, keyEncryptor);
headers = Collections.unmodifiableList(headerList);
this.attributes = Collections.unmodifiableList(attributes);
}
public ExtendedPGPSecretKey(List headerList, List attributes, int certificationLevel, PGPKeyPair keyPair, String id, PGPDigestCalculator checksumCalculator, PGPSignatureSubpacketVector hashedPcks, PGPSignatureSubpacketVector unhashedPcks, PGPContentSignerBuilder certificationSignerBuilder, PBESecretKeyEncryptor keyEncryptor)
throws PGPException
{
super(certificationLevel, keyPair, id, checksumCalculator, hashedPcks, unhashedPcks, certificationSignerBuilder, keyEncryptor);
headers = Collections.unmodifiableList(headerList);
this.attributes = Collections.unmodifiableList(attributes);
}
public List getHeaders()
{
return headers;
}
public List getAttributes()
{
return attributes;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy