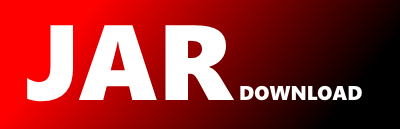
org.bouncycastle.pqc.legacy.math.linearalgebra.Matrix Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bcprov-ext-debug-jdk18on Show documentation
Show all versions of bcprov-ext-debug-jdk18on Show documentation
The Bouncy Castle Crypto package is a Java implementation of cryptographic algorithms. This jar contains JCE provider and lightweight API for the Bouncy Castle Cryptography APIs for JDK 1.8 and up. Note: this package includes the NTRU encryption algorithms.
The newest version!
package org.bouncycastle.pqc.legacy.math.linearalgebra;
/**
* This abstract class defines matrices. It holds the number of rows and the
* number of columns of the matrix and defines some basic methods.
*/
public abstract class Matrix
{
/**
* number of rows
*/
protected int numRows;
/**
* number of columns
*/
protected int numColumns;
// ----------------------------------------------------
// some constants (matrix types)
// ----------------------------------------------------
/**
* zero matrix
*/
public static final char MATRIX_TYPE_ZERO = 'Z';
/**
* unit matrix
*/
public static final char MATRIX_TYPE_UNIT = 'I';
/**
* random lower triangular matrix
*/
public static final char MATRIX_TYPE_RANDOM_LT = 'L';
/**
* random upper triangular matrix
*/
public static final char MATRIX_TYPE_RANDOM_UT = 'U';
/**
* random regular matrix
*/
public static final char MATRIX_TYPE_RANDOM_REGULAR = 'R';
// ----------------------------------------------------
// getters
// ----------------------------------------------------
/**
* @return the number of rows in the matrix
*/
public int getNumRows()
{
return numRows;
}
/**
* @return the number of columns in the binary matrix
*/
public int getNumColumns()
{
return numColumns;
}
/**
* @return the encoded matrix, i.e., this matrix in byte array form.
*/
public abstract byte[] getEncoded();
// ----------------------------------------------------
// arithmetic
// ----------------------------------------------------
/**
* Compute the inverse of this matrix.
*
* @return the inverse of this matrix (newly created).
*/
public abstract Matrix computeInverse();
/**
* Check if this is the zero matrix (i.e., all entries are zero).
*
* @return true if this is the zero matrix
*/
public abstract boolean isZero();
/**
* Compute the product of this matrix and another matrix.
*
* @param a the other matrix
* @return this * a (newly created)
*/
public abstract Matrix rightMultiply(Matrix a);
/**
* Compute the product of this matrix and a permutation.
*
* @param p the permutation
* @return this * p (newly created)
*/
public abstract Matrix rightMultiply(Permutation p);
/**
* Compute the product of a vector and this matrix. If the length of the
* vector is greater than the number of rows of this matrix, the matrix is
* multiplied by each m-bit part of the vector.
*
* @param vector a vector
* @return vector * this (newly created)
*/
public abstract Vector leftMultiply(Vector vector);
/**
* Compute the product of this matrix and a vector.
*
* @param vector a vector
* @return this * vector (newly created)
*/
public abstract Vector rightMultiply(Vector vector);
/**
* @return a human readable form of the matrix.
*/
public abstract String toString();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy